在Python中判断x是否比y大,可以使用大于运算符(>)。 通过使用比较运算符,我们可以直接比较两个值,并根据结果做出相应的操作。比较运算符是Python中的一种常见运算符,用于比较两个值并返回布尔值(True或False)。在判断x是否比y大时,我们使用大于运算符(>)进行比较。如果x大于y,则表达式x > y将返回True,否则返回False。下面我们将详细探讨这个过程,并举例说明如何在不同情况下使用大于运算符进行比较。
一、基本用法
在Python中,大于运算符(>)是最基本的比较运算符之一。它用于比较两个数值(包括整数和浮点数),并返回布尔值。通过使用大于运算符,我们可以简单地判断一个数是否大于另一个数。例如:
x = 10
y = 5
if x > y:
print("x is greater than y")
else:
print("x is not greater than y")
在上面的代码中,变量x的值为10,变量y的值为5。通过使用大于运算符进行比较,程序会输出"x is greater than y",因为10大于5。
二、比较字符串
在Python中,除了比较数值之外,我们还可以使用大于运算符比较字符串。字符串比较基于字典序,即字符在字母表中的顺序。例如:
str1 = "apple"
str2 = "banana"
if str1 > str2:
print("str1 is greater than str2")
else:
print("str1 is not greater than str2")
在上面的代码中,变量str1的值为"apple",变量str2的值为"banana"。虽然"apple"在字母表中排在"banana"之前,但由于字母'a'的ASCII码值比字母'b'小,比较结果将为False,程序会输出"str1 is not greater than str2"。
三、比较列表和元组
在Python中,我们还可以使用大于运算符比较列表和元组。列表和元组的比较是基于字典序的,即从第一个元素开始逐个比较,直到找到不同的元素为止。例如:
list1 = [1, 2, 3]
list2 = [1, 2, 4]
if list1 > list2:
print("list1 is greater than list2")
else:
print("list1 is not greater than list2")
在上面的代码中,变量list1的值为[1, 2, 3],变量list2的值为[1, 2, 4]。由于列表中的第一个和第二个元素相同,而第三个元素3小于4,比较结果将为False,程序会输出"list1 is not greater than list2"。
四、自定义对象的比较
在Python中,我们还可以通过定义自定义类和实现比较运算符的方法来比较自定义对象。例如:
class Person:
def __init__(self, age):
self.age = age
def __gt__(self, other):
return self.age > other.age
person1 = Person(30)
person2 = Person(25)
if person1 > person2:
print("person1 is older than person2")
else:
print("person1 is not older than person2")
在上面的代码中,我们定义了一个Person类,并通过实现__gt__方法来重载大于运算符。变量person1的age属性为30,变量person2的age属性为25。通过使用大于运算符进行比较,程序会输出"person1 is older than person2",因为30大于25。
五、在函数中使用比较运算符
在Python中,我们还可以在函数中使用大于运算符进行比较。例如:
def compare(x, y):
if x > y:
return "x is greater than y"
else:
return "x is not greater than y"
result = compare(10, 5)
print(result)
在上面的代码中,我们定义了一个compare函数,该函数接受两个参数x和y,并使用大于运算符进行比较。通过调用compare函数并传递参数10和5,程序会输出"x is greater than y",因为10大于5。
六、使用三元表达式进行比较
在Python中,我们还可以使用三元表达式进行比较。三元表达式是一种简洁的条件表达式,用于根据条件返回不同的值。例如:
x = 10
y = 5
result = "x is greater than y" if x > y else "x is not greater than y"
print(result)
在上面的代码中,我们使用三元表达式进行比较。如果x大于y,则result变量的值为"x is greater than y";否则,result变量的值为"x is not greater than y"。最终程序会输出"x is greater than y",因为10大于5。
七、比较浮点数
在Python中,除了比较整数和字符串之外,我们还可以使用大于运算符比较浮点数。例如:
x = 10.5
y = 5.3
if x > y:
print("x is greater than y")
else:
print("x is not greater than y")
在上面的代码中,变量x的值为10.5,变量y的值为5.3。通过使用大于运算符进行比较,程序会输出"x is greater than y",因为10.5大于5.3。
八、比较时间和日期
在Python中,我们还可以使用大于运算符比较时间和日期。例如:
from datetime import datetime
date1 = datetime(2023, 1, 1)
date2 = datetime(2022, 1, 1)
if date1 > date2:
print("date1 is after date2")
else:
print("date1 is not after date2")
在上面的代码中,我们使用datetime模块创建了两个日期对象date1和date2。通过使用大于运算符进行比较,程序会输出"date1 is after date2",因为2023年1月1日比2022年1月1日晚。
九、比较集合
在Python中,我们还可以使用大于运算符比较集合。例如:
set1 = {1, 2, 3}
set2 = {1, 2}
if set1 > set2:
print("set1 is a proper superset of set2")
else:
print("set1 is not a proper superset of set2")
在上面的代码中,变量set1的值为{1, 2, 3},变量set2的值为{1, 2}。通过使用大于运算符进行比较,程序会输出"set1 is a proper superset of set2",因为set1包含set2的所有元素,并且set1有额外的元素3。
十、使用断言进行比较
在Python中,我们还可以使用断言进行比较。断言是一种调试工具,用于在代码中插入检查语句。例如:
x = 10
y = 5
assert x > y, "x is not greater than y"
print("x is greater than y")
在上面的代码中,我们使用断言检查x是否大于y。如果x不大于y,程序会抛出AssertionError异常,并输出错误消息"x is not greater than y"。否则,程序会继续执行,并输出"x is greater than y"。
十一、比较自定义类的实例
在Python中,我们还可以通过定义自定义类,并实现比较运算符的方法,来比较自定义类的实例。例如:
class Rectangle:
def __init__(self, width, height):
self.width = width
self.height = height
def area(self):
return self.width * self.height
def __gt__(self, other):
return self.area() > other.area()
rect1 = Rectangle(5, 10)
rect2 = Rectangle(4, 9)
if rect1 > rect2:
print("rect1 has a greater area than rect2")
else:
print("rect1 does not have a greater area than rect2")
在上面的代码中,我们定义了一个Rectangle类,并通过实现__gt__方法来重载大于运算符。变量rect1的宽度和高度分别为5和10,变量rect2的宽度和高度分别为4和9。通过使用大于运算符进行比较,程序会输出"rect1 has a greater area than rect2",因为rect1的面积(510=50)大于rect2的面积(49=36)。
十二、使用NumPy数组进行比较
在Python中,我们还可以使用NumPy数组进行比较。例如:
import numpy as np
arr1 = np.array([1, 2, 3])
arr2 = np.array([1, 2, 4])
if np.all(arr1 > arr2):
print("All elements in arr1 are greater than arr2")
else:
print("Not all elements in arr1 are greater than arr2")
在上面的代码中,我们使用NumPy库创建了两个数组arr1和arr2。通过使用大于运算符进行比较,并使用np.all函数检查所有元素是否满足条件,程序会输出"Not all elements in arr1 are greater than arr2",因为arr1的第三个元素3小于arr2的第三个元素4。
十三、比较嵌套列表
在Python中,我们还可以使用大于运算符比较嵌套列表。例如:
list1 = [[1, 2], [3, 4]]
list2 = [[1, 2], [3, 5]]
if list1 > list2:
print("list1 is greater than list2")
else:
print("list1 is not greater than list2")
在上面的代码中,变量list1的值为[[1, 2], [3, 4]],变量list2的值为[[1, 2], [3, 5]]。通过使用大于运算符进行比较,程序会输出"list1 is not greater than list2",因为嵌套列表中的第二个子列表[3, 4]的第二个元素4小于[3, 5]的第二个元素5。
十四、比较字典的值
在Python中,我们还可以使用大于运算符比较字典的值。例如:
dict1 = {'a': 10, 'b': 20}
dict2 = {'a': 10, 'b': 15}
if dict1['b'] > dict2['b']:
print("dict1 has a greater value for key 'b' than dict2")
else:
print("dict1 does not have a greater value for key 'b' than dict2")
在上面的代码中,变量dict1的值为{'a': 10, 'b': 20},变量dict2的值为{'a': 10, 'b': 15}。通过使用大于运算符比较字典中key为'b'的值,程序会输出"dict1 has a greater value for key 'b' than dict2",因为dict1中key为'b'的值20大于dict2中key为'b'的值15。
十五、比较复数的实部和虚部
在Python中,我们不能直接比较复数,但可以比较复数的实部和虚部。例如:
x = 3 + 4j
y = 1 + 2j
if x.real > y.real:
print("x has a greater real part than y")
else:
print("x does not have a greater real part than y")
if x.imag > y.imag:
print("x has a greater imaginary part than y")
else:
print("x does not have a greater imaginary part than y")
在上面的代码中,变量x的值为3 + 4j,变量y的值为1 + 2j。通过使用大于运算符比较复数的实部和虚部,程序会分别输出"x has a greater real part than y"和"x has a greater imaginary part than y",因为x的实部3大于y的实部1,x的虚部4大于y的虚部2。
十六、比较不同类型的数据
在Python中,我们可以比较不同类型的数据,但需要注意类型转换。例如:
x = 10
y = "5"
if x > int(y):
print("x is greater than y")
else:
print("x is not greater than y")
在上面的代码中,变量x的值为10,变量y的值为字符串"5"。通过将y转换为整数并使用大于运算符进行比较,程序会输出"x is greater than y",因为10大于5。
十七、在列表推导式中使用比较运算符
在Python中,我们还可以在列表推导式中使用大于运算符进行比较。例如:
numbers = [10, 20, 30, 40, 50]
threshold = 25
greater_numbers = [num for num in numbers if num > threshold]
print(greater_numbers)
在上面的代码中,我们定义了一个包含数值的列表numbers和一个阈值threshold。通过在列表推导式中使用大于运算符进行比较,我们创建了一个新的列表greater_numbers,其中包含所有大于阈值的数值。最终程序会输出"[30, 40, 50]",因为这些数值都大于25。
十八、在生成器表达式中使用比较运算符
在Python中,我们还可以在生成器表达式中使用大于运算符进行比较。例如:
numbers = [10, 20, 30, 40, 50]
threshold = 25
greater_numbers = (num for num in numbers if num > threshold)
for num in greater_numbers:
print(num)
在上面的代码中,我们定义了一个包含数值的列表numbers和一个阈值threshold。通过在生成器表达式中使用大于运算符进行比较,我们创建了一个生成器greater_numbers,其中包含所有大于阈值的数值。通过迭代生成器并打印每个数值,程序会输出"30"、"40"和"50",因为这些数值都大于25。
十九、在过滤器中使用比较运算符
在Python中,我们还可以在过滤器中使用大于运算符进行比较。例如:
numbers = [10, 20, 30, 40, 50]
threshold = 25
greater_numbers = filter(lambda num: num > threshold, numbers)
for num in greater_numbers:
print(num)
在上面的代码中,我们定义了一个包含数值的列表numbers和一个阈值threshold。通过在过滤器中使用大于运算符进行比较,我们创建了一个过滤器greater_numbers,其中包含所有大于阈值的数值。通过迭代过滤器并打印每个数值,程序会输出"30"、"40"和"50",因为这些数值都大于25。
二十、在排序中使用比较运算符
在Python中,我们还可以在排序中使用大于运算符进行比较。例如:
numbers = [50, 10, 40, 20, 30]
sorted_numbers = sorted(numbers, reverse=True)
print(sorted_numbers)
在上面的代码中,我们定义了一个包含数值的列表numbers。通过使用sorted函数并设置参数reverse=True,我们可以对列表进行降序排序。最终程序会输出"[50, 40, 30, 20, 10]",因为这些数值按从大到小的顺序排列。
通过上述各种方法,我们可以在Python中判断x是否比y大。无论是基本的数值比较、字符串比较,还是自定义对象和集合的比较,Python提供了丰富的工具和方法来满足不同场景的需求。通过灵活运用这些方法,我们可以在编写代码时更好地处理各种比较操作,提高代码的健壮性和可读性。
相关问答FAQs:
如何在Python中比较两个变量的大小?
在Python中,可以使用比较运算符“>”来判断一个变量是否大于另一个变量。例如,若要判断x是否大于y,可以使用以下代码:if x > y:
。如果条件成立,后续的代码块将被执行。
如果需要比较多个变量的大小,应该怎么做?
在Python中,可以通过链式比较来同时判断多个变量的大小。例如,要判断x是否大于y且y又大于z,可以使用if x > y > z:
。这种方式不仅简洁,还能提高代码的可读性。
在进行大小比较时,如何处理不同数据类型的变量?
在Python中,比较不同数据类型的变量时,需要注意类型的兼容性。例如,整数和浮点数可以直接比较,但字符串和数字之间的比较会引发类型错误。为了避免这种情况,建议在比较前使用type()
函数检查变量类型,或使用类型转换函数如int()
或float()
确保比较的有效性。
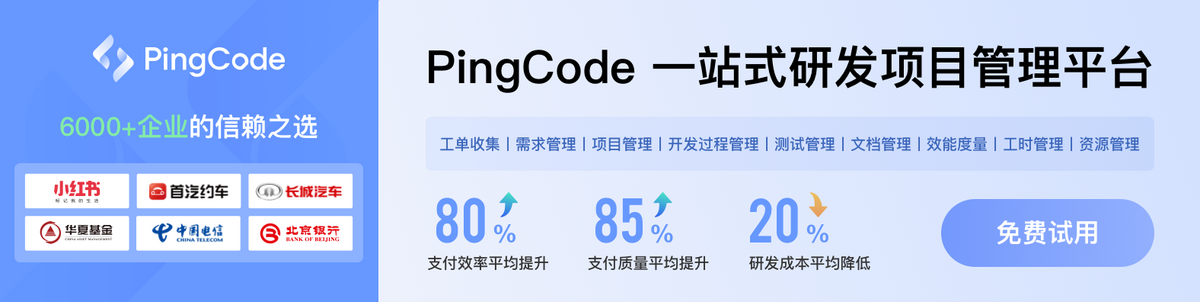