在Python中使用正则表达式,可以通过导入re
模块来实现。 正则表达式(Regular Expressions,简称RE)是一种用来匹配字符串的强大工具,主要用于字符串的搜索、匹配和替换。常用的操作有:匹配字符、捕获组、搜索和替换、预编译正则表达式。其中,匹配字符是最基本的功能,使用广泛。通过详细描述这些操作,可以帮助你更好地理解和使用正则表达式。
一、导入re模块
在使用正则表达式之前,需要先导入Python的re
模块。这个模块包含了所有你需要的正则表达式功能。
import re
二、匹配字符
匹配字符是正则表达式最基本的功能。正则表达式通过特定的模式来匹配字符串中的字符。
1、基本字符匹配
最简单的正则表达式是字面字符匹配。下面的例子展示了如何使用正则表达式匹配字符串中的一个单词。
pattern = r'hello'
text = 'hello world'
match = re.search(pattern, text)
if match:
print('Match found:', match.group())
else:
print('No match')
在这个例子中,re.search()
函数搜索字符串text
中是否包含模式pattern
,如果找到匹配,则返回一个匹配对象,否则返回None
。
2、元字符匹配
元字符是正则表达式中的特殊字符,用于匹配特定类型的字符。常用的元字符包括.
(匹配任意字符)、^
(匹配字符串的开始)、$
(匹配字符串的结尾)等。
pattern = r'he..o'
text = 'hello'
match = re.search(pattern, text)
if match:
print('Match found:', match.group())
else:
print('No match')
在这个例子中,模式he..o
中的.
匹配任意字符,因此hello
与模式匹配。
三、捕获组
捕获组是正则表达式的一个重要功能,用于从匹配中提取子字符串。使用圆括号()
来定义捕获组。
pattern = r'(hello) (world)'
text = 'hello world'
match = re.search(pattern, text)
if match:
print('Match found:', match.group())
print('Group 1:', match.group(1))
print('Group 2:', match.group(2))
else:
print('No match')
在这个例子中,模式(hello) (world)
定义了两个捕获组,分别为hello
和world
。match.group(1)
和match.group(2)
分别返回第一个和第二个捕获组的内容。
四、搜索和替换
正则表达式还可以用于搜索和替换字符串中的内容。re.sub()
函数可以实现这个功能。
pattern = r'world'
text = 'hello world'
replacement = 'Python'
new_text = re.sub(pattern, replacement, text)
print('Original text:', text)
print('New text:', new_text)
在这个例子中,模式world
匹配字符串中的world
,并用Python
替换它。re.sub()
函数返回替换后的新字符串。
五、预编译正则表达式
为了提高效率,可以预编译正则表达式,然后在多个地方重复使用。使用re.compile()
函数可以实现预编译。
pattern = re.compile(r'hello')
text = 'hello world'
match = pattern.search(text)
if match:
print('Match found:', match.group())
else:
print('No match')
在这个例子中,正则表达式模式hello
被预编译,然后使用预编译的模式对象进行搜索。
六、正则表达式匹配模式
正则表达式可以通过设置匹配模式来改变其行为。常用的匹配模式包括re.IGNORECASE
(忽略大小写)、re.MULTILINE
(多行匹配)、re.DOTALL
(使.
匹配换行符)等。
pattern = re.compile(r'hello', re.IGNORECASE)
text = 'Hello world'
match = pattern.search(text)
if match:
print('Match found:', match.group())
else:
print('No match')
在这个例子中,匹配模式re.IGNORECASE
使得正则表达式匹配时忽略大小写,因此Hello
与模式hello
匹配。
七、常用正则表达式示例
1、匹配邮箱地址
pattern = r'[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}'
text = 'Contact us at support@example.com'
match = re.search(pattern, text)
if match:
print('Email found:', match.group())
else:
print('No email found')
2、匹配电话号码
pattern = r'\b\d{3}[-.]?\d{3}[-.]?\d{4}\b'
text = 'Call us at 123-456-7890 or 123.456.7890'
matches = re.findall(pattern, text)
for match in matches:
print('Phone number found:', match)
3、匹配URL
pattern = r'https?://(?:[-\w.]|(?:%[\da-fA-F]{2}))+'
text = 'Visit our website at https://www.example.com'
match = re.search(pattern, text)
if match:
print('URL found:', match.group())
else:
print('No URL found')
八、总结
通过以上示例和解释,相信你已经对Python中如何使用正则表达式有了更深入的了解。正则表达式是一个非常强大的工具,可以帮助你高效地进行字符串匹配、搜索和替换。在实际应用中,灵活运用正则表达式可以大大提高你的编程效率和代码质量。希望这些内容对你有所帮助,能够在你的Python编程中发挥更大的作用。
相关问答FAQs:
在Python中如何导入和使用正则表达式库?
在Python中,使用re
模块来处理正则表达式。首先,需要通过import re
导入该模块。之后,可以使用诸如re.match()
、re.search()
、re.findall()
和re.sub()
等函数来进行匹配、搜索和替换操作。这些函数提供了灵活的工具来处理字符串数据,满足不同的需求。
如何使用正则表达式进行字符串匹配?
正则表达式提供了强大的匹配功能,可以用于检查字符串是否符合特定格式。例如,re.match(pattern, string)
用于从字符串的开始位置匹配给定的模式。如果需要在字符串中查找模式,可以使用re.search(pattern, string)
,它会返回匹配的第一个位置。如果想要找到所有匹配的结果,可以使用re.findall(pattern, string)
,这个函数会返回一个列表,包含所有匹配的子串。
在Python中如何处理复杂的正则表达式?
处理复杂的正则表达式时,可以使用分组、选择和量词等特性。通过使用括号()
创建分组,可以提取特定的子串。选择符|
允许定义多种匹配条件,而量词如*
、+
和?
则控制字符出现的次数。此外,使用re.compile()
函数可以将正则表达式编译成一个模式对象,这样可以提高多次使用同一个表达式时的效率。
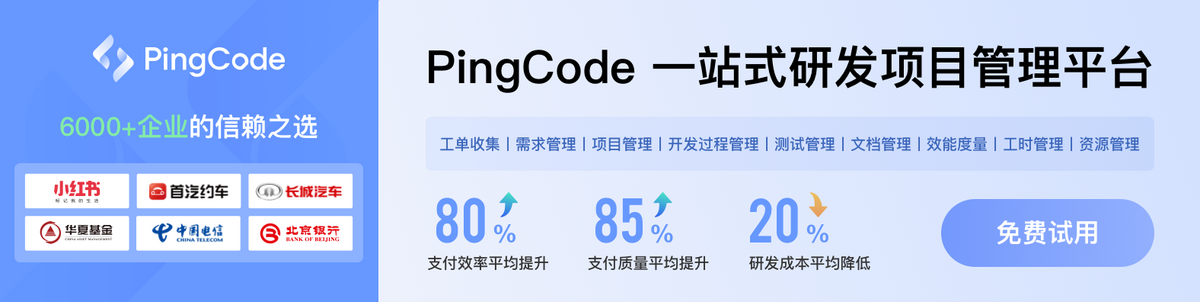