Python调用命令行程序的几种方法有:使用os.system()、subprocess模块、sh模块、os.popen()、pexpect等。其中,最常用和推荐的方法是使用subprocess模块,因为它提供了更强大的功能和更好的安全性。下面将详细介绍如何使用subprocess模块来调用命令行程序。
一、os.system()
os.system() 是最简单的方法之一。它可以直接调用命令行程序,并将命令的输出直接打印到标准输出中。但这种方法有一些局限性,比如无法获取命令的输出结果,也不能很好地处理复杂的命令。
import os
os.system("ls -l")
二、subprocess模块
subprocess模块是Python 3.x中推荐的调用命令行程序的方法。它提供了更多的功能,包括捕获命令输出、处理标准输入/输出/错误、设置超时等。
1、subprocess.run()
subprocess.run() 是最常用的函数,它可以执行命令并等待命令完成。它返回一个CompletedProcess实例,包含命令的返回码、标准输出、标准错误等信息。
import subprocess
result = subprocess.run(["ls", "-l"], capture_output=True, text=True)
print(result.stdout)
2、subprocess.Popen()
subprocess.Popen() 提供了更强大的功能,适用于需要与子进程进行复杂交互的场景。它允许我们手动管理子进程的输入、输出和错误管道。
import subprocess
process = subprocess.Popen(["ls", "-l"], stdout=subprocess.PIPE, stderr=subprocess.PIPE, text=True)
stdout, stderr = process.communicate()
print(stdout)
三、sh模块
sh模块是一个第三方库,提供了更高层次的API来调用命令行程序。它的语法更加简洁和直观,但需要额外安装。
import sh
print(sh.ls("-l"))
四、os.popen()
os.popen() 可以打开一个管道,从中读取命令的输出。虽然它功能简单,但已经被subprocess模块取代。
import os
output = os.popen("ls -l").read()
print(output)
五、pexpect
pexpect是一个用于自动化和控制交互式命令行程序的第三方库,适用于需要模拟用户输入的场景。
import pexpect
child = pexpect.spawn("ssh user@host")
child.expect("password:")
child.sendline("mypassword")
child.expect("$")
child.sendline("ls -l")
child.expect("$")
print(child.before.decode())
详细描述subprocess模块的使用
捕获命令输出
subprocess模块允许我们捕获命令的标准输出和标准错误。我们可以使用capture_output=True
或者显式设置stdout
和stderr
参数。
import subprocess
result = subprocess.run(["ls", "-l"], capture_output=True, text=True)
print("Standard Output:", result.stdout)
print("Standard Error:", result.stderr)
处理输入输出
我们可以通过设置stdin
、stdout
和stderr
参数来处理子进程的输入和输出。比如,我们可以将子进程的输出重定向到文件中。
import subprocess
with open("output.txt", "w") as f:
subprocess.run(["ls", "-l"], stdout=f)
设置超时
subprocess.run() 提供了一个timeout
参数,可以设置命令的超时时间。如果命令在指定时间内没有完成,将抛出subprocess.TimeoutExpired
异常。
import subprocess
try:
result = subprocess.run(["sleep", "10"], timeout=5)
except subprocess.TimeoutExpired:
print("Command timed out")
管道
subprocess模块允许我们创建管道,将一个命令的输出作为另一个命令的输入。我们可以使用Popen
类来实现这一点。
import subprocess
p1 = subprocess.Popen(["ls", "-l"], stdout=subprocess.PIPE)
p2 = subprocess.Popen(["grep", "py"], stdin=p1.stdout, stdout=subprocess.PIPE, text=True)
output, _ = p2.communicate()
print(output)
检查返回码
我们可以通过检查CompletedProcess
实例的returncode
属性来判断命令是否成功执行。返回码为0表示成功,非0表示失败。
import subprocess
result = subprocess.run(["ls", "-l"], capture_output=True, text=True)
if result.returncode == 0:
print("Command succeeded")
else:
print("Command failed with return code", result.returncode)
环境变量
我们可以通过设置env
参数来传递环境变量给子进程。它接受一个字典,其中键是环境变量的名称,值是环境变量的值。
import subprocess
import os
my_env = os.environ.copy()
my_env["MY_VAR"] = "my_value"
result = subprocess.run(["printenv", "MY_VAR"], capture_output=True, text=True, env=my_env)
print(result.stdout)
异常处理
subprocess模块会抛出几种异常,常见的包括subprocess.CalledProcessError
和subprocess.TimeoutExpired
。我们可以通过捕获这些异常来处理错误情况。
import subprocess
try:
result = subprocess.run(["ls", "-l", "/nonexistent"], capture_output=True, text=True, check=True)
except subprocess.CalledProcessError as e:
print("Command failed with error code", e.returncode)
print("Standard Error:", e.stderr)
使用shell
有时我们需要在shell中执行命令,比如使用管道或者重定向。这时可以设置shell=True
参数。但需要注意,使用shell=True
时可能存在安全风险,特别是当命令包含用户输入时。
import subprocess
result = subprocess.run("ls -l | grep py", shell=True, capture_output=True, text=True)
print(result.stdout)
总结
Python提供了多种调用命令行程序的方法,其中subprocess模块是最推荐的方式。它功能强大,能够满足大多数需求。通过合理使用subprocess模块,我们可以更好地控制和管理子进程,提高程序的健壮性和安全性。无论是简单的命令执行,还是复杂的管道和交互,subprocess模块都能提供有效的解决方案。通过对subprocess模块的详细了解和灵活运用,我们可以在Python程序中轻松调用各种命令行程序,提升开发效率。
相关问答FAQs:
如何在Python中调用外部命令行程序?
在Python中,可以使用内置的subprocess
模块来调用外部命令行程序。这个模块提供了强大的功能来创建和管理子进程,您可以使用subprocess.run()
、subprocess.call()
等函数来执行命令,并可以捕获输出和错误信息。例如,您可以使用以下代码来执行一个简单的命令:
import subprocess
result = subprocess.run(['ls', '-l'], capture_output=True, text=True)
print(result.stdout)
这段代码会在Linux或macOS系统中列出当前目录的文件。
调用命令行程序时如何处理输出和错误?
当您使用subprocess
模块时,可以通过设置capture_output=True
来捕获标准输出和标准错误。这样,您可以在代码中检查命令的执行结果。例如,如果命令失败,您可以通过result.stderr
获取错误信息,做出相应的处理。这是确保程序稳定性和调试的重要步骤。
是否可以在Python中传递参数给命令行程序?
当然可以!在使用subprocess.run()
时,您可以将参数作为列表传递。例如,如果您想要执行grep
命令并查找特定字符串,可以这样写:
import subprocess
search_string = 'example'
result = subprocess.run(['grep', search_string, 'file.txt'], capture_output=True, text=True)
print(result.stdout)
这样,您就可以灵活地传递不同的参数,以满足程序的需求。
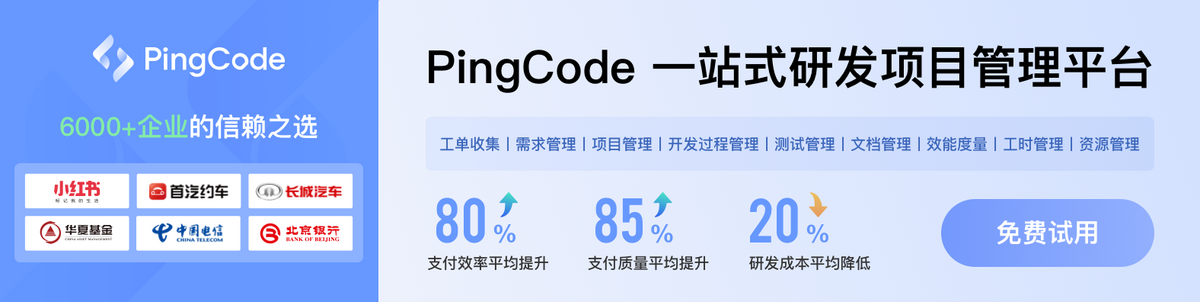