在Python中,函数可以通过多种方式返回两个值:使用元组、列表、字典或类。函数返回多个值的能力使得代码更加简洁和灵活,尤其是在需要返回多个相关数据时。其中,使用元组是最常见和最简单的方法。以下将详细描述如何通过不同方式在Python中实现函数返回两个值。
一、使用元组
使用元组是最常见的方式。元组是不可变的数据类型,可以轻松返回多个值。
def get_coordinates():
x = 5
y = 10
return x, y
coordinates = get_coordinates()
print(coordinates) # 输出: (5, 10)
x, y = coordinates
print(f"x: {x}, y: {y}") # 输出: x: 5, y: 10
通过上述代码示例,可以看到元组可以轻松返回多个值,并且可以使用解包语法来分别获取这些值。
二、使用列表
列表是一种可变的数据类型,也可以用来返回多个值。
def get_coordinates():
x = 5
y = 10
return [x, y]
coordinates = get_coordinates()
print(coordinates) # 输出: [5, 10]
x, y = coordinates
print(f"x: {x}, y: {y}") # 输出: x: 5, y: 10
使用列表可以方便地操作返回值,因为列表是可变的,所以可以随时修改。
三、使用字典
字典通过键值对的方式来存储数据,可以返回带有标签的多个值,增加可读性。
def get_coordinates():
return {"x": 5, "y": 10}
coordinates = get_coordinates()
print(coordinates) # 输出: {'x': 5, 'y': 10}
x = coordinates["x"]
y = coordinates["y"]
print(f"x: {x}, y: {y}") # 输出: x: 5, y: 10
使用字典可以让返回值更加具有描述性,便于理解和使用。
四、使用类
定义一个类并返回其实例,可以更好地组织和管理返回值,特别是在需要返回多个复杂值时。
class Coordinates:
def __init__(self, x, y):
self.x = x
self.y = y
def get_coordinates():
return Coordinates(5, 10)
coordinates = get_coordinates()
print(f"x: {coordinates.x}, y: {coordinates.y}") # 输出: x: 5, y: 10
使用类可以将多个相关值和方法封装在一起,提高代码的可读性和可维护性。
五、使用命名元组
命名元组是collections模块中的一种数据类型,结合了元组和字典的优点。
from collections import namedtuple
Coordinates = namedtuple('Coordinates', ['x', 'y'])
def get_coordinates():
return Coordinates(5, 10)
coordinates = get_coordinates()
print(coordinates) # 输出: Coordinates(x=5, y=10)
print(f"x: {coordinates.x}, y: {coordinates.y}") # 输出: x: 5, y: 10
命名元组使得返回值既可以像元组一样高效,又具有字典的可读性。
六、使用数据类(Data Classes)
数据类是Python 3.7引入的一个特性,用于简化类的定义,特别适用于存储数据。
from dataclasses import dataclass
@dataclass
class Coordinates:
x: int
y: int
def get_coordinates():
return Coordinates(5, 10)
coordinates = get_coordinates()
print(coordinates) # 输出: Coordinates(x=5, y=10)
print(f"x: {coordinates.x}, y: {coordinates.y}") # 输出: x: 5, y: 10
数据类提供了一个简洁的语法来定义类,并自动生成常用方法,如__init__和__repr__。
七、使用生成器
生成器是一种迭代器,可以逐个返回值。虽然不常用于返回多个值,但在某些情况下是有用的。
def get_coordinates():
yield 5
yield 10
coordinates = list(get_coordinates())
print(coordinates) # 输出: [5, 10]
x, y = coordinates
print(f"x: {x}, y: {y}") # 输出: x: 5, y: 10
生成器可以通过惰性求值逐个返回值,适合处理大量数据或流式数据。
八、使用自定义返回对象
在需要更多控制和扩展时,可以定义一个自定义的返回对象。
class ReturnObject:
def __init__(self, x, y):
self.x = x
self.y = y
def get_coordinates():
return ReturnObject(5, 10)
coordinates = get_coordinates()
print(f"x: {coordinates.x}, y: {coordinates.y}") # 输出: x: 5, y: 10
自定义返回对象可以根据需要添加更多属性和方法,增强代码的灵活性。
总结
在Python中,函数可以通过多种方式返回两个值,包括元组、列表、字典、类、命名元组、数据类、生成器和自定义返回对象。使用元组是最常见和最简单的方式,但其他方法也有各自的优势,可以根据具体需求选择合适的方法。通过这些方式,Python函数可以高效地返回多个值,使代码更加简洁和易于维护。
相关问答FAQs:
如何在Python中定义一个返回多个值的函数?
在Python中,可以通过使用逗号分隔的方式来定义一个返回多个值的函数。例如,您可以在函数中使用return
语句返回多个值,像这样:return value1, value2
。调用该函数时,您可以将返回的值赋给多个变量,类似于a, b = my_function()
。
返回的多个值在调用时如何处理?
当函数返回多个值时,您可以选择将它们存储在单独的变量中,也可以将它们存储在一个元组中。例如,您可以用result = my_function()
来获取返回值的元组,然后通过索引访问特定的值,如result[0]
。
在Python中返回多个值的优点是什么?
返回多个值的函数使得代码更加简洁和易于维护。它可以减少函数的数量,避免在多个函数之间传递参数的复杂性。此外,这种方式可以提高代码的可读性,因为调用者可以清楚地看到函数返回的所有相关数据。
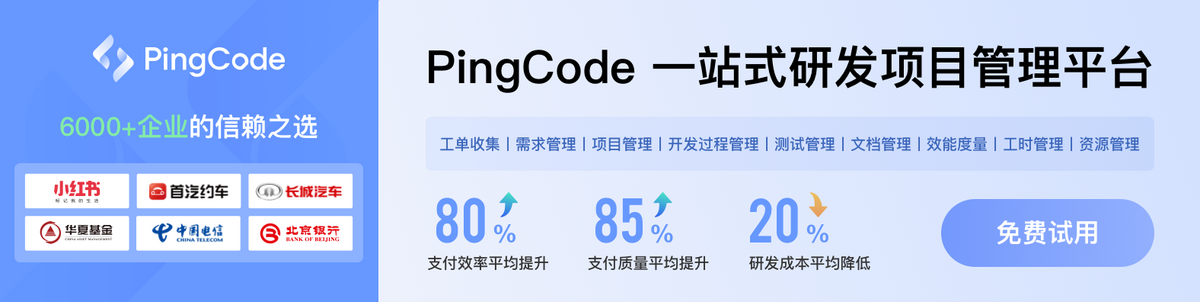