一、回答标题所提问题
用Python编写程序将温度转换的方法包括:使用基本的输入输出函数、定义转换函数、使用条件语句处理不同温度单位之间的转换、添加错误处理机制。使用基本的输入输出函数是最基础的方法,通过Python内置的input()
和print()
函数,可以实现简单的用户交互和结果输出。
使用基本的输入输出函数:这是最基础的方法,通过Python内置的input()
和print()
函数,可以实现简单的用户交互和结果输出。例如,可以通过input()
函数让用户输入温度值和单位,然后通过定义转换函数进行计算,最后使用print()
函数输出结果。这种方法简单易行,适合初学者。
定义转换函数:为了实现不同温度单位之间的转换,可以定义几个专门的转换函数。例如,可以定义celsius_to_fahrenheit()
函数将摄氏温度转换为华氏温度,fahrenheit_to_celsius()
函数将华氏温度转换为摄氏温度,celsius_to_kelvin()
函数将摄氏温度转换为开尔文温度等等。通过这些函数,可以更加模块化和清晰地进行温度转换。
使用条件语句处理不同温度单位之间的转换:在温度转换程序中,用户可能会输入不同的温度单位(例如摄氏温度、华氏温度、开尔文温度等)。为了处理这些不同单位之间的转换,可以使用条件语句(如if
、elif
、else
)进行判断和处理。例如,用户输入摄氏温度时,可以调用celsius_to_fahrenheit()
函数进行转换;用户输入华氏温度时,可以调用fahrenheit_to_celsius()
函数进行转换。
添加错误处理机制:在温度转换程序中,用户输入可能会出现错误。例如,用户可能会输入非数字字符或无效的温度单位。为了处理这些错误,可以添加错误处理机制。例如,可以使用try
和except
语句捕获用户输入的异常,提示用户重新输入有效的温度值和单位。
以下是详细的Python编写程序将温度转换的实现方法:
一、使用基本的输入输出函数
使用Python编写温度转换程序的最简单方法是通过input()
函数获取用户输入,通过print()
函数输出转换结果。以下是一个基本的示例:
# 获取用户输入的温度值和单位
temperature = float(input("请输入温度值: "))
unit = input("请输入温度单位 (C/F/K): ")
根据单位进行转换
if unit.upper() == 'C':
fahrenheit = (temperature * 9/5) + 32
kelvin = temperature + 273.15
print(f"摄氏温度: {temperature}°C")
print(f"华氏温度: {fahrenheit}°F")
print(f"开尔文温度: {kelvin}K")
elif unit.upper() == 'F':
celsius = (temperature - 32) * 5/9
kelvin = celsius + 273.15
print(f"华氏温度: {temperature}°F")
print(f"摄氏温度: {celsius}°C")
print(f"开尔文温度: {kelvin}K")
elif unit.upper() == 'K':
celsius = temperature - 273.15
fahrenheit = (celsius * 9/5) + 32
print(f"开尔文温度: {temperature}K")
print(f"摄氏温度: {celsius}°C")
print(f"华氏温度: {fahrenheit}°F")
else:
print("无效的温度单位,请输入C、F或K")
二、定义转换函数
为了使代码更加模块化和清晰,可以定义几个专门的转换函数。以下是定义转换函数的示例:
# 定义转换函数
def celsius_to_fahrenheit(celsius):
return (celsius * 9/5) + 32
def fahrenheit_to_celsius(fahrenheit):
return (fahrenheit - 32) * 5/9
def celsius_to_kelvin(celsius):
return celsius + 273.15
def kelvin_to_celsius(kelvin):
return kelvin - 273.15
获取用户输入的温度值和单位
temperature = float(input("请输入温度值: "))
unit = input("请输入温度单位 (C/F/K): ")
根据单位进行转换
if unit.upper() == 'C':
fahrenheit = celsius_to_fahrenheit(temperature)
kelvin = celsius_to_kelvin(temperature)
print(f"摄氏温度: {temperature}°C")
print(f"华氏温度: {fahrenheit}°F")
print(f"开尔文温度: {kelvin}K")
elif unit.upper() == 'F':
celsius = fahrenheit_to_celsius(temperature)
kelvin = celsius_to_kelvin(celsius)
print(f"华氏温度: {temperature}°F")
print(f"摄氏温度: {celsius}°C")
print(f"开尔文温度: {kelvin}K")
elif unit.upper() == 'K':
celsius = kelvin_to_celsius(temperature)
fahrenheit = celsius_to_fahrenheit(celsius)
print(f"开尔文温度: {temperature}K")
print(f"摄氏温度: {celsius}°C")
print(f"华氏温度: {fahrenheit}°F")
else:
print("无效的温度单位,请输入C、F或K")
三、使用条件语句处理不同温度单位之间的转换
在温度转换程序中,用户可能会输入不同的温度单位。为了处理这些不同单位之间的转换,可以使用条件语句进行判断和处理。以下是一个示例:
# 定义转换函数
def celsius_to_fahrenheit(celsius):
return (celsius * 9/5) + 32
def fahrenheit_to_celsius(fahrenheit):
return (fahrenheit - 32) * 5/9
def celsius_to_kelvin(celsius):
return celsius + 273.15
def kelvin_to_celsius(kelvin):
return kelvin - 273.15
获取用户输入的温度值和单位
temperature = float(input("请输入温度值: "))
unit = input("请输入温度单位 (C/F/K): ")
根据单位进行转换
if unit.upper() == 'C':
fahrenheit = celsius_to_fahrenheit(temperature)
kelvin = celsius_to_kelvin(temperature)
print(f"摄氏温度: {temperature}°C")
print(f"华氏温度: {fahrenheit}°F")
print(f"开尔文温度: {kelvin}K")
elif unit.upper() == 'F':
celsius = fahrenheit_to_celsius(temperature)
kelvin = celsius_to_kelvin(celsius)
print(f"华氏温度: {temperature}°F")
print(f"摄氏温度: {celsius}°C")
print(f"开尔文温度: {kelvin}K")
elif unit.upper() == 'K':
celsius = kelvin_to_celsius(temperature)
fahrenheit = celsius_to_fahrenheit(celsius)
print(f"开尔文温度: {temperature}K")
print(f"摄氏温度: {celsius}°C")
print(f"华氏温度: {fahrenheit}°F")
else:
print("无效的温度单位,请输入C、F或K")
四、添加错误处理机制
为了处理用户输入的错误,可以添加错误处理机制。例如,可以使用try
和except
语句捕获用户输入的异常,提示用户重新输入有效的温度值和单位。以下是一个示例:
# 定义转换函数
def celsius_to_fahrenheit(celsius):
return (celsius * 9/5) + 32
def fahrenheit_to_celsius(fahrenheit):
return (fahrenheit - 32) * 5/9
def celsius_to_kelvin(celsius):
return celsius + 273.15
def kelvin_to_celsius(kelvin):
return kelvin - 273.15
while True:
try:
# 获取用户输入的温度值和单位
temperature = float(input("请输入温度值: "))
unit = input("请输入温度单位 (C/F/K): ")
# 根据单位进行转换
if unit.upper() == 'C':
fahrenheit = celsius_to_fahrenheit(temperature)
kelvin = celsius_to_kelvin(temperature)
print(f"摄氏温度: {temperature}°C")
print(f"华氏温度: {fahrenheit}°F")
print(f"开尔文温度: {kelvin}K")
elif unit.upper() == 'F':
celsius = fahrenheit_to_celsius(temperature)
kelvin = celsius_to_kelvin(celsius)
print(f"华氏温度: {temperature}°F")
print(f"摄氏温度: {celsius}°C")
print(f"开尔文温度: {kelvin}K")
elif unit.upper() == 'K':
celsius = kelvin_to_celsius(temperature)
fahrenheit = celsius_to_fahrenheit(celsius)
print(f"开尔文温度: {temperature}K")
print(f"摄氏温度: {celsius}°C")
print(f"华氏温度: {fahrenheit}°F")
else:
print("无效的温度单位,请输入C、F或K")
except ValueError:
print("无效的温度值,请输入一个有效的数字")
通过上述方法,可以实现一个完整的温度转换程序,能够处理不同温度单位之间的转换,并具有基本的错误处理机制。希望这些示例能够帮助你更好地理解如何用Python编写温度转换程序。
相关问答FAQs:
如何使用Python将华氏温度转换为摄氏温度?
华氏温度转换为摄氏温度可以通过公式进行:摄氏温度 = (华氏温度 – 32) × 5/9。您可以使用Python编写一个简单的函数来实现这一转换。以下是一个示例代码:
def fahrenheit_to_celsius(fahrenheit):
return (fahrenheit - 32) * 5/9
# 示例
temp_f = 100
temp_c = fahrenheit_to_celsius(temp_f)
print(f"{temp_f} 华氏度 = {temp_c:.2f} 摄氏度")
这个程序会将输入的华氏温度转换并打印出对应的摄氏温度。
在Python中如何将摄氏温度转换为开尔文温度?
摄氏温度转换为开尔文温度的公式是:开尔文温度 = 摄氏温度 + 273.15。您可以编写如下的Python函数:
def celsius_to_kelvin(celsius):
return celsius + 273.15
# 示例
temp_c = 25
temp_k = celsius_to_kelvin(temp_c)
print(f"{temp_c} 摄氏度 = {temp_k:.2f} 开尔文")
这个函数将摄氏温度转换为开尔文温度并输出结果。
如何在Python中处理温度转换的用户输入?
要处理用户输入的温度转换,可以使用input()
函数来获取用户的输入,并使用条件语句来确定转换方向。例如:
def convert_temperature():
temp = float(input("请输入温度值:"))
scale = input("请输入温度单位(C 表示摄氏度,F 表示华氏度):").upper()
if scale == 'C':
print(f"{temp} 摄氏度 = {celsius_to_kelvin(temp):.2f} 开尔文")
print(f"{temp} 摄氏度 = {temp * 9/5 + 32:.2f} 华氏度")
elif scale == 'F':
print(f"{temp} 华氏度 = {fahrenheit_to_celsius(temp):.2f} 摄氏度")
else:
print("无效的温度单位。请使用 C 或 F。")
convert_temperature()
这个程序将根据用户输入的温度值和单位进行相应的转换并输出结果。
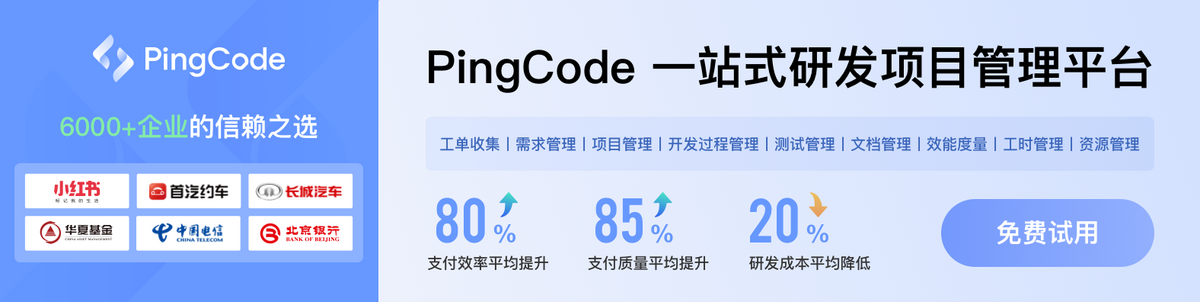