如何给自己的Python程序添加激活码
通过生成唯一激活码、使用加密技术保护激活码、设计用户友好的激活流程、定期更新和维护激活系统、提供客户支持、确保激活码的安全性、使用第三方工具和库来简化激活过程等步骤,可以有效地给自己的Python程序添加激活码。其中,生成唯一激活码是关键步骤之一,确保每个用户获得的激活码都是唯一的,可以防止激活码的重复使用和滥用。
生成唯一激活码的详细描述: 要生成唯一的激活码,可以使用Python中的UUID库,UUID(Universally Unique Identifier)是一种生成唯一标识符的标准方法。UUID库生成的激活码具有全局唯一性,能够确保每个用户获得的激活码都是独一无二的。下面是一个生成UUID激活码的示例代码:
import uuid
def generate_activation_code():
return str(uuid.uuid4())
activation_code = generate_activation_code()
print(f"Your activation code: {activation_code}")
通过上述代码,每次调用generate_activation_code函数时,都会生成一个新的唯一激活码,用户可以使用该激活码来激活程序。
一、生成唯一激活码
生成唯一激活码是给Python程序添加激活码的第一步。唯一激活码确保每个用户获得的激活码都是独一无二的,从而防止激活码的重复使用和滥用。可以使用Python中的UUID库来生成唯一激活码,以下是生成UUID激活码的示例代码:
import uuid
def generate_activation_code():
return str(uuid.uuid4())
activation_code = generate_activation_code()
print(f"Your activation code: {activation_code}")
UUID(Universally Unique Identifier)是一种生成唯一标识符的标准方法。UUID库生成的激活码具有全局唯一性,能够确保每个用户获得的激活码都是独一无二的。通过上述代码,每次调用generate_activation_code函数时,都会生成一个新的唯一激活码,用户可以使用该激活码来激活程序。
二、使用加密技术保护激活码
在生成唯一激活码后,需要确保激活码的安全性。可以使用加密技术来保护激活码,防止激活码被破解或篡改。常见的加密技术包括对称加密和非对称加密。
对称加密: 对称加密使用相同的密钥进行加密和解密。Python中可以使用Cryptography库来实现对称加密。以下是使用对称加密保护激活码的示例代码:
from cryptography.fernet import Fernet
生成密钥
key = Fernet.generate_key()
cipher_suite = Fernet(key)
加密激活码
activation_code = generate_activation_code()
encrypted_code = cipher_suite.encrypt(activation_code.encode())
解密激活码
decrypted_code = cipher_suite.decrypt(encrypted_code).decode()
print(f"Original code: {activation_code}")
print(f"Encrypted code: {encrypted_code}")
print(f"Decrypted code: {decrypted_code}")
非对称加密: 非对称加密使用一对密钥(公钥和私钥)进行加密和解密。Python中可以使用RSA算法来实现非对称加密。以下是使用非对称加密保护激活码的示例代码:
from cryptography.hazmat.primitives.asymmetric import rsa
from cryptography.hazmat.primitives.asymmetric import padding
from cryptography.hazmat.primitives import hashes
from cryptography.hazmat.primitives import serialization
生成密钥对
private_key = rsa.generate_private_key(
public_exponent=65537,
key_size=2048
)
public_key = private_key.public_key()
加密激活码
activation_code = generate_activation_code()
encrypted_code = public_key.encrypt(
activation_code.encode(),
padding.OAEP(
mgf=padding.MGF1(algorithm=hashes.SHA256()),
algorithm=hashes.SHA256(),
label=None
)
)
解密激活码
decrypted_code = private_key.decrypt(
encrypted_code,
padding.OAEP(
mgf=padding.MGF1(algorithm=hashes.SHA256()),
algorithm=hashes.SHA256(),
label=None
)
).decode()
print(f"Original code: {activation_code}")
print(f"Encrypted code: {encrypted_code}")
print(f"Decrypted code: {decrypted_code}")
三、设计用户友好的激活流程
为了让用户能够方便地激活程序,需要设计一个用户友好的激活流程。激活流程应该简单明了,用户只需输入激活码即可完成激活。可以设计一个图形用户界面(GUI)或命令行界面(CLI)来提示用户输入激活码。
图形用户界面(GUI): 使用Python的Tkinter库可以轻松创建一个简单的图形用户界面,提示用户输入激活码。以下是一个简单的激活界面的示例代码:
import tkinter as tk
from tkinter import messagebox
def activate():
input_code = activation_code_entry.get()
if input_code == activation_code:
messagebox.showinfo("Activation", "Activation successful!")
else:
messagebox.showerror("Activation", "Invalid activation code.")
生成激活码
activation_code = generate_activation_code()
创建GUI
root = tk.Tk()
root.title("Activation")
tk.Label(root, text="Enter activation code:").pack()
activation_code_entry = tk.Entry(root)
activation_code_entry.pack()
tk.Button(root, text="Activate", command=activate).pack()
root.mainloop()
命令行界面(CLI): 如果不需要图形界面,可以使用命令行界面提示用户输入激活码。以下是一个简单的命令行界面的示例代码:
def activate():
input_code = input("Enter activation code: ")
if input_code == activation_code:
print("Activation successful!")
else:
print("Invalid activation code.")
生成激活码
activation_code = generate_activation_code()
激活流程
activate()
四、定期更新和维护激活系统
为了确保激活系统的安全性和有效性,需要定期更新和维护激活系统。可以定期生成新的激活码,并更新激活码的加密算法,以防止激活码被破解或滥用。此外,还可以监控激活码的使用情况,发现异常使用行为时及时采取措施。
定期生成新的激活码: 可以定期生成新的激活码,并将旧的激活码失效,以确保激活码的安全性。以下是定期生成新的激活码的示例代码:
import time
def generate_new_activation_code():
global activation_code
activation_code = generate_activation_code()
print(f"New activation code: {activation_code}")
定期生成新的激活码
while True:
generate_new_activation_code()
time.sleep(3600) # 每小时生成一个新的激活码
更新激活码的加密算法: 可以定期更新激活码的加密算法,以提高激活系统的安全性。例如,可以使用不同的加密算法或增加密钥的长度。以下是更新激活码加密算法的示例代码:
from cryptography.hazmat.primitives.kdf.pbkdf2 import PBKDF2HMAC
from cryptography.hazmat.primitives import hashes
from cryptography.hazmat.primitives.kdf.pbkdf2 import PBKDF2HMAC
使用PBKDF2HMAC生成密钥
password = b"my_secret_password"
salt = b"my_secret_salt"
kdf = PBKDF2HMAC(
algorithm=hashes.SHA256(),
length=32,
salt=salt,
iterations=100000,
)
key = base64.urlsafe_b64encode(kdf.derive(password))
加密激活码
cipher_suite = Fernet(key)
encrypted_code = cipher_suite.encrypt(activation_code.encode())
解密激活码
decrypted_code = cipher_suite.decrypt(encrypted_code).decode()
print(f"Original code: {activation_code}")
print(f"Encrypted code: {encrypted_code}")
print(f"Decrypted code: {decrypted_code}")
五、提供客户支持
在激活系统的使用过程中,用户可能会遇到各种问题,例如激活码失效、无法输入激活码等。为了提高用户体验,需要提供及时的客户支持,帮助用户解决问题。可以通过以下几种方式提供客户支持:
在线客服: 可以在程序的官方网站或用户界面中提供在线客服,用户可以通过在线聊天或提交工单的方式获得帮助。
电子邮件支持: 提供一个专用的电子邮件地址,用户可以通过发送电子邮件的方式获得支持。以下是一个简单的电子邮件支持示例代码:
import smtplib
from email.mime.text import MIMEText
def send_support_email(user_email, issue_description):
sender_email = "support@example.com"
sender_password = "your_password"
recipient_email = "support@example.com"
message = MIMEText(issue_description)
message["Subject"] = "Support Request"
message["From"] = sender_email
message["To"] = recipient_email
with smtplib.SMTP_SSL("smtp.example.com", 465) as server:
server.login(sender_email, sender_password)
server.sendmail(sender_email, recipient_email, message.as_string())
用户发送支持请求
user_email = "user@example.com"
issue_description = "I cannot activate my program with the provided activation code."
send_support_email(user_email, issue_description)
常见问题解答(FAQ): 在程序的官方网站或用户界面中提供常见问题解答,用户可以自行查找解决方案。以下是一个简单的FAQ示例:
faq = {
"Q: How do I activate the program?": "A: Enter the activation code in the provided field and click 'Activate'.",
"Q: What should I do if the activation code is invalid?": "A: Please check if the activation code is entered correctly. If the issue persists, contact support.",
"Q: Can I use the same activation code on multiple devices?": "A: No, each activation code is unique and can only be used on one device."
}
def display_faq():
for question, answer in faq.items():
print(question)
print(answer)
print()
显示FAQ
display_faq()
六、确保激活码的安全性
为了确保激活码的安全性,需要采取一系列措施防止激活码被破解或滥用。以下是一些确保激活码安全性的建议:
使用强加密算法: 使用强加密算法保护激活码,例如AES、RSA等。确保加密算法和密钥的安全性,定期更新加密算法和密钥。
限制激活码的使用次数: 限制激活码的使用次数,例如每个激活码只能使用一次或在一定时间内只能使用一定次数。以下是限制激活码使用次数的示例代码:
class ActivationCodeManager:
def __init__(self):
self.activation_codes = {}
def generate_activation_code(self):
code = generate_activation_code()
self.activation_codes[code] = 0
return code
def activate(self, code):
if code in self.activation_codes:
if self.activation_codes[code] < 1:
self.activation_codes[code] += 1
return True
else:
return False
else:
return False
使用示例
manager = ActivationCodeManager()
activation_code = manager.generate_activation_code()
print(f"Generated code: {activation_code}")
激活程序
if manager.activate(activation_code):
print("Activation successful!")
else:
print("Invalid or already used activation code.")
监控激活码的使用情况: 监控激活码的使用情况,发现异常使用行为时及时采取措施。例如,可以记录每个激活码的使用时间、IP地址等信息,分析使用情况,发现异常使用行为时采取措施。以下是监控激活码使用情况的示例代码:
import datetime
class ActivationCodeManager:
def __init__(self):
self.activation_codes = {}
def generate_activation_code(self):
code = generate_activation_code()
self.activation_codes[code] = {"used": False, "timestamp": None, "ip_address": None}
return code
def activate(self, code, ip_address):
if code in self.activation_codes:
if not self.activation_codes[code]["used"]:
self.activation_codes[code]["used"] = True
self.activation_codes[code]["timestamp"] = datetime.datetime.now()
self.activation_codes[code]["ip_address"] = ip_address
return True
else:
return False
else:
return False
def get_activation_info(self, code):
if code in self.activation_codes:
return self.activation_codes[code]
else:
return None
使用示例
manager = ActivationCodeManager()
activation_code = manager.generate_activation_code()
print(f"Generated code: {activation_code}")
激活程序
ip_address = "192.168.1.1"
if manager.activate(activation_code, ip_address):
print("Activation successful!")
else:
print("Invalid or already used activation code.")
获取激活信息
activation_info = manager.get_activation_info(activation_code)
print(f"Activation info: {activation_info}")
七、使用第三方工具和库来简化激活过程
为了简化激活过程,可以使用一些第三方工具和库。这些工具和库可以帮助生成激活码、加密激活码、管理激活码的使用情况等,节省开发时间和精力。
LicenseManager库: LicenseManager是一个Python库,用于生成和验证软件许可证。以下是使用LicenseManager库生成和验证激活码的示例代码:
from license_manager import LicenseManager
创建LicenseManager实例
manager = LicenseManager()
生成激活码
activation_code = manager.generate_license()
print(f"Generated code: {activation_code}")
验证激活码
if manager.validate_license(activation_code):
print("Activation successful!")
else:
print("Invalid activation code.")
PyCryptodome库: PyCryptodome是一个Python库,用于实现各种加密算法。可以使用PyCryptodome库对激活码进行加密和解密。以下是使用PyCryptodome库加密和解密激活码的示例代码:
from Crypto.Cipher import AES
from Crypto.Random import get_random_bytes
import base64
生成密钥
key = get_random_bytes(16)
cipher = AES.new(key, AES.MODE_EAX)
加密激活码
activation_code = generate_activation_code()
nonce = cipher.nonce
ciphertext, tag = cipher.encrypt_and_digest(activation_code.encode())
解密激活码
cipher = AES.new(key, AES.MODE_EAX, nonce=nonce)
decrypted_code = cipher.decrypt(ciphertext).decode()
print(f"Original code: {activation_code}")
print(f"Encrypted code: {base64.b64encode(ciphertext).decode()}")
print(f"Decrypted code: {decrypted_code}")
使用第三方激活服务: 除了使用Python库,还可以使用一些第三方激活服务,例如Paddle、FastSpring等。这些服务提供完整的激活管理解决方案,包括激活码生成、验证、使用监控等,可以帮助简化激活过程。
总之,通过生成唯一激活码、使用加密技术保护激活码、设计用户友好的激活流程、定期更新和维护激活系统、提供客户支持、确保激活码的安全性、使用第三方工具和库来简化激活过程,可以有效地给自己的Python程序添加激活码,提高程序的安全性和用户体验。
相关问答FAQs:
如何获取Python的激活码?
Python本身是一个开源的编程语言,并不需要激活码。用户可以直接从Python官方网站下载并安装最新版本。所有功能都是免费的,用户只需遵循相应的安装指南即可开始使用。
在安装Python时需要注意哪些事项?
安装Python时,确保选择合适的版本(如Python 3.x),并根据操作系统选择相应的安装包。此外,建议在安装过程中勾选“将Python添加到环境变量”选项,这样可以在命令行中方便地使用Python命令。
如何解决Python安装后无法使用的问题?
如果安装后无法使用Python,首先检查是否已正确设置环境变量。可以在命令行中输入“python”来测试。如果提示“不是内部或外部命令”,则需要手动添加Python的安装路径到系统环境变量中。确保重启命令行窗口以使更改生效。如果问题仍然存在,可以尝试重新安装Python,并确保选择“修复”选项。
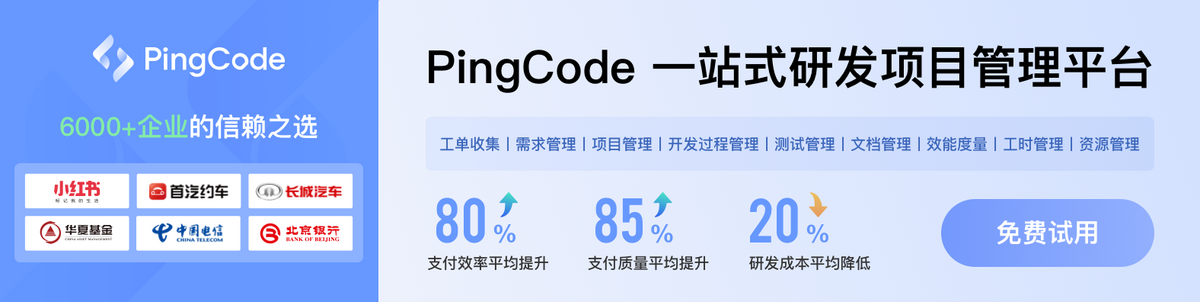