Python3 如何制作放置类游戏
制作放置类游戏的关键要点包括:选择合适的游戏框架、设计游戏机制、实现资源管理、创建用户界面、添加游戏逻辑、数据持久化和测试。选择合适的游戏框架、设计游戏机制、实现资源管理、创建用户界面、添加游戏逻辑、数据持久化和测试。以下将详细讨论其中一个关键要点——设计游戏机制,并且逐步介绍制作放置类游戏的其他方面。
一、选择合适的游戏框架
Python 中有许多适合制作游戏的框架和库,最常用的是 Pygame 和 Arcade。Pygame 是一个功能强大且易于学习的库,适合初学者和小型项目。Arcade 是一个更现代的选择,提供了更高级的功能和更简洁的代码结构。选择框架时需要考虑项目的规模、所需功能和个人偏好。
二、设计游戏机制
设计游戏机制是制作放置类游戏的核心。放置类游戏通常包括资源生产、资源管理、升级和自动化等元素。
1. 资源生产
资源生产是放置类游戏的基础。通常,资源生产是基于时间的,每秒或每分钟产生一定数量的资源。可以使用 Python 的时间模块来实现这一功能。
import time
class ResourceProducer:
def __init__(self, production_rate):
self.production_rate = production_rate
self.last_update_time = time.time()
self.resources = 0
def update_resources(self):
current_time = time.time()
time_passed = current_time - self.last_update_time
self.resources += self.production_rate * time_passed
self.last_update_time = current_time
def get_resources(self):
self.update_resources()
return self.resources
producer = ResourceProducer(1) # 每秒生产1个资源
while True:
time.sleep(1)
print(f"资源数量: {producer.get_resources()}")
2. 资源管理
资源管理包括资源的收集、存储和使用。玩家可以通过点击或其他操作来收集资源,资源可以存储在玩家的库存中,并用于购买升级或其他物品。
class Inventory:
def __init__(self):
self.resources = 0
def add_resources(self, amount):
self.resources += amount
def use_resources(self, amount):
if self.resources >= amount:
self.resources -= amount
return True
return False
inventory = Inventory()
inventory.add_resources(producer.get_resources())
print(f"库存中的资源: {inventory.resources}")
3. 升级和自动化
升级和自动化是放置类游戏的重要元素。玩家可以通过花费资源来升级生产设备,提高资源生产率或解锁自动化功能。
class Upgrade:
def __init__(self, cost, production_increase):
self.cost = cost
self.production_increase = production_increase
def apply_upgrade(self, producer, inventory):
if inventory.use_resources(self.cost):
producer.production_rate += self.production_increase
return True
return False
upgrade = Upgrade(10, 0.5) # 花费10个资源,增加0.5的生产率
if upgrade.apply_upgrade(producer, inventory):
print("升级成功")
else:
print("资源不足,无法升级")
三、实现资源管理
资源管理是放置类游戏的核心功能之一,玩家需要通过管理资源来实现游戏中的各种目标。资源管理包括资源的收集、存储和使用。
1. 资源收集
资源收集可以通过点击或自动生成的方式进行。点击收集是指玩家通过点击某个按钮来收集资源,而自动生成则是指资源根据时间自动增加。
class ClickCollector:
def __init__(self, inventory):
self.inventory = inventory
def collect_resources(self, amount):
self.inventory.add_resources(amount)
click_collector = ClickCollector(inventory)
click_collector.collect_resources(5)
print(f"点击收集后的资源: {inventory.resources}")
2. 资源存储
资源存储通常是通过库存来实现的,库存可以存储玩家收集到的资源,并在需要时进行使用。
class Storage:
def __init__(self, capacity):
self.capacity = capacity
self.resources = 0
def add_resources(self, amount):
if self.resources + amount <= self.capacity:
self.resources += amount
return True
return False
def use_resources(self, amount):
if self.resources >= amount:
self.resources -= amount
return True
return False
storage = Storage(100)
if storage.add_resources(inventory.resources):
print(f"资源存储成功,当前存储: {storage.resources}")
else:
print("存储空间不足")
3. 资源使用
资源使用包括购买升级、解锁新功能和完成任务等。玩家可以通过使用资源来提升游戏体验和进展。
class Task:
def __init__(self, cost):
self.cost = cost
def complete_task(self, storage):
if storage.use_resources(self.cost):
print("任务完成")
else:
print("资源不足,无法完成任务")
task = Task(20)
task.complete_task(storage)
四、创建用户界面
用户界面是玩家与游戏交互的重要部分。一个好的用户界面可以提高玩家的游戏体验,使游戏更加直观和易于操作。在 Python 中,可以使用 Tkinter、Pygame 或 Arcade 等库来创建用户界面。
1. 使用 Tkinter 创建简单用户界面
Tkinter 是 Python 自带的 GUI 库,非常适合创建简单的用户界面。
import tkinter as tk
class GameUI:
def __init__(self, root, click_collector):
self.root = root
self.click_collector = click_collector
self.label = tk.Label(root, text="资源: 0")
self.label.pack()
self.button = tk.Button(root, text="收集资源", command=self.collect_resources)
self.button.pack()
def collect_resources(self):
self.click_collector.collect_resources(1)
self.label.config(text=f"资源: {inventory.resources}")
root = tk.Tk()
ui = GameUI(root, click_collector)
root.mainloop()
2. 使用 Pygame 创建用户界面
Pygame 是一个功能强大的游戏开发库,适合创建复杂的用户界面和游戏逻辑。
import pygame
pygame.init()
screen = pygame.display.set_mode((800, 600))
font = pygame.font.Font(None, 36)
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.MOUSEBUTTONDOWN:
click_collector.collect_resources(1)
screen.fill((0, 0, 0))
text = font.render(f"资源: {inventory.resources}", True, (255, 255, 255))
screen.blit(text, (10, 10))
pygame.display.flip()
pygame.quit()
五、添加游戏逻辑
游戏逻辑是放置类游戏的核心,包括资源生产、资源管理、升级和自动化等功能。
1. 资源生产逻辑
资源生产逻辑通常是基于时间的,每秒或每分钟产生一定数量的资源。
def update_resources(producer):
producer.update_resources()
while True:
time.sleep(1)
update_resources(producer)
print(f"资源数量: {producer.get_resources()}")
2. 升级和自动化逻辑
升级和自动化是放置类游戏的重要元素,玩家可以通过花费资源来升级生产设备,提高资源生产率或解锁自动化功能。
def apply_upgrade(upgrade, producer, inventory):
if upgrade.apply_upgrade(producer, inventory):
print("升级成功")
else:
print("资源不足,无法升级")
upgrade = Upgrade(10, 0.5)
apply_upgrade(upgrade, producer, inventory)
六、数据持久化
数据持久化是指将游戏数据保存到文件或数据库中,以便在游戏重新启动时恢复游戏进度。Python 提供了多种数据持久化的方法,如 JSON、SQLite 和 Pickle 等。
1. 使用 JSON 保存和加载数据
JSON 是一种轻量级的数据交换格式,非常适合保存游戏数据。
import json
def save_game_data(filename, data):
with open(filename, 'w') as f:
json.dump(data, f)
def load_game_data(filename):
with open(filename, 'r') as f:
return json.load(f)
game_data = {
'resources': inventory.resources,
'production_rate': producer.production_rate
}
save_game_data('game_data.json', game_data)
loaded_data = load_game_data('game_data.json')
inventory.resources = loaded_data['resources']
producer.production_rate = loaded_data['production_rate']
2. 使用 SQLite 保存和加载数据
SQLite 是一个轻量级的关系型数据库管理系统,非常适合保存结构化数据。
import sqlite3
def create_database():
conn = sqlite3.connect('game_data.db')
cursor = conn.cursor()
cursor.execute('''CREATE TABLE IF NOT EXISTS game_data
(id INTEGER PRIMARY KEY, resources INTEGER, production_rate REAL)''')
conn.commit()
conn.close()
def save_game_data(resources, production_rate):
conn = sqlite3.connect('game_data.db')
cursor = conn.cursor()
cursor.execute('''INSERT INTO game_data (resources, production_rate)
VALUES (?, ?)''', (resources, production_rate))
conn.commit()
conn.close()
def load_game_data():
conn = sqlite3.connect('game_data.db')
cursor = conn.cursor()
cursor.execute('''SELECT resources, production_rate FROM game_data ORDER BY id DESC LIMIT 1''')
data = cursor.fetchone()
conn.close()
return data
create_database()
save_game_data(inventory.resources, producer.production_rate)
loaded_data = load_game_data()
if loaded_data:
inventory.resources, producer.production_rate = loaded_data
七、测试
测试是确保游戏正常运行的关键步骤。在开发过程中,应该不断进行测试,以发现并修复潜在的问题。
1. 单元测试
单元测试是对游戏中的各个功能进行独立测试,以确保它们能够正常工作。Python 提供了 unittest 模块,可以方便地进行单元测试。
import unittest
class TestGame(unittest.TestCase):
def test_resource_production(self):
producer = ResourceProducer(1)
time.sleep(1)
self.assertGreater(producer.get_resources(), 0)
def test_resource_collection(self):
inventory = Inventory()
click_collector = ClickCollector(inventory)
click_collector.collect_resources(5)
self.assertEqual(inventory.resources, 5)
def test_upgrade(self):
inventory = Inventory()
producer = ResourceProducer(1)
upgrade = Upgrade(10, 0.5)
inventory.add_resources(10)
upgrade.apply_upgrade(producer, inventory)
self.assertEqual(producer.production_rate, 1.5)
self.assertEqual(inventory.resources, 0)
if __name__ == '__main__':
unittest.main()
2. 集成测试
集成测试是对游戏的各个部分进行整体测试,以确保它们能够正常协同工作。
def test_game():
inventory = Inventory()
producer = ResourceProducer(1)
click_collector = ClickCollector(inventory)
upgrade = Upgrade(10, 0.5)
click_collector.collect_resources(5)
assert inventory.resources == 5
time.sleep(2)
inventory.add_resources(producer.get_resources())
assert inventory.resources > 5
if inventory.use_resources(10):
upgrade.apply_upgrade(producer, inventory)
assert producer.production_rate == 1.5
test_game()
结论
通过选择合适的游戏框架、设计游戏机制、实现资源管理、创建用户界面、添加游戏逻辑、数据持久化和测试,我们可以使用 Python3 制作一个放置类游戏。制作放置类游戏的过程需要不断进行设计、编码和测试,以确保游戏的功能和体验达到预期。希望这篇文章能为您提供一些有价值的参考和指导,祝您在制作放置类游戏的过程中取得成功。
相关问答FAQs:
如何开始使用Python3开发放置类游戏?
开发放置类游戏的第一步是选择合适的游戏引擎或框架。常用的有Pygame和Godot等。Pygame适合初学者,它提供了丰富的功能,可以帮助你快速实现游戏逻辑和图形显示。可以从简单的项目开始,逐步学习如何处理用户输入、游戏循环和资源管理。
放置类游戏的核心机制是什么?
放置类游戏通常依赖于自动生成资源的机制。玩家的目标是在不需要持续操作的情况下,通过购买升级和管理资源来提升效率。你可以设计一些基本的元素,例如生产单位、资源收集和升级系统,使玩家能够逐步提高游戏的进展。确保在游戏中引入增量反馈,让玩家感受到成就感。
如何设计放置类游戏的用户界面?
用户界面的设计对于放置类游戏至关重要。应该确保界面直观且易于操作。可以使用图形化工具来创建按钮、进度条和信息显示区域。设计时要考虑到玩家的交互习惯,确保游戏信息清晰可见,并且操作流畅。通过测试和反馈,不断优化界面布局和功能,使玩家能够轻松上手并享受游戏乐趣。
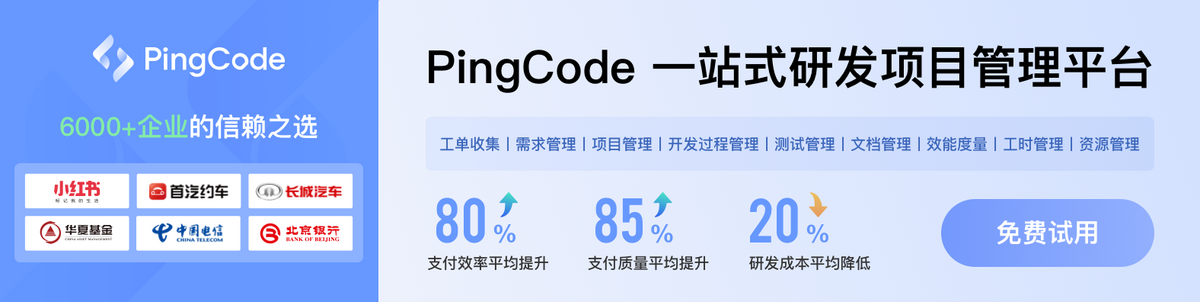