Python查询文件的绝对路径的方法包括使用os模块、使用pathlib模块、避免相对路径陷阱。其中,os模块是最常用的方法之一,接下来我们将详细描述如何使用os模块查询文件的绝对路径。
在Python中,查询文件的绝对路径是一项常见的任务,特别是在处理文件和目录时。绝对路径是从根目录开始的完整路径,而相对路径是从当前工作目录开始的路径。使用绝对路径可以避免由于当前工作目录的变化导致的路径错误。
一、使用os模块
os模块是Python标准库中的一个模块,提供了与操作系统进行交互的功能。使用os模块,可以轻松地查询文件的绝对路径。
import os
获取文件的绝对路径
file_name = 'example.txt'
absolute_path = os.path.abspath(file_name)
print(f"The absolute path of the file is: {absolute_path}")
在上面的代码中,我们使用了os.path.abspath
函数来获取文件的绝对路径。os.path.abspath
函数会将相对路径转换为绝对路径。
1、获取当前工作目录
在查询文件的绝对路径之前,有时需要知道当前工作目录。可以使用os.getcwd
函数来获取当前工作目录。
import os
获取当前工作目录
current_directory = os.getcwd()
print(f"The current working directory is: {current_directory}")
2、改变当前工作目录
有时需要临时改变当前工作目录,可以使用os.chdir
函数。
import os
改变当前工作目录
new_directory = '/path/to/new/directory'
os.chdir(new_directory)
print(f"The new working directory is: {os.getcwd()}")
二、使用pathlib模块
pathlib模块是Python 3.4引入的一个模块,提供了面向对象的路径操作方法。使用pathlib模块也可以查询文件的绝对路径。
from pathlib import Path
获取文件的绝对路径
file_name = 'example.txt'
absolute_path = Path(file_name).resolve()
print(f"The absolute path of the file is: {absolute_path}")
在上面的代码中,我们使用了Path
类和resolve
方法来获取文件的绝对路径。resolve
方法会将相对路径转换为绝对路径。
1、获取当前工作目录
pathlib模块中也可以获取当前工作目录,使用Path.cwd
方法。
from pathlib import Path
获取当前工作目录
current_directory = Path.cwd()
print(f"The current working directory is: {current_directory}")
2、改变当前工作目录
pathlib模块中可以使用os.chdir
函数来改变当前工作目录,因为pathlib模块没有直接提供改变当前工作目录的方法。
import os
from pathlib import Path
改变当前工作目录
new_directory = Path('/path/to/new/directory')
os.chdir(new_directory)
print(f"The new working directory is: {Path.cwd()}")
三、避免相对路径陷阱
在编写脚本时,如果不注意路径的使用,很容易掉入相对路径的陷阱。例如,在一个脚本中使用相对路径读取文件时,如果脚本的当前工作目录发生变化,就会导致文件无法找到的错误。为了避免这种情况,建议在脚本中尽量使用绝对路径。
1、使用__file__获取脚本所在目录
可以使用__file__
变量获取当前脚本所在的目录,然后基于这个目录构建绝对路径。
import os
获取脚本所在目录
script_directory = os.path.dirname(os.path.abspath(__file__))
file_name = 'example.txt'
absolute_path = os.path.join(script_directory, file_name)
print(f"The absolute path of the file is: {absolute_path}")
在上面的代码中,我们使用了__file__
变量获取当前脚本的路径,然后使用os.path.dirname
函数获取脚本所在的目录,最后使用os.path.join
函数构建文件的绝对路径。
2、使用pathlib模块获取脚本所在目录
同样,可以使用pathlib模块来获取脚本所在的目录,并基于这个目录构建绝对路径。
from pathlib import Path
获取脚本所在目录
script_directory = Path(__file__).parent
file_name = 'example.txt'
absolute_path = (script_directory / file_name).resolve()
print(f"The absolute path of the file is: {absolute_path}")
在上面的代码中,我们使用了Path
类和parent
属性获取脚本所在的目录,然后使用/
运算符和resolve
方法构建文件的绝对路径。
四、处理文件路径的其他常用方法
在处理文件路径时,除了查询绝对路径,还有一些常用的方法可以提高代码的可读性和健壮性。
1、检查文件或目录是否存在
在处理文件时,首先需要检查文件或目录是否存在。可以使用os.path.exists
函数或pathlib.Path.exists
方法。
import os
from pathlib import Path
使用os模块检查文件是否存在
file_name = 'example.txt'
if os.path.exists(file_name):
print(f"The file {file_name} exists.")
else:
print(f"The file {file_name} does not exist.")
使用pathlib模块检查文件是否存在
file_path = Path(file_name)
if file_path.exists():
print(f"The file {file_name} exists.")
else:
print(f"The file {file_name} does not exist.")
2、创建目录
在处理文件时,有时需要创建目录。可以使用os.makedirs
函数或pathlib.Path.mkdir
方法。
import os
from pathlib import Path
使用os模块创建目录
directory_name = 'new_directory'
if not os.path.exists(directory_name):
os.makedirs(directory_name)
print(f"The directory {directory_name} has been created.")
else:
print(f"The directory {directory_name} already exists.")
使用pathlib模块创建目录
directory_path = Path(directory_name)
if not directory_path.exists():
directory_path.mkdir(parents=True)
print(f"The directory {directory_name} has been created.")
else:
print(f"The directory {directory_name} already exists.")
3、删除文件或目录
在处理文件时,有时需要删除文件或目录。可以使用os.remove
函数删除文件,使用os.rmdir
或shutil.rmtree
函数删除目录。使用pathlib.Path.unlink
方法删除文件,使用pathlib.Path.rmdir
方法删除目录。
import os
import shutil
from pathlib import Path
使用os模块删除文件
file_name = 'example.txt'
if os.path.exists(file_name):
os.remove(file_name)
print(f"The file {file_name} has been deleted.")
else:
print(f"The file {file_name} does not exist.")
使用os模块删除目录
directory_name = 'new_directory'
if os.path.exists(directory_name):
shutil.rmtree(directory_name)
print(f"The directory {directory_name} has been deleted.")
else:
print(f"The directory {directory_name} does not exist.")
使用pathlib模块删除文件
file_path = Path(file_name)
if file_path.exists():
file_path.unlink()
print(f"The file {file_name} has been deleted.")
else:
print(f"The file {file_name} does not exist.")
使用pathlib模块删除目录
directory_path = Path(directory_name)
if directory_path.exists():
directory_path.rmdir()
print(f"The directory {directory_name} has been deleted.")
else:
print(f"The directory {directory_name} does not exist.")
五、跨平台路径处理
在处理文件路径时,考虑到代码的跨平台兼容性非常重要。不同操作系统的路径表示方式不同,例如Windows使用反斜杠(\)作为路径分隔符,而Unix/Linux和MacOS使用正斜杠(/)作为路径分隔符。为了确保代码在不同平台上都能正常运行,建议使用os.path
模块或pathlib
模块进行路径处理。
1、使用os.path模块进行跨平台路径处理
os.path
模块提供了一些函数,可以处理跨平台路径问题。
import os
拼接路径
directory_name = 'new_directory'
file_name = 'example.txt'
file_path = os.path.join(directory_name, file_name)
print(f"The file path is: {file_path}")
获取路径的各个部分
path = '/path/to/directory/example.txt'
directory_name = os.path.dirname(path)
file_name = os.path.basename(path)
print(f"Directory name: {directory_name}")
print(f"File name: {file_name}")
获取路径的扩展名
file_extension = os.path.splitext(path)[1]
print(f"File extension: {file_extension}")
2、使用pathlib模块进行跨平台路径处理
pathlib
模块提供了更为简洁和直观的路径处理方法,推荐使用pathlib
模块进行跨平台路径处理。
from pathlib import Path
拼接路径
directory_name = Path('new_directory')
file_name = 'example.txt'
file_path = directory_name / file_name
print(f"The file path is: {file_path}")
获取路径的各个部分
path = Path('/path/to/directory/example.txt')
directory_name = path.parent
file_name = path.name
print(f"Directory name: {directory_name}")
print(f"File name: {file_name}")
获取路径的扩展名
file_extension = path.suffix
print(f"File extension: {file_extension}")
六、总结
在Python中,查询文件的绝对路径是一个常见而重要的任务。使用os
模块和pathlib
模块都可以轻松地查询文件的绝对路径,并进行路径处理。在编写代码时,建议尽量使用绝对路径,避免相对路径带来的问题。同时,考虑到代码的跨平台兼容性,推荐使用pathlib
模块进行路径处理。通过掌握这些方法和技巧,可以编写出更为健壮和可维护的代码。
相关问答FAQs:
如何在Python中获取文件的绝对路径?
在Python中,可以使用os
模块的abspath()
函数来获取文件的绝对路径。首先需要导入os
模块,然后调用os.path.abspath(file_name)
,其中file_name
是文件的相对路径或文件名。该函数将返回文件的完整绝对路径。
如果我只知道文件的相对路径,如何确认它的绝对路径?
可以使用os.path.abspath()
函数将相对路径转换为绝对路径。只需传递相对路径作为参数,该函数会返回当前工作目录下该文件的绝对路径。确保在运行该代码之前,文件确实存在于指定的相对路径中。
如何检查文件是否存在于指定的绝对路径?
可以使用os.path.exists(path)
来检查文件是否存在。传入文件的绝对路径作为参数,该函数将返回一个布尔值,指示文件是否存在。这在处理文件时非常有用,以避免因路径错误而导致的错误。
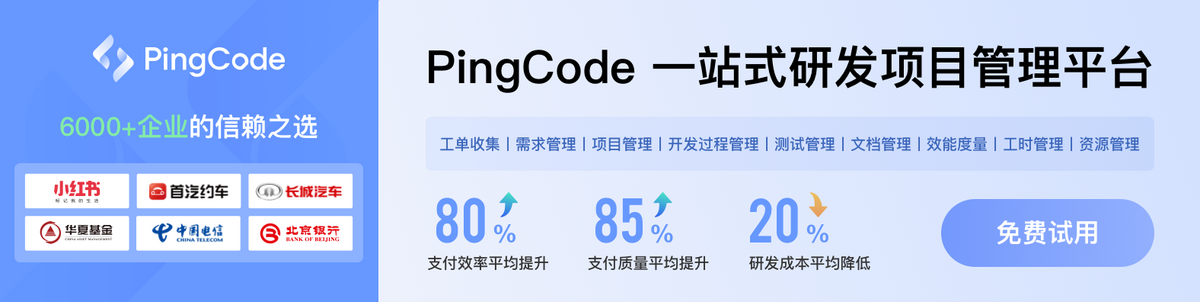