在Python中使用三角函数的方法有很多,主要通过math库、numpy库和scipy库来实现。math库提供了基本的三角函数,如sin、cos、tan等,numpy库提供了对数组操作的三角函数,scipy库则提供了更高级的三角函数和相关功能。 例如,math库中的math.sin()
函数可以用于计算一个角度的正弦值。
一、使用math库中的三角函数
math库是Python的内置库之一,提供了常用的数学函数,包括基本的三角函数。使用math库中的三角函数非常简单,首先需要导入math库:
import math
1.1 正弦函数(sin)
正弦函数可以用来计算一个角度的正弦值。角度是以弧度为单位的,1弧度等于180/π度。比如:
import math
angle = math.pi / 6 # 30度角
sin_value = math.sin(angle)
print(f"Sin(30 degrees) = {sin_value}")
1.2 余弦函数(cos)
余弦函数可以用来计算一个角度的余弦值。使用方法与正弦函数类似:
import math
angle = math.pi / 3 # 60度角
cos_value = math.cos(angle)
print(f"Cos(60 degrees) = {cos_value}")
1.3 正切函数(tan)
正切函数可以用来计算一个角度的正切值:
import math
angle = math.pi / 4 # 45度角
tan_value = math.tan(angle)
print(f"Tan(45 degrees) = {tan_value}")
1.4 反三角函数
math库还提供了反三角函数,用于从正弦、余弦、正切值反推出角度值。常用的反三角函数包括math.asin()
、math.acos()
和math.atan()
。
import math
sin_value = 0.5
angle = math.asin(sin_value)
print(f"Arcsin(0.5) = {angle} radians")
cos_value = 0.5
angle = math.acos(cos_value)
print(f"Arccos(0.5) = {angle} radians")
tan_value = 1
angle = math.atan(tan_value)
print(f"Arctan(1) = {angle} radians")
二、使用numpy库中的三角函数
numpy库提供了强大的数组操作功能,包括对数组元素进行三角函数计算。首先需要安装并导入numpy库:
import numpy as np
2.1 正弦函数(sin)
numpy的sin函数与math库类似,但可以对数组进行操作:
import numpy as np
angles = np.array([0, np.pi / 6, np.pi / 4, np.pi / 3, np.pi / 2])
sin_values = np.sin(angles)
print(f"Sin values: {sin_values}")
2.2 余弦函数(cos)
使用numpy计算数组的余弦值:
import numpy as np
angles = np.array([0, np.pi / 6, np.pi / 4, np.pi / 3, np.pi / 2])
cos_values = np.cos(angles)
print(f"Cos values: {cos_values}")
2.3 正切函数(tan)
使用numpy计算数组的正切值:
import numpy as np
angles = np.array([0, np.pi / 6, np.pi / 4, np.pi / 3, np.pi / 2])
tan_values = np.tan(angles)
print(f"Tan values: {tan_values}")
2.4 反三角函数
numpy库中的反三角函数与math库类似,但可以对数组进行操作:
import numpy as np
sin_values = np.array([0, 0.5, 1])
angles = np.arcsin(sin_values)
print(f"Arcsin values: {angles}")
cos_values = np.array([1, 0.5, 0])
angles = np.arccos(cos_values)
print(f"Arccos values: {angles}")
tan_values = np.array([0, 1, np.inf])
angles = np.arctan(tan_values)
print(f"Arctan values: {angles}")
三、使用scipy库中的三角函数
scipy库提供了更高级的科学计算功能,包括许多数学函数和统计函数。首先需要安装并导入scipy库:
import scipy.special as sp
3.1 球面三角函数
scipy库提供了球面三角函数,用于计算球面上的角度和距离。比如:
import scipy.special as sp
球面正弦和余弦
theta = np.pi / 4
phi = np.pi / 6
spherical_sin = sp.sph_harm(0, 0, theta, phi).real
spherical_cos = sp.sph_harm(0, 0, theta, phi).imag
print(f"Spherical Sin: {spherical_sin}, Spherical Cos: {spherical_cos}")
3.2 双曲三角函数
scipy库提供了双曲三角函数,比如双曲正弦和双曲余弦:
import scipy.special as sp
angle = 1
sinh_value = sp.sinh(angle)
cosh_value = sp.cosh(angle)
print(f"Sinh(1) = {sinh_value}, Cosh(1) = {cosh_value}")
3.3 反双曲三角函数
scipy库还提供了反双曲三角函数:
import scipy.special as sp
sinh_value = 1
angle = sp.asinh(sinh_value)
print(f"Asinh(1) = {angle}")
cosh_value = 1
angle = sp.acosh(cosh_value)
print(f"Acosh(1) = {angle}")
tanh_value = 1
angle = sp.atanh(tanh_value)
print(f"Atanh(1) = {angle}")
四、三角函数的实际应用
三角函数在实际应用中有广泛的用途,包括信号处理、图像处理、物理计算等。以下是几个常见的应用示例:
4.1 信号处理
在信号处理中,三角函数常用于生成和分析周期信号。比如,用sin函数生成一个正弦波信号:
import numpy as np
import matplotlib.pyplot as plt
t = np.linspace(0, 1, 500) # 时间序列
frequency = 5 # 频率为5Hz
signal = np.sin(2 * np.pi * frequency * t)
plt.plot(t, signal)
plt.title("5 Hz Sine Wave")
plt.xlabel("Time [s]")
plt.ylabel("Amplitude")
plt.show()
4.2 图像处理
在图像处理中,三角函数常用于滤波和边缘检测。比如,用cos函数生成一个二维余弦图像:
import numpy as np
import matplotlib.pyplot as plt
x = np.linspace(0, 2 * np.pi, 100)
y = np.linspace(0, 2 * np.pi, 100)
X, Y = np.meshgrid(x, y)
image = np.cos(X) * np.cos(Y)
plt.imshow(image, cmap='gray')
plt.title("2D Cosine Image")
plt.colorbar()
plt.show()
4.3 物理计算
在物理计算中,三角函数常用于描述波动、振动等现象。比如,用tan函数描述一个简单摆的运动:
import numpy as np
import matplotlib.pyplot as plt
t = np.linspace(0, 10, 500) # 时间序列
length = 1 # 摆长
g = 9.81 # 重力加速度
theta_0 = np.pi / 6 # 初始角度
theta = theta_0 * np.cos(np.sqrt(g / length) * t)
plt.plot(t, theta)
plt.title("Simple Pendulum Motion")
plt.xlabel("Time [s]")
plt.ylabel("Angle [rad]")
plt.show()
五、常见问题与解决方案
5.1 角度与弧度转换
在使用三角函数时,常常需要在角度和弧度之间进行转换。math库提供了math.degrees()
和math.radians()
函数:
import math
angle_in_degrees = 45
angle_in_radians = math.radians(angle_in_degrees)
print(f"45 degrees = {angle_in_radians} radians")
angle_in_radians = math.pi / 4
angle_in_degrees = math.degrees(angle_in_radians)
print(f"π/4 radians = {angle_in_degrees} degrees")
5.2 数值精度问题
在计算三角函数时,有时会遇到数值精度问题。可以使用numpy库的高精度函数或增加计算精度:
import numpy as np
angle = np.pi / 3
sin_value = np.sin(angle)
print(f"Sin(π/3) = {sin_value}")
使用高精度函数
from mpmath import mp
mp.dps = 50 # 设置精度
angle = mp.pi / 3
sin_value = mp.sin(angle)
print(f"High precision Sin(π/3) = {sin_value}")
5.3 数组操作
在处理大量数据时,使用numpy库的数组操作可以显著提高性能:
import numpy as np
angles = np.linspace(0, 2 * np.pi, 1000)
sin_values = np.sin(angles)
print(f"Sin values: {sin_values[:10]}") # 只显示前10个值
5.4 函数的可视化
可视化是理解三角函数行为的重要手段。可以使用matplotlib库进行可视化:
import numpy as np
import matplotlib.pyplot as plt
angles = np.linspace(0, 2 * np.pi, 1000)
sin_values = np.sin(angles)
cos_values = np.cos(angles)
tan_values = np.tan(angles)
plt.figure(figsize=(12, 6))
plt.subplot(3, 1, 1)
plt.plot(angles, sin_values, label="sin")
plt.title("Sine Function")
plt.xlabel("Angle [rad]")
plt.ylabel("sin(x)")
plt.grid(True)
plt.subplot(3, 1, 2)
plt.plot(angles, cos_values, label="cos")
plt.title("Cosine Function")
plt.xlabel("Angle [rad]")
plt.ylabel("cos(x)")
plt.grid(True)
plt.subplot(3, 1, 3)
plt.plot(angles, tan_values, label="tan")
plt.title("Tangent Function")
plt.xlabel("Angle [rad]")
plt.ylabel("tan(x)")
plt.grid(True)
plt.tight_layout()
plt.show()
通过以上内容,我们可以全面了解Python中如何使用三角函数,包括基本使用方法、库的选择、实际应用和常见问题解决方案。无论是初学者还是有经验的开发者,都可以通过这些示例和技巧更好地掌握三角函数的使用。
相关问答FAQs:
在Python中可以使用哪些三角函数库?
Python中常用的三角函数库主要有math
和numpy
。math
库提供了基本的三角函数,如sin()
, cos()
, tan()
等,适合处理单一数值的计算。而numpy
库则适合处理数组和矩阵中的三角函数运算,支持批量计算,提升了运算效率。
如何在Python中计算角度和弧度的三角函数?
计算三角函数时,通常需要将角度转换为弧度。在Python中,可以使用math.radians()
函数将角度转换为弧度。例如,要计算30度的正弦值,可以先将30度转换为弧度,然后调用math.sin()
函数进行计算。代码示例如下:
import math
angle_deg = 30
angle_rad = math.radians(angle_deg)
sine_value = math.sin(angle_rad)
在Python中如何绘制三角函数图像?
可以使用matplotlib
库来绘制三角函数图像。通过生成一系列角度值,计算对应的三角函数值,最后利用plt.plot()
函数将这些点连接起来。以下是绘制正弦函数图像的示例代码:
import numpy as np
import matplotlib.pyplot as plt
x = np.linspace(0, 2 * np.pi, 100) # 生成0到2π之间的100个点
y = np.sin(x) # 计算每个点的正弦值
plt.plot(x, y)
plt.title('Sine Function')
plt.xlabel('Angle (radians)')
plt.ylabel('Sine Value')
plt.grid()
plt.show()
通过以上代码,可以清晰地展示正弦函数的图形特征。
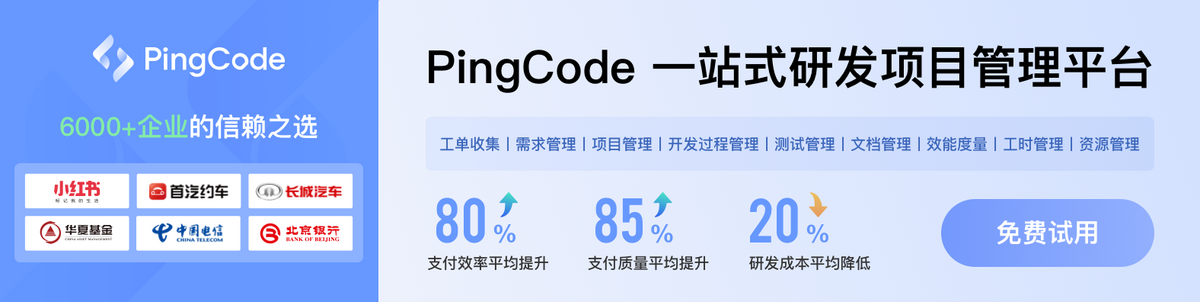