在Python中使用两个文件的方法有多种,包括导入模块、读取和写入文件、以及通过命令行参数传递文件名等。关键步骤包括:导入模块、使用open()
函数、使用命令行参数。本文将详细解释这些方法,并提供示例代码。
在Python中,使用两个文件的常见方法包括:导入模块、读取和写入文件、通过命令行参数传递文件名。其中,导入模块是指在一个文件中导入另一个Python文件作为模块使用;读取和写入文件是指在代码中操作文件进行读写;通过命令行参数传递文件名是指通过命令行传递文件名参数,并在代码中使用这些参数。下面将详细介绍这三种方法。
一、导入模块
在Python中,可以将一个文件作为模块导入到另一个文件中使用。这种方法适用于将代码分割成多个文件,以提高代码的可维护性和重用性。
1. 创建模块文件
首先,创建一个Python文件作为模块,例如module1.py
:
# module1.py
def greet(name):
return f"Hello, {name}!"
2. 导入模块并使用
在另一个Python文件中导入并使用该模块,例如main.py
:
# main.py
import module1
name = "Alice"
message = module1.greet(name)
print(message)
运行main.py
时,输出将是Hello, Alice!
。
二、读取和写入文件
在Python中,可以使用内置的open()
函数读取和写入文件。下面将介绍如何在一个文件中读取数据,并在另一个文件中写入数据。
1. 读取文件
首先,创建一个文本文件input.txt
,并在其中写入一些内容:
Hello, world!
This is a test file.
接下来,编写代码读取文件内容:
# read_file.py
def read_file(file_path):
with open(file_path, 'r') as file:
content = file.read()
return content
file_path = 'input.txt'
file_content = read_file(file_path)
print(file_content)
2. 写入文件
编写代码将读取的内容写入另一个文件:
# write_file.py
def write_file(file_path, content):
with open(file_path, 'w') as file:
file.write(content)
output_path = 'output.txt'
write_file(output_path, file_content)
运行read_file.py
和write_file.py
后,内容将被读取并写入到output.txt
文件中。
三、通过命令行参数传递文件名
在Python中,可以使用sys.argv
获取命令行参数,从而在运行时传递文件名。
1. 编写代码使用命令行参数
编写一个Python脚本file_operations.py
,从命令行参数获取文件名并进行操作:
# file_operations.py
import sys
def read_file(file_path):
with open(file_path, 'r') as file:
content = file.read()
return content
def write_file(file_path, content):
with open(file_path, 'w') as file:
file.write(content)
if len(sys.argv) != 3:
print("Usage: python file_operations.py <input_file> <output_file>")
sys.exit(1)
input_file = sys.argv[1]
output_file = sys.argv[2]
file_content = read_file(input_file)
write_file(output_file, file_content)
2. 运行脚本并传递参数
在命令行中运行脚本,并传递输入文件和输出文件名:
python file_operations.py input.txt output.txt
脚本将读取input.txt
文件的内容,并将其写入到output.txt
文件中。
四、处理文件异常
在文件操作过程中,可能会遇到各种异常情况,例如文件不存在、权限不足等。为了提高代码的健壮性,可以在文件操作时添加异常处理。
1. 添加异常处理
在读取和写入文件的函数中添加异常处理:
# file_operations_with_exception.py
import sys
def read_file(file_path):
try:
with open(file_path, 'r') as file:
content = file.read()
return content
except FileNotFoundError:
print(f"Error: File '{file_path}' not found.")
sys.exit(1)
except IOError:
print(f"Error: Could not read file '{file_path}'.")
sys.exit(1)
def write_file(file_path, content):
try:
with open(file_path, 'w') as file:
file.write(content)
except IOError:
print(f"Error: Could not write to file '{file_path}'.")
sys.exit(1)
if len(sys.argv) != 3:
print("Usage: python file_operations_with_exception.py <input_file> <output_file>")
sys.exit(1)
input_file = sys.argv[1]
output_file = sys.argv[2]
file_content = read_file(input_file)
write_file(output_file, file_content)
2. 测试异常情况
运行脚本时传递不存在的文件名或没有写权限的文件名,以测试异常处理:
python file_operations_with_exception.py nonexistent.txt output.txt
输出将是Error: File 'nonexistent.txt' not found.
。
五、使用上下文管理器
在Python中,使用上下文管理器(context manager)可以更简洁地管理文件的打开和关闭。with
语句是上下文管理器的常见用法,可以确保文件在操作完成后自动关闭。
1. 使用上下文管理器读取文件
修改读取文件的函数,使用with
语句:
# read_file_with_context.py
def read_file(file_path):
try:
with open(file_path, 'r') as file:
content = file.read()
return content
except FileNotFoundError:
print(f"Error: File '{file_path}' not found.")
sys.exit(1)
except IOError:
print(f"Error: Could not read file '{file_path}'.")
sys.exit(1)
2. 使用上下文管理器写入文件
修改写入文件的函数,使用with
语句:
# write_file_with_context.py
def write_file(file_path, content):
try:
with open(file_path, 'w') as file:
file.write(content)
except IOError:
print(f"Error: Could not write to file '{file_path}'.")
sys.exit(1)
六、处理大文件
在处理大文件时,读取整个文件内容可能会导致内存不足。可以使用逐行读取的方法,逐行处理文件内容。
1. 逐行读取文件
编写代码逐行读取文件内容:
# read_large_file.py
def read_large_file(file_path):
try:
with open(file_path, 'r') as file:
for line in file:
process_line(line)
except FileNotFoundError:
print(f"Error: File '{file_path}' not found.")
sys.exit(1)
except IOError:
print(f"Error: Could not read file '{file_path}'.")
sys.exit(1)
def process_line(line):
print(line.strip())
file_path = 'large_input.txt'
read_large_file(file_path)
2. 逐行写入文件
编写代码逐行写入文件内容:
# write_large_file.py
def write_large_file(file_path, lines):
try:
with open(file_path, 'w') as file:
for line in lines:
file.write(line + '\n')
except IOError:
print(f"Error: Could not write to file '{file_path}'.")
sys.exit(1)
lines = ["Line 1", "Line 2", "Line 3"]
output_path = 'large_output.txt'
write_large_file(output_path, lines)
七、总结
本文介绍了在Python中使用两个文件的多种方法,包括导入模块、读取和写入文件、通过命令行参数传递文件名、处理文件异常、使用上下文管理器以及处理大文件。这些方法适用于不同的应用场景,可以根据具体需求选择合适的方法进行文件操作。
通过学习和掌握这些方法,可以提高代码的可维护性、健壮性和可读性,从而更高效地完成文件操作任务。希望本文对你在Python中使用两个文件的操作有所帮助。
相关问答FAQs:
如何在Python中同时处理两个文件?
在Python中,可以使用内置的open()
函数来打开多个文件。通过使用with
语句,可以确保文件在使用后自动关闭,从而避免资源泄露。你可以同时读取两个文件的内容,进行比较或合并等操作。例如:
with open('file1.txt', 'r') as file1, open('file2.txt', 'r') as file2:
content1 = file1.read()
content2 = file2.read()
# 在这里可以对content1和content2进行操作
如何在Python中将两个文件的内容合并到一个新文件中?
合并两个文件的内容可以通过读取每个文件并将其写入新文件来实现。使用with
语句打开源文件和目标文件,读取源文件内容后,写入到目标文件中。示例代码如下:
with open('file1.txt', 'r') as file1, open('file2.txt', 'r') as file2, open('merged_file.txt', 'w') as merged_file:
merged_file.write(file1.read())
merged_file.write(file2.read())
在Python中如何处理文件读取时的错误?
在文件操作中,错误处理是一个重要的方面。使用try-except
语句可以捕获并处理文件读取时可能发生的错误。例如,如果文件不存在,程序将抛出一个异常。代码示例如下:
try:
with open('file1.txt', 'r') as file1, open('file2.txt', 'r') as file2:
content1 = file1.read()
content2 = file2.read()
except FileNotFoundError:
print("一个或多个文件未找到,请检查文件路径。")
except Exception as e:
print(f"发生了一个错误: {e}")
这种方式确保即使文件不存在或出现其他错误,程序也能够优雅地处理这些问题。
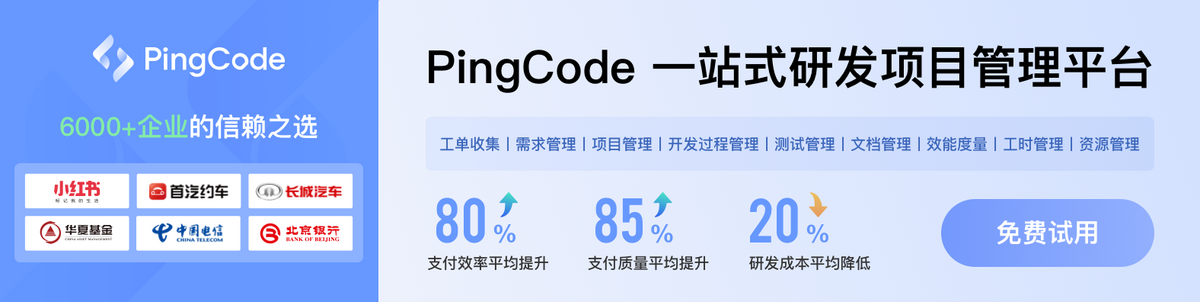