在Python中更改绘图的纵坐标单位尺度,可以使用多个库和方法,例如Matplotlib、Seaborn等。常见的方法包括使用set_yticks
、set_yticklabels
、FuncFormatter
等。下面将详细介绍如何使用这些方法进行纵坐标单位尺度的更改。
一、使用Matplotlib更改纵坐标单位尺度
Matplotlib是Python中最广泛使用的绘图库之一。它提供了丰富的功能来定制绘图,包括更改坐标轴的刻度和标签。
1、使用set_yticks
和set_yticklabels
首先,可以使用set_yticks
和set_yticklabels
方法来手动设置纵坐标的刻度和标签。
import matplotlib.pyplot as plt
创建一个简单的图形
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4], [10, 20, 25, 30])
设置纵坐标的刻度和标签
ax.set_yticks([10, 15, 20, 25, 30])
ax.set_yticklabels(['10 units', '15 units', '20 units', '25 units', '30 units'])
plt.show()
在上面的代码中,使用set_yticks
方法来设置纵坐标的刻度位置,并使用set_yticklabels
方法来设置对应的标签。
2、使用FuncFormatter
另一种方法是使用FuncFormatter
来格式化纵坐标的标签。
import matplotlib.pyplot as plt
from matplotlib.ticker import FuncFormatter
创建一个简单的图形
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4], [10, 20, 25, 30])
定义格式化函数
def y_formatter(y, pos):
return f'{y} units'
应用格式化函数
ax.yaxis.set_major_formatter(FuncFormatter(y_formatter))
plt.show()
在上面的代码中,定义了一个格式化函数y_formatter
,然后使用FuncFormatter
将其应用于纵坐标。
二、使用Seaborn更改纵坐标单位尺度
Seaborn是基于Matplotlib的高级绘图库,提供了更简单的接口来创建美观的统计图形。可以结合Matplotlib的方法来定制Seaborn图形的纵坐标单位尺度。
import seaborn as sns
import matplotlib.pyplot as plt
from matplotlib.ticker import FuncFormatter
创建一个简单的Seaborn图形
data = sns.load_dataset('tips')
ax = sns.scatterplot(x='total_bill', y='tip', data=data)
定义格式化函数
def y_formatter(y, pos):
return f'{y} units'
应用格式化函数
ax.yaxis.set_major_formatter(FuncFormatter(y_formatter))
plt.show()
在上面的代码中,使用Seaborn创建了一个散点图,然后使用Matplotlib的FuncFormatter
来更改纵坐标的标签格式。
三、使用Logarithmic Scale(对数尺度)
在某些情况下,可能需要使用对数尺度来表示数据。Matplotlib提供了简单的方法来设置对数尺度。
import matplotlib.pyplot as plt
创建一个简单的图形
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4], [10, 100, 1000, 10000])
设置纵坐标为对数尺度
ax.set_yscale('log')
plt.show()
在上面的代码中,使用set_yscale
方法将纵坐标设置为对数尺度。
四、使用科学计数法(Scientific Notation)
对于表示非常大的或非常小的数值,可以使用科学计数法。
import matplotlib.pyplot as plt
from matplotlib.ticker import ScalarFormatter
创建一个简单的图形
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4], [1e10, 2e10, 3e10, 4e10])
使用科学计数法
ax.yaxis.set_major_formatter(ScalarFormatter(useMathText=True))
ax.ticklabel_format(style='sci', axis='y', scilimits=(0,0))
plt.show()
在上面的代码中,使用ScalarFormatter
来设置科学计数法格式。
五、结合使用多个方法
可以结合上述方法来创建更复杂的图形。例如,同时使用对数尺度和自定义标签格式。
import matplotlib.pyplot as plt
from matplotlib.ticker import FuncFormatter
创建一个简单的图形
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4], [10, 100, 1000, 10000])
设置纵坐标为对数尺度
ax.set_yscale('log')
定义格式化函数
def y_formatter(y, pos):
return f'{y:.1e} units'
应用格式化函数
ax.yaxis.set_major_formatter(FuncFormatter(y_formatter))
plt.show()
在上面的代码中,结合使用了对数尺度和自定义格式化函数来创建一个复杂的图形。
总结
在Python中更改绘图的纵坐标单位尺度,可以使用多种方法和库。Matplotlib提供了丰富的功能来定制坐标轴,Seaborn可以结合Matplotlib的方法进行定制。此外,还可以使用对数尺度和科学计数法来表示数据。通过结合使用这些方法,可以创建满足各种需求的图形。
相关问答FAQs:
如何在Python绘图中更改纵坐标的单位尺度?
在Python中,可以使用Matplotlib库来绘制图形并自定义坐标轴的单位尺度。通过plt.ylim()
函数可以设置纵坐标的范围,而通过plt.yticks()
函数可以调整纵坐标的刻度值和标签。示例代码如下:
import matplotlib.pyplot as plt
# 示例数据
x = [1, 2, 3, 4, 5]
y = [10, 20, 30, 40, 50]
plt.plot(x, y)
plt.ylim(0, 60) # 设置纵坐标范围
plt.yticks([0, 10, 20, 30, 40, 50, 60], ['0', '10', '20', '30', '40', '50', '60']) # 自定义刻度标签
plt.show()
在Python中如何将纵坐标转换为对数尺度?
如果需要对纵坐标进行对数变换,可以使用Matplotlib中的plt.yscale('log')
。这将使纵坐标以对数尺度显示,适合处理大范围的数据。示例代码如下:
plt.plot(x, y)
plt.yscale('log') # 将纵坐标设置为对数尺度
plt.show()
如何使用Seaborn更改纵坐标的单位尺度?
Seaborn是基于Matplotlib的一个绘图库,使用Seaborn绘制图形时,也可以通过Matplotlib的方式来调整纵坐标的单位尺度。例如,可以在绘制图形后调用plt.ylim()
和plt.yticks()
,或者直接使用Seaborn的sns.set()
进行全局设置。示例代码如下:
import seaborn as sns
sns.lineplot(x=x, y=y)
plt.ylim(0, 60) # 设置纵坐标范围
plt.show()
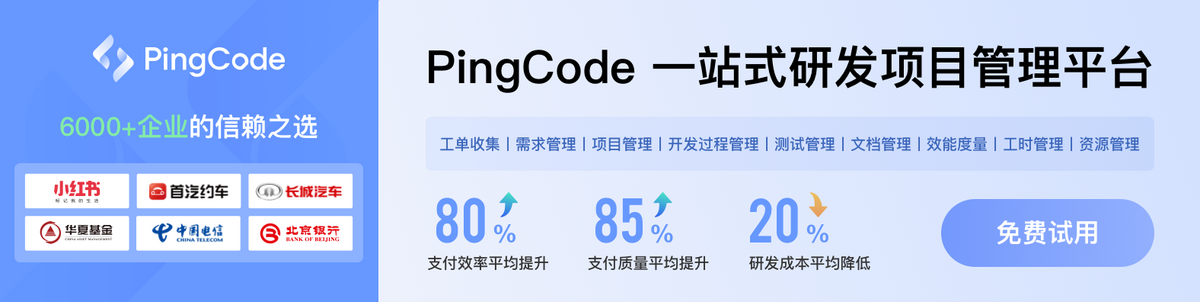