Python中可以通过多种方法实现替换多个字符串。使用replace()方法、使用正则表达式、通过字典映射、使用字符串的translate()方法等。其中,通过字典映射是一种常见且高效的方法。接下来,我将详细介绍这种方法。
要替换多个字符串,可以将待替换的字符串和对应的新字符串存储在字典中,然后遍历字典并使用字符串的replace()方法进行替换。这样可以清晰地管理和替换多个字符串。
def replace_multiple_strings(text, replacements):
for old, new in replacements.items():
text = text.replace(old, new)
return text
text = "Hello, world! Welcome to the Python world."
replacements = {
"Hello": "Hi",
"world": "universe",
"Python": "programming"
}
new_text = replace_multiple_strings(text, replacements)
print(new_text)
在上述代码中,我们定义了一个函数replace_multiple_strings
,它接受一个字符串和一个包含替换对的字典。通过遍历字典中的每一对键值对,利用replace()
方法逐个替换字符串。
一、使用正则表达式
使用正则表达式也是替换多个字符串的有效方法。通过正则表达式,我们可以一次性匹配多个模式并进行替换。
import re
def replace_multiple_strings_regex(text, replacements):
pattern = re.compile("|".join(re.escape(key) for key in replacements.keys()))
return pattern.sub(lambda match: replacements[match.group(0)], text)
text = "Hello, world! Welcome to the Python world."
replacements = {
"Hello": "Hi",
"world": "universe",
"Python": "programming"
}
new_text = replace_multiple_strings_regex(text, replacements)
print(new_text)
在上述代码中,我们使用了re.compile()
来编译一个正则表达式模式,该模式匹配字典中的所有键。然后,使用pattern.sub()
方法进行替换,匹配的字符串将被替换为字典中的相应值。
二、使用字符串的translate()方法
translate()方法结合str.maketrans()方法也可以实现多字符串替换,但这种方法通常用于单字符替换。
def replace_multiple_characters(text, replacements):
translation_table = str.maketrans(replacements)
return text.translate(translation_table)
text = "Hello, world! Welcome to the Python world."
replacements = {
"H": "h",
"w": "W",
"P": "p"
}
new_text = replace_multiple_characters(text, replacements)
print(new_text)
在上述代码中,我们使用str.maketrans()
创建一个翻译表,然后使用translate()
方法进行替换。由于translate()
方法是针对单字符的替换,因此适用于较简单的替换需求。
三、通过自定义函数实现多字符串替换
我们还可以定义一个更通用的函数来实现多字符串替换,这样可以在替换过程中进行更多的控制和处理。
def custom_replace(text, replacements):
for old, new in replacements.items():
text = text.replace(old, new)
return text
text = "Hello, world! Welcome to the Python world."
replacements = {
"Hello": "Hi",
"world": "universe",
"Python": "programming",
"Welcome": "Greetings"
}
new_text = custom_replace(text, replacements)
print(new_text)
在上述代码中,我们定义了一个名为custom_replace
的函数,该函数接受一个字符串和一个包含替换对的字典。通过遍历字典中的每一对键值对,利用replace()
方法逐个替换字符串。
四、使用第三方库
对于更复杂的替换需求,我们可以借助第三方库,如multistr
,该库提供了更强大和灵活的多字符串替换功能。
from multistr import multistr
text = "Hello, world! Welcome to the Python world."
replacements = {
"Hello": "Hi",
"world": "universe",
"Python": "programming",
"Welcome": "Greetings"
}
new_text = multistr(text).replace(replacements)
print(new_text)
在上述代码中,我们使用multistr
库的replace
方法进行多字符串替换。该库提供了更简洁和高效的替换功能,非常适合复杂的替换需求。
五、总结
在Python中替换多个字符串的方法有很多种,常见的方法包括使用replace()方法、使用正则表达式、通过字典映射、使用字符串的translate()方法等。选择哪种方法取决于具体的需求和应用场景。通过以上介绍和示例代码,相信你已经掌握了多种替换多个字符串的技巧和方法。希望这些内容对你有所帮助!
相关问答FAQs:
如何在Python中同时替换多个字符串?
在Python中,可以使用str.replace()
方法逐个替换字符串,但当需要替换多个字符串时,使用字典结合str.translate()
和str.maketrans()
方法会更高效。例如,可以创建一个替换映射字典,然后使用这两个函数来替换多个字符串,代码示例如下:
replacements = str.maketrans({"old1": "new1", "old2": "new2"})
result = original_string.translate(replacements)
有没有使用正则表达式来替换多个字符串的方式?
使用Python的re
模块可以通过正则表达式实现多个字符串的替换。可以利用re.sub()
函数配合一个模式字符串和替换函数来实现。例如,可以将需要替换的字符串放入一个列表中,构建正则表达式并替换:
import re
def replace_multiple(original_string):
replacements = {"old1": "new1", "old2": "new2"}
pattern = re.compile("|".join(re.escape(key) for key in replacements.keys()))
return pattern.sub(lambda match: replacements[match.group(0)], original_string)
在Python中如何处理大小写敏感的字符串替换?
处理大小写敏感的字符串替换可以使用re
模块的re.IGNORECASE
标志。在使用正则表达式时,可以指定该标志来实现不区分大小写的替换。这对于用户在处理文本时非常有用,确保替换能覆盖所有情况。例如:
import re
def replace_case_insensitive(original_string):
replacements = {"old": "new"}
pattern = re.compile(re.escape("old"), re.IGNORECASE)
return pattern.sub(replacements["old"], original_string)
通过这些方法,可以灵活地在Python中实现多个字符串的替换,满足不同场景的需求。
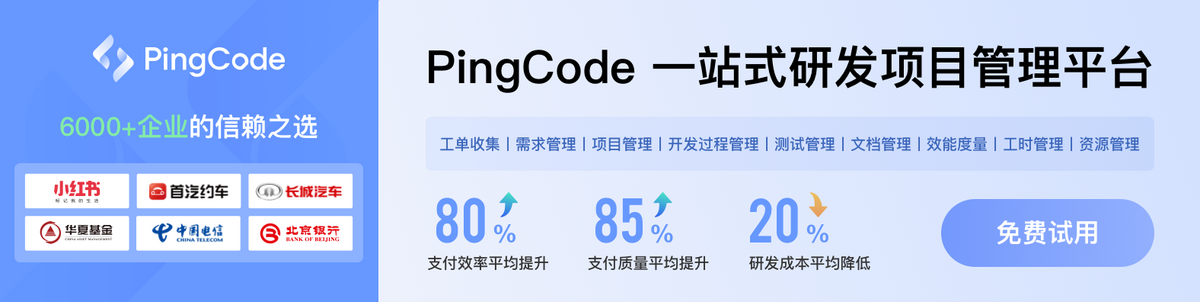