在Python中操作文件中的数据,主要通过内置的open()
函数来实现。使用open()
函数可以读取文件内容、写入数据到文件、修改文件内容等。以下是几个常用方法:读取文件内容、写入数据到文件、使用with
语句管理文件上下文。 详细描述如下:
读取文件内容:
with open('example.txt', 'r') as file:
content = file.read()
print(content)
写入数据到文件:
with open('example.txt', 'w') as file:
file.write('Hello, World!')
使用with
语句管理文件上下文:
with open('example.txt', 'r') as file:
for line in file:
print(line)
以上是概述,下面将详细介绍在Python中如何操作文件中的数据,包括各种文件操作的具体方法和注意事项。
一、文件操作的基本概念
在开始操作文件之前,理解一些基本概念是非常有必要的:
- 文件路径:文件路径可以是绝对路径或相对路径。绝对路径是文件在系统中的完整路径,而相对路径是相对于当前工作目录的路径。
- 文件模式:Python的
open()
函数接受一个模式参数,用于指定文件的操作模式。常见模式包括:'r'
:只读模式(默认)。'w'
:写入模式。如果文件不存在会创建文件,如果文件存在会覆盖文件。'a'
:追加模式。如果文件不存在会创建文件,如果文件存在会在文件末尾追加内容。'b'
:二进制模式。与其他模式结合使用,如'rb'
表示以二进制模式读取文件。
二、读取文件内容
读取文件内容是文件操作的最基本的任务之一,以下是几种常用的方法:
1. 使用read()
方法读取整个文件
with open('example.txt', 'r') as file:
content = file.read()
print(content)
这种方法适合读取较小的文件,因为它会将整个文件内容加载到内存中。
2. 使用readline()
方法读取文件的一行
with open('example.txt', 'r') as file:
line = file.readline()
while line:
print(line, end='')
line = file.readline()
这种方法适合逐行处理文件内容。
3. 使用readlines()
方法读取文件的所有行
with open('example.txt', 'r') as file:
lines = file.readlines()
for line in lines:
print(line, end='')
这种方法会将文件的所有行作为列表返回,每行作为列表的一项。
三、写入数据到文件
写入数据到文件也是非常常见的操作,以下是几种常用的方法:
1. 使用write()
方法写入数据
with open('example.txt', 'w') as file:
file.write('Hello, World!')
这种方法适合写入简单的字符串数据。
2. 使用writelines()
方法写入多行数据
lines = ['First line\n', 'Second line\n', 'Third line\n']
with open('example.txt', 'w') as file:
file.writelines(lines)
这种方法适合写入多个字符串数据。
四、追加数据到文件
有时候需要在文件末尾追加数据,可以使用追加模式:
with open('example.txt', 'a') as file:
file.write('This is an appended line.\n')
五、使用with
语句管理文件上下文
使用with
语句可以确保文件正确关闭,即使在发生异常时:
with open('example.txt', 'r') as file:
for line in file:
print(line)
六、处理二进制文件
处理二进制文件(如图片、音频文件等)时,需要使用二进制模式:
1. 读取二进制文件
with open('example.png', 'rb') as file:
content = file.read()
print(content)
2. 写入二进制文件
with open('example.png', 'wb') as file:
file.write(content)
七、文件操作的高级技巧
1. 使用seek()
和tell()
方法
seek()
方法可以移动文件的读取指针,tell()
方法可以返回当前文件指针的位置:
with open('example.txt', 'r') as file:
file.seek(5)
content = file.read()
print(content)
print('Current position:', file.tell())
2. 使用os
模块进行文件操作
os
模块提供了许多文件和目录操作的函数,如删除文件、重命名文件等:
import os
删除文件
os.remove('example.txt')
重命名文件
os.rename('old_name.txt', 'new_name.txt')
创建目录
os.mkdir('new_directory')
删除目录
os.rmdir('new_directory')
3. 使用shutil
模块进行高级文件操作
shutil
模块提供了高级的文件操作,如复制文件、移动文件等:
import shutil
复制文件
shutil.copy('source.txt', 'destination.txt')
移动文件
shutil.move('source.txt', 'destination.txt')
八、处理CSV文件
CSV文件是一种常见的文件格式,Python的csv
模块可以方便地处理CSV文件:
1. 读取CSV文件
import csv
with open('example.csv', 'r') as file:
reader = csv.reader(file)
for row in reader:
print(row)
2. 写入CSV文件
import csv
with open('example.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerow(['name', 'age', 'city'])
writer.writerow(['Alice', 30, 'New York'])
writer.writerow(['Bob', 25, 'San Francisco'])
九、处理JSON文件
JSON文件是一种常见的数据交换格式,Python的json
模块可以方便地处理JSON文件:
1. 读取JSON文件
import json
with open('example.json', 'r') as file:
data = json.load(file)
print(data)
2. 写入JSON文件
import json
data = {'name': 'Alice', 'age': 30, 'city': 'New York'}
with open('example.json', 'w') as file:
json.dump(data, file)
十、文件操作的最佳实践
1. 使用with
语句管理文件上下文
使用with
语句可以确保文件正确关闭,避免资源泄漏。
2. 处理大文件时使用逐行读取
处理大文件时,避免一次性读取整个文件内容,使用逐行读取的方法。
3. 使用异常处理
文件操作时可能会发生异常,如文件不存在、权限不足等,使用异常处理可以提高程序的健壮性:
try:
with open('example.txt', 'r') as file:
content = file.read()
print(content)
except FileNotFoundError:
print('File not found')
except PermissionError:
print('Permission denied')
十一、总结
Python提供了丰富的文件操作功能,可以方便地读取、写入、修改文件内容。通过使用open()
函数和with
语句,可以高效地管理文件上下文,避免资源泄漏。处理不同类型的文件(如文本文件、二进制文件、CSV文件、JSON文件)时,可以使用相应的模块和方法。此外,使用os
和shutil
模块可以进行更高级的文件操作,如删除文件、重命名文件、复制文件、移动文件等。在实际应用中,遵循文件操作的最佳实践,可以提高程序的健壮性和可维护性。
相关问答FAQs:
在Python中,如何读取文本文件的内容?
在Python中,读取文本文件的内容可以通过内置的open()
函数实现。使用open()
函数打开文件后,可以调用read()
方法读取整个文件的内容,或者使用readline()
逐行读取。最后,别忘了使用close()
方法关闭文件,以释放系统资源。示例代码如下:
with open('example.txt', 'r') as file:
content = file.read()
print(content)
使用with
语句可以确保文件在操作完成后自动关闭,推荐使用这种方式。
如何在Python中向文件写入数据?
在Python中,可以使用open()
函数以写入模式('w')或追加模式('a')打开文件,然后使用write()
方法将数据写入文件。写入模式会覆盖文件中的现有内容,而追加模式则会在文件末尾添加内容。下面是一个写入文件的例子:
with open('example.txt', 'w') as file:
file.write('Hello, World!\n')
确保在写入完成后关闭文件,或者使用with
语句来自动管理文件的打开和关闭。
如何处理文件中的数据并进行格式化?
在处理文件中的数据时,可以使用Python的字符串方法和数据结构来格式化数据。例如,可以读取文件内容后,将数据分割成列表,并使用循环结构进行处理。对于格式化输出,可以使用format()
方法或f-strings来提高可读性。示例代码如下:
with open('data.txt', 'r') as file:
for line in file:
data = line.strip().split(',')
formatted_data = f'Name: {data[0]}, Age: {data[1]}'
print(formatted_data)
这种方式让数据处理更加灵活,同时也提高了信息呈现的清晰度。
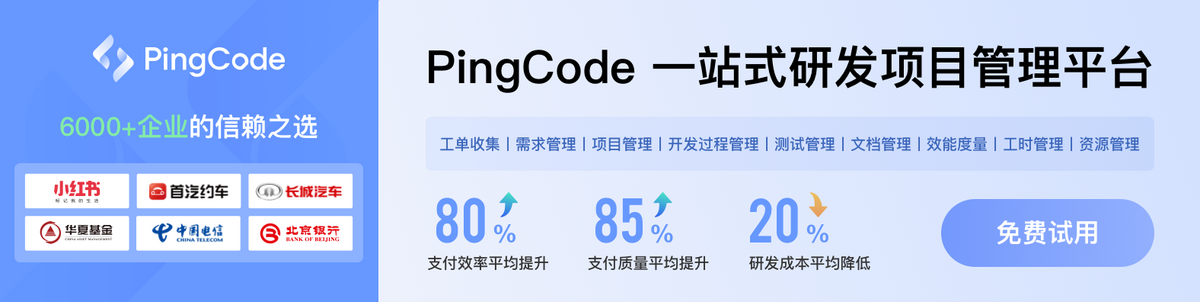