使用Python将输出结果写入文件的方法有多种,包括使用内置的open
函数、with
语句以及库函数。常见的方法有:使用open
函数、使用with
语句、使用csv
模块、使用pandas
库。 其中,最常用和推荐的方法是使用with
语句和open
函数相结合,因为这样可以更好地管理文件资源,避免资源泄漏。
一、使用open
函数
使用open
函数打开一个文件,并使用write
方法将内容写入文件。使用open
函数时,需要指定文件路径和模式(如w
表示写入模式,a
表示追加模式)。
# 示例:将结果写入文件
result = "Hello, World!"
file = open('output.txt', 'w')
file.write(result)
file.close()
然而,这种方法需要手动关闭文件,这可能会导致文件未关闭的风险。
二、使用with
语句
使用with
语句可以自动管理文件资源,无需手动关闭文件,确保文件在操作完成后正确关闭。
# 示例:使用with语句将结果写入文件
result = "Hello, World!"
with open('output.txt', 'w') as file:
file.write(result)
这种方法是最常用和推荐的,因为它简化了资源管理,避免了文件资源泄漏。
三、使用csv
模块
如果需要将数据写入CSV文件,可以使用Python内置的csv
模块。csv
模块提供了方便的接口来处理CSV文件。
import csv
示例:将结果写入CSV文件
data = [
['Name', 'Age'],
['Alice', 30],
['Bob', 25]
]
with open('output.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerows(data)
四、使用pandas
库
如果处理的是数据框,可以使用pandas
库,该库提供了强大的数据处理和文件读写功能。
import pandas as pd
示例:将数据写入CSV文件
data = {
'Name': ['Alice', 'Bob'],
'Age': [30, 25]
}
df = pd.DataFrame(data)
df.to_csv('output.csv', index=False)
五、写入JSON文件
如果需要将数据写入JSON文件,可以使用Python内置的json
模块。json
模块提供了方便的接口来处理JSON文件。
import json
示例:将数据写入JSON文件
data = {
'Name': 'Alice',
'Age': 30
}
with open('output.json', 'w') as file:
json.dump(data, file)
六、写入Excel文件
如果需要将数据写入Excel文件,可以使用pandas
库,该库提供了强大的数据处理和文件读写功能。
import pandas as pd
示例:将数据写入Excel文件
data = {
'Name': ['Alice', 'Bob'],
'Age': [30, 25]
}
df = pd.DataFrame(data)
df.to_excel('output.xlsx', index=False)
七、写入二进制文件
如果需要将数据写入二进制文件,可以使用wb
模式打开文件。
# 示例:将二进制数据写入文件
data = b'\x00\x01\x02\x03\x04\x05'
with open('output.bin', 'wb') as file:
file.write(data)
八、写入多个文件
如果需要将多个结果写入多个文件,可以在循环中使用with
语句和open
函数。
# 示例:将多个结果写入多个文件
results = ["Hello, World!", "Python is great!", "File handling in Python"]
for i, result in enumerate(results):
with open(f'output_{i}.txt', 'w') as file:
file.write(result)
九、写入日志文件
如果需要将日志信息写入文件,可以使用Python内置的logging
模块。logging
模块提供了灵活的日志处理接口。
import logging
设置日志配置
logging.basicConfig(filename='app.log', filemode='w', format='%(name)s - %(levelname)s - %(message)s', level=logging.INFO)
示例:将日志信息写入文件
logging.info('This is an info message')
logging.warning('This is a warning message')
logging.error('This is an error message')
十、写入HTML文件
如果需要将数据写入HTML文件,可以将数据格式化为HTML字符串,然后写入文件。
# 示例:将数据写入HTML文件
html_content = """
<!DOCTYPE html>
<html>
<head>
<title>Output</title>
</head>
<body>
<h1>Hello, World!</h1>
<p>This is an example of writing HTML content to a file.</p>
</body>
</html>
"""
with open('output.html', 'w') as file:
file.write(html_content)
十一、写入XML文件
如果需要将数据写入XML文件,可以使用xml.etree.ElementTree
模块。
import xml.etree.ElementTree as ET
示例:将数据写入XML文件
root = ET.Element("root")
child = ET.SubElement(root, "child")
child.text = "Hello, World!"
tree = ET.ElementTree(root)
tree.write("output.xml")
十二、写入配置文件
如果需要将数据写入配置文件,可以使用configparser
模块。configparser
模块提供了方便的接口来处理配置文件。
import configparser
示例:将数据写入配置文件
config = configparser.ConfigParser()
config['DEFAULT'] = {'ServerAliveInterval': '45',
'Compression': 'yes',
'CompressionLevel': '9'}
config['bitbucket.org'] = {}
config['bitbucket.org']['User'] = 'hg'
config['topsecret.server.com'] = {}
topsecret = config['topsecret.server.com']
topsecret['Host Port'] = '50022'
topsecret['ForwardX11'] = 'no'
with open('example.ini', 'w') as configfile:
config.write(configfile)
十三、写入YAML文件
如果需要将数据写入YAML文件,可以使用PyYAML
库。PyYAML
库提供了方便的接口来处理YAML文件。
import yaml
示例:将数据写入YAML文件
data = {
'name': 'Alice',
'age': 30,
'children': ['Bob', 'Charlie']
}
with open('output.yaml', 'w') as file:
yaml.dump(data, file)
十四、写入压缩文件
如果需要将数据写入压缩文件,可以使用gzip
或zipfile
模块。
import gzip
示例:将数据写入gzip压缩文件
data = b"Hello, World!"
with gzip.open('output.txt.gz', 'wb') as file:
file.write(data)
十五、使用第三方库
如果需要更多高级功能,可以使用第三方库,例如h5py
用于处理HDF5文件,xlwt
用于处理Excel文件等。
import h5py
示例:将数据写入HDF5文件
data = [1, 2, 3, 4, 5]
with h5py.File('output.h5', 'w') as file:
file.create_dataset('dataset', data=data)
通过上述方法,可以根据具体需求选择合适的方式将输出结果写入文件。无论是简单的文本文件、CSV文件、JSON文件,还是更复杂的二进制文件、配置文件,都能轻松应对。使用with
语句和open
函数是最基本和推荐的方法,确保文件操作的安全性和简洁性。
相关问答FAQs:
如何在Python中将输出结果写入文件?
在Python中,可以使用内置的open()
函数来创建或打开一个文件,并使用write()
方法将输出结果写入该文件。示例代码如下:
with open('output.txt', 'w') as file:
file.write('这是要写入文件的内容。')
这种方法不仅简洁易懂,还能确保文件在写入后自动关闭,避免资源泄露。
使用Python写入文件时,有哪些常见的模式可选择?
Python的open()
函数支持多种文件打开模式,包括:
'w'
:写入模式,若文件已存在则会被覆盖。'a'
:追加模式,若文件已存在则会在文件末尾添加内容。'r+'
:读写模式,允许对文件进行读和写操作。
根据具体需求选择合适的模式,可以更好地管理文件内容。
如何确保写入文件的内容是正确的?
为了确保写入的内容正确,建议在写入文件之前进行数据验证。可以使用print()
函数将输出结果打印到控制台,并仔细检查。还可以在写入文件后,重新打开文件并读取内容,以确认写入操作的成功与否。示例代码如下:
with open('output.txt', 'w') as file:
file.write('测试内容。')
with open('output.txt', 'r') as file:
content = file.read()
print(content) # 验证写入的内容
通过这种方式,可以有效避免因错误写入而导致的数据丢失或错误。
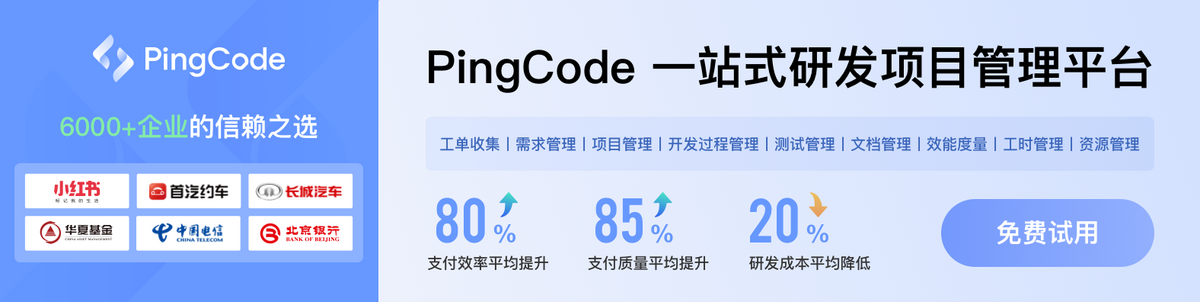