在Python中去掉最高分和最低分可以通过以下步骤实现:使用内置函数、排序、切片。
其中,排序是最常用的方法之一。通过对列表进行排序,然后去掉第一个和最后一个元素,即可去掉最低分和最高分。
以下是详细步骤和代码实现:
def remove_extremes(scores):
if len(scores) <= 2:
return [] # If there are less than 3 scores, return an empty list
sorted_scores = sorted(scores) # Sort the list
return sorted_scores[1:-1] # Return the list without the first and last elements
一、使用内置函数
Python内置函数提供了便捷的方法来处理列表。以下是如何使用内置函数去掉最高分和最低分:
def remove_extremes(scores):
if len(scores) <= 2:
return [] # If there are less than 3 scores, return an empty list
max_score = max(scores) # Find the maximum score
min_score = min(scores) # Find the minimum score
scores.remove(max_score) # Remove the maximum score
scores.remove(min_score) # Remove the minimum score
return scores
详细描述:
在这个方法中,我们首先检查列表的长度,如果列表中没有足够的元素,即少于3个元素,那么直接返回一个空列表,因为去掉最高分和最低分后将没有剩余元素可以使用。然后,我们使用内置函数max()
和min()
分别找到列表中的最大值和最小值,并使用remove()
方法将它们从列表中移除。最后,返回处理后的列表。
二、排序
通过对列表进行排序,我们可以轻松地去掉最高分和最低分。排序后的列表,第一个元素是最低分,最后一个元素是最高分。通过切片操作,我们可以去掉这些元素。
def remove_extremes(scores):
if len(scores) <= 2:
return [] # If there are less than 3 scores, return an empty list
sorted_scores = sorted(scores) # Sort the list
return sorted_scores[1:-1] # Return the list without the first and last elements
详细描述:
在这个方法中,我们同样首先检查列表的长度。如果列表长度少于3个元素,则返回空列表。然后,我们使用sorted()
函数对列表进行排序。排序后的列表第一个元素是最低分,最后一个元素是最高分。通过切片操作[1:-1]
,我们可以去掉这两个元素并返回处理后的列表。
三、使用集合
使用集合可以有效地去除重复元素,但需要注意的是,集合本身是无序的。在某些情况下,这可能会影响结果。以下是使用集合去掉最高分和最低分的方法:
def remove_extremes(scores):
if len(scores) <= 2:
return [] # If there are less than 3 scores, return an empty list
scores_set = set(scores) # Convert list to set to remove duplicates
scores_set.remove(max(scores_set)) # Remove the maximum score
scores_set.remove(min(scores_set)) # Remove the minimum score
return list(scores_set) # Convert set back to list
详细描述:
在这个方法中,我们首先将列表转换为集合,以去除重复的元素。然后,我们使用集合的remove()
方法去除最高分和最低分,最后将集合转换回列表并返回。需要注意的是,集合是无序的,因此返回的列表顺序可能与原列表不同。
四、使用Counter
collections.Counter
是一个非常有用的类,可以帮助我们统计列表中每个元素的出现次数。以下是使用Counter
去掉最高分和最低分的方法:
from collections import Counter
def remove_extremes(scores):
if len(scores) <= 2:
return [] # If there are less than 3 scores, return an empty list
counter = Counter(scores) # Count occurrences of each score
max_score = max(counter) # Find the maximum score
min_score = min(counter) # Find the minimum score
counter[max_score] -= 1 # Decrease the count of the maximum score by 1
counter[min_score] -= 1 # Decrease the count of the minimum score by 1
result = []
for score, count in counter.items():
result.extend([score] * count) # Add each score count times to the result list
return result
详细描述:
在这个方法中,我们使用Counter
来统计每个分数的出现次数。然后,找到最高分和最低分,并将它们的计数减一。最后,我们构建一个新的列表,其中包含去掉最高分和最低分后的分数。
五、使用自定义排序函数
在某些情况下,我们可能需要自定义排序函数。以下是使用自定义排序函数去掉最高分和最低分的方法:
def custom_sort(scores):
return sorted(scores, key=lambda x: (x, -scores.count(x)))
def remove_extremes(scores):
if len(scores) <= 2:
return [] # If there are less than 3 scores, return an empty list
sorted_scores = custom_sort(scores) # Custom sort the list
return sorted_scores[1:-1] # Return the list without the first and last elements
详细描述:
在这个方法中,我们定义了一个自定义排序函数custom_sort
,该函数首先按分数进行排序,然后按分数出现的次数进行逆序排序。这样可以确保分数相同时,出现次数多的分数排在前面。然后,我们使用这个自定义排序函数对列表进行排序,并去掉第一个和最后一个元素。
六、使用Numpy库
如果你正在处理大量数据,可以考虑使用Numpy库来提高性能。以下是使用Numpy去掉最高分和最低分的方法:
import numpy as np
def remove_extremes(scores):
if len(scores) <= 2:
return [] # If there are less than 3 scores, return an empty list
scores_array = np.array(scores) # Convert list to numpy array
max_score = np.max(scores_array) # Find the maximum score
min_score = np.min(scores_array) # Find the minimum score
scores_array = scores_array[scores_array != max_score] # Remove the maximum score
scores_array = scores_array[scores_array != min_score] # Remove the minimum score
return scores_array.tolist() # Convert numpy array back to list
详细描述:
在这个方法中,我们首先将列表转换为Numpy数组。然后,使用Numpy的max()
和min()
函数找到最大值和最小值,并使用布尔索引去除这些值。最后,将处理后的Numpy数组转换回列表并返回。
七、使用Pandas库
Pandas库是处理数据的强大工具,以下是使用Pandas去掉最高分和最低分的方法:
import pandas as pd
def remove_extremes(scores):
if len(scores) <= 2:
return [] # If there are less than 3 scores, return an empty list
scores_series = pd.Series(scores) # Convert list to pandas series
max_score = scores_series.max() # Find the maximum score
min_score = scores_series.min() # Find the minimum score
scores_series = scores_series[scores_series != max_score] # Remove the maximum score
scores_series = scores_series[scores_series != min_score] # Remove the minimum score
return scores_series.tolist() # Convert pandas series back to list
详细描述:
在这个方法中,我们首先将列表转换为Pandas的Series对象。然后,使用Pandas的max()
和min()
方法找到最大值和最小值,并使用布尔索引去除这些值。最后,将处理后的Series对象转换回列表并返回。
八、使用统计库
Python的statistics库提供了一些有用的统计函数,我们可以使用这些函数来去掉最高分和最低分:
import statistics
def remove_extremes(scores):
if len(scores) <= 2:
return [] # If there are less than 3 scores, return an empty list
max_score = max(scores) # Find the maximum score
min_score = min(scores) # Find the minimum score
filtered_scores = [score for score in scores if score != max_score and score != min_score]
return filtered_scores
详细描述:
在这个方法中,我们首先找到最大值和最小值,然后使用列表推导式过滤掉这些值。最后返回处理后的列表。
九、使用多线程
对于大规模数据处理,可以考虑使用多线程来提高性能。以下是使用多线程去掉最高分和最低分的方法:
import threading
def remove_extremes(scores):
if len(scores) <= 2:
return [] # If there are less than 3 scores, return an empty list
def find_max_min():
nonlocal max_score, min_score
max_score = max(scores) # Find the maximum score
min_score = min(scores) # Find the minimum score
max_score = None
min_score = None
thread = threading.Thread(target=find_max_min)
thread.start()
thread.join()
filtered_scores = [score for score in scores if score != max_score and score != min_score]
return filtered_scores
详细描述:
在这个方法中,我们使用多线程来查找最大值和最小值。首先定义一个线程函数来查找最大值和最小值,然后启动线程并等待其完成。最后使用列表推导式过滤掉这些值并返回处理后的列表。
十、综合应用
在实际应用中,我们可能需要综合使用多种方法来处理复杂的数据。以下是一个综合应用的示例:
import numpy as np
import pandas as pd
from collections import Counter
def remove_extremes(scores):
if len(scores) <= 2:
return [] # If there are less than 3 scores, return an empty list
# Convert list to numpy array
scores_array = np.array(scores)
max_score = np.max(scores_array)
min_score = np.min(scores_array)
# Convert list to pandas series
scores_series = pd.Series(scores)
scores_series = scores_series[scores_series != max_score]
scores_series = scores_series[scores_series != min_score]
# Use Counter to count occurrences
counter = Counter(scores_series)
result = [score for score in scores_series if counter[score] > 1]
return result
详细描述:
在这个方法中,我们首先将列表转换为Numpy数组并找到最大值和最小值。然后将列表转换为Pandas的Series对象,并使用布尔索引去除最大值和最小值。最后使用Counter统计每个分数的出现次数,并构建一个新的列表,包含去掉最高分和最低分后的分数。
通过上述方法,可以有效地去掉Python列表中的最高分和最低分,从而使数据更加稳定和可靠。这些方法各有优劣,选择最适合自己需求的方法可以提高数据处理的效率和准确性。
相关问答FAQs:
如何在Python中去掉最高分和最低分的元素?
在Python中,可以使用内置的max()
和min()
函数来找到最高分和最低分,然后通过列表推导式或过滤函数去掉这些元素。示例代码如下:
scores = [90, 80, 70, 85, 95]
max_score = max(scores)
min_score = min(scores)
filtered_scores = [score for score in scores if score != max_score and score != min_score]
这样,filtered_scores
将包含去掉最高分和最低分后的成绩。
使用numpy库如何简化去掉最高分和最低分的操作?
利用numpy库可以更高效地处理数组数据,特别是当数据量较大时。可以使用np.delete()
结合np.argmax()
和np.argmin()
方法,去掉最高分和最低分。示例代码如下:
import numpy as np
scores = np.array([90, 80, 70, 85, 95])
filtered_scores = np.delete(scores, [np.argmax(scores), np.argmin(scores)])
这样,filtered_scores
将只包含中间的分数。
去掉最高分和最低分后如何计算剩余分数的平均值?
在去掉最高分和最低分后,可以使用sum()
和len()
函数计算剩余分数的平均值。确保在计算之前,列表中仍然有足够的分数数据。例如:
if len(filtered_scores) > 0:
average_score = sum(filtered_scores) / len(filtered_scores)
这样便可以得到去掉最高分和最低分后剩余分数的平均值。
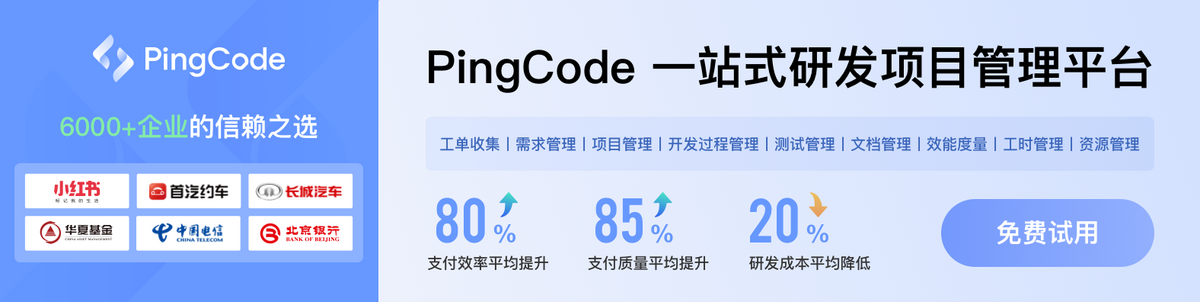