使用Matplotlib为Python的柱形图添加数据标签,使用text
方法、使用annotate
方法、通过库如Seaborn、通过库如Pandas
Python的Matplotlib库提供了多种方法可以为柱形图添加数据标签。最常用的方法是使用text
方法直接在柱形图上添加文本标签。这种方法可以帮助我们更清晰地展示每个柱子的数值,更好地理解和分析数据。
一、使用text方法
text
方法允许我们在指定的位置添加文本标签。我们可以通过调整文本的位置和样式,使其与柱形图完美契合。示例如下:
import matplotlib.pyplot as plt
import numpy as np
数据
categories = ['A', 'B', 'C', 'D']
values = [10, 15, 7, 20]
创建柱形图
fig, ax = plt.subplots()
bars = ax.bar(categories, values)
添加数据标签
for bar in bars:
height = bar.get_height()
ax.text(bar.get_x() + bar.get_width() / 2, height, f'{height}', ha='center', va='bottom')
plt.show()
在上面的示例中,我们首先创建了一个简单的柱形图,然后使用text
方法在每个柱子的顶部添加了对应的数值标签。通过bar.get_x()
和bar.get_width()
我们可以准确地确定文本的横向位置,而bar.get_height()
则用于确定文本的纵向位置。
二、使用annotate方法
annotate
方法提供了更多的功能和灵活性,例如可以添加箭头指向某个点。示例如下:
import matplotlib.pyplot as plt
import numpy as np
数据
categories = ['A', 'B', 'C', 'D']
values = [10, 15, 7, 20]
创建柱形图
fig, ax = plt.subplots()
bars = ax.bar(categories, values)
添加数据标签
for bar in bars:
height = bar.get_height()
ax.annotate(f'{height}', xy=(bar.get_x() + bar.get_width() / 2, height),
xytext=(0, 3), textcoords="offset points", ha='center', va='bottom')
plt.show()
在这个示例中,我们使用annotate
方法添加标签。xytext
参数用来指定文本的偏移量,以确保文本不会与柱子重叠。
三、通过库如Seaborn
Seaborn是一个基于Matplotlib的高级绘图库,提供了更为简便的方法来创建带有数据标签的柱形图。例如:
import seaborn as sns
import matplotlib.pyplot as plt
数据
tips = sns.load_dataset("tips")
创建柱形图
ax = sns.barplot(x="day", y="total_bill", data=tips)
添加数据标签
for p in ax.patches:
ax.annotate(format(p.get_height(), '.1f'),
(p.get_x() + p.get_width() / 2, p.get_height()),
ha='center', va='center',
xytext=(0, 9),
textcoords='offset points')
plt.show()
在这个示例中,我们使用Seaborn的barplot
方法创建了一个柱形图,然后通过annotate
方法为每个柱子添加了数据标签。
四、通过库如Pandas
Pandas库也可以用于创建和标注柱形图。例如:
import pandas as pd
import matplotlib.pyplot as plt
数据
data = {'Category': ['A', 'B', 'C', 'D'], 'Values': [10, 15, 7, 20]}
df = pd.DataFrame(data)
创建柱形图
ax = df.plot(kind='bar', x='Category', y='Values', legend=False)
添加数据标签
for p in ax.patches:
ax.annotate(str(p.get_height()), (p.get_x() * 1.005, p.get_height() * 1.005))
plt.show()
在这个示例中,我们使用Pandas的plot
方法创建了一个柱形图,然后通过annotate
方法为每个柱子添加了数据标签。
总结
为Python的柱形图添加数据标签有多种方法,包括使用Matplotlib的text
和annotate
方法,使用高级绘图库如Seaborn,以及使用数据处理库如Pandas。每种方法都有其优点和适用场景,选择合适的方法可以使我们的图表更加清晰和易于理解。
相关问答FAQs:
如何在Python的柱形图上显示数据标签?
在使用Python绘制柱形图时,可以通过在绘制柱形图时添加数据标签来展示每个柱子的数值。通常使用Matplotlib库,可以使用ax.text()
方法在每个柱子的顶部显示相应的数值。例如,首先绘制柱形图,然后在每个柱子上添加文本标签,具体的代码示例如下:
import matplotlib.pyplot as plt
x = ['A', 'B', 'C']
y = [3, 7, 5]
plt.bar(x, y)
for i in range(len(y)):
plt.text(i, y[i] + 0.1, str(y[i]), ha='center')
plt.show()
使用Seaborn库时,如何在柱形图上添加数据标签?
如果你使用Seaborn库来绘制柱形图,可以通过结合Matplotlib来实现数据标签的添加。Seaborn的barplot
函数可以用来绘制柱形图,之后可以用matplotlib
的text()
方法添加标签。示例代码如下:
import seaborn as sns
import matplotlib.pyplot as plt
data = {'Category': ['A', 'B', 'C'], 'Values': [3, 7, 5]}
sns_bar = sns.barplot(x='Category', y='Values', data=data)
for p in sns_bar.patches:
sns_bar.annotate(f'{p.get_height()}',
(p.get_x() + p.get_width() / 2., p.get_height()),
ha='center', va='bottom')
plt.show()
为什么在柱形图上添加数据标签是重要的?
在柱形图上添加数据标签可以提高图表的可读性,让观众更容易理解每个柱子所代表的具体数值。这对于展示数据时尤为重要,尤其是在需要快速传达信息的场合。此外,数据标签可以帮助用户在没有额外解释的情况下,直接获取关键信息,从而增强数据的表达效果。
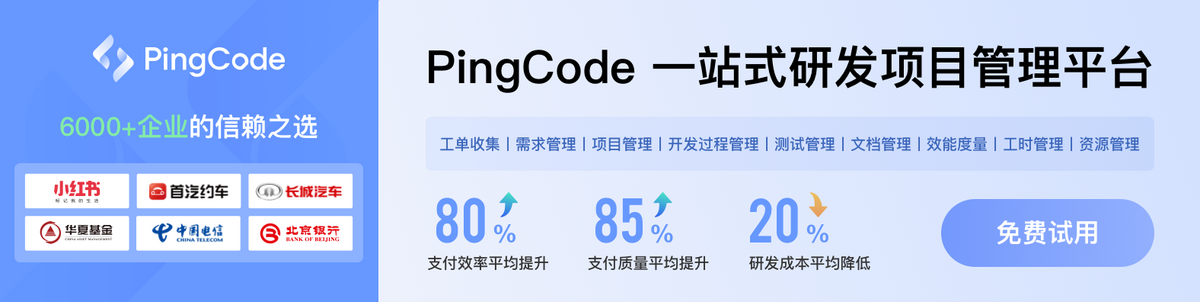