在Python3中获取MySQL数据库中的表,可以使用MySQL连接库,如mysql-connector-python
、PyMySQL
和SQLAlchemy
等。首先,您需要安装相应的库,然后通过编写Python代码来连接到MySQL数据库并获取表信息。以下是详细步骤:安装库、建立连接、执行查询、处理结果。
安装mysql-connector-python
库
在开始之前,您需要确保已经安装了mysql-connector-python
库。可以使用以下命令安装:
pip install mysql-connector-python
建立连接
接下来,您需要编写代码来连接到MySQL数据库。以下是一个示例:
import mysql.connector
建立连接
cnx = mysql.connector.connect(
user='your_username',
password='your_password',
host='your_host',
database='your_database'
)
创建游标
cursor = cnx.cursor()
在上面的代码中,your_username
、your_password
、your_host
和your_database
需要替换为您的MySQL数据库的实际连接信息。
执行查询
连接成功后,您可以执行查询以获取数据库中的表信息。以下是一个示例查询:
# 执行查询
cursor.execute("SHOW TABLES")
获取查询结果
tables = cursor.fetchall()
输出表名
for table in tables:
print(table[0])
处理结果
在上面的代码中,我们使用SHOW TABLES
查询来获取数据库中的所有表名,并使用fetchall()
方法来获取查询结果。然后,我们遍历结果并输出每个表名。
关闭连接
完成后,别忘了关闭连接和游标:
# 关闭游标和连接
cursor.close()
cnx.close()
以下是完整的代码示例:
import mysql.connector
建立连接
cnx = mysql.connector.connect(
user='your_username',
password='your_password',
host='your_host',
database='your_database'
)
创建游标
cursor = cnx.cursor()
执行查询
cursor.execute("SHOW TABLES")
获取查询结果
tables = cursor.fetchall()
输出表名
for table in tables:
print(table[0])
关闭游标和连接
cursor.close()
cnx.close()
通过上述步骤,您可以成功获取MySQL数据库中的表信息。接下来,我们将详细介绍如何使用不同的库来实现相同的功能。
一、使用mysql-connector-python
库
mysql-connector-python
是MySQL官方提供的Python连接器,支持MySQL的所有功能,并且易于使用。以下是详细步骤:
安装库
首先,确保已经安装了mysql-connector-python
库:
pip install mysql-connector-python
建立连接
建立连接的代码如下:
import mysql.connector
建立连接
cnx = mysql.connector.connect(
user='your_username',
password='your_password',
host='your_host',
database='your_database'
)
创建游标
cursor = cnx.cursor()
请确保替换your_username
、your_password
、your_host
和your_database
为实际的连接信息。
执行查询
使用SHOW TABLES
查询来获取数据库中的表信息:
# 执行查询
cursor.execute("SHOW TABLES")
获取查询结果
tables = cursor.fetchall()
输出表名
for table in tables:
print(table[0])
关闭连接
完成后,关闭游标和连接:
# 关闭游标和连接
cursor.close()
cnx.close()
二、使用PyMySQL
库
PyMySQL
是一个纯Python实现的MySQL客户端库,支持MySQL协议。以下是使用PyMySQL
获取表信息的详细步骤:
安装库
首先,确保已经安装了PyMySQL
库:
pip install PyMySQL
建立连接
建立连接的代码如下:
import pymysql
建立连接
connection = pymysql.connect(
host='your_host',
user='your_username',
password='your_password',
database='your_database'
)
创建游标
cursor = connection.cursor()
请确保替换your_username
、your_password
、your_host
和your_database
为实际的连接信息。
执行查询
使用SHOW TABLES
查询来获取数据库中的表信息:
# 执行查询
cursor.execute("SHOW TABLES")
获取查询结果
tables = cursor.fetchall()
输出表名
for table in tables:
print(table[0])
关闭连接
完成后,关闭游标和连接:
# 关闭游标和连接
cursor.close()
connection.close()
三、使用SQLAlchemy
库
SQLAlchemy
是一个功能强大的ORM框架,支持多种数据库,包括MySQL。以下是使用SQLAlchemy
获取表信息的详细步骤:
安装库
首先,确保已经安装了SQLAlchemy
和PyMySQL
库:
pip install SQLAlchemy PyMySQL
建立连接
建立连接的代码如下:
from sqlalchemy import create_engine
创建引擎
engine = create_engine('mysql+pymysql://your_username:your_password@your_host/your_database')
连接到数据库
connection = engine.connect()
请确保替换your_username
、your_password
、your_host
和your_database
为实际的连接信息。
执行查询
使用SHOW TABLES
查询来获取数据库中的表信息:
# 执行查询
result = connection.execute("SHOW TABLES")
获取查询结果
tables = result.fetchall()
输出表名
for table in tables:
print(table[0])
关闭连接
完成后,关闭连接:
# 关闭连接
connection.close()
四、处理常见错误
在连接和查询过程中,您可能会遇到一些常见错误。以下是一些常见错误及其处理方法:
连接错误
如果无法连接到数据库,请确保以下几点:
- 数据库服务器正在运行,并且可以通过网络访问。
- 用户名、密码、主机名和数据库名称是正确的。
- 防火墙未阻止连接。
权限错误
如果遇到权限错误,请确保连接的用户具有访问数据库和执行查询的权限。
查询错误
如果查询语法错误,请检查查询语句的正确性。使用SHOW TABLES
查询时,确保数据库名称是正确的。
五、优化性能
在处理大量表信息时,性能可能会成为问题。以下是一些优化性能的方法:
使用批量查询
如果需要获取多个数据库的表信息,可以使用批量查询来减少连接开销。
使用连接池
使用连接池可以提高连接效率,减少连接建立和关闭的开销。以下是一个使用SQLAlchemy
连接池的示例:
from sqlalchemy import create_engine
from sqlalchemy.orm import sessionmaker
创建引擎和连接池
engine = create_engine('mysql+pymysql://your_username:your_password@your_host/your_database', pool_size=10, max_overflow=20)
创建会话
Session = sessionmaker(bind=engine)
session = Session()
执行查询
result = session.execute("SHOW TABLES")
获取查询结果
tables = result.fetchall()
输出表名
for table in tables:
print(table[0])
关闭会话
session.close()
通过以上步骤,您可以在Python3中轻松获取MySQL数据库中的表信息。根据您的需求选择合适的库,并根据实际情况进行优化,以提高性能和可靠性。
相关问答FAQs:
如何使用Python3连接MySQL数据库以获取表信息?
要连接MySQL数据库并获取表信息,您需要安装mysql-connector-python
库。可以通过运行pip install mysql-connector-python
进行安装。连接数据库后,使用游标对象执行SHOW TABLES;
查询以获取数据库中的所有表名。以下是一个简单的示例代码:
import mysql.connector
# 连接到MySQL数据库
conn = mysql.connector.connect(
host='localhost',
user='your_username',
password='your_password',
database='your_database'
)
cursor = conn.cursor()
cursor.execute("SHOW TABLES;")
tables = cursor.fetchall()
for table in tables:
print(table)
cursor.close()
conn.close()
在Python3中如何处理MySQL表的异常情况?
处理MySQL表的异常情况是确保程序稳定性的重要环节。可以使用try-except语句来捕获连接和查询中的错误。例如,在连接数据库时,如果提供的凭据不正确或数据库不存在,程序会引发异常。通过捕获这些异常,可以提供用户友好的错误消息并安全地关闭连接。
try:
conn = mysql.connector.connect(...)
cursor = conn.cursor()
cursor.execute("SHOW TABLES;")
except mysql.connector.Error as err:
print(f"Error: {err}")
finally:
if conn:
cursor.close()
conn.close()
如何使用ORM框架在Python中获取MySQL表?
使用ORM(对象关系映射)框架,如SQLAlchemy,可以简化与数据库的交互。首先,安装SQLAlchemy库:pip install SQLAlchemy
。接下来,创建数据库引擎,并使用Table
类获取表的信息。示例如下:
from sqlalchemy import create_engine, MetaData
engine = create_engine('mysql+mysqlconnector://username:password@localhost/database')
metadata = MetaData()
metadata.reflect(bind=engine)
for table in metadata.tables:
print(table)
通过这些方法,您可以轻松地在Python3中获取MySQL数据库中的表信息。
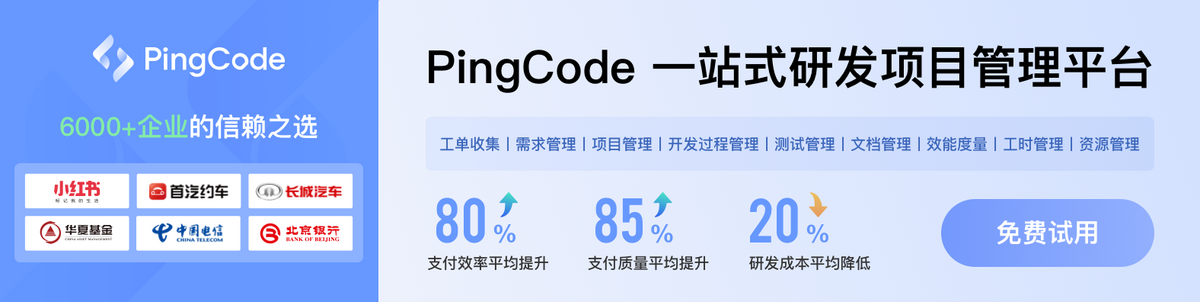