要获取字符串在另一个字符串中的位置,可以使用Python提供的多种方法,包括find()方法、index()方法和正则表达式。
1. 使用find()方法
Python中的find()方法用于在字符串中查找子字符串,并返回子字符串在字符串中第一次出现的位置。如果找不到子字符串,则返回-1。
text = "Hello, welcome to the world of Python."
position = text.find("Python")
print(position) # 输出: 29
2. 使用index()方法
index()方法与find()方法类似,不同之处在于如果找不到子字符串,index()方法会引发ValueError异常。
try:
text = "Hello, welcome to the world of Python."
position = text.index("Python")
print(position) # 输出: 29
except ValueError:
print("子字符串未找到")
3. 使用正则表达式
正则表达式提供了更灵活和强大的字符串查找功能。Python的re模块可以用来实现复杂的字符串查找。
import re
text = "Hello, welcome to the world of Python."
match = re.search("Python", text)
if match:
print(match.start()) # 输出: 29
else:
print("子字符串未找到")
展开详细描述:
find()方法的详细介绍
find()方法是一个简单且高效的字符串查找方法。该方法的语法如下:
str.find(sub[, start[, end]])
- sub: 要查找的子字符串。
- start: 查找的起始位置(可选)。
- end: 查找的结束位置(可选)。
find()方法默认从字符串的开头开始查找,可以通过指定start和end参数来限制查找范围。例如:
text = "Hello, welcome to the world of Python."
position = text.find("Python", 10, 35)
print(position) # 输出: 29
在这个例子中,find()方法从位置10到35之间查找"Python"。
index()方法的详细介绍
index()方法与find()方法类似,但在找不到子字符串时会引发异常。其语法如下:
str.index(sub[, start[, end]])
- sub: 要查找的子字符串。
- start: 查找的起始位置(可选)。
- end: 查找的结束位置(可选)。
通过index()方法,可以在找不到子字符串时捕获并处理异常。例如:
try:
text = "Hello, welcome to the world of Python."
position = text.index("Java")
print(position)
except ValueError:
print("子字符串未找到")
由于字符串中没有"Java",因此会输出"子字符串未找到"。
正则表达式的详细介绍
正则表达式提供了强大的字符串查找功能,可以处理复杂的查找需求。re模块的search()方法用于查找子字符串,并返回一个匹配对象。其语法如下:
re.search(pattern, string, flags=0)
- pattern: 要查找的正则表达式模式。
- string: 要查找的字符串。
- flags: 可选的标志位,用于修改正则表达式的匹配方式。
通过正则表达式,可以进行复杂的模式匹配。例如:
import re
text = "Hello, welcome to the world of Python."
pattern = r"\bPython\b"
match = re.search(pattern, text)
if match:
print("匹配开始位置:", match.start()) # 输出: 29
print("匹配结束位置:", match.end()) # 输出: 35
else:
print("子字符串未找到")
在这个例子中,\b表示单词边界,确保只匹配完整的"Python"单词。
二、使用find()方法查找所有出现位置
find()方法只能返回第一个匹配的位置,要查找所有匹配的位置,可以使用循环配合find()方法。例如:
text = "Python is great. Python is dynamic. Python is popular."
sub = "Python"
start = 0
positions = []
while True:
start = text.find(sub, start)
if start == -1:
break
positions.append(start)
start += len(sub) # 移动起始位置
print("所有匹配位置:", positions) # 输出: [0, 17, 34]
通过循环和调整起始位置,可以查找子字符串在主字符串中的所有出现位置。
三、使用正则表达式查找所有出现位置
使用re模块的finditer()方法,可以更方便地查找所有匹配的位置。finditer()方法返回一个迭代器,包含所有匹配对象。例如:
import re
text = "Python is great. Python is dynamic. Python is popular."
pattern = "Python"
matches = re.finditer(pattern, text)
positions = [match.start() for match in matches]
print("所有匹配位置:", positions) # 输出: [0, 17, 34]
finditer()方法的优势在于可以同时获取匹配的起始位置和结束位置。
四、性能考虑
在处理大文本时,选择合适的方法可以提高性能。find()方法和index()方法适合简单的字符串查找,性能较高;正则表达式则适合复杂的查找需求,但性能较低。因此,在使用正则表达式时,应尽量优化正则表达式模式,避免不必要的性能开销。
五、总结
在Python中,获取字符串的位置可以使用多种方法,包括find()方法、index()方法和正则表达式。find()方法适合简单的字符串查找,index()方法适合需要处理异常的场景,正则表达式适合复杂的模式匹配需求。在实际应用中,应根据具体需求选择合适的方法,并考虑性能因素。通过了解和掌握这些方法,可以在处理字符串查找时更加得心应手。
相关问答FAQs:
如何在Python中查找子字符串的位置?
在Python中,可以使用str.find()
或str.index()
方法来查找子字符串的位置。find()
方法会返回子字符串的最低索引,如果没有找到则返回-1;而index()
方法在未找到时会抛出异常。示例代码如下:
text = "Hello, welcome to the world of Python."
position = text.find("welcome") # 返回7
有没有办法获取所有出现的子字符串位置?
是的,可以通过循环结合str.find()
方法来获取所有出现的子字符串位置。使用str.find()
时,可以指定起始位置,从而找到后续的所有索引。示例代码如下:
text = "Python is great. Python is fun."
positions = []
start = 0
while True:
start = text.find("Python", start)
if start == -1: break
positions.append(start)
start += len("Python")
在Python中,如何处理大小写敏感的字符串查找?
Python的字符串查找方法默认是大小写敏感的。如果希望进行不区分大小写的查找,可以先将字符串转换为统一的大小写。使用str.lower()
或str.upper()
可以实现。以下是相关代码示例:
text = "Python is awesome. python is powerful."
position = text.lower().find("python") # 返回0
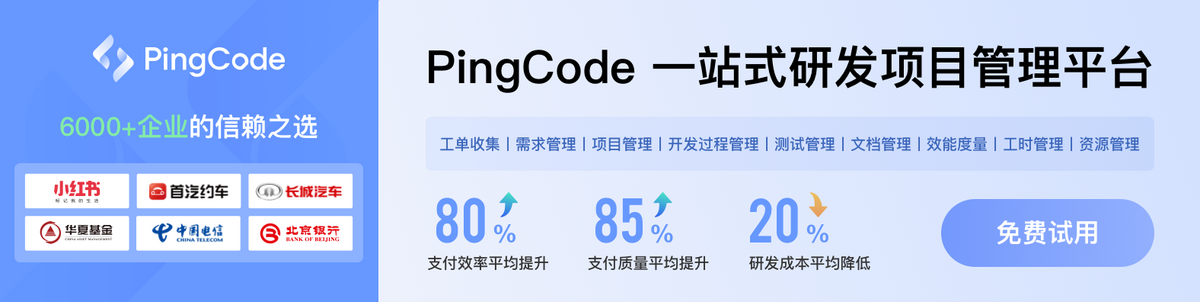