在 Python 中,可以通过多种方式判断列表中的数字大小。使用内置函数、通过循环迭代、使用列表推导式是常见的方法。使用内置函数最为便捷,通过循环迭代可以进行更复杂的判断,而列表推导式则适合于简单的条件过滤。下面详细介绍这几种方法。
一、使用内置函数
1、max() 和 min() 函数
Python 提供了 max()
和 min()
函数,可以分别用来获取列表中的最大值和最小值。这是最简单、最直接的方法。
numbers = [3, 5, 1, 8, 4]
max_value = max(numbers)
min_value = min(numbers)
print(f"The maximum value is: {max_value}")
print(f"The minimum value is: {min_value}")
2、sorted() 函数
sorted()
函数可以对列表进行排序,默认升序排列。通过排序后的列表可以方便地获取最大值和最小值。
numbers = [3, 5, 1, 8, 4]
sorted_numbers = sorted(numbers)
print(f"The sorted list is: {sorted_numbers}")
print(f"The maximum value is: {sorted_numbers[-1]}")
print(f"The minimum value is: {sorted_numbers[0]}")
二、通过循环迭代
使用循环迭代可以实现更复杂的判断逻辑,例如找出列表中所有大于某个值的元素,或者比较多个列表中的元素。
1、查找特定条件下的最大值和最小值
numbers = [3, 5, 1, 8, 4]
threshold = 4
max_value = float('-inf')
min_value = float('inf')
for number in numbers:
if number > threshold:
if number > max_value:
max_value = number
if number < min_value:
min_value = number
print(f"The maximum value greater than {threshold} is: {max_value}")
print(f"The minimum value greater than {threshold} is: {min_value}")
2、比较多个列表中的元素
list1 = [3, 5, 1, 8, 4]
list2 = [7, 2, 9, 6, 0]
max_value = float('-inf')
for number in list1 + list2:
if number > max_value:
max_value = number
print(f"The maximum value in both lists is: {max_value}")
三、使用列表推导式
列表推导式是一种简洁的语法,可以用来创建新列表。它适合于简单的条件过滤和映射操作。
1、过滤出大于某个值的元素
numbers = [3, 5, 1, 8, 4]
threshold = 4
filtered_numbers = [number for number in numbers if number > threshold]
print(f"Numbers greater than {threshold} are: {filtered_numbers}")
2、获取满足条件的最大值和最小值
numbers = [3, 5, 1, 8, 4]
threshold = 4
filtered_numbers = [number for number in numbers if number > threshold]
if filtered_numbers:
max_value = max(filtered_numbers)
min_value = min(filtered_numbers)
print(f"The maximum value greater than {threshold} is: {max_value}")
print(f"The minimum value greater than {threshold} is: {min_value}")
else:
print(f"No numbers greater than {threshold}")
四、综合运用
在实际应用中,往往需要综合运用上述方法来实现复杂的判断逻辑。例如,统计满足某个条件的元素个数,或者对多个列表进行并集、交集操作。
1、统计满足条件的元素个数
numbers = [3, 5, 1, 8, 4]
threshold = 4
count = sum(1 for number in numbers if number > threshold)
print(f"Count of numbers greater than {threshold} is: {count}")
2、列表并集和交集操作
list1 = [3, 5, 1, 8, 4]
list2 = [7, 2, 9, 6, 0]
union = list(set(list1) | set(list2))
intersection = list(set(list1) & set(list2))
print(f"Union of the two lists is: {union}")
print(f"Intersection of the two lists is: {intersection}")
五、使用第三方库
对于更复杂的数据操作,可以使用 Pandas 等第三方库。Pandas 提供了强大的数据处理能力,特别适合处理大型数据集和复杂的数据分析任务。
1、使用 Pandas 进行数据分析
import pandas as pd
numbers = [3, 5, 1, 8, 4]
df = pd.DataFrame(numbers, columns=["Numbers"])
max_value = df["Numbers"].max()
min_value = df["Numbers"].min()
print(f"The maximum value is: {max_value}")
print(f"The minimum value is: {min_value}")
2、使用 NumPy 进行数值计算
NumPy 是另一个常用的库,特别适合进行数值计算和数组操作。
import numpy as np
numbers = [3, 5, 1, 8, 4]
np_numbers = np.array(numbers)
max_value = np_numbers.max()
min_value = np_numbers.min()
print(f"The maximum value is: {max_value}")
print(f"The minimum value is: {min_value}")
六、实例应用
1、找出列表中第二大的数
numbers = [3, 5, 1, 8, 4]
unique_numbers = list(set(numbers))
unique_numbers.sort()
if len(unique_numbers) > 1:
second_max = unique_numbers[-2]
else:
second_max = unique_numbers[0]
print(f"The second maximum value is: {second_max}")
2、找出列表中出现次数最多的数
from collections import Counter
numbers = [3, 5, 1, 8, 4, 3, 8, 8, 5]
number_counts = Counter(numbers)
most_common_number, most_common_count = number_counts.most_common(1)[0]
print(f"The most common number is: {most_common_number} with {most_common_count} occurrences")
综上所述,Python 提供了多种方法来判断列表中的数字大小,包括使用内置函数、循环迭代、列表推导式,以及第三方库如 Pandas 和 NumPy。根据具体需求选择合适的方法,可以大大提高代码的效率和可读性。
相关问答FAQs:
如何在Python中找到列表中的最大和最小数字?
在Python中,可以使用内置的max()
和min()
函数来快速找到列表中的最大值和最小值。例如,假设有一个列表numbers = [3, 5, 1, 9, 2]
,可以通过max(numbers)
来获得最大值9,通过min(numbers)
来获得最小值1。这些方法简单易用,适用于任意长度的列表。
在Python中如何比较两个列表中的数字大小?
如果需要比较两个列表中数字的大小,可以使用列表解析和内置的any()
或all()
函数。例如,可以通过列表解析生成一个布尔列表,表示一个列表中的每个数字是否大于另一个列表的对应数字。使用any()
可以检查是否有任意一个数字满足条件,而all()
则用于确认所有数字都满足条件。
如何判断Python列表中是否所有数字都大于某个特定值?
可以使用all()
函数配合列表解析来判断列表中的所有数字是否都大于某个特定值。例如,给定一个列表numbers = [4, 5, 6]
,要检查是否所有数字都大于3,可以使用all(num > 3 for num in numbers)
。如果返回值为True,则表明列表中的所有数字都大于3,否则至少有一个数字不满足条件。
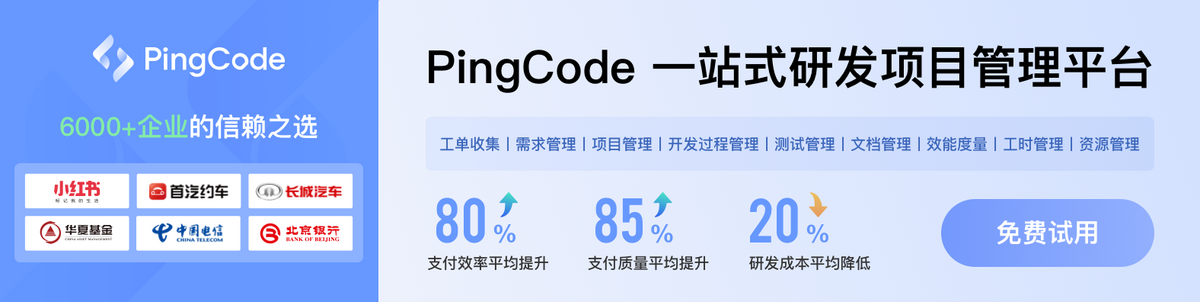