Python打开一个json文件的方法有几种,包括使用内置的json
模块、第三方库如pandas
和simplejson
等。最常用且推荐的方法是使用内置的json
模块,因为它简单、直接且符合大多数需求。以下详细介绍如何使用json模块来打开和处理json文件。
要使用Python打开一个json文件,首先需要导入json模块。接下来,通过文件操作函数打开文件,然后使用json.load()或json.loads()方法将json文件内容解析为Python对象。以下是具体步骤和示例代码。
一、使用Python的json模块
Python内置的json模块提供了读取和写入json数据的功能。以下是使用json模块打开json文件的步骤:
import json
打开并读取json文件
with open('data.json', 'r') as file:
data = json.load(file)
print(data)
在这个例子中,open
函数以读取模式('r')打开json文件,然后使用json.load()
方法将其内容解析为Python对象(通常是字典或列表)。
二、使用pandas读取json文件
pandas库也提供了读取json文件的功能,特别适合处理较大的数据集。以下是使用pandas读取json文件的步骤:
import pandas as pd
读取json文件
data = pd.read_json('data.json')
print(data)
read_json
方法可以直接将json文件内容读取为DataFrame,这在处理数据分析任务时非常方便。
三、使用simplejson库
simplejson是json模块的第三方扩展版,提供了更强大的功能和更好的性能。以下是使用simplejson读取json文件的步骤:
import simplejson as json
打开并读取json文件
with open('data.json', 'r') as file:
data = json.load(file)
print(data)
四、json模块的详细介绍
json模块不仅能够读取json文件,还能够将Python对象转换为json格式并写入文件。以下是一些详细的用法和注意事项。
1、读取json数据
使用json.load()
方法可以将打开的文件对象转换为Python对象:
import json
打开并读取json文件
with open('data.json', 'r') as file:
data = json.load(file)
data现在是一个Python字典或列表
print(data)
如果json数据是字符串形式,可以使用json.loads()
方法:
json_string = '{"name": "John", "age": 30, "city": "New York"}'
data = json.loads(json_string)
print(data)
2、写入json数据
使用json.dump()
方法可以将Python对象转换为json格式并写入文件:
import json
data = {
"name": "John",
"age": 30,
"city": "New York"
}
将Python对象写入json文件
with open('data.json', 'w') as file:
json.dump(data, file)
如果需要将Python对象转换为json字符串,可以使用json.dumps()
方法:
json_string = json.dumps(data)
print(json_string)
3、json模块的选项
json模块提供了多个选项用于控制编码和解码过程。以下是一些常用选项:
indent
:指定缩进级别,使输出的json数据更具可读性。separators
:指定分隔符,通常用于定制json数据的格式。sort_keys
:指定是否对字典的键进行排序。
示例如下:
import json
data = {
"name": "John",
"age": 30,
"city": "New York"
}
将Python对象写入json文件并设置缩进级别
with open('data.json', 'w') as file:
json.dump(data, file, indent=4, sort_keys=True)
五、处理错误
在处理json文件时,可能会遇到各种错误,例如文件不存在、json格式错误等。以下是一些常见错误及其处理方法:
1、文件不存在
try:
with open('data.json', 'r') as file:
data = json.load(file)
except FileNotFoundError:
print("文件未找到")
2、json格式错误
json_string = '{"name": "John", "age": 30, "city": "New York"'
try:
data = json.loads(json_string)
except json.JSONDecodeError as e:
print(f"JSON格式错误: {e}")
六、实际应用示例
以下是一个综合示例,展示了从json文件读取数据、处理数据并将结果写入另一个json文件的完整流程:
import json
读取json文件
try:
with open('input.json', 'r') as file:
data = json.load(file)
except FileNotFoundError:
print("文件未找到")
data = {}
处理数据
if data:
data['age'] = 31 # 假设要更新年龄
data['city'] = "San Francisco" # 假设要更新城市
将处理后的数据写入新的json文件
with open('output.json', 'w') as file:
json.dump(data, file, indent=4)
这个示例展示了从文件读取数据、处理数据并将结果写入文件的完整流程,是实际应用中常见的操作。
七、总结
使用Python处理json文件非常方便,无论是内置的json模块,还是第三方库如pandas和simplejson,都提供了丰富的功能来满足不同需求。无论是简单的数据读取和写入,还是复杂的数据处理和错误处理,都可以通过合理使用这些工具来高效完成。
在实际应用中,根据具体需求选择合适的方法和库,并注意处理可能出现的错误,可以确保程序的健壮性和可靠性。
相关问答FAQs:
如何在Python中读取json文件的内容?
在Python中,可以使用内置的json
模块来读取json文件。首先,您需要打开文件,使用json.load()
方法将文件内容解析为Python对象。示例代码如下:
import json
with open('file.json', 'r') as file:
data = json.load(file)
print(data)
这个方法可以轻松地将json格式的数据转换为Python的字典或列表。
在处理大规模json文件时,应该注意哪些问题?
处理大规模的json文件时,内存消耗可能会成为一个问题。建议使用json.load()
时,配合open()
的buffering
参数,或者使用ijson
库,它可以逐行读取json数据,降低内存使用。例如:
import ijson
with open('large_file.json', 'r') as file:
for item in ijson.items(file, 'item'):
print(item)
这样可以有效地处理大文件,避免一次性加载全部数据。
如果json文件格式不正确,Python会如何处理?
如果json文件格式不正确,调用json.load()
时将抛出json.JSONDecodeError
异常。这意味着文件中存在语法错误,比如缺失的逗号、引号不匹配等。为了避免程序崩溃,可以使用try
和except
语句来捕获异常并进行相应处理:
import json
try:
with open('file.json', 'r') as file:
data = json.load(file)
except json.JSONDecodeError as e:
print(f"JSON解码错误: {e}")
通过这种方式,可以安全地处理文件读取错误。
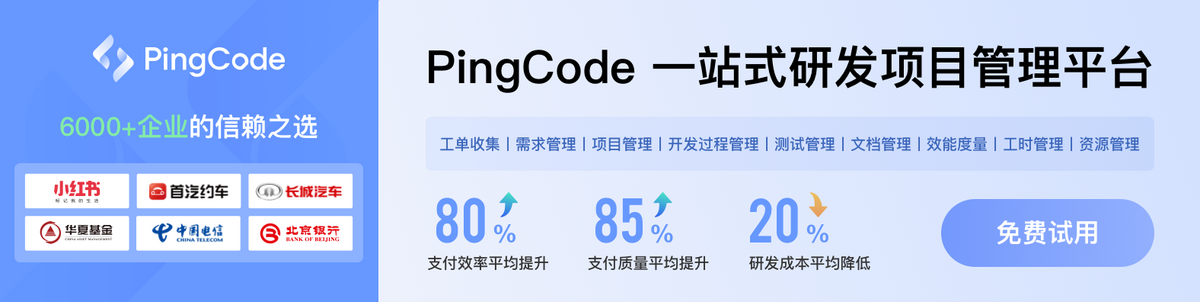