如何用Python让浏览器发通知
在现代浏览器中,发出通知是一种非常有效的方式来引起用户的注意。用Python实现这一功能并不复杂,主要通过结合JavaScript和相关的Web技术来完成。使用Flask、WebSocket、Service Workers等技术手段可以帮助实现这一目标。下面,我们将详细解释如何通过这些手段实现这一功能。
一、使用Flask构建简单的Web服务器
Flask是一个轻量级的Python Web框架,它可以帮助我们快速构建Web应用。在这个部分,我们将使用Flask来创建一个简单的Web服务器,并在服务器端处理浏览器通知的请求。
安装Flask
首先,我们需要安装Flask。可以通过pip来安装:
pip install flask
创建Flask应用
创建一个新的Python文件,如app.py
,并添加以下代码:
from flask import Flask, render_template
app = Flask(__name__)
@app.route('/')
def index():
return render_template('index.html')
if __name__ == '__main__':
app.run(debug=True)
这个简单的Flask应用将会在访问根路径(/
)时返回一个HTML模板。
创建HTML模板
在你的项目目录中创建一个名为templates
的文件夹,并在其中创建index.html
文件。添加以下HTML代码:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Browser Notification</title>
</head>
<body>
<h1>Browser Notification with Python</h1>
<button id="notify-btn">Send Notification</button>
<script>
document.getElementById('notify-btn').addEventListener('click', () => {
if (Notification.permission === 'granted') {
new Notification('Hello from Python!');
} else if (Notification.permission !== 'denied') {
Notification.requestPermission().then(permission => {
if (permission === 'granted') {
new Notification('Hello from Python!');
}
});
}
});
</script>
</body>
</html>
这个简单的HTML页面包含一个按钮,点击该按钮时将会检查浏览器的通知权限,并在权限被授予时发送一条通知。
二、使用WebSocket实现实时通知
为了实现实时通知,我们需要引入WebSocket。WebSocket是一种通信协议,可以在客户端和服务器之间实现全双工通信。我们可以使用flask-socketio
来实现。
安装flask-socketio
可以通过pip来安装:
pip install flask-socketio
修改Flask应用以支持WebSocket
更新app.py
文件以支持WebSocket:
from flask import Flask, render_template
from flask_socketio import SocketIO, emit
app = Flask(__name__)
socketio = SocketIO(app)
@app.route('/')
def index():
return render_template('index.html')
@socketio.on('connect')
def handle_connect():
print('Client connected')
@socketio.on('disconnect')
def handle_disconnect():
print('Client disconnected')
@socketio.on('send_notification')
def handle_notification(data):
emit('receive_notification', data, broadcast=True)
if __name__ == '__main__':
socketio.run(app, debug=True)
更新HTML模板以支持WebSocket
修改index.html
文件以支持WebSocket:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Browser Notification</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/socket.io/4.0.0/socket.io.js"></script>
</head>
<body>
<h1>Browser Notification with Python</h1>
<button id="notify-btn">Send Notification</button>
<script>
const socket = io();
document.getElementById('notify-btn').addEventListener('click', () => {
socket.emit('send_notification', { message: 'Hello from Python!' });
});
socket.on('receive_notification', data => {
if (Notification.permission === 'granted') {
new Notification(data.message);
} else if (Notification.permission !== 'denied') {
Notification.requestPermission().then(permission => {
if (permission === 'granted') {
new Notification(data.message);
}
});
}
});
</script>
</body>
</html>
通过这种方式,我们就可以在服务器端发送通知到所有已连接的客户端。
三、使用Service Workers实现持久化通知
Service Workers 是一种运行在浏览器后台的脚本,能够处理推送通知等任务。它们可以让Web应用在没有打开的情况下接收并显示通知。
注册Service Worker
首先,我们需要在index.html
中注册Service Worker:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Browser Notification</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/socket.io/4.0.0/socket.io.js"></script>
</head>
<body>
<h1>Browser Notification with Python</h1>
<button id="notify-btn">Send Notification</button>
<script>
if ('serviceWorker' in navigator) {
navigator.serviceWorker.register('/service-worker.js').then(registration => {
console.log('Service Worker registered with scope:', registration.scope);
}).catch(error => {
console.error('Service Worker registration failed:', error);
});
}
const socket = io();
document.getElementById('notify-btn').addEventListener('click', () => {
socket.emit('send_notification', { message: 'Hello from Python!' });
});
socket.on('receive_notification', data => {
if (Notification.permission === 'granted') {
new Notification(data.message);
} else if (Notification.permission !== 'denied') {
Notification.requestPermission().then(permission => {
if (permission === 'granted') {
new Notification(data.message);
}
});
}
});
</script>
</body>
</html>
创建Service Worker文件
在项目根目录下创建service-worker.js
文件,并添加以下代码:
self.addEventListener('push', event => {
const data = event.data.json();
self.registration.showNotification(data.title, {
body: data.body,
icon: 'icon.png'
});
});
修改Flask应用以支持Service Worker
更新app.py
文件,增加Service Worker的路由:
from flask import Flask, render_template, send_from_directory
from flask_socketio import SocketIO, emit
app = Flask(__name__)
socketio = SocketIO(app)
@app.route('/')
def index():
return render_template('index.html')
@app.route('/service-worker.js')
def service_worker():
return send_from_directory('static', 'service-worker.js')
@socketio.on('connect')
def handle_connect():
print('Client connected')
@socketio.on('disconnect')
def handle_disconnect():
print('Client disconnected')
@socketio.on('send_notification')
def handle_notification(data):
emit('receive_notification', data, broadcast=True)
if __name__ == '__main__':
socketio.run(app, debug=True)
发送推送通知
为了发送推送通知,我们需要一个推送服务。可以使用第三方服务如Firebase Cloud Messaging (FCM)或自建推送服务。以下是使用FCM的示例:
- 注册FCM并获取服务器密钥和发送者ID。
- 将这些信息集成到你的Flask应用中,发送推送通知。
四、总结
通过上述步骤,我们使用Python结合Flask、WebSocket和Service Workers实现了浏览器通知功能。Flask帮助我们构建了一个简单的Web服务器,WebSocket实现了实时通知的功能,Service Workers则使得我们的通知能够在浏览器后台运行时显示。
这种方法不仅能有效提高用户体验,还能确保用户在浏览器后台或关闭时仍能接收到重要通知。通过不断优化和扩展这些技术,我们可以实现更加复杂和强大的通知系统,为用户提供更好的服务。
相关问答FAQs:
如何使用Python与浏览器进行通知交互?
使用Python与浏览器进行通知交互通常需要结合一些前端技术,如JavaScript和Web APIs。可以通过Flask或Django等框架创建后端服务,使用JavaScript来处理浏览器的通知权限请求,并通过WebSocket实现实时更新,从而实现通知功能。
在Python中实现浏览器通知的步骤是什么?
实现浏览器通知通常需要以下几个步骤:首先,设置一个后端服务,使用Flask或Django等框架来处理HTTP请求。接着,在前端页面中使用JavaScript请求用户的通知权限。最后,通过后端发送数据到前端,使用JavaScript的Notification API来显示通知。
使用Python发送浏览器通知是否需要特定的库或工具?
虽然Python本身没有直接发送浏览器通知的库,但可以使用一些框架和库来实现这一功能。例如,Flask-SocketIO可以用于实现实时通信,而使用JavaScript的Notification API则可以处理实际的通知显示。这种结合可以让Python脚本与浏览器通知功能无缝对接。
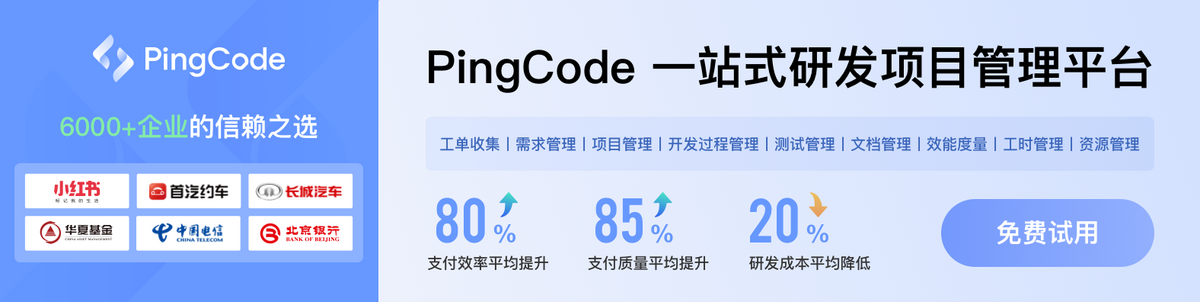