Python向字符串添加元素的多种方法包括:使用字符串连接操作符、使用格式化方法、使用f-strings、使用join方法。其中,使用格式化方法是最常见和灵活的方式之一。
使用字符串格式化方法可以提供一种更直观和灵活的方式来插入变量或添加元素到字符串中。Python提供了多种字符串格式化方法,包括百分号(%)格式化、str.format()方法和f-strings(在Python 3.6及以上版本中)。下面将详细介绍这些方法,并给出具体示例和应用场景。
一、使用字符串连接操作符
字符串连接操作符(+)是最简单的方式之一,可以将两个或多个字符串连接在一起。尽管这种方法直观,但在处理大量数据时可能效率较低。
str1 = "Hello"
str2 = "World"
result = str1 + " " + str2
print(result) # 输出: Hello World
字符串连接操作符的优势在于其简单性和直观性,但在需要进行复杂字符串操作时,其局限性也十分明显。
二、使用百分号(%)格式化
百分号(%)格式化是Python中较为传统的字符串格式化方法,类似于C语言中的printf。
name = "John"
age = 25
result = "My name is %s and I am %d years old." % (name, age)
print(result) # 输出: My name is John and I am 25 years old.
这种方法使用占位符(如%s、%d)来表示字符串中的变量位置,并在字符串末尾通过元组传入实际变量值。尽管这种方法较为古老,但仍然在一些旧代码中较为常见。
三、使用str.format()方法
str.format()方法是Python 2.7及以上版本中引入的一种更强大和灵活的字符串格式化方法。
name = "Jane"
age = 28
result = "My name is {} and I am {} years old.".format(name, age)
print(result) # 输出: My name is Jane and I am 28 years old.
str.format()方法支持通过位置参数和关键字参数来插入变量,并且可以通过索引和字段名来进行更复杂的格式化操作。
result = "My name is {0} and I am {1} years old. I love {2}".format("Alice", 30, "coding")
print(result) # 输出: My name is Alice and I am 30 years old. I love coding
此外,str.format()还支持对变量进行格式化控制,如指定宽度、对齐方式、精度等。
pi = 3.14159265
result = "The value of Pi is approximately {:.2f}".format(pi)
print(result) # 输出: The value of Pi is approximately 3.14
四、使用f-strings
f-strings(格式化字符串字面量)是在Python 3.6及以上版本中引入的一种新型字符串格式化方法,以其简洁和高效受到了广泛欢迎。
name = "Tom"
age = 35
result = f"My name is {name} and I am {age} years old."
print(result) # 输出: My name is Tom and I am 35 years old.
f-strings通过在字符串前加上字母“f”或“F”,并在字符串内部使用大括号{}来嵌入变量或表达式,提供了一种更为简洁的方式。
a = 5
b = 10
result = f"The sum of {a} and {b} is {a + b}."
print(result) # 输出: The sum of 5 and 10 is 15.
f-strings不仅支持变量插入,还可以直接在大括号内进行表达式计算和调用函数,极大地提高了字符串操作的灵活性。
五、使用join方法
join方法主要用于将一个可迭代对象(如列表、元组等)的元素连接成一个字符串。尽管其主要用途并非直接向字符串添加元素,但在一些场景下仍然非常有用。
words = ["Hello", "from", "the", "other", "side"]
result = " ".join(words)
print(result) # 输出: Hello from the other side
join方法的优势在于其高效性,尤其是在需要将大量小字符串连接成一个大字符串时,比字符串连接操作符更为高效。
六、使用字符串切片和插入
在某些情况下,我们可能需要在字符串的特定位置插入元素,这时可以结合字符串切片和连接操作符来实现。
original = "HelloWorld"
insert = " "
position = 5
result = original[:position] + insert + original[position:]
print(result) # 输出: Hello World
这种方法通过字符串切片将字符串分为两部分,然后在中间插入新的元素,再将其连接成一个新的字符串。
七、利用字符串模板(string.Template)
字符串模板(string.Template)是Python标准库中的一个模块,提供了一种更为安全和灵活的字符串替换方式,尤其适用于需要处理用户输入的场景。
from string import Template
template = Template("My name is $name and I am $age years old.")
result = template.substitute(name="Alice", age=30)
print(result) # 输出: My name is Alice and I am 30 years old.
字符串模板通过使用美元符号$作为占位符,并通过substitute方法进行变量替换,提供了一种更为安全的方式来处理字符串插值。
八、总结与最佳实践
在实际开发中,选择哪种方法取决于具体的应用场景和需求。以下是一些最佳实践建议:
- 简单场景:对于简单的字符串连接和插值操作,使用f-strings或str.format()方法。
- 复杂格式化:当需要进行复杂的字符串格式化时,优先选择str.format()方法。
- 高效连接:在需要处理大量字符串连接操作时,使用join方法。
- 安全性:在处理用户输入或需要额外安全性的场景中,考虑使用字符串模板(string.Template)。
通过合理选择和使用这些方法,可以大大提高字符串操作的效率和代码的可读性,从而更好地满足各种应用需求。
相关问答FAQs:
如何在Python中向字符串中插入字符?
在Python中,字符串是不可变的,这意味着一旦创建,就无法直接修改它。要向字符串中插入字符,可以使用字符串拼接、格式化或列表转换的方法。例如,可以通过+
运算符将两个字符串连接起来,或者使用str.format()
方法将字符插入到特定位置。
可以使用哪些方法来扩展Python字符串的内容?
扩展Python字符串的内容可以通过多种方式实现。常见的方法包括使用join()
函数将多个字符串连接在一起,使用列表推导式生成新字符串,或通过字符串格式化方法(如f-string
或format()
)将变量的值嵌入字符串中。
字符串拼接时需要注意哪些性能问题?
在Python中,频繁地拼接字符串可能会导致性能问题,因为每次拼接都会生成一个新的字符串。如果需要进行大量字符串拼接,考虑使用StringIO
模块或列表收集字符串,然后使用''.join()
方法进行一次性连接,这样可以提高效率。
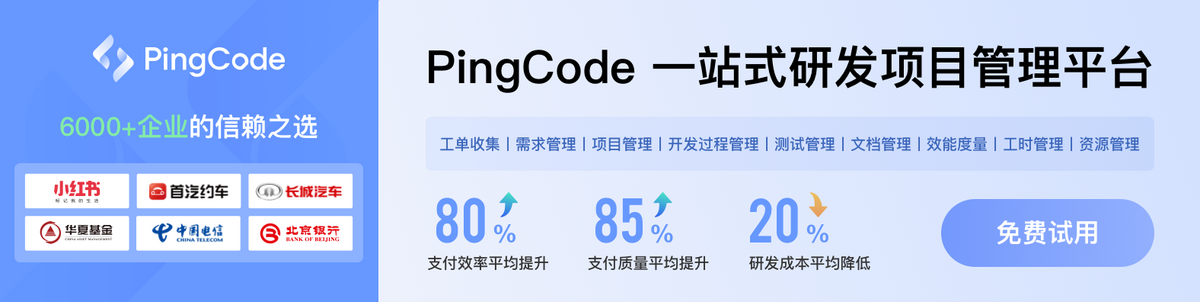