在Python中从文件中查找字符串的方法包括:使用文件操作函数读取文件、使用字符串方法查找、使用正则表达式进行匹配。 其中,使用文件操作函数读取文件和字符串方法查找是最基础和常用的方法。本文将详细介绍这些方法,并提供代码示例。
一、文件操作函数读取文件
在Python中,处理文件的操作是非常常见的任务之一。要从文件中查找字符串,首先需要打开文件并读取其内容。Python提供了多种方法来读取文件,下面将详细介绍几种常用的方法。
1.1、使用open()
函数
open()
函数是Python内置的一个函数,用来打开文件。它的基本语法如下:
open(file, mode='r', buffering=-1, encoding=None, errors=None, newline=None, closefd=True, opener=None)
file
:文件路径。mode
:打开文件的模式,常用的模式有'r'
(只读)、'w'
(写入,覆盖文件内容)等。encoding
:文件编码,默认为None
,但建议显式指定为'utf-8'
。
以下是一个示例,展示如何使用open()
函数读取文件内容并查找字符串:
def find_string_in_file(file_path, search_string):
with open(file_path, 'r', encoding='utf-8') as file:
for line_number, line in enumerate(file, start=1):
if search_string in line:
print(f"Found '{search_string}' in line {line_number}: {line.strip()}")
示例调用
find_string_in_file('example.txt', 'hello')
在这个示例中,open()
函数以只读模式打开文件,with
语句确保文件在操作完成后自动关闭。enumerate()
函数用于获取行号,if
语句检查搜索字符串是否在当前行中。
1.2、使用read()
、readline()
、readlines()
方法
Python提供了三种方法来读取文件内容:
read()
:一次性读取整个文件内容。readline()
:逐行读取文件内容。readlines()
:一次性读取文件的所有行,并返回一个列表。
以下是这三种方法的示例:
def find_string_with_read(file_path, search_string):
with open(file_path, 'r', encoding='utf-8') as file:
content = file.read()
if search_string in content:
print(f"Found '{search_string}' in the file.")
def find_string_with_readline(file_path, search_string):
with open(file_path, 'r', encoding='utf-8') as file:
line_number = 0
while True:
line = file.readline()
if not line:
break
line_number += 1
if search_string in line:
print(f"Found '{search_string}' in line {line_number}: {line.strip()}")
def find_string_with_readlines(file_path, search_string):
with open(file_path, 'r', encoding='utf-8') as file:
lines = file.readlines()
for line_number, line in enumerate(lines, start=1):
if search_string in line:
print(f"Found '{search_string}' in line {line_number}: {line.strip()}")
这些方法各有优缺点,read()
适用于小文件,readline()
和readlines()
适用于逐行处理和较大文件。
二、字符串方法查找
字符串方法查找是Python中非常常用的操作,主要方法包括find()
、index()
、startswith()
、endswith()
等。这些方法可以帮助我们在文件内容中快速找到目标字符串。
2.1、find()
方法
find()
方法返回子字符串在字符串中第一次出现的位置,如果没有找到,则返回-1
。基本语法如下:
str.find(sub, start=0, end=len(str))
以下是一个示例,展示如何使用find()
方法查找字符串:
def find_string_with_find(file_path, search_string):
with open(file_path, 'r', encoding='utf-8') as file:
content = file.read()
position = content.find(search_string)
if position != -1:
print(f"Found '{search_string}' at position {position}.")
else:
print(f"'{search_string}' not found in the file.")
2.2、index()
方法
index()
方法与find()
类似,但如果子字符串没有找到,则会抛出ValueError
异常。基本语法如下:
str.index(sub, start=0, end=len(str))
以下是一个示例,展示如何使用index()
方法查找字符串:
def find_string_with_index(file_path, search_string):
try:
with open(file_path, 'r', encoding='utf-8') as file:
content = file.read()
position = content.index(search_string)
print(f"Found '{search_string}' at position {position}.")
except ValueError:
print(f"'{search_string}' not found in the file.")
2.3、startswith()
和endswith()
方法
startswith()
和endswith()
方法分别用于检查字符串是否以指定的子字符串开头或结尾。基本语法如下:
str.startswith(prefix[, start[, end]])
str.endswith(suffix[, start[, end]])
以下是一个示例,展示如何使用startswith()
和endswith()
方法:
def find_string_with_start_end(file_path, search_string):
with open(file_path, 'r', encoding='utf-8') as file:
for line_number, line in enumerate(file, start=1):
if line.startswith(search_string):
print(f"Line {line_number} starts with '{search_string}': {line.strip()}")
if line.endswith(search_string + '\n'):
print(f"Line {line_number} ends with '{search_string}': {line.strip()}")
三、正则表达式匹配
正则表达式是一种强大的字符串匹配工具,在Python中可以使用re
模块来处理。正则表达式可以用于复杂的字符串查找和替换操作。
3.1、使用re.search()
方法
re.search()
方法用于查找字符串中是否存在匹配的子字符串。基本语法如下:
re.search(pattern, string, flags=0)
以下是一个示例,展示如何使用re.search()
方法:
import re
def find_string_with_re_search(file_path, search_pattern):
with open(file_path, 'r', encoding='utf-8') as file:
for line_number, line in enumerate(file, start=1):
if re.search(search_pattern, line):
print(f"Found pattern '{search_pattern}' in line {line_number}: {line.strip()}")
示例调用
find_string_with_re_search('example.txt', r'\bhello\b')
3.2、使用re.findall()
方法
re.findall()
方法返回字符串中所有非重叠匹配的子字符串。基本语法如下:
re.findall(pattern, string, flags=0)
以下是一个示例,展示如何使用re.findall()
方法:
import re
def find_all_matches(file_path, search_pattern):
with open(file_path, 'r', encoding='utf-8') as file:
content = file.read()
matches = re.findall(search_pattern, content)
for match in matches:
print(f"Found match: {match}")
示例调用
find_all_matches('example.txt', r'\bhello\b')
3.3、使用re.match()
和re.fullmatch()
方法
re.match()
方法从字符串的起始位置匹配,re.fullmatch()
方法要求整个字符串完全匹配。基本语法如下:
re.match(pattern, string, flags=0)
re.fullmatch(pattern, string, flags=0)
以下是一个示例,展示如何使用re.match()
和re.fullmatch()
方法:
import re
def find_string_with_re_match(file_path, search_pattern):
with open(file_path, 'r', encoding='utf-8') as file:
for line_number, line in enumerate(file, start=1):
if re.match(search_pattern, line):
print(f"Line {line_number} matches pattern '{search_pattern}': {line.strip()}")
def find_string_with_re_fullmatch(file_path, search_pattern):
with open(file_path, 'r', encoding='utf-8') as file:
for line_number, line in enumerate(file, start=1):
if re.fullmatch(search_pattern, line.strip()):
print(f"Line {line_number} fully matches pattern '{search_pattern}': {line.strip()}")
示例调用
find_string_with_re_match('example.txt', r'^hello')
find_string_with_re_fullmatch('example.txt', r'hello world')
四、结合多种方法优化查找
在实际应用中,可以结合多种方法来优化查找操作,以提高效率和灵活性。例如,可以先使用字符串方法进行初步筛选,再使用正则表达式进行精确匹配。
4.1、结合字符串方法和正则表达式
以下是一个示例,展示如何结合字符串方法和正则表达式:
import re
def optimized_find_string(file_path, search_string, search_pattern):
with open(file_path, 'r', encoding='utf-8') as file:
for line_number, line in enumerate(file, start=1):
if search_string in line:
if re.search(search_pattern, line):
print(f"Found pattern '{search_pattern}' in line {line_number}: {line.strip()}")
示例调用
optimized_find_string('example.txt', 'hello', r'\bhello\b')
4.2、使用生成器优化内存使用
对于大文件,可以使用生成器来优化内存使用。以下是一个示例,展示如何使用生成器:
def find_string_with_generator(file_path, search_string):
def file_generator(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
for line in file:
yield line
for line_number, line in enumerate(file_generator(file_path), start=1):
if search_string in line:
print(f"Found '{search_string}' in line {line_number}: {line.strip()}")
示例调用
find_string_with_generator('example.txt', 'hello')
4.3、结合多线程或多进程加速查找
对于特别大的文件或需要高效处理的场景,可以结合多线程或多进程来加速查找。以下是一个示例,展示如何使用多线程:
import threading
def find_string_in_chunk(file_path, search_string, start, end):
with open(file_path, 'r', encoding='utf-8') as file:
file.seek(start)
lines = file.read(end - start).splitlines()
for line_number, line in enumerate(lines, start=1):
if search_string in line:
print(f"Found '{search_string}' in line {line_number + start}: {line.strip()}")
def find_string_with_multithreading(file_path, search_string, num_threads=4):
file_size = os.path.getsize(file_path)
chunk_size = file_size // num_threads
threads = []
for i in range(num_threads):
start = i * chunk_size
end = start + chunk_size if i != num_threads - 1 else file_size
thread = threading.Thread(target=find_string_in_chunk, args=(file_path, search_string, start, end))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
示例调用
find_string_with_multithreading('example.txt', 'hello')
五、总结
通过本篇文章,我们详细介绍了在Python中从文件中查找字符串的多种方法,包括文件操作函数读取文件、字符串方法查找、正则表达式匹配等。并结合多种方法优化查找操作,提高效率和灵活性。希望这些方法和示例代码能够帮助到你在实际项目中更高效地处理文件字符串查找任务。
相关问答FAQs:
如何使用Python从文本文件中查找特定字符串?
在Python中,可以使用内置的文件操作功能来打开和读取文本文件,结合字符串方法来查找特定的字符串。首先,打开文件并读取内容,然后使用in
关键字或str.find()
方法来检查字符串是否存在。例如:
with open('yourfile.txt', 'r') as file:
content = file.read()
if 'target_string' in content:
print("字符串找到!")
在处理大文件时,如何高效查找字符串?
当文件较大时,逐行读取文件会更高效。可以使用for
循环逐行读取,并在每一行中查找目标字符串。例如:
with open('yourfile.txt', 'r') as file:
for line in file:
if 'target_string' in line:
print("字符串在以下行找到:", line)
如何查找字符串并返回所有出现的位置?
如果需要找到字符串在文件中所有出现的位置,可以使用str.find()
结合循环来记录每次出现的索引。例如:
positions = []
with open('yourfile.txt', 'r') as file:
content = file.read()
start = 0
while True:
start = content.find('target_string', start)
if start == -1:
break
positions.append(start)
start += len('target_string') # 移动到下一个可能的位置
print("字符串出现的位置:", positions)
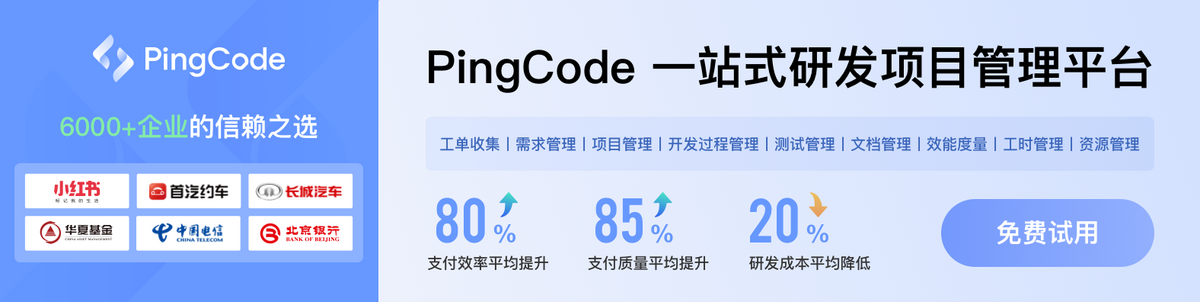