如何将Python程序改写并行计算程序
将Python程序改写为并行计算程序的核心在于:识别计算密集型任务、使用多线程与多进程、利用并行计算库如multiprocessing
和concurrent.futures
、优化数据共享与通信。 在这篇文章中,我们将详细探讨如何通过这些方法来实现Python程序的并行化,以提高计算效率和性能。
并行计算是一种通过同时执行多个计算任务来加速计算过程的技术。Python虽然因其GIL(Global Interpreter Lock)限制了多线程的真正并行性,但通过多进程、协程等技术,仍然可以显著提升程序的运行效率。本文将详细介绍并行计算的相关概念,并提供具体的代码示例,帮助你将Python程序改写为并行计算程序。
一、识别计算密集型任务
在将程序改写为并行计算之前,首先需要识别哪些部分是计算密集型任务。这些任务通常是需要大量CPU时间的操作,例如数据处理、科学计算和图像处理等。
1.1 分析程序瓶颈
使用性能分析工具如cProfile
和line_profiler
来找出程序中的瓶颈。通过这些工具,你可以确定哪些函数或代码段消耗了最多的时间。
import cProfile
import pstats
def long_running_function():
# 模拟一个长时间运行的函数
for _ in range(1000000):
pass
if __name__ == "__main__":
profiler = cProfile.Profile()
profiler.enable()
long_running_function()
profiler.disable()
stats = pstats.Stats(profiler)
stats.sort_stats('cumulative').print_stats(10)
1.2 选择合适的并行化策略
根据分析结果,选择合适的并行化策略。如果任务是CPU密集型的,使用多进程(multiprocessing
)可能更合适;如果是I/O密集型的,使用多线程(threading
)或异步编程(asyncio
)可能更有效。
二、使用多线程与多进程
Python的多线程和多进程是实现并行计算的两种主要方式。多线程适用于I/O密集型任务,而多进程适用于CPU密集型任务。
2.1 多线程(Threading)
多线程通过threading
模块实现。虽然Python的GIL限制了多线程的并行性,但在I/O密集型任务中,多线程仍然能显著提高性能。
import threading
def io_bound_task():
with open('large_file.txt', 'r') as file:
data = file.read()
print("File read successfully")
threads = []
for _ in range(5):
thread = threading.Thread(target=io_bound_task)
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
2.2 多进程(Multiprocessing)
多进程通过multiprocessing
模块实现,适用于CPU密集型任务。每个进程都有独立的内存空间,避免了GIL的限制。
import multiprocessing
def cpu_bound_task(n):
result = 0
for i in range(n):
result += i * i
print(f"Result: {result}")
processes = []
for _ in range(4):
process = multiprocessing.Process(target=cpu_bound_task, args=(1000000,))
processes.append(process)
process.start()
for process in processes:
process.join()
三、利用并行计算库
Python有许多并行计算库,如multiprocessing
、concurrent.futures
、joblib
等。这些库提供了更高层次的抽象,简化了并行编程的复杂性。
3.1 concurrent.futures
concurrent.futures
模块提供了一个高级接口,支持多线程和多进程。你可以使用ThreadPoolExecutor
或ProcessPoolExecutor
来轻松实现并行计算。
from concurrent.futures import ThreadPoolExecutor, as_completed
def fetch_data(url):
response = requests.get(url)
return response.text
urls = ["http://example.com"] * 10
with ThreadPoolExecutor(max_workers=5) as executor:
future_to_url = {executor.submit(fetch_data, url): url for url in urls}
for future in as_completed(future_to_url):
url = future_to_url[future]
try:
data = future.result()
print(f"Data from {url}: {data[:100]}")
except Exception as exc:
print(f"{url} generated an exception: {exc}")
3.2 joblib
joblib
是另一个强大的并行计算库,特别适用于科学计算和数据处理。它提供了简洁的接口来实现任务并行化。
from joblib import Parallel, delayed
def process_data(data):
return sum(data)
data_chunks = [range(1000000)] * 10
results = Parallel(n_jobs=4)(delayed(process_data)(chunk) for chunk in data_chunks)
print(results)
四、优化数据共享与通信
并行计算中的数据共享与通信是一个复杂的问题,需要仔细设计来避免竞争条件和数据不一致。
4.1 使用共享内存
在多进程环境中,可以使用multiprocessing.Value
和multiprocessing.Array
来共享数据。这些对象是进程安全的,可以避免竞争条件。
from multiprocessing import Process, Value
def increment(counter):
for _ in range(100000):
with counter.get_lock():
counter.value += 1
counter = Value('i', 0)
processes = [Process(target=increment, args=(counter,)) for _ in range(4)]
for process in processes:
process.start()
for process in processes:
process.join()
print(f"Final counter value: {counter.value}")
4.2 管道与队列
multiprocessing
模块还提供了Pipe
和Queue
来实现进程间通信。Queue
是线程和进程安全的,可以在多个进程之间安全地传递数据。
from multiprocessing import Process, Queue
def producer(queue):
for i in range(10):
queue.put(i)
def consumer(queue):
while True:
item = queue.get()
if item is None:
break
print(f"Consumed: {item}")
queue = Queue()
producer_process = Process(target=producer, args=(queue,))
consumer_process = Process(target=consumer, args=(queue,))
producer_process.start()
consumer_process.start()
producer_process.join()
queue.put(None) # Signal the consumer to exit
consumer_process.join()
五、并行化常见问题与解决方案
并行计算虽然能显著提升性能,但也带来了新的挑战,如竞争条件、死锁和负载不均衡等问题。
5.1 竞争条件与锁
竞争条件是指多个线程或进程同时访问共享资源时,可能导致数据不一致的问题。使用锁(Lock
)可以避免竞争条件,但也会带来性能开销。
from threading import Thread, Lock
counter = 0
lock = Lock()
def increment():
global counter
for _ in range(100000):
with lock:
counter += 1
threads = [Thread(target=increment) for _ in range(4)]
for thread in threads:
thread.start()
for thread in threads:
thread.join()
print(f"Final counter value: {counter}")
5.2 死锁与解决方法
死锁是指两个或多个线程或进程相互等待对方释放资源,从而导致程序无法继续执行。避免死锁的方法包括:尽量减少锁的使用、使用超时机制和设计无锁算法。
from threading import Thread, Lock
import time
lock1 = Lock()
lock2 = Lock()
def task1():
with lock1:
time.sleep(1)
with lock2:
print("Task 1 completed")
def task2():
with lock2:
time.sleep(1)
with lock1:
print("Task 2 completed")
t1 = Thread(target=task1)
t2 = Thread(target=task2)
t1.start()
t2.start()
t1.join()
t2.join()
六、实战案例:并行化图像处理
为了更好地理解如何将Python程序改写为并行计算程序,我们来看一个实际案例:并行化图像处理。
6.1 图像处理任务
假设我们有一组图像需要进行处理,例如缩放、旋转和滤波等操作。我们可以通过并行计算来加速这一过程。
import cv2
import os
from concurrent.futures import ProcessPoolExecutor
def process_image(image_path):
image = cv2.imread(image_path)
processed_image = cv2.GaussianBlur(image, (15, 15), 0)
output_path = f"processed/{os.path.basename(image_path)}"
cv2.imwrite(output_path, processed_image)
print(f"Processed {image_path}")
image_paths = [f"images/{filename}" for filename in os.listdir("images")]
with ProcessPoolExecutor(max_workers=4) as executor:
executor.map(process_image, image_paths)
6.2 优化与扩展
进一步优化可以通过调整进程数、改进算法或使用GPU加速等方式来实现。我们还可以扩展程序,添加更多的图像处理功能,例如颜色转换、边缘检测等。
import cv2
import os
from concurrent.futures import ProcessPoolExecutor
def process_image(image_path):
image = cv2.imread(image_path)
processed_image = cv2.Canny(image, 100, 200)
output_path = f"processed/{os.path.basename(image_path)}"
cv2.imwrite(output_path, processed_image)
print(f"Processed {image_path}")
image_paths = [f"images/{filename}" for filename in os.listdir("images")]
with ProcessPoolExecutor(max_workers=4) as executor:
executor.map(process_image, image_paths)
七、总结
通过本文的介绍,我们已经详细了解了如何将Python程序改写为并行计算程序。关键步骤包括识别计算密集型任务、选择合适的并行化策略、利用并行计算库、优化数据共享与通信,并解决常见的并行计算问题。通过实际案例的讲解,我们也看到了并行计算在实际应用中的巨大潜力。希望这些内容能帮助你在实际项目中更好地应用并行计算,提高程序的性能和效率。
相关问答FAQs:
如何判断我的Python程序是否适合并行计算?
在决定是否将Python程序改写为并行计算程序之前,可以考虑程序的结构和任务类型。如果程序中存在多个独立的计算任务,且这些任务之间没有太多依赖关系,那么它就适合并行计算。例如,数据处理、图像处理和机器学习中的训练过程通常都是可以并行化的。此外,使用Profiler工具可以帮助识别程序的瓶颈,从而判断并行化的必要性。
在Python中有哪些库可以实现并行计算?
Python提供了多种库来实现并行计算,其中最常用的包括multiprocessing
、concurrent.futures
、joblib
和dask
等。multiprocessing
库允许您创建多个进程并共享数据,而concurrent.futures
提供了更高级别的接口来管理线程和进程。joblib
适合处理大规模数据并行计算,dask
则是一个灵活的并行计算框架,能够处理超出内存限制的大数据集。
并行计算会对程序性能产生怎样的影响?
并行计算能够显著提高程序的执行效率,尤其是在处理大规模计算任务时。然而,性能提升的程度取决于多个因素,包括任务的可并行化程度、系统的硬件配置以及任务间的通信开销。如果任务之间需要频繁地交换数据,可能会导致性能下降。此外,过多的进程或线程也可能造成上下文切换的开销,从而影响效率。因此,在实施并行计算时,合理的设计和优化是至关重要的。
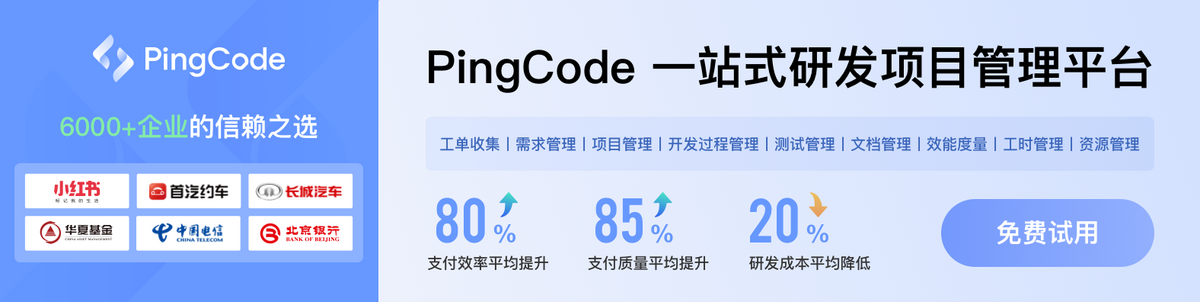