使用Python写一个强提醒信息可以通过多种方式实现,包括桌面通知、电子邮件、短信等。 桌面通知 是一种简单且直接的方式,适合本地提醒; 电子邮件 则适用于需要跨设备提醒的情况; 短信 则是最为即时的提醒方式之一。接下来,我们将详细介绍如何通过这三种方式实现强提醒信息。
一、桌面通知
桌面通知是一种简单且有效的提醒方式,尤其适用于需要即时提醒但不需要跨设备同步的场景。我们可以使用多个Python库来实现这一功能,如 plyer
、win10toast
等。
1.1 使用 plyer
库
plyer
是一个跨平台的库,支持多个操作系统,包括Windows、MacOS和Linux。以下是一个简单的示例:
from plyer import notification
def send_notification(title, message):
notification.notify(
title=title,
message=message,
timeout=10 # 持续时间,单位为秒
)
if __name__ == "__main__":
send_notification("提醒", "这是一个强提醒信息")
1.2 使用 win10toast
库
如果你使用的是Windows系统,那么 win10toast
是一个非常简洁的选择。以下是一个简单的示例:
from win10toast import ToastNotifier
def send_notification(title, message):
toaster = ToastNotifier()
toaster.show_toast(title, message, duration=10)
if __name__ == "__main__":
send_notification("提醒", "这是一个强提醒信息")
二、电子邮件提醒
电子邮件提醒适用于需要跨设备同步的场景。通过电子邮件,你可以在任何有网络连接的设备上收到提醒。我们可以使用 smtplib
库来发送电子邮件。
2.1 配置SMTP服务器
首先,需要配置SMTP服务器。以下是一个发送电子邮件的示例:
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
def send_email(subject, body, to_email):
from_email = "your_email@example.com"
from_password = "your_password"
msg = MIMEMultipart()
msg['From'] = from_email
msg['To'] = to_email
msg['Subject'] = subject
msg.attach(MIMEText(body, 'plain'))
try:
server = smtplib.SMTP('smtp.example.com', 587)
server.starttls()
server.login(from_email, from_password)
text = msg.as_string()
server.sendmail(from_email, to_email, text)
server.quit()
print("邮件发送成功")
except Exception as e:
print(f"邮件发送失败: {e}")
if __name__ == "__main__":
send_email("提醒", "这是一个强提醒信息", "receiver@example.com")
三、短信提醒
短信提醒是最为即时的提醒方式之一,适用于需要在第一时间通知用户的重要信息。我们可以使用第三方服务提供商如 Twilio 来发送短信。
3.1 使用 Twilio 发送短信
首先,需要注册一个 Twilio 账号,并获取相应的 API 密钥和电话号码。以下是一个发送短信的示例:
from twilio.rest import Client
def send_sms(message, to_phone):
account_sid = 'your_account_sid'
auth_token = 'your_auth_token'
from_phone = 'your_twilio_phone_number'
client = Client(account_sid, auth_token)
message = client.messages.create(
body=message,
from_=from_phone,
to=to_phone
)
print(f"短信发送成功: {message.sid}")
if __name__ == "__main__":
send_sms("这是一个强提醒信息", "+1234567890")
四、综合应用
在实际应用中,可能需要结合多种提醒方式来确保信息的传递。例如,可以先发送桌面通知,如果用户未响应,再发送电子邮件或短信。以下是一个综合应用的示例:
import time
from plyer import notification
from twilio.rest import Client
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
def send_notification(title, message):
notification.notify(
title=title,
message=message,
timeout=10
)
def send_email(subject, body, to_email):
from_email = "your_email@example.com"
from_password = "your_password"
msg = MIMEMultipart()
msg['From'] = from_email
msg['To'] = to_email
msg['Subject'] = subject
msg.attach(MIMEText(body, 'plain'))
try:
server = smtplib.SMTP('smtp.example.com', 587)
server.starttls()
server.login(from_email, from_password)
text = msg.as_string()
server.sendmail(from_email, to_email, text)
server.quit()
print("邮件发送成功")
except Exception as e:
print(f"邮件发送失败: {e}")
def send_sms(message, to_phone):
account_sid = 'your_account_sid'
auth_token = 'your_auth_token'
from_phone = 'your_twilio_phone_number'
client = Client(account_sid, auth_token)
message = client.messages.create(
body=message,
from_=from_phone,
to=to_phone
)
print(f"短信发送成功: {message.sid}")
if __name__ == "__main__":
send_notification("提醒", "这是一个强提醒信息")
time.sleep(10) # 等待10秒,模拟用户未响应
send_email("提醒", "这是一个强提醒信息", "receiver@example.com")
send_sms("这是一个强提醒信息", "+1234567890")
总结
通过以上示例,我们可以看到,使用Python实现强提醒信息的方法多种多样,可以根据具体需求选择合适的方式。桌面通知 简单直接,适合本地提醒;电子邮件 适用于需要跨设备同步的场景;短信 是最为即时的提醒方式。综合应用这些方法,可以确保信息及时、准确地传递给目标用户。
相关问答FAQs:
如何通过Python创建定时提醒功能?
可以使用Python的schedule
库来创建定时提醒。首先安装该库,然后通过编写一个简单的函数,设置定时任务来发送提醒信息。你可以使用time.sleep()
或while
循环来持续运行程序,确保提醒在设定的时间触发。
Python中有哪些库可以用于发送提醒信息?
常用的库包括smptlib
用于发送电子邮件提醒,twilio
用于发送短信提醒,以及plyer
用于桌面通知。根据你的需求选择合适的库,搭配相应的API密钥,可以方便地实现提醒功能。
如何提高提醒信息的用户体验?
为了提升用户体验,可以考虑在提醒信息中加入个性化内容,比如用户的名字或特定事件的详情。此外,使用图形用户界面(如tkinter
或PyQt
)提供可视化的提醒,或者添加声音提示,均能增强用户的互动感和及时性。
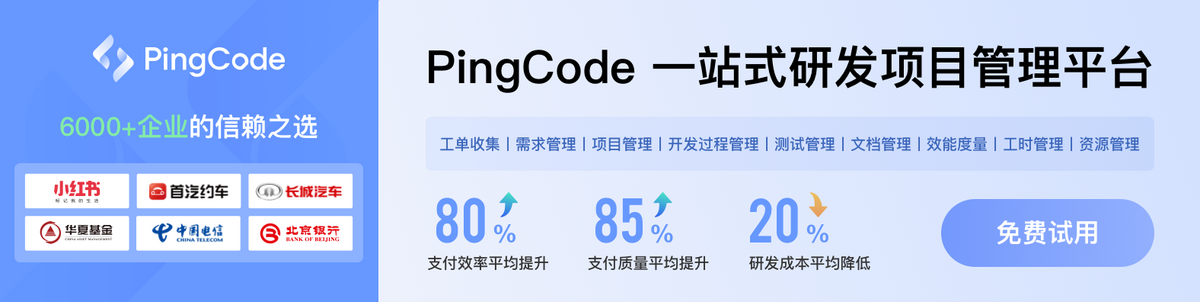