在Python中对视频中的物体进行框选,可以使用OpenCV、TensorFlow、PyTorch等工具。这些工具提供了强大的计算机视觉和深度学习功能,可以帮助你实现物体检测和框选。在本文中,我们将详细介绍如何使用OpenCV和深度学习模型来实现这一功能,并提供一些代码示例来帮助你更好地理解。
一、理解物体检测的基本概念
物体检测是计算机视觉中的一个重要任务,旨在识别和定位图像或视频中的特定物体。这一任务通常包括两个主要步骤:物体分类和物体定位。物体分类是指识别图像或视频中的物体类型,而物体定位是指在图像或视频中找到物体的位置,并用边界框将其框选出来。
在物体检测中,常用的算法包括YOLO(You Only Look Once)、SSD(Single Shot MultiBox Detector)和Faster R-CNN(Region-Based Convolutional Neural Networks)。这些算法在准确性和速度上各有优劣,可以根据具体应用场景进行选择。
二、使用OpenCV进行物体检测
OpenCV是一个开源的计算机视觉和机器学习软件库,支持多种编程语言,包括Python。OpenCV提供了丰富的图像处理功能,可以用于实现物体检测和框选。
- 安装OpenCV
首先,你需要安装OpenCV库,可以使用pip命令进行安装:
pip install opencv-python
pip install opencv-python-headless
- 加载视频文件
在开始物体检测之前,需要加载视频文件。可以使用OpenCV的cv2.VideoCapture
函数来加载视频文件:
import cv2
加载视频文件
video_path = 'path/to/your/video.mp4'
cap = cv2.VideoCapture(video_path)
检查视频文件是否成功加载
if not cap.isOpened():
print("Error: Could not open video.")
exit()
- 使用预训练模型进行物体检测
OpenCV提供了一些预训练模型,可以用于物体检测。这里我们使用MobileNet-SSD模型,该模型在速度和准确性上表现良好。
首先,下载MobileNet-SSD模型的权重文件和配置文件:
- 权重文件:
MobileNetSSD_deploy.caffemodel
- 配置文件:
MobileNetSSD_deploy.prototxt
然后,加载模型并进行物体检测:
import numpy as np
加载模型
net = cv2.dnn.readNetFromCaffe('path/to/MobileNetSSD_deploy.prototxt',
'path/to/MobileNetSSD_deploy.caffemodel')
物体类别
CLASSES = ["background", "aeroplane", "bicycle", "bird", "boat",
"bottle", "bus", "car", "cat", "chair", "cow", "diningtable",
"dog", "horse", "motorbike", "person", "pottedplant", "sheep",
"sofa", "train", "tvmonitor"]
while True:
# 读取视频帧
ret, frame = cap.read()
if not ret:
break
# 预处理视频帧
(h, w) = frame.shape[:2]
blob = cv2.dnn.blobFromImage(cv2.resize(frame, (300, 300)), 0.007843, (300, 300), 127.5)
# 将图像输入到网络中
net.setInput(blob)
detections = net.forward()
# 遍历检测结果
for i in range(detections.shape[2]):
confidence = detections[0, 0, i, 2]
# 过滤掉低置信度的检测结果
if confidence > 0.2:
idx = int(detections[0, 0, i, 1])
box = detections[0, 0, i, 3:7] * np.array([w, h, w, h])
(startX, startY, endX, endY) = box.astype("int")
# 绘制边界框和标签
label = "{}: {:.2f}%".format(CLASSES[idx], confidence * 100)
cv2.rectangle(frame, (startX, startY), (endX, endY), (0, 255, 0), 2)
y = startY - 15 if startY - 15 > 15 else startY + 15
cv2.putText(frame, label, (startX, y), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 255, 0), 2)
# 显示视频帧
cv2.imshow("Frame", frame)
key = cv2.waitKey(1) & 0xFF
# 按'q'键退出
if key == ord('q'):
break
释放资源
cap.release()
cv2.destroyAllWindows()
三、使用深度学习模型进行物体检测
除了OpenCV的预训练模型外,还可以使用深度学习模型进行物体检测。常用的深度学习框架包括TensorFlow和PyTorch。
- 使用TensorFlow进行物体检测
TensorFlow是一个开源的机器学习框架,支持多种深度学习模型。可以使用TensorFlow的Object Detection API进行物体检测。
首先,安装TensorFlow和Object Detection API:
pip install tensorflow
pip install tensorflow-object-detection-api
然后,下载预训练模型并进行物体检测:
import tensorflow as tf
import numpy as np
import cv2
from object_detection.utils import label_map_util
from object_detection.utils import visualization_utils as vis_util
加载模型
model_path = 'path/to/saved_model'
detection_model = tf.saved_model.load(model_path)
加载标签映射文件
label_map_path = 'path/to/label_map.pbtxt'
category_index = label_map_util.create_category_index_from_labelmap(label_map_path, use_display_name=True)
while True:
# 读取视频帧
ret, frame = cap.read()
if not ret:
break
# 转换图像格式
input_tensor = tf.convert_to_tensor(frame)
input_tensor = input_tensor[tf.newaxis, ...]
# 进行物体检测
detections = detection_model(input_tensor)
# 处理检测结果
num_detections = int(detections.pop('num_detections'))
detections = {key: value[0, :num_detections].numpy() for key, value in detections.items()}
detections['num_detections'] = num_detections
# 检测类别和置信度
detection_classes = detections['detection_classes'].astype(np.int64)
detection_boxes = detections['detection_boxes']
detection_scores = detections['detection_scores']
# 可视化检测结果
vis_util.visualize_boxes_and_labels_on_image_array(
frame,
detection_boxes,
detection_classes,
detection_scores,
category_index,
use_normalized_coordinates=True,
line_thickness=8)
# 显示视频帧
cv2.imshow("Frame", frame)
key = cv2.waitKey(1) & 0xFF
# 按'q'键退出
if key == ord('q'):
break
释放资源
cap.release()
cv2.destroyAllWindows()
- 使用PyTorch进行物体检测
PyTorch是另一个流行的深度学习框架,也可以用于物体检测。可以使用PyTorch的预训练模型进行物体检测。
首先,安装PyTorch:
pip install torch
pip install torchvision
然后,加载预训练模型并进行物体检测:
import torch
import torchvision
from torchvision import transforms
import cv2
加载预训练模型
model = torchvision.models.detection.fasterrcnn_resnet50_fpn(pretrained=True)
model.eval()
图像预处理
transform = transforms.Compose([
transforms.ToTensor(),
])
while True:
# 读取视频帧
ret, frame = cap.read()
if not ret:
break
# 转换图像格式
image = transform(frame)
image = image.unsqueeze(0)
# 进行物体检测
with torch.no_grad():
detections = model(image)
# 处理检测结果
detection_boxes = detections[0]['boxes'].numpy()
detection_scores = detections[0]['scores'].numpy()
detection_labels = detections[0]['labels'].numpy()
# 绘制边界框和标签
for i in range(len(detection_boxes)):
if detection_scores[i] > 0.5:
box = detection_boxes[i]
label = detection_labels[i]
startX, startY, endX, endY = box.astype("int")
cv2.rectangle(frame, (startX, startY), (endX, endY), (0, 255, 0), 2)
label_text = f"Label: {label}, Score: {detection_scores[i]:.2f}"
cv2.putText(frame, label_text, (startX, startY - 10), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 255, 0), 2)
# 显示视频帧
cv2.imshow("Frame", frame)
key = cv2.waitKey(1) & 0xFF
# 按'q'键退出
if key == ord('q'):
break
释放资源
cap.release()
cv2.destroyAllWindows()
四、总结
在本文中,我们介绍了如何在Python中对视频中的物体进行框选。我们首先介绍了物体检测的基本概念,然后详细介绍了如何使用OpenCV和深度学习模型(包括TensorFlow和PyTorch)进行物体检测。通过这些示例代码,你可以更好地理解如何在实际应用中实现物体检测和框选。希望这篇文章对你有所帮助,能够为你的项目提供指导。
相关问答FAQs:
如何在Python中处理视频文件以进行物体检测?
在Python中,可以使用OpenCV库来读取视频文件并进行处理。通过结合深度学习模型(如YOLO或SSD),可以实现物体检测并在视频中框选这些物体。首先,确保安装了OpenCV和相应的深度学习框架,然后使用cv2.VideoCapture读取视频文件,处理每一帧并应用物体检测算法。
在视频中框选物体时,如何提高检测的准确性?
为了提高物体检测的准确性,可以考虑使用经过预训练的深度学习模型,并对其进行微调以适应特定场景。此外,调整检测阈值、使用数据增强技术和优化输入图像的质量也能有效提升检测效果。
是否可以使用其他库来实现视频中的物体框选?
除了OpenCV,TensorFlow和PyTorch等深度学习框架也可以用于物体检测。TensorFlow提供了对象检测API,支持多种预训练模型,用户可以方便地加载和使用这些模型来进行视频处理和物体框选。PyTorch同样有丰富的社区支持和模型选择,适合进行定制化开发。
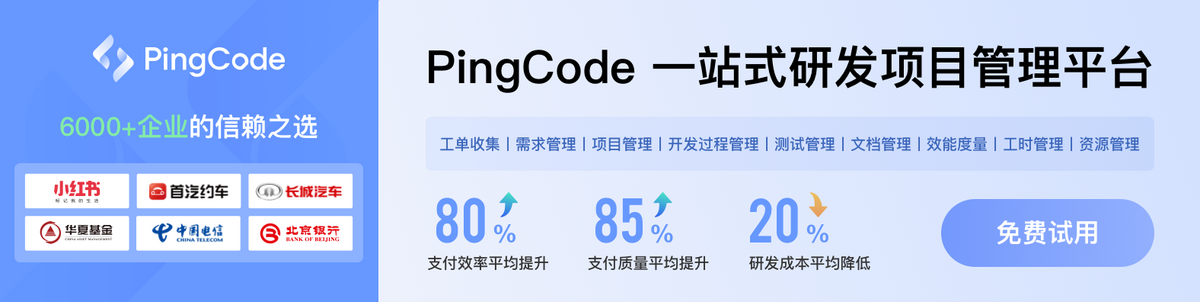