一、如何读取Python中的txt字典并将其存入数据库
在Python中读取txt字典并将其存入数据库的过程可以总结为以下几个关键步骤:读取txt文件、解析字典数据、连接数据库、插入数据。其中,解析字典数据是最关键的一步,因为它直接影响到数据的准确性和完整性。
解析字典数据的过程需要特别注意字典结构的复杂性。如果字典是简单的键值对形式,解析起来会比较简单。但如果字典嵌套了更复杂的数据结构(如列表、嵌套字典等),则需要更复杂的解析逻辑。接下来,我们将详细探讨这些步骤。
二、读取txt文件
读取txt文件是整个流程的第一步。Python提供了多种读取文本文件的方法,其中最常用的是使用内置的open
函数。通过open
函数,我们可以轻松地读取文件内容并将其存储在一个变量中以便后续处理。
def read_txt_file(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
data = file.read()
return data
在上述代码中,我们使用open
函数打开文件,并通过read
方法将文件内容读取到一个字符串变量data
中。with
语句确保文件在操作完成后自动关闭,避免资源泄露。
三、解析字典数据
解析字典数据是将读取到的文本内容转换为Python字典对象的关键步骤。根据文件内容的不同,解析方法也会有所不同。常见的字典格式包括JSON、CSV等。
1、解析JSON格式的字典
如果txt文件中的字典是JSON格式,我们可以使用json
模块来解析。
import json
def parse_json_data(data):
try:
dictionary = json.loads(data)
except json.JSONDecodeError as e:
print(f"Error decoding JSON: {e}")
return None
return dictionary
在上述代码中,我们使用json.loads
方法将字符串数据转换为Python字典对象。如果解析过程中出现错误,JSONDecodeError
异常将被捕获并处理。
2、解析CSV格式的字典
如果txt文件中的字典是CSV格式,我们可以使用csv
模块来解析。
import csv
def parse_csv_data(data):
dictionary = {}
reader = csv.DictReader(data.splitlines())
for row in reader:
key = row['key_column']
value = row['value_column']
dictionary[key] = value
return dictionary
在上述代码中,我们使用csv.DictReader
将CSV数据解析为字典对象。需要注意的是,key_column
和value_column
应替换为实际的列名。
四、连接数据库
将解析好的字典数据存入数据库之前,需要先连接到数据库。Python支持多种数据库类型,包括SQLite、MySQL、PostgreSQL等。我们以SQLite为例,演示如何连接数据库。
import sqlite3
def connect_to_database(db_path):
try:
connection = sqlite3.connect(db_path)
except sqlite3.Error as e:
print(f"Error connecting to database: {e}")
return None
return connection
在上述代码中,我们使用sqlite3.connect
方法连接SQLite数据库。如果连接过程中出现错误,sqlite3.Error
异常将被捕获并处理。
五、插入数据
将字典数据存入数据库的过程可以分为创建表结构和插入数据两个步骤。
1、创建表结构
在插入数据之前,需要先创建表结构。假设我们的字典数据包含两个字段key
和value
,可以使用以下SQL语句创建表结构。
def create_table(connection):
create_table_sql = """
CREATE TABLE IF NOT EXISTS dictionary (
key TEXT PRIMARY KEY,
value TEXT NOT NULL
);
"""
try:
cursor = connection.cursor()
cursor.execute(create_table_sql)
connection.commit()
except sqlite3.Error as e:
print(f"Error creating table: {e}")
在上述代码中,我们使用cursor.execute
方法执行SQL语句创建表结构,并使用connection.commit
方法提交事务。
2、插入数据
创建表结构后,可以使用INSERT
语句将字典数据插入数据库。
def insert_data(connection, dictionary):
insert_sql = """
INSERT INTO dictionary (key, value)
VALUES (?, ?)
"""
try:
cursor = connection.cursor()
for key, value in dictionary.items():
cursor.execute(insert_sql, (key, value))
connection.commit()
except sqlite3.Error as e:
print(f"Error inserting data: {e}")
在上述代码中,我们使用cursor.execute
方法执行INSERT
语句将字典数据插入数据库,并使用connection.commit
方法提交事务。
六、综合示例
将上述步骤整合在一起,我们可以得到一个完整的示例程序。
import json
import sqlite3
import csv
def read_txt_file(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
data = file.read()
return data
def parse_json_data(data):
try:
dictionary = json.loads(data)
except json.JSONDecodeError as e:
print(f"Error decoding JSON: {e}")
return None
return dictionary
def parse_csv_data(data):
dictionary = {}
reader = csv.DictReader(data.splitlines())
for row in reader:
key = row['key_column']
value = row['value_column']
dictionary[key] = value
return dictionary
def connect_to_database(db_path):
try:
connection = sqlite3.connect(db_path)
except sqlite3.Error as e:
print(f"Error connecting to database: {e}")
return None
return connection
def create_table(connection):
create_table_sql = """
CREATE TABLE IF NOT EXISTS dictionary (
key TEXT PRIMARY KEY,
value TEXT NOT NULL
);
"""
try:
cursor = connection.cursor()
cursor.execute(create_table_sql)
connection.commit()
except sqlite3.Error as e:
print(f"Error creating table: {e}")
def insert_data(connection, dictionary):
insert_sql = """
INSERT INTO dictionary (key, value)
VALUES (?, ?)
"""
try:
cursor = connection.cursor()
for key, value in dictionary.items():
cursor.execute(insert_sql, (key, value))
connection.commit()
except sqlite3.Error as e:
print(f"Error inserting data: {e}")
def main():
file_path = 'path/to/your/txt/file.txt'
db_path = 'path/to/your/database.db'
data = read_txt_file(file_path)
dictionary = parse_json_data(data) # or parse_csv_data(data) based on your file format
if dictionary is None:
print("Failed to parse dictionary data.")
return
connection = connect_to_database(db_path)
if connection is None:
print("Failed to connect to database.")
return
create_table(connection)
insert_data(connection, dictionary)
connection.close()
print("Data inserted successfully.")
if __name__ == "__main__":
main()
在上述代码中,我们通过调用main
函数整合所有步骤,实现了从读取txt文件到将字典数据存入数据库的完整流程。
七、优化和扩展
在实际应用中,可能会遇到一些特殊情况和需求。例如,字典数据量较大时,可以考虑使用批量插入的方式提高效率。此外,还可以根据具体需求,对数据库表结构和数据处理逻辑进行优化和扩展。
1、批量插入数据
当字典数据量较大时,逐条插入数据的效率较低。可以使用executemany
方法进行批量插入。
def insert_data_batch(connection, dictionary):
insert_sql = """
INSERT INTO dictionary (key, value)
VALUES (?, ?)
"""
try:
cursor = connection.cursor()
data = [(key, value) for key, value in dictionary.items()]
cursor.executemany(insert_sql, data)
connection.commit()
except sqlite3.Error as e:
print(f"Error inserting data: {e}")
在上述代码中,我们使用executemany
方法批量插入数据,提高了插入效率。
2、处理复杂字典结构
如果字典结构较为复杂(如嵌套字典、列表等),需要设计更复杂的解析和存储逻辑。可以通过递归解析字典结构,并在数据库中创建多张表存储不同层级的数据。
def parse_complex_dict(data):
# Implement your logic to parse complex dictionary structure
pass
def create_complex_table(connection):
# Implement your logic to create tables for complex dictionary structure
pass
def insert_complex_data(connection, dictionary):
# Implement your logic to insert complex dictionary data into database
pass
在上述代码中,根据具体的字典结构和需求,实现相应的解析、表创建和数据插入逻辑。
八、结论
通过上述步骤,我们可以实现从读取txt文件、解析字典数据到将数据存入数据库的完整流程。根据具体需求,可以对解析和存储逻辑进行优化和扩展,以适应不同的数据格式和复杂度。在实际应用中,建议根据数据量和性能需求,选择合适的数据库类型和优化策略,以确保系统的稳定性和效率。
相关问答FAQs:
如何将txt字典中的数据导入到Python数据库中?
要将txt字典中的数据导入到Python数据库中,您可以使用Python内置的文件处理功能读取文件内容,将其解析为字典格式,然后使用数据库连接库(如sqlite3或SQLAlchemy)将数据插入数据库。确保在读取时处理好数据格式,以便正确映射到数据库表中。
txt字典格式如何影响数据库的读取过程?
txt字典的格式会直接影响读取和解析的方式。常见的格式包括JSON、CSV或特定的键值对格式。确保了解字典的结构,使用相应的解析方法以便能顺利将数据导入数据库。此外,清晰的数据结构还可以减少后续数据处理的复杂性。
在读取txt字典时,如何处理异常数据?
在读取txt字典时,可能会遇到格式不正确或缺失值的情况。可以使用try-except语句捕获异常,记录错误并继续处理其他数据。此外,建议在数据插入数据库之前进行数据验证和清洗,以确保数据库中的数据质量和一致性。
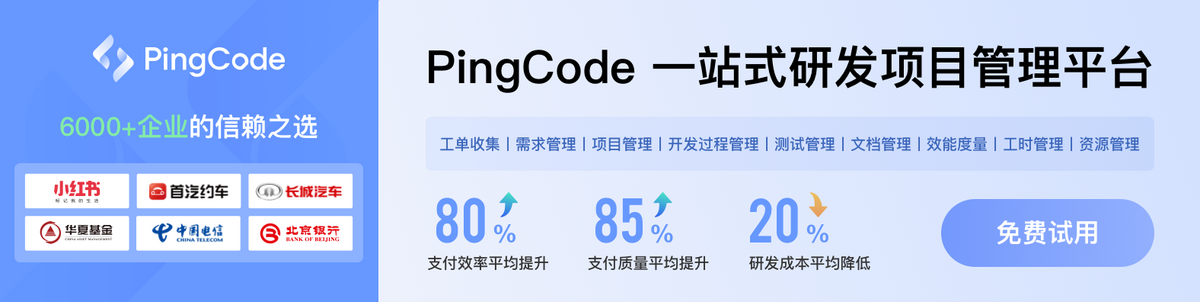