如何用Python做一个密码锁
用Python做一个密码锁的方法有:设计逻辑简单、使用安全库、提供用户交互、实现加密存储、处理错误和异常。 其中,设计逻辑简单是最关键的一点。一个简单的设计逻辑可以确保代码易于理解和维护,并减少潜在的错误。在这篇文章中,我们将详细探讨每一个方法,帮助你构建一个功能齐全的密码锁系统。
一、设计逻辑简单
设计一个简单的逻辑是创建密码锁系统的第一步。简单的逻辑不仅可以使代码更易于理解,还能减少潜在的错误。以下是一些基本步骤:
- 定义密码:首先,定义一个预设密码或从用户输入获取密码。
- 创建验证函数:编写一个函数,接收用户输入并验证其是否与预设密码匹配。
- 设置尝试次数:设置一个允许的最大尝试次数,以防止暴力破解。
- 反馈机制:为用户提供正确或错误的反馈。
一个简单的例子如下:
def password_lock():
preset_password = "1234"
max_attempts = 3
attempts = 0
while attempts < max_attempts:
user_input = input("Enter the password: ")
if user_input == preset_password:
print("Access Granted")
return True
else:
print("Incorrect Password")
attempts += 1
print("Access Denied")
return False
password_lock()
二、使用安全库
为了增强密码锁的安全性,可以使用一些Python安全库,如hashlib
和hmac
。这些库可以帮助你加密存储的密码,并进行安全的比较。
1. 使用 hashlib
进行加密
hashlib
是一个内置的Python库,可以用于生成密码的哈希值。以下是一个使用hashlib
进行密码加密和验证的示例:
import hashlib
def hash_password(password):
return hashlib.sha256(password.encode()).hexdigest()
def password_lock():
preset_password_hash = hash_password("1234")
max_attempts = 3
attempts = 0
while attempts < max_attempts:
user_input = input("Enter the password: ")
if hash_password(user_input) == preset_password_hash:
print("Access Granted")
return True
else:
print("Incorrect Password")
attempts += 1
print("Access Denied")
return False
password_lock()
2. 使用 hmac
进行安全比较
为了防止时间攻击,可以使用hmac.compare_digest
进行安全比较:
import hmac
def secure_compare(a, b):
return hmac.compare_digest(a, b)
def password_lock():
preset_password = "1234"
preset_password_hash = hashlib.sha256(preset_password.encode()).hexdigest()
max_attempts = 3
attempts = 0
while attempts < max_attempts:
user_input = input("Enter the password: ")
user_input_hash = hashlib.sha256(user_input.encode()).hexdigest()
if secure_compare(user_input_hash, preset_password_hash):
print("Access Granted")
return True
else:
print("Incorrect Password")
attempts += 1
print("Access Denied")
return False
password_lock()
三、提供用户交互
一个良好的用户交互体验是密码锁系统的关键部分。使用命令行界面(CLI)或图形用户界面(GUI)都可以增强用户体验。
1. 命令行界面
命令行界面是最简单的实现方式,只需要使用input
函数接收用户输入,并使用print
函数提供反馈。
def password_lock():
preset_password = "1234"
max_attempts = 3
attempts = 0
while attempts < max_attempts:
user_input = input("Enter the password: ")
if user_input == preset_password:
print("Access Granted")
return True
else:
print("Incorrect Password")
attempts += 1
print("Access Denied")
return False
password_lock()
2. 图形用户界面
使用tkinter
库可以创建一个简单的图形用户界面:
import tkinter as tk
from tkinter import messagebox
def check_password():
preset_password = "1234"
user_input = password_entry.get()
if user_input == preset_password:
messagebox.showinfo("Result", "Access Granted")
else:
messagebox.showwarning("Result", "Incorrect Password")
app = tk.Tk()
app.title("Password Lock")
password_label = tk.Label(app, text="Enter Password:")
password_label.pack()
password_entry = tk.Entry(app, show="*")
password_entry.pack()
submit_button = tk.Button(app, text="Submit", command=check_password)
submit_button.pack()
app.mainloop()
四、实现加密存储
为了进一步增强安全性,可以将密码存储在一个加密的文件中。使用cryptography
库可以实现这一点。
1. 安装 cryptography
库
首先,安装cryptography
库:
pip install cryptography
2. 使用 cryptography
进行加密和解密
以下是一个使用cryptography
库进行加密存储和验证的示例:
from cryptography.fernet import Fernet
def generate_key():
return Fernet.generate_key()
def encrypt_password(password, key):
f = Fernet(key)
return f.encrypt(password.encode())
def decrypt_password(encrypted_password, key):
f = Fernet(key)
return f.decrypt(encrypted_password).decode()
def password_lock():
key = generate_key()
preset_password = "1234"
encrypted_password = encrypt_password(preset_password, key)
max_attempts = 3
attempts = 0
while attempts < max_attempts:
user_input = input("Enter the password: ")
if user_input == decrypt_password(encrypted_password, key):
print("Access Granted")
return True
else:
print("Incorrect Password")
attempts += 1
print("Access Denied")
return False
password_lock()
五、处理错误和异常
在实际应用中,处理错误和异常是确保系统稳定性的重要环节。以下是一些常见的错误和异常处理方法:
1. 捕获输入错误
使用try-except
块捕获和处理输入错误:
def password_lock():
preset_password = "1234"
max_attempts = 3
attempts = 0
while attempts < max_attempts:
try:
user_input = input("Enter the password: ")
if user_input == preset_password:
print("Access Granted")
return True
else:
print("Incorrect Password")
attempts += 1
except Exception as e:
print(f"Error: {e}")
attempts += 1
print("Access Denied")
return False
password_lock()
2. 处理文件读写错误
在进行文件操作时,处理可能的读写错误:
def read_password_file(file_path):
try:
with open(file_path, 'r') as file:
return file.read().strip()
except FileNotFoundError:
print("Password file not found")
except Exception as e:
print(f"Error: {e}")
def password_lock():
preset_password = read_password_file("password.txt")
if not preset_password:
return False
max_attempts = 3
attempts = 0
while attempts < max_attempts:
user_input = input("Enter the password: ")
if user_input == preset_password:
print("Access Granted")
return True
else:
print("Incorrect Password")
attempts += 1
print("Access Denied")
return False
password_lock()
结论
通过设计简单的逻辑、使用安全库、提供用户交互、实现加密存储和处理错误和异常,你可以用Python创建一个功能齐全且安全的密码锁系统。无论是用于个人项目还是实际应用,这些方法都可以帮助你提高系统的安全性和用户体验。
关键点总结:设计逻辑简单、使用安全库、提供用户交互、实现加密存储、处理错误和异常。 通过详细理解和实现这些关键点,你将能够创建一个高效、安全的密码锁系统。
相关问答FAQs:
1. 如何使用Python创建一个简单的命令行密码锁程序?
要创建一个简单的命令行密码锁程序,可以使用Python的input函数来接收用户输入的密码,并与预设的密码进行比较。可以使用循环来允许用户多次尝试输入密码,直到输入正确或达到最大尝试次数。示例代码如下:
correct_password = "1234"
max_attempts = 3
attempts = 0
while attempts < max_attempts:
user_input = input("请输入密码: ")
if user_input == correct_password:
print("密码正确,欢迎进入!")
break
else:
attempts += 1
print("密码错误,请重试。")
else:
print("尝试次数过多,锁定。")
2. 如何在Python中实现图形用户界面的密码锁?
要在Python中实现图形用户界面的密码锁,可以使用Tkinter库。这个库提供了简单的方式来创建窗口和输入框。通过设置一个输入框来接受用户输入的密码,并通过按钮进行验证。示例代码如下:
import tkinter as tk
from tkinter import messagebox
def check_password():
if entry.get() == "1234":
messagebox.showinfo("成功", "密码正确,欢迎进入!")
else:
messagebox.showwarning("失败", "密码错误,请重试。")
root = tk.Tk()
root.title("密码锁")
entry = tk.Entry(root, show='*')
entry.pack(pady=10)
button = tk.Button(root, text="验证", command=check_password)
button.pack(pady=5)
root.mainloop()
3. 在创建密码锁时,如何确保安全性和防止暴力破解?
为了提高密码锁的安全性,可以采取多种措施。首先,选择较复杂的密码,例如包含字母、数字和特殊字符的组合。其次,可以在多次输入错误密码后暂时锁定账户,增加等待时间。还可以考虑使用哈希函数存储密码,而不是直接存储明文密码,这样即使程序被攻击,密码也不会被轻易获取。此外,建议定期更换密码,确保安全性。
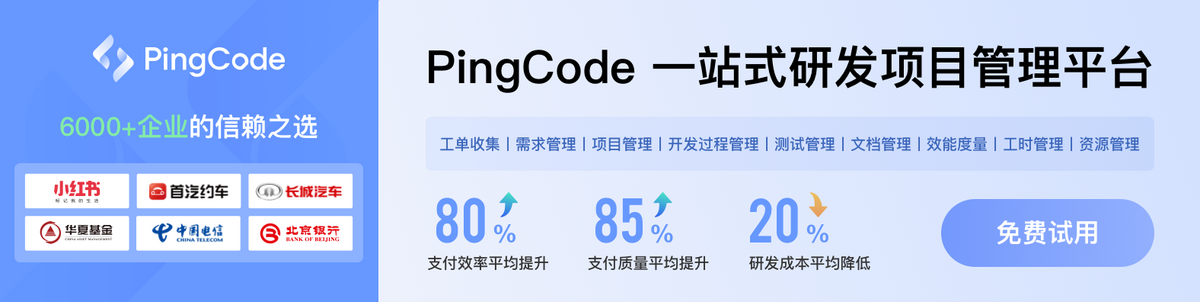