使用Python将文件放入指定文件夹的方法有多种,包括os模块、shutil模块等。首先,你需要导入必要的库、使用os.path.join()构造路径、使用shutil.move()移动文件。
一、导入必要的库
Python提供了一些内置模块,如os和shutil,这些模块可以帮助你进行文件和目录的操作。导入这些模块是你进行文件操作的第一步。
import os
import shutil
二、使用os.path.join()构造路径
os.path.join() 是一个非常方便的方法,可以帮助你构建跨平台的路径。你只需提供路径的各个部分,它会自动处理路径分隔符等问题。
详细描述:os.path.join()的使用
os.path.join() 函数用于智能地连接一个或多个路径部分。它会根据你的操作系统自动选择正确的路径分隔符。例如,在Windows上使用反斜杠(\),而在Unix/Linux上则使用正斜杠(/)。这使得你的代码更具可移植性。
source_file = "source.txt"
destination_dir = "destination_folder"
destination_path = os.path.join(destination_dir, os.path.basename(source_file))
三、使用shutil.move()移动文件
shutil.move() 是一个高效的方法,用于将文件或目录从一个位置移动到另一个位置。如果目标位置已经存在文件,它会覆盖该文件。
shutil.move(source_file, destination_path)
一、导入必要的库
Python 提供了多个内置模块来进行文件操作,这使得任务变得相对简单。os 和 shutil 模块是最常用的,它们允许你进行各种文件和目录操作,包括复制、移动和删除文件。
1、导入os模块
os 模块提供了一种便携的方式来使用操作系统功能。无论你使用的是 Windows、Linux 还是 macOS,os 模块都能帮助你进行文件和目录操作。
import os
2、导入shutil模块
shutil 模块提供了更高级别的文件操作功能,例如复制和移动文件。它是 os 模块的一个补充,专门用于文件和目录的高级操作。
import shutil
二、构造路径
构建正确的文件路径是文件操作中的关键步骤。使用 os.path.join() 可以确保路径在各种操作系统上都能正常工作。
1、os.path.join()的使用
os.path.join() 函数可以智能地连接一个或多个路径部分。它会根据你的操作系统自动选择正确的路径分隔符,确保路径的正确性。
source_file = "source.txt"
destination_dir = "destination_folder"
destination_path = os.path.join(destination_dir, os.path.basename(source_file))
2、确保目标文件夹存在
在将文件移动到目标文件夹之前,确保该文件夹存在是非常重要的。你可以使用 os.makedirs() 函数来创建目标文件夹及其父文件夹(如果它们不存在)。
if not os.path.exists(destination_dir):
os.makedirs(destination_dir)
三、移动文件
使用 shutil.move() 可以将文件从一个位置移动到另一个位置。如果目标位置已经存在文件,它会覆盖该文件。
1、shutil.move()的使用
shutil.move() 是一个高效的方法,用于将文件或目录从一个位置移动到另一个位置。它不仅可以移动文件,还可以移动整个目录。
shutil.move(source_file, destination_path)
2、处理移动过程中的错误
在移动文件时,可能会遇到各种错误,例如文件不存在、权限不足等。你可以使用 try-except 结构来捕获和处理这些错误。
try:
shutil.move(source_file, destination_path)
print(f"{source_file} has been moved to {destination_path}")
except FileNotFoundError:
print(f"{source_file} not found")
except PermissionError:
print(f"Permission denied while moving {source_file}")
except Exception as e:
print(f"Error occurred: {e}")
四、操作示例
为了更好地理解如何将文件放入指定文件夹,我们可以看一个完整的示例。这个示例展示了如何使用 os 和 shutil 模块来移动文件,并处理可能出现的错误。
import os
import shutil
def move_file(source_file, destination_dir):
# 构造目标路径
destination_path = os.path.join(destination_dir, os.path.basename(source_file))
# 确保目标文件夹存在
if not os.path.exists(destination_dir):
os.makedirs(destination_dir)
try:
# 移动文件
shutil.move(source_file, destination_path)
print(f"{source_file} has been moved to {destination_path}")
except FileNotFoundError:
print(f"{source_file} not found")
except PermissionError:
print(f"Permission denied while moving {source_file}")
except Exception as e:
print(f"Error occurred: {e}")
示例调用
source_file = "source.txt"
destination_dir = "destination_folder"
move_file(source_file, destination_dir)
五、使用场景
在实际应用中,文件操作是非常常见的需求。以下是几个常见的使用场景:
1、备份文件
你可以使用 os 和 shutil 模块来实现文件的自动备份。例如,每天定时将某个文件夹中的文件备份到另一个文件夹。
import os
import shutil
from datetime import datetime
def backup_files(source_dir, backup_dir):
# 获取当前日期
date_str = datetime.now().strftime("%Y-%m-%d")
# 构造备份文件夹路径
backup_path = os.path.join(backup_dir, date_str)
if not os.path.exists(backup_path):
os.makedirs(backup_path)
# 移动文件到备份文件夹
for file_name in os.listdir(source_dir):
source_file = os.path.join(source_dir, file_name)
destination_path = os.path.join(backup_path, file_name)
shutil.move(source_file, destination_path)
print(f"{source_file} has been backed up to {destination_path}")
示例调用
source_dir = "source_folder"
backup_dir = "backup_folder"
backup_files(source_dir, backup_dir)
2、文件分类
你可以根据文件类型将文件分类存放到不同的文件夹中。例如,将所有的图片文件存放到一个文件夹,所有的文档文件存放到另一个文件夹。
import os
import shutil
def classify_files(source_dir, image_dir, doc_dir):
for file_name in os.listdir(source_dir):
source_file = os.path.join(source_dir, file_name)
if file_name.endswith((".jpg", ".png", ".gif")):
destination_path = os.path.join(image_dir, file_name)
elif file_name.endswith((".doc", ".docx", ".pdf")):
destination_path = os.path.join(doc_dir, file_name)
else:
continue
if not os.path.exists(os.path.dirname(destination_path)):
os.makedirs(os.path.dirname(destination_path))
shutil.move(source_file, destination_path)
print(f"{source_file} has been moved to {destination_path}")
示例调用
source_dir = "source_folder"
image_dir = "images"
doc_dir = "documents"
classify_files(source_dir, image_dir, doc_dir)
六、注意事项
在进行文件操作时,有一些需要特别注意的事项:
1、权限问题
在某些操作系统上,文件和目录可能具有权限设置,这会影响你的文件操作。确保你有足够的权限进行文件操作。
2、文件锁定
在某些情况下,文件可能被其他程序占用,导致无法移动或删除。你需要处理这些情况,确保程序的健壮性。
3、错误处理
在进行文件操作时,可能会遇到各种错误。例如,文件不存在、路径错误、权限不足等。使用 try-except 结构来捕获和处理这些错误,确保程序不会因错误而崩溃。
import os
import shutil
def move_file(source_file, destination_dir):
destination_path = os.path.join(destination_dir, os.path.basename(source_file))
if not os.path.exists(destination_dir):
os.makedirs(destination_dir)
try:
shutil.move(source_file, destination_path)
print(f"{source_file} has been moved to {destination_path}")
except FileNotFoundError:
print(f"{source_file} not found")
except PermissionError:
print(f"Permission denied while moving {source_file}")
except Exception as e:
print(f"Error occurred: {e}")
示例调用
source_file = "source.txt"
destination_dir = "destination_folder"
move_file(source_file, destination_dir)
通过本文的详细讲解,你现在应该掌握了如何使用Python将文件放入指定文件夹。无论是简单的文件移动还是复杂的文件分类和备份,这些方法都能帮助你高效地完成任务。在实际应用中,灵活运用这些方法可以极大地提高你的工作效率。
相关问答FAQs:
如何使用Python将文件移动到特定目录?
您可以使用Python的shutil
模块来移动文件。通过shutil.move()
函数,您只需指定源文件路径和目标文件夹路径,即可完成文件的移动。例如:
import shutil
shutil.move('source/file.txt', 'destination/folder/')
确保您拥有目标目录的写入权限。
在Python中,如何创建一个新的文件夹以便存放文件?
在Python中,您可以使用os
模块的os.makedirs()
函数来创建新的文件夹。此函数允许您创建多层目录,如果目录已存在,则不会引发错误。例如:
import os
os.makedirs('new_folder', exist_ok=True)
这将创建一个名为new_folder
的文件夹,若该文件夹已存在则不会做任何操作。
如果目标文件夹不存在,如何在Python中处理文件移动?
在移动文件之前,您可以先检查目标文件夹是否存在。如果不存在,可以使用os.makedirs()
函数创建它。以下是一个示例:
import os
import shutil
destination_folder = 'destination/folder/'
if not os.path.exists(destination_folder):
os.makedirs(destination_folder)
shutil.move('source/file.txt', destination_folder)
这种方法确保了文件在移动之前目标文件夹已经存在,避免了可能的错误。
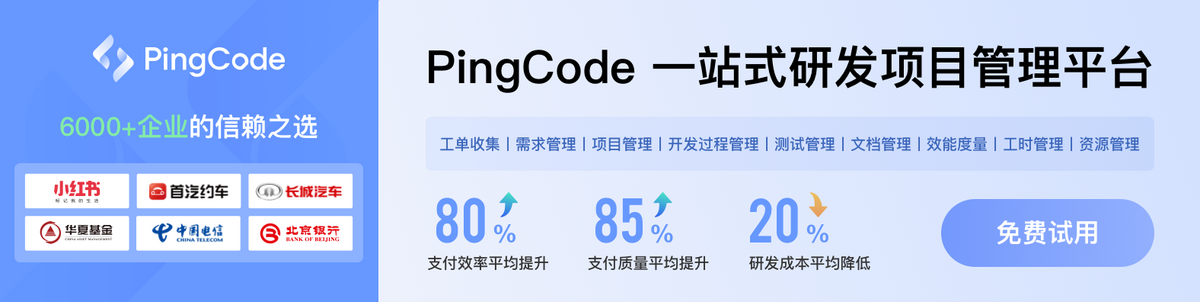