在Python中制作验证码的步骤主要包括以下几步:生成随机字符、绘制图像、添加噪点、保存图像。 其中,生成随机字符 是最核心的一步,因为验证码的主要目的是防止自动化脚本的攻击。我们可以使用Python的Pillow库和随机库来实现这些步骤。下面将详细描述如何实现这些步骤。
一、生成随机字符
为了生成验证码,我们需要生成一些随机字符。常见的验证码字符包括字母和数字。我们可以使用Python的random库来生成这些字符。
import random
import string
def generate_random_text(length=6):
characters = string.ascii_letters + string.digits
random_text = ''.join(random.choice(characters) for _ in range(length))
return random_text
在这个函数中,我们使用string.ascii_letters
和string.digits
来生成一个包含所有字母和数字的字符串,然后使用random.choice
来从这个字符串中随机选择字符,最后将这些字符连接成一个字符串。
二、绘制图像
生成随机字符后,我们需要将这些字符绘制到图像上。我们可以使用Python的Pillow库来实现这一点。
from PIL import Image, ImageDraw, ImageFont
def draw_text_on_image(text):
width, height = 200, 100
image = Image.new('RGB', (width, height), (255, 255, 255))
font = ImageFont.truetype("arial.ttf", 40)
draw = ImageDraw.Draw(image)
text_width, text_height = draw.textsize(text, font=font)
position = ((width - text_width) / 2, (height - text_height) / 2)
draw.text(position, text, font=font, fill=(0, 0, 0))
return image
在这个函数中,我们首先创建一个新的白色图像,然后使用ImageFont.truetype
来加载字体,使用ImageDraw.Draw
来创建一个绘图对象,最后使用draw.text
方法将文本绘制到图像上。
三、添加噪点
为了增加验证码的安全性,我们可以在图像上添加一些噪点。
def add_noise(image):
draw = ImageDraw.Draw(image)
width, height = image.size
for _ in range(100):
x = random.randint(0, width)
y = random.randint(0, height)
draw.point((x, y), fill=(0, 0, 0))
return image
在这个函数中,我们使用draw.point
方法在图像上随机绘制一些黑点。
四、保存图像
最后,我们需要将生成的图像保存到文件中。
def save_image(image, file_path):
image.save(file_path)
完整示例代码如下:
import random
import string
from PIL import Image, ImageDraw, ImageFont
def generate_random_text(length=6):
characters = string.ascii_letters + string.digits
random_text = ''.join(random.choice(characters) for _ in range(length))
return random_text
def draw_text_on_image(text):
width, height = 200, 100
image = Image.new('RGB', (width, height), (255, 255, 255))
font = ImageFont.truetype("arial.ttf", 40)
draw = ImageDraw.Draw(image)
text_width, text_height = draw.textsize(text, font=font)
position = ((width - text_width) / 2, (height - text_height) / 2)
draw.text(position, text, font=font, fill=(0, 0, 0))
return image
def add_noise(image):
draw = ImageDraw.Draw(image)
width, height = image.size
for _ in range(100):
x = random.randint(0, width)
y = random.randint(0, height)
draw.point((x, y), fill=(0, 0, 0))
return image
def save_image(image, file_path):
image.save(file_path)
if __name__ == '__main__':
text = generate_random_text()
image = draw_text_on_image(text)
image = add_noise(image)
save_image(image, 'captcha.png')
通过运行上述代码,我们可以生成一个包含随机字符并带有噪点的验证码图像。
相关问答FAQs:
如何用Python生成简单的验证码图像?
要用Python生成验证码图像,可以使用Pillow
库来创建图像,random
库生成随机字符串。首先,安装Pillow库:pip install Pillow
。接着,生成一个包含随机字符的字符串,并使用Pillow将其绘制到图像上。可以添加干扰线和噪点以增强验证码的安全性。
有哪些Python库可以帮助生成验证码?
Python中有几个库专门用于生成验证码,包括captcha
和Pillow
。captcha
库提供了多种验证码类型,如字母、数字和数学题,使用起来非常方便。Pillow
则更灵活,适合自定义复杂的验证码图案。
验证码生成后,如何验证用户输入的验证码?
在生成验证码的过程中,可以将生成的验证码存储在会话中(session)或数据库中。当用户输入验证码时,将其与存储的验证码进行比较。如果匹配,则验证通过;否则,提示用户重新输入。确保在验证过程中注意大小写和字符格式的一致性。
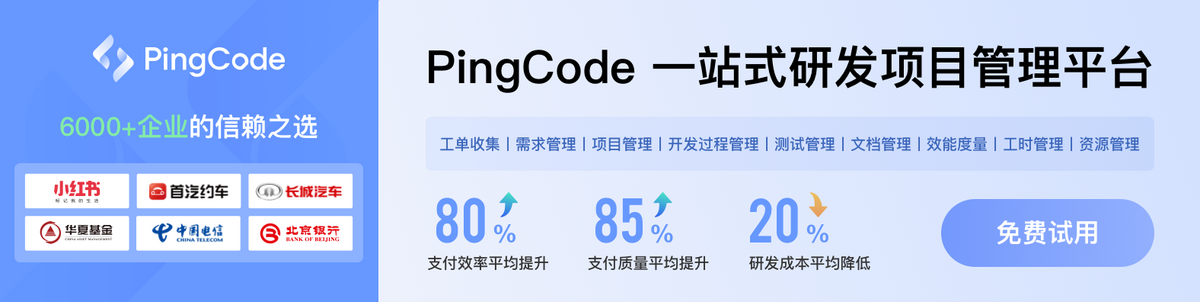