Python如何从txt按行搜索关键字
Python能够通过读取文件内容、逐行检查、匹配关键字等方式实现按行搜索关键字,具体步骤包括打开文件、逐行读取、使用正则表达式进行匹配等。本文将深入探讨这些步骤,并提供详细的代码示例。
文件操作、正则表达式、逐行读取是Python中按行搜索关键字的核心步骤。让我们详细讨论其中的文件操作。
文件操作
文件操作是指在Python中如何打开、读取、写入和关闭文件。Python提供了内置的函数来处理这些操作,使得与文件的交互变得非常简便。以下是文件操作的基本步骤:
- 打开文件:使用
open()
函数打开文件,并指定模式(如读取模式'r'
)。 - 读取文件内容:使用
read()
,readlines()
或readline()
函数逐行或整体读取文件内容。 - 处理文件内容:对读取的内容进行处理,如逐行搜索关键字。
- 关闭文件:使用
close()
函数关闭文件,释放资源。
示例代码
# 打开文件并读取内容
with open('example.txt', 'r') as file:
lines = file.readlines()
打印文件内容
for line in lines:
print(line.strip())
一、读取文件
在进行任何文件操作之前,首先需要读取文件内容。Python的open()
函数可以实现这一点。open()
函数的第一个参数是文件路径,第二个参数是模式(如读取模式'r'
)。
示例代码
# 打开文件
file = open('example.txt', 'r')
读取文件内容
content = file.readlines()
关闭文件
file.close()
二、逐行读取
在读取文件内容后,可以使用逐行读取的方式来处理每一行。逐行读取有助于逐个检查每一行是否包含所需的关键字。可以使用for
循环来遍历每一行。
示例代码
# 打开文件
with open('example.txt', 'r') as file:
for line in file:
print(line.strip())
三、使用正则表达式
正则表达式是一种强大的工具,可以帮助我们匹配复杂的字符串模式。在Python中,re
模块提供了对正则表达式的支持。通过使用正则表达式,可以更灵活地搜索关键字。
示例代码
import re
定义关键字
keyword = 'Python'
打开文件
with open('example.txt', 'r') as file:
for line in file:
if re.search(keyword, line):
print(f'Found "{keyword}" in line: {line.strip()}')
四、综合示例
以下是一个综合示例,演示了如何将上述步骤结合起来,以实现从txt文件中按行搜索关键字的功能。
示例代码
import re
def search_keyword_in_file(file_path, keyword):
results = []
# 打开文件
with open(file_path, 'r') as file:
for line_num, line in enumerate(file, start=1):
if re.search(keyword, line):
results.append((line_num, line.strip()))
return results
定义文件路径和关键字
file_path = 'example.txt'
keyword = 'Python'
搜索关键字
matches = search_keyword_in_file(file_path, keyword)
打印结果
if matches:
print(f'Found "{keyword}" in the following lines:')
for line_num, line in matches:
print(f'Line {line_num}: {line}')
else:
print(f'No matches found for "{keyword}".')
五、处理大文件
对于大文件,逐行读取是一种高效的处理方式,因为它可以避免一次性加载整个文件到内存中,从而节省内存资源。在处理大文件时,使用with open()
上下文管理器和逐行读取是推荐的做法。
示例代码
import re
def search_keyword_in_large_file(file_path, keyword):
results = []
# 打开文件
with open(file_path, 'r') as file:
for line_num, line in enumerate(file, start=1):
if re.search(keyword, line):
results.append((line_num, line.strip()))
return results
定义文件路径和关键字
file_path = 'large_example.txt'
keyword = 'Python'
搜索关键字
matches = search_keyword_in_large_file(file_path, keyword)
打印结果
if matches:
print(f'Found "{keyword}" in the following lines:')
for line_num, line in matches:
print(f'Line {line_num}: {line}')
else:
print(f'No matches found for "{keyword}".')
六、处理多关键字
有时我们可能需要搜索多个关键字。可以将多个关键字存储在一个列表中,并使用正则表达式来匹配任何一个关键字。
示例代码
import re
def search_multiple_keywords_in_file(file_path, keywords):
results = []
# 创建正则表达式模式
pattern = '|'.join(keywords)
# 打开文件
with open(file_path, 'r') as file:
for line_num, line in enumerate(file, start=1):
if re.search(pattern, line):
results.append((line_num, line.strip()))
return results
定义文件路径和关键字列表
file_path = 'example.txt'
keywords = ['Python', 'Java', 'C++']
搜索关键字
matches = search_multiple_keywords_in_file(file_path, keywords)
打印结果
if matches:
print(f'Found keywords in the following lines:')
for line_num, line in matches:
print(f'Line {line_num}: {line}')
else:
print(f'No matches found for keywords.')
七、忽略大小写
在某些情况下,我们可能需要忽略关键字的大小写。可以在正则表达式中使用re.IGNORECASE
标志来实现这一点。
示例代码
import re
def search_keyword_ignore_case(file_path, keyword):
results = []
# 打开文件
with open(file_path, 'r') as file:
for line_num, line in enumerate(file, start=1):
if re.search(keyword, line, re.IGNORECASE):
results.append((line_num, line.strip()))
return results
定义文件路径和关键字
file_path = 'example.txt'
keyword = 'python'
搜索关键字
matches = search_keyword_ignore_case(file_path, keyword)
打印结果
if matches:
print(f'Found "{keyword}" (case insensitive) in the following lines:')
for line_num, line in matches:
print(f'Line {line_num}: {line}')
else:
print(f'No matches found for "{keyword}".')
八、使用生成器处理文件
生成器是一种惰性求值的机制,可以在需要时生成数据,而不是一次性加载所有数据。这在处理大文件时非常有用。通过使用生成器,可以更高效地逐行处理文件内容。
示例代码
import re
def search_keyword_with_generator(file_path, keyword):
def line_generator(file_path):
with open(file_path, 'r') as file:
for line in file:
yield line
results = []
# 使用生成器逐行读取文件
for line_num, line in enumerate(line_generator(file_path), start=1):
if re.search(keyword, line):
results.append((line_num, line.strip()))
return results
定义文件路径和关键字
file_path = 'example.txt'
keyword = 'Python'
搜索关键字
matches = search_keyword_with_generator(file_path, keyword)
打印结果
if matches:
print(f'Found "{keyword}" in the following lines:')
for line_num, line in matches:
print(f'Line {line_num}: {line}')
else:
print(f'No matches found for "{keyword}".')
九、总结
通过以上步骤,我们可以看到Python提供了丰富的工具和方法来从txt文件中按行搜索关键字。文件操作、正则表达式、逐行读取是实现这一功能的核心步骤。无论是处理小文件还是大文件,Python都能高效地完成任务。同时,通过使用生成器和正则表达式,可以进一步提高代码的效率和灵活性。
希望本文能够帮助你更好地理解和掌握如何使用Python从txt文件中按行搜索关键字。如果你有任何问题或建议,欢迎在评论区留言。
相关问答FAQs:
如何在Python中打开并读取一个txt文件?
在Python中,可以使用内置的open()
函数来打开一个txt文件,并通过readlines()
方法按行读取文件内容。示例如下:
with open('file.txt', 'r') as file:
lines = file.readlines()
这样就可以将文件中的每一行存储到一个列表中,便于后续的关键字搜索。
如何在读取的行中搜索特定的关键字?
可以使用Python的字符串方法in
来检查关键字是否存在于每一行中。以下是一个简单的示例:
keyword = '搜索的关键字'
for line in lines:
if keyword in line:
print(line)
这种方式能够快速找到并打印出包含关键字的所有行。
是否可以在搜索时忽略大小写?
当然可以!在进行关键字搜索时,可以将行和关键字都转换为同一种大小写格式,比如使用lower()
方法。这样可以确保搜索不受大小写影响。示例如下:
keyword = '搜索的关键字'.lower()
for line in lines:
if keyword in line.lower():
print(line)
这样的处理使得搜索更加灵活,用户无论输入何种形式的关键字,都能得到正确的结果。
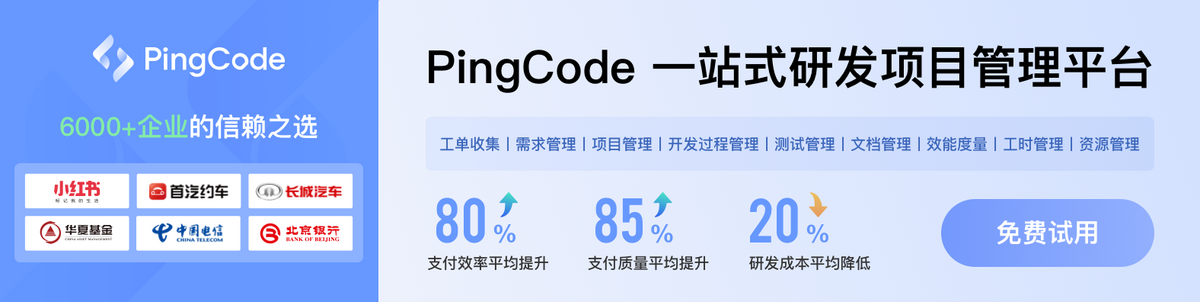