如何用Python制作一个电子相册
Python是一种功能强大且灵活的编程语言,适用于各种任务,包括创建电子相册。通过使用Python的各种库,如Pillow(用于图像处理)、Tkinter(用于GUI创建)、以及OS和shutil(用于文件操作)等,可以轻松创建一个功能丰富的电子相册。本文将详细介绍如何利用这些库和工具来制作一个电子相册,涵盖从图像处理、用户界面设计到文件管理的各个方面。
一、准备工作
在开始之前,我们需要确保安装了所需的Python库。你可以使用以下命令来安装这些库:
pip install pillow tk
这些库将帮助我们处理图像、创建图形用户界面以及管理文件。
二、图像处理
图像处理是创建电子相册的关键部分。Pillow库提供了丰富的图像处理功能,包括图像加载、保存、大小调整、旋转等。
1、加载与显示图像
要加载和显示图像,我们可以使用Pillow库的基本功能:
from PIL import Image, ImageTk
import tkinter as tk
def display_image(image_path):
root = tk.Tk()
img = Image.open(image_path)
img = ImageTk.PhotoImage(img)
panel = tk.Label(root, image=img)
panel.pack(side="bottom", fill="both", expand="yes")
root.mainloop()
display_image("path/to/your/image.jpg")
上述代码将加载并显示指定路径的图像。
2、调整图像大小
我们可以使用Pillow库来调整图像大小,以适应我们的电子相册布局:
from PIL import Image
def resize_image(image_path, output_path, size):
img = Image.open(image_path)
img = img.resize(size, Image.ANTIALIAS)
img.save(output_path)
resize_image("path/to/your/image.jpg", "path/to/your/resized_image.jpg", (800, 600))
三、创建用户界面
一个好的电子相册需要一个友好的用户界面。我们可以使用Tkinter库来创建图形用户界面。
1、基本窗口
首先,我们创建一个基本的窗口:
import tkinter as tk
root = tk.Tk()
root.title("电子相册")
root.geometry("800x600")
root.mainloop()
2、添加图像显示区域
接下来,我们添加一个区域来显示图像:
from PIL import Image, ImageTk
import tkinter as tk
class PhotoAlbum:
def __init__(self, master):
self.master = master
self.master.title("电子相册")
self.master.geometry("800x600")
self.image_label = tk.Label(self.master)
self.image_label.pack(side="top", fill="both", expand="yes")
def display_image(self, image_path):
img = Image.open(image_path)
img = ImageTk.PhotoImage(img)
self.image_label.config(image=img)
self.image_label.image = img
root = tk.Tk()
album = PhotoAlbum(root)
album.display_image("path/to/your/image.jpg")
root.mainloop()
四、文件管理
为了方便用户浏览和管理图像,我们需要实现一些基本的文件管理功能。
1、加载图像文件
我们可以使用Tkinter的文件对话框来选择图像文件:
from tkinter import filedialog
def load_image():
file_path = filedialog.askopenfilename(filetypes=[("Image files", "*.jpg;*.jpeg;*.png")])
if file_path:
album.display_image(file_path)
load_button = tk.Button(root, text="加载图像", command=load_image)
load_button.pack(side="bottom", fill="both", expand="yes")
2、浏览图像
为了实现图像的浏览功能,我们需要存储图像文件的路径,并实现前后浏览的功能:
class PhotoAlbum:
def __init__(self, master):
self.master = master
self.master.title("电子相册")
self.master.geometry("800x600")
self.image_label = tk.Label(self.master)
self.image_label.pack(side="top", fill="both", expand="yes")
self.image_paths = []
self.current_image_index = -1
def load_images(self, directory):
self.image_paths = [os.path.join(directory, f) for f in os.listdir(directory) if f.endswith(('jpg', 'jpeg', 'png'))]
self.current_image_index = 0
self.display_image(self.image_paths[self.current_image_index])
def display_image(self, image_path):
img = Image.open(image_path)
img = ImageTk.PhotoImage(img)
self.image_label.config(image=img)
self.image_label.image = img
def next_image(self):
if self.current_image_index < len(self.image_paths) - 1:
self.current_image_index += 1
self.display_image(self.image_paths[self.current_image_index])
def previous_image(self):
if self.current_image_index > 0:
self.current_image_index -= 1
self.display_image(self.image_paths[self.current_image_index])
root = tk.Tk()
album = PhotoAlbum(root)
def load_images():
directory = filedialog.askdirectory()
if directory:
album.load_images(directory)
load_button = tk.Button(root, text="加载图像文件夹", command=load_images)
load_button.pack(side="bottom", fill="both", expand="yes")
next_button = tk.Button(root, text="下一张", command=album.next_image)
next_button.pack(side="right", fill="both", expand="yes")
prev_button = tk.Button(root, text="上一张", command=album.previous_image)
prev_button.pack(side="left", fill="both", expand="yes")
root.mainloop()
五、添加更多功能
为了让电子相册更加实用和有趣,我们可以添加一些额外的功能,例如幻灯片播放、图像滤镜、以及图像排序等。
1、幻灯片播放
我们可以使用Tkinter的after方法来实现幻灯片播放功能:
class PhotoAlbum:
def __init__(self, master):
self.master = master
self.master.title("电子相册")
self.master.geometry("800x600")
self.image_label = tk.Label(self.master)
self.image_label.pack(side="top", fill="both", expand="yes")
self.image_paths = []
self.current_image_index = -1
self.slideshow_running = False
def load_images(self, directory):
self.image_paths = [os.path.join(directory, f) for f in os.listdir(directory) if f.endswith(('jpg', 'jpeg', 'png'))]
self.current_image_index = 0
self.display_image(self.image_paths[self.current_image_index])
def display_image(self, image_path):
img = Image.open(image_path)
img = ImageTk.PhotoImage(img)
self.image_label.config(image=img)
self.image_label.image = img
def next_image(self):
if self.current_image_index < len(self.image_paths) - 1:
self.current_image_index += 1
self.display_image(self.image_paths[self.current_image_index])
def previous_image(self):
if self.current_image_index > 0:
self.current_image_index -= 1
self.display_image(self.image_paths[self.current_image_index])
def start_slideshow(self):
self.slideshow_running = True
self.slideshow()
def stop_slideshow(self):
self.slideshow_running = False
def slideshow(self):
if self.slideshow_running:
self.next_image()
self.master.after(3000, self.slideshow) # Change image every 3 seconds
root = tk.Tk()
album = PhotoAlbum(root)
def load_images():
directory = filedialog.askdirectory()
if directory:
album.load_images(directory)
load_button = tk.Button(root, text="加载图像文件夹", command=load_images)
load_button.pack(side="bottom", fill="both", expand="yes")
next_button = tk.Button(root, text="下一张", command=album.next_image)
next_button.pack(side="right", fill="both", expand="yes")
prev_button = tk.Button(root, text="上一张", command=album.previous_image)
prev_button.pack(side="left", fill="both", expand="yes")
start_slideshow_button = tk.Button(root, text="开始幻灯片", command=album.start_slideshow)
start_slideshow_button.pack(side="top", fill="both", expand="yes")
stop_slideshow_button = tk.Button(root, text="停止幻灯片", command=album.stop_slideshow)
stop_slideshow_button.pack(side="top", fill="both", expand="yes")
root.mainloop()
2、图像滤镜
我们可以使用Pillow库来应用一些基本的图像滤镜:
from PIL import ImageFilter
def apply_filter(image_path, filter_type):
img = Image.open(image_path)
if filter_type == "BLUR":
img = img.filter(ImageFilter.BLUR)
elif filter_type == "CONTOUR":
img = img.filter(ImageFilter.CONTOUR)
elif filter_type == "DETAIL":
img = img.filter(ImageFilter.DETAIL)
img.show()
apply_filter("path/to/your/image.jpg", "BLUR")
我们可以将这些功能集成到我们的电子相册中,以便用户可以应用各种滤镜。
六、总结
通过上述步骤,我们已经创建了一个功能丰富的电子相册。Python的多样化库和工具使得这一过程变得相对简单和高效。我们可以进一步扩展这个项目,添加更多高级功能,例如云存储集成、社交分享功能等,以提升用户体验。希望这篇文章能为你提供有用的指导,帮助你创建自己的电子相册。
相关问答FAQs:
如何用Python制作电子相册需要哪些基本知识?
制作电子相册通常需要掌握Python的基本语法,了解如何使用图形用户界面(GUI)库,例如Tkinter或PyQt。此外,熟悉图像处理库如Pillow,以及文件操作和数据结构的使用也是非常重要的。这些知识将帮助你更好地设计和实现电子相册的功能。
使用Python制作电子相册有哪些推荐的库或工具?
为了制作电子相册,推荐使用以下库:Pillow(用于图像处理),Tkinter或PyQt(用于创建用户界面),以及OpenCV(用于更高级的图像处理)。这些库能够帮助你实现图像的加载、显示、编辑和保存等功能,提升电子相册的交互性和美观性。
制作电子相册时,如何处理图片的格式和质量?
在制作电子相册时,选择合适的图片格式非常重要。常见的格式有JPEG、PNG和GIF。JPEG适合存储照片,PNG适合需要透明背景的图片,而GIF则适用于简单动画。在处理图片质量时,可以使用Pillow库调整图片的分辨率和压缩率,以确保在保持良好质量的同时,优化文件大小,提升加载速度。
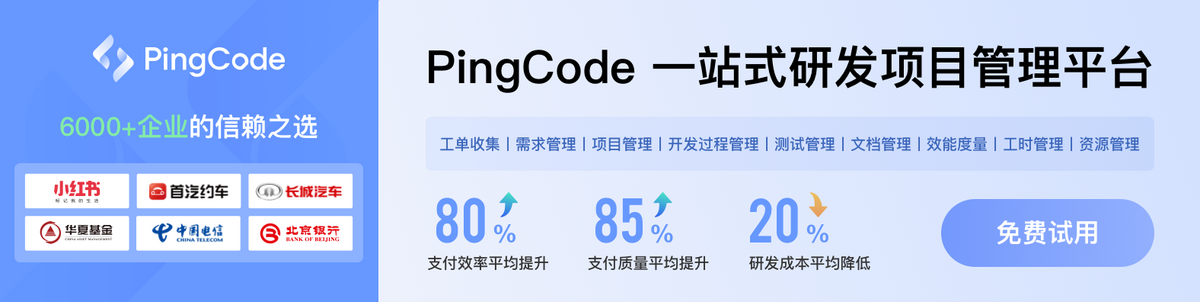