在Python中,替换文件中的字符串可以通过以下步骤进行:读取文件内容、进行字符串替换、将修改后的内容写回文件。最常用的方法包括使用内置的字符串方法、正则表达式库以及文件操作函数。以下是详细步骤:读取文件内容、字符串替换、写回文件。
一、读取文件内容
首先,需要读取文件的内容到内存中。Python 提供了多种读取文件的方法,最常用的是使用 open()
函数。
with open('example.txt', 'r') as file:
data = file.read()
上面的代码使用 with
语句打开文件,这样可以确保文件在操作完成后会自动关闭。'r'
模式表示文件以只读模式打开,file.read()
将文件的全部内容读取到一个字符串中。
二、字符串替换
读取文件内容后,可以使用字符串的 replace()
方法进行替换。
data = data.replace('old_string', 'new_string')
replace()
方法会将所有匹配的字符串替换为新的字符串。
三、写回文件
替换完成后,需要将修改后的内容写回文件。为了确保文件内容被正确覆盖,通常使用写模式 'w'
。
with open('example.txt', 'w') as file:
file.write(data)
这样,修改后的内容就会写回到同一个文件中。
具体实现
下面是一个完整的例子,展示如何在Python中替换文件中的字符串:
def replace_string_in_file(file_path, old_string, new_string):
# 读取文件内容
with open(file_path, 'r') as file:
data = file.read()
# 替换字符串
new_data = data.replace(old_string, new_string)
# 写回文件
with open(file_path, 'w') as file:
file.write(new_data)
调用函数
replace_string_in_file('example.txt', 'old_string', 'new_string')
正则表达式替换
有时候,字符串替换需要更复杂的模式匹配,这时可以使用 re
模块。
import re
def replace_string_in_file_with_regex(file_path, pattern, replacement):
# 读取文件内容
with open(file_path, 'r') as file:
data = file.read()
# 使用正则表达式替换字符串
new_data = re.sub(pattern, replacement, data)
# 写回文件
with open(file_path, 'w') as file:
file.write(new_data)
调用函数
replace_string_in_file_with_regex('example.txt', r'old_pattern', 'new_string')
在这个例子中,re.sub()
函数用于将匹配的模式替换为新的字符串。
替换多行文本
如果需要替换的内容跨越多行,可以使用多行匹配模式。
import re
def replace_multiline_string_in_file(file_path, old_multiline_string, new_multiline_string):
# 读取文件内容
with open(file_path, 'r') as file:
data = file.read()
# 使用正则表达式替换多行字符串
new_data = re.sub(old_multiline_string, new_multiline_string, data, flags=re.DOTALL)
# 写回文件
with open(file_path, 'w') as file:
file.write(new_data)
多行字符串
old_multiline_string = r'''old
multiline
string'''
new_multiline_string = '''new
multiline
string'''
调用函数
replace_multiline_string_in_file('example.txt', old_multiline_string, new_multiline_string)
在这个例子中,re.DOTALL
标志使得 .
可以匹配换行符,从而实现多行匹配替换。
四、处理大文件
当文件非常大时,读取整个文件到内存中可能会导致内存不足。此时,可以逐行读取和处理文件。
def replace_string_in_large_file(file_path, old_string, new_string):
# 创建一个临时文件
temp_file_path = file_path + '.tmp'
with open(file_path, 'r') as file, open(temp_file_path, 'w') as temp_file:
for line in file:
# 替换每一行中的字符串
new_line = line.replace(old_string, new_string)
temp_file.write(new_line)
# 替换原文件
import os
os.remove(file_path)
os.rename(temp_file_path, file_path)
调用函数
replace_string_in_large_file('example.txt', 'old_string', 'new_string')
在这个例子中,逐行读取文件并将替换后的内容写入一个临时文件,最后将临时文件重命名为原文件名。
五、备份文件
为了防止意外情况导致数据丢失,可以在进行替换操作之前备份原文件。
import shutil
def replace_string_in_file_with_backup(file_path, old_string, new_string):
# 备份原文件
backup_file_path = file_path + '.bak'
shutil.copy(file_path, backup_file_path)
# 读取文件内容
with open(file_path, 'r') as file:
data = file.read()
# 替换字符串
new_data = data.replace(old_string, new_string)
# 写回文件
with open(file_path, 'w') as file:
file.write(new_data)
调用函数
replace_string_in_file_with_backup('example.txt', 'old_string', 'new_string')
这个例子中,使用 shutil.copy
函数创建原文件的备份,以防替换操作出现问题时可以恢复原文件。
六、总结
通过上述几个小节,我们可以看到,在Python中替换文件中的字符串可以通过多种方法实现,包括简单的字符串替换、正则表达式、多行文本替换、大文件处理和备份文件。选择合适的方法可以根据具体的应用场景和需求来决定。
核心步骤包括读取文件内容、进行字符串替换、将修改后的内容写回文件。 无论是处理小文件还是大文件,都需要考虑文件的读写效率和数据安全性。希望这篇文章能为您在Python中进行文件字符串替换提供有用的指导。
相关问答FAQs:
如何使用Python替换文件中的特定字符串?
可以使用Python的内置函数打开文件并读取其内容。通过str.replace()
方法替换指定的字符串,然后将修改后的内容写回文件。示例代码如下:
with open('文件名.txt', 'r', encoding='utf-8') as file:
data = file.read()
data = data.replace('旧字符串', '新字符串')
with open('文件名.txt', 'w', encoding='utf-8') as file:
file.write(data)
在替换字符串时,如何确保不会影响到其他相似的字符串?
可以使用正则表达式的re
模块来进行更精确的字符串匹配。通过定义模式,可以更好地控制替换过程。例如,使用re.sub()
方法可以只替换特定条件下的字符串。
import re
with open('文件名.txt', 'r', encoding='utf-8') as file:
data = file.read()
data = re.sub(r'\b旧字符串\b', '新字符串', data)
with open('文件名.txt', 'w', encoding='utf-8') as file:
file.write(data)
在替换字符串后,如何验证文件的内容是否已被正确更新?
可以在文件写入后,重新打开文件并读取其内容进行验证。通过简单的打印或比较操作,可以确认替换是否成功。
with open('文件名.txt', 'r', encoding='utf-8') as file:
updated_data = file.read()
print(updated_data) # 检查替换后的内容
这些方法能帮助用户有效地在文件中替换字符串,并确保结果符合预期。
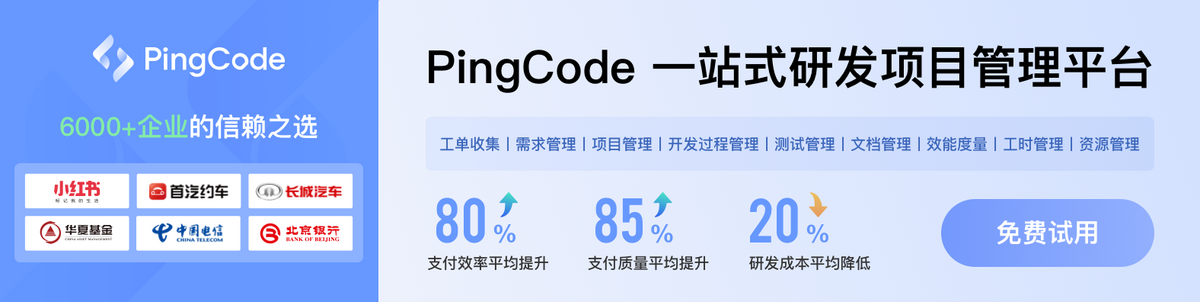