Python判断一个数是否存在的方法包括使用if语句、in运算符、集合等。常见的方式有:使用列表和集合的in运算符、使用字典的键判断、使用条件判断语句。本文将详细介绍这几种方法,并举例说明如何在不同情况下使用这些方法。
一、列表和集合的in运算符
列表的in运算符
在Python中,列表是一种有序的集合,可以包含重复的元素。要判断一个数是否存在于列表中,可以使用in运算符。in运算符的时间复杂度是O(n),因为它需要遍历整个列表来查找元素。
my_list = [1, 2, 3, 4, 5]
number_to_check = 3
if number_to_check in my_list:
print(f"{number_to_check} exists in the list.")
else:
print(f"{number_to_check} does not exist in the list.")
集合的in运算符
集合(set)是一种无序且不重复的元素集合。由于集合底层实现是哈希表,使用in运算符查找元素的时间复杂度为O(1),比列表高效得多。
my_set = {1, 2, 3, 4, 5}
number_to_check = 3
if number_to_check in my_set:
print(f"{number_to_check} exists in the set.")
else:
print(f"{number_to_check} does not exist in the set.")
二、字典的键判断
字典(dictionary)是另一种常用的Python数据结构,它以键值对的形式存储数据。要判断一个数是否存在于字典中,可以检查它是否是字典的键。字典的键查找时间复杂度同样为O(1)。
my_dict = {1: 'a', 2: 'b', 3: 'c', 4: 'd'}
number_to_check = 3
if number_to_check in my_dict:
print(f"{number_to_check} exists as a key in the dictionary.")
else:
print(f"{number_to_check} does not exist as a key in the dictionary.")
三、条件判断语句
除了上述方法,还可以使用条件判断语句来判断一个数是否存在。虽然这种方法比较繁琐,但在某些特定情况下可能会用到。
number_to_check = 3
found = False
for number in my_list:
if number == number_to_check:
found = True
break
if found:
print(f"{number_to_check} exists in the list.")
else:
print(f"{number_to_check} does not exist in the list.")
四、使用函数封装判断逻辑
为了代码的复用性和可读性,可以将判断逻辑封装在函数中。
判断列表
def is_number_in_list(number, lst):
return number in lst
my_list = [1, 2, 3, 4, 5]
number_to_check = 3
if is_number_in_list(number_to_check, my_list):
print(f"{number_to_check} exists in the list.")
else:
print(f"{number_to_check} does not exist in the list.")
判断集合
def is_number_in_set(number, st):
return number in st
my_set = {1, 2, 3, 4, 5}
number_to_check = 3
if is_number_in_set(number_to_check, my_set):
print(f"{number_to_check} exists in the set.")
else:
print(f"{number_to_check} does not exist in the set.")
判断字典键
def is_key_in_dict(key, dct):
return key in dct
my_dict = {1: 'a', 2: 'b', 3: 'c', 4: 'd'}
number_to_check = 3
if is_key_in_dict(number_to_check, my_dict):
print(f"{number_to_check} exists as a key in the dictionary.")
else:
print(f"{number_to_check} does not exist as a key in the dictionary.")
五、使用异常处理
在某些特殊情况下,我们可以通过异常处理来判断一个数是否存在。这种方法一般不推荐,除非在特定场景下有必要。
my_list = [1, 2, 3, 4, 5]
number_to_check = 3
try:
index = my_list.index(number_to_check)
print(f"{number_to_check} exists in the list at index {index}.")
except ValueError:
print(f"{number_to_check} does not exist in the list.")
六、综合应用
在实际项目中,往往需要综合应用多种方法来判断一个数是否存在。例如,我们可能需要先判断一个数是否在一个列表中,然后再根据结果进行进一步操作。
def process_number(number, lst, st, dct):
if number in lst:
print(f"{number} exists in the list.")
else:
print(f"{number} does not exist in the list.")
if number in st:
print(f"{number} exists in the set.")
else:
print(f"{number} does not exist in the set.")
if number in dct:
print(f"{number} exists as a key in the dictionary.")
else:
print(f"{number} does not exist as a key in the dictionary.")
my_list = [1, 2, 3, 4, 5]
my_set = {1, 2, 3, 4, 5}
my_dict = {1: 'a', 2: 'b', 3: 'c', 4: 'd'}
number_to_check = 3
process_number(number_to_check, my_list, my_set, my_dict)
七、性能比较
当数据量较大时,选择合适的数据结构和方法可以显著提高性能。一般来说,集合和字典的查找效率高于列表。以下是一个简单的性能比较示例:
import time
large_list = list(range(1000000))
large_set = set(large_list)
large_dict = {i: None for i in large_list}
number_to_check = 999999
列表查找
start_time = time.time()
number_to_check in large_list
end_time = time.time()
print(f"List lookup took {end_time - start_time:.6f} seconds.")
集合查找
start_time = time.time()
number_to_check in large_set
end_time = time.time()
print(f"Set lookup took {end_time - start_time:.6f} seconds.")
字典查找
start_time = time.time()
number_to_check in large_dict
end_time = time.time()
print(f"Dictionary lookup took {end_time - start_time:.6f} seconds.")
在这个示例中,集合和字典的查找时间明显优于列表,尤其是在数据量很大的情况下。
八、总结
Python提供了多种方法来判断一个数是否存在于某个数据结构中。列表适用于有序且可能有重复元素的情况;集合适用于需要快速查找且元素唯一的情况;字典适用于需要快速查找键值对的情况。选择合适的方法和数据结构,可以有效提高代码的性能和可读性。
相关问答FAQs:
如何在Python中检查一个数字是否在列表中存在?
在Python中,可以使用in
关键字来判断一个数字是否存在于列表中。例如,如果你有一个列表numbers = [1, 2, 3, 4, 5]
,你可以使用表达式3 in numbers
来检查数字3是否在该列表中。如果存在,它将返回True
,否则返回False
。
使用Python中的函数来判断数字是否存在的最佳实践是什么?
编写一个函数来检查数字的存在性是一种良好的编程习惯。可以定义一个简单的函数,如下所示:
def is_number_in_list(number, num_list):
return number in num_list
调用这个函数时,你只需传入要检查的数字和列表。例如,is_number_in_list(3, [1, 2, 3, 4, 5])
将返回True
。
在Python中如何优化数字存在性检查的性能?
如果你需要频繁检查数字的存在性,考虑使用集合(set)来存储数字,因为集合的查找时间复杂度为O(1)。例如:
numbers_set = {1, 2, 3, 4, 5}
exists = 3 in numbers_set
这种方法比在列表中查找更高效,特别是在处理大量数据时。
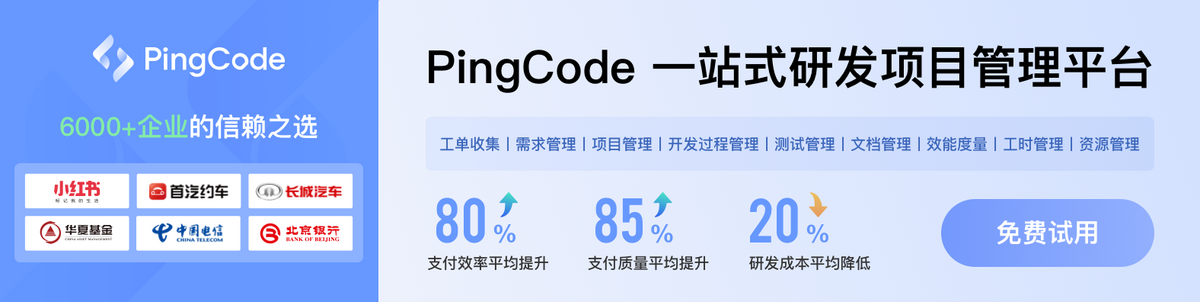