如何用Python写入HTML文件数据库
使用Python写入HTML文件数据库,主要步骤包括:读取数据、生成HTML内容、写入文件、确保数据格式正确。下面将详细描述如何实现这些步骤,并深入探讨每一步的具体实现方法。
一、读取数据
读取数据是生成HTML文件的基础。数据可以来自多种来源,例如CSV文件、JSON文件、数据库等。读取数据的方式决定了后续操作的便利性和效率。这里以读取CSV文件为例,展示如何使用Python的pandas库读取数据。
import pandas as pd
读取CSV文件
data = pd.read_csv('data.csv')
读取数据后,我们需要对数据进行处理,以便后续生成HTML文件时能够使用。
二、生成HTML内容
生成HTML内容是关键步骤。我们需要将读取到的数据转换为HTML格式,并确保生成的HTML内容符合要求。可以使用Python的内置库html
来生成HTML内容,或者使用pandas
的to_html
方法直接将DataFrame转换为HTML表格。
# 将DataFrame转换为HTML表格
html_content = data.to_html()
此外,我们还可以手动生成HTML内容,以便对HTML结构和样式进行更细致的控制。
html_content = """
<!DOCTYPE html>
<html>
<head>
<title>Data</title>
</head>
<body>
<table border="1">
<tr>
<th>Column1</th>
<th>Column2</th>
</tr>
"""
for index, row in data.iterrows():
html_content += f"""
<tr>
<td>{row['Column1']}</td>
<td>{row['Column2']}</td>
</tr>
"""
html_content += """
</table>
</body>
</html>
"""
三、写入文件
生成HTML内容后,我们需要将其写入文件。可以使用Python的内置函数open
来创建并写入文件。
with open('output.html', 'w') as file:
file.write(html_content)
这一步相对简单,但需要确保文件路径和文件名正确,以免覆盖已有文件或写入失败。
四、确保数据格式正确
在生成和写入HTML内容时,确保数据格式正确是至关重要的。HTML文件需要符合HTML标准,数据内容需要正确转换为HTML实体。此外,特殊字符(如&、<、>等)需要正确转义,以防止HTML解析错误。
使用html
库的escape
方法可以帮助我们转义特殊字符。
import html
html_content = html.escape(data.to_html())
五、优化和美化HTML文件
生成基础的HTML文件后,我们可以进一步优化和美化HTML内容,以提升用户体验和可读性。可以使用CSS样式表、JavaScript脚本等方法对HTML文件进行美化。
<!DOCTYPE html>
<html>
<head>
<title>Data</title>
<style>
table {
width: 100%;
border-collapse: collapse;
}
table, th, td {
border: 1px solid black;
}
th, td {
padding: 15px;
text-align: left;
}
tr:nth-child(even) {
background-color: #f2f2f2;
}
</style>
</head>
<body>
<table>
<tr>
<th>Column1</th>
<th>Column2</th>
</tr>
<!-- Data rows will be inserted here -->
</table>
</body>
</html>
在生成HTML内容时,将数据行插入到预定义的表格结构中。
html_content = """
<!DOCTYPE html>
<html>
<head>
<title>Data</title>
<style>
table {
width: 100%;
border-collapse: collapse;
}
table, th, td {
border: 1px solid black;
}
th, td {
padding: 15px;
text-align: left;
}
tr:nth-child(even) {
background-color: #f2f2f2;
}
</style>
</head>
<body>
<table>
<tr>
<th>Column1</th>
<th>Column2</th>
</tr>
"""
for index, row in data.iterrows():
html_content += f"""
<tr>
<td>{html.escape(str(row['Column1']))}</td>
<td>{html.escape(str(row['Column2']))}</td>
</tr>
"""
html_content += """
</table>
</body>
</html>
"""
with open('output.html', 'w') as file:
file.write(html_content)
六、自动化和扩展
在实际应用中,我们通常需要处理大量数据,生成多个HTML文件,或定期更新HTML文件。这时,可以通过脚本自动化和扩展功能提高效率。
可以使用Python的调度库schedule
实现定期执行脚本。
import schedule
import time
def job():
# 读取数据
data = pd.read_csv('data.csv')
# 生成HTML内容
html_content = """
<!DOCTYPE html>
<html>
<head>
<title>Data</title>
<style>
table {
width: 100%;
border-collapse: collapse;
}
table, th, td {
border: 1px solid black;
}
th, td {
padding: 15px;
text-align: left;
}
tr:nth-child(even) {
background-color: #f2f2f2;
}
</style>
</head>
<body>
<table>
<tr>
<th>Column1</th>
<th>Column2</th>
</tr>
"""
for index, row in data.iterrows():
html_content += f"""
<tr>
<td>{html.escape(str(row['Column1']))}</td>
<td>{html.escape(str(row['Column2']))}</td>
</tr>
"""
html_content += """
</table>
</body>
</html>
"""
# 写入文件
with open('output.html', 'w') as file:
file.write(html_content)
定期执行任务
schedule.every().day.at("10:00").do(job)
while True:
schedule.run_pending()
time.sleep(1)
七、错误处理和日志记录
在自动化过程中,错误处理和日志记录是保障脚本稳定运行的重要措施。可以使用Python的logging
库记录日志,并在脚本中添加错误处理机制。
import logging
配置日志记录
logging.basicConfig(filename='script.log', level=logging.INFO)
def job():
try:
# 读取数据
data = pd.read_csv('data.csv')
# 生成HTML内容
html_content = """
<!DOCTYPE html>
<html>
<head>
<title>Data</title>
<style>
table {
width: 100%;
border-collapse: collapse;
}
table, th, td {
border: 1px solid black;
}
th, td {
padding: 15px;
text-align: left;
}
tr:nth-child(even) {
background-color: #f2f2f2;
}
</style>
</head>
<body>
<table>
<tr>
<th>Column1</th>
<th>Column2</th>
</tr>
"""
for index, row in data.iterrows():
html_content += f"""
<tr>
<td>{html.escape(str(row['Column1']))}</td>
<td>{html.escape(str(row['Column2']))}</td>
</tr>
"""
html_content += """
</table>
</body>
</html>
"""
# 写入文件
with open('output.html', 'w') as file:
file.write(html_content)
logging.info('Job completed successfully')
except Exception as e:
logging.error(f'Error occurred: {e}')
定期执行任务
schedule.every().day.at("10:00").do(job)
while True:
schedule.run_pending()
time.sleep(1)
八、总结
使用Python写入HTML文件数据库涉及多个步骤,从读取数据、生成HTML内容、写入文件,到自动化和扩展,每一步都需要仔细规划和实施。通过本文的详细介绍和代码示例,希望能够帮助读者理解并掌握这一过程,为实际应用提供有价值的参考。
相关问答FAQs:
如何使用Python将数据写入HTML文件?
使用Python写入HTML文件通常涉及文件操作和字符串格式化。可以使用Python内置的open()
函数以写入模式打开一个HTML文件,并使用write()
方法将内容写入文件。可以通过字符串拼接或使用模板引擎(如Jinja2)来生成HTML代码,从而实现动态内容的插入。
可以用哪些库来简化HTML文件的生成过程?
有多个库可以帮助简化HTML文件的生成,例如BeautifulSoup
和Flask
。BeautifulSoup
可以方便地创建和解析HTML文档,而Flask
是一个轻量级的Web框架,支持动态生成HTML页面。使用这些库,能够更轻松地处理HTML结构和数据插入。
如何将Python中的数据存储到HTML文件中?
将Python中的数据存储到HTML文件中,通常需要将数据转换为HTML格式。可以使用Python的列表和字典结构,将数据格式化为HTML表格或列表,然后将生成的HTML字符串写入文件。确保正确使用HTML标签,以便在浏览器中正确显示数据。
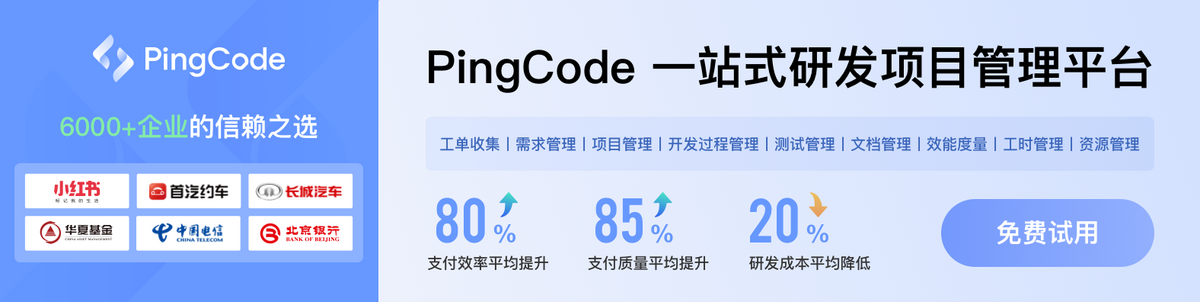