要将两个数据库文件(db文件)合并,您需要使用一个数据库管理系统(DBMS)或编程语言,如Python。 使用Python进行数据库文件合并 是一个常见的任务,可以通过以下几步实现:连接两个数据库、读取数据、合并数据、写入新数据库。下面将详细讲解如何通过Python实现这一操作。
一、连接两个数据库
首先,您需要连接到两个数据库文件。Python提供了多种数据库连接库,最常见的是SQLite库。SQLite是一个轻量级的数据库,它的数据库是存储在一个文件中的,因此非常适合用来处理db文件。
import sqlite3
连接第一个数据库
conn1 = sqlite3.connect('database1.db')
cursor1 = conn1.cursor()
连接第二个数据库
conn2 = sqlite3.connect('database2.db')
cursor2 = conn2.cursor()
这段代码中,sqlite3.connect()
函数用于建立到数据库文件的连接,cursor()
方法则用于创建一个游标对象,用于执行SQL语句。
二、读取数据
读取数据是合并数据库文件的关键步骤。首先,我们需要获取两个数据库中的表结构,然后读取数据。
# 获取第一个数据库中的所有表
cursor1.execute("SELECT name FROM sqlite_master WHERE type='table';")
tables1 = cursor1.fetchall()
获取第二个数据库中的所有表
cursor2.execute("SELECT name FROM sqlite_master WHERE type='table';")
tables2 = cursor2.fetchall()
这段代码中,sqlite_master
是一个内置表,存储了数据库中的所有表的信息。通过查询sqlite_master
表,可以获取数据库中的所有表名。
三、合并数据
合并数据时,需要确保两个数据库的表结构一致,然后将数据插入到新的数据库中。
# 创建一个新的数据库文件
conn_merged = sqlite3.connect('merged_database.db')
cursor_merged = conn_merged.cursor()
遍历第一个数据库中的所有表,创建表结构并插入数据
for table in tables1:
table_name = table[0]
# 获取表结构
cursor1.execute(f"PRAGMA table_info({table_name});")
columns = cursor1.fetchall()
# 创建表
column_definitions = ", ".join([f"{col[1]} {col[2]}" for col in columns])
cursor_merged.execute(f"CREATE TABLE {table_name} ({column_definitions});")
# 插入数据
cursor1.execute(f"SELECT * FROM {table_name};")
rows = cursor1.fetchall()
for row in rows:
placeholders = ", ".join(["?" for _ in row])
cursor_merged.execute(f"INSERT INTO {table_name} VALUES ({placeholders});", row)
遍历第二个数据库中的所有表,插入数据
for table in tables2:
table_name = table[0]
# 检查表是否已存在
cursor_merged.execute(f"SELECT count(name) FROM sqlite_master WHERE type='table' AND name='{table_name}';")
if cursor_merged.fetchone()[0] == 0:
# 获取表结构
cursor2.execute(f"PRAGMA table_info({table_name});")
columns = cursor2.fetchall()
# 创建表
column_definitions = ", ".join([f"{col[1]} {col[2]}" for col in columns])
cursor_merged.execute(f"CREATE TABLE {table_name} ({column_definitions});")
# 插入数据
cursor2.execute(f"SELECT * FROM {table_name};")
rows = cursor2.fetchall()
for row in rows:
placeholders = ", ".join(["?" for _ in row])
cursor_merged.execute(f"INSERT INTO {table_name} VALUES ({placeholders});", row)
在这段代码中,首先遍历第一个数据库中的所有表,获取表结构并在新的数据库中创建表,然后插入数据。接着遍历第二个数据库中的所有表,检查表是否已经存在,如果不存在则创建表,然后插入数据。
四、写入新数据库
最后一步是将合并后的数据写入新的数据库文件。
# 提交更改并关闭连接
conn_merged.commit()
conn1.close()
conn2.close()
conn_merged.close()
这段代码中,commit()
方法用于提交更改,close()
方法用于关闭数据库连接。
总结
通过以上步骤,您可以使用Python将两个数据库文件合并。连接两个数据库、读取数据、合并数据、写入新数据库 是合并数据库文件的关键步骤。在实际应用中,可能还需要处理更多的细节问题,例如处理冲突、优化性能等。以下是完整的代码示例,供您参考:
import sqlite3
连接第一个数据库
conn1 = sqlite3.connect('database1.db')
cursor1 = conn1.cursor()
连接第二个数据库
conn2 = sqlite3.connect('database2.db')
cursor2 = conn2.cursor()
创建一个新的数据库文件
conn_merged = sqlite3.connect('merged_database.db')
cursor_merged = conn_merged.cursor()
获取第一个数据库中的所有表
cursor1.execute("SELECT name FROM sqlite_master WHERE type='table';")
tables1 = cursor1.fetchall()
获取第二个数据库中的所有表
cursor2.execute("SELECT name FROM sqlite_master WHERE type='table';")
tables2 = cursor2.fetchall()
遍历第一个数据库中的所有表,创建表结构并插入数据
for table in tables1:
table_name = table[0]
# 获取表结构
cursor1.execute(f"PRAGMA table_info({table_name});")
columns = cursor1.fetchall()
# 创建表
column_definitions = ", ".join([f"{col[1]} {col[2]}" for col in columns])
cursor_merged.execute(f"CREATE TABLE {table_name} ({column_definitions});")
# 插入数据
cursor1.execute(f"SELECT * FROM {table_name};")
rows = cursor1.fetchall()
for row in rows:
placeholders = ", ".join(["?" for _ in row])
cursor_merged.execute(f"INSERT INTO {table_name} VALUES ({placeholders});", row)
遍历第二个数据库中的所有表,插入数据
for table in tables2:
table_name = table[0]
# 检查表是否已存在
cursor_merged.execute(f"SELECT count(name) FROM sqlite_master WHERE type='table' AND name='{table_name}';")
if cursor_merged.fetchone()[0] == 0:
# 获取表结构
cursor2.execute(f"PRAGMA table_info({table_name});")
columns = cursor2.fetchall()
# 创建表
column_definitions = ", ".join([f"{col[1]} {col[2]}" for col in columns])
cursor_merged.execute(f"CREATE TABLE {table_name} ({column_definitions});")
# 插入数据
cursor2.execute(f"SELECT * FROM {table_name};")
rows = cursor2.fetchall()
for row in rows:
placeholders = ", ".join(["?" for _ in row])
cursor_merged.execute(f"INSERT INTO {table_name} VALUES ({placeholders});", row)
提交更改并关闭连接
conn_merged.commit()
conn1.close()
conn2.close()
conn_merged.close()
相关问答FAQs:
如何在Python中有效地合并两个数据库文件?
合并两个数据库文件通常涉及到读取每个文件的内容并将其写入一个新的数据库。可以使用sqlite3
模块,对于SQLite数据库非常有效。首先,您需要连接到两个数据库,读取表中的数据,然后将其插入到一个目标数据库中。确保在合并时处理数据冲突和重复项。
合并数据库文件时需要注意哪些数据完整性问题?
在合并数据库时,数据完整性至关重要。您需要检查两个数据库中的主键和外键约束,确保合并后的数据不会导致重复或丢失信息。使用事务来确保数据一致性,可以在合并过程中进行回滚以防止错误。
是否有推荐的库或工具来简化Python中的数据库合并过程?
在Python中,除了使用sqlite3
模块,还可以考虑使用pandas
库,它提供了高效的数据处理功能。通过读取数据库表到DataFrame,您可以轻松地合并和清理数据,最后再将合并后的DataFrame写入新的数据库文件。其他工具如SQLAlchemy也可以帮助简化操作,特别是对于复杂的数据库结构。
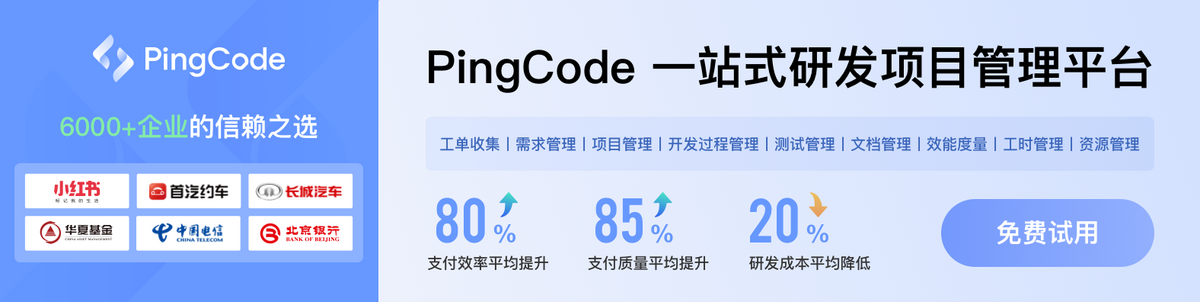