Python如何比较三个数的大小?
Python提供了多种方法来比较三个数的大小,包括使用简单的if-else语句、max()和min()函数、sorted()函数等方法。最常见的方法是使用if-else语句,因为它提供了最直观的控制流。以下是详细描述:
使用if-else语句可以清晰地比较三个数,找到最大值和最小值。例如,假设有三个数a、b和c,我们可以通过一系列的条件判断来确定它们的大小关系。具体实现如下:
a = 5
b = 10
c = 7
if a > b and a > c:
print(f"{a} is the largest")
elif b > a and b > c:
print(f"{b} is the largest")
else:
print(f"{c} is the largest")
if a < b and a < c:
print(f"{a} is the smallest")
elif b < a and b < c:
print(f"{b} is the smallest")
else:
print(f"{c} is the smallest")
一、使用if-else语句比较三个数
使用if-else语句是比较三个数最常见的方法。它可以清晰地描述不同的条件,便于理解和维护。以下是详细的步骤和示例:
1. 基本比较方法
首先,我们需要初始化三个数,然后使用if-else语句进行比较:
a = 10
b = 15
c = 20
if a > b and a > c:
largest = a
elif b > a and b > c:
largest = b
else:
largest = c
if a < b and a < c:
smallest = a
elif b < a and b < c:
smallest = b
else:
smallest = c
print(f"The largest number is {largest}")
print(f"The smallest number is {smallest}")
2. 处理特殊情况
在实际使用中,我们还需要处理一些特殊情况,例如当两个或三个数相等时:
a = 10
b = 10
c = 5
if a == b == c:
print("All three numbers are equal")
elif a == b or a == c or b == c:
if a == b and a > c:
print(f"The largest number is {a} (a and b are equal)")
elif a == c and a > b:
print(f"The largest number is {a} (a and c are equal)")
elif b == c and b > a:
print(f"The largest number is {b} (b and c are equal)")
else:
if a > b and a > c:
print(f"The largest number is {a}")
elif b > a and b > c:
print(f"The largest number is {b}")
else:
print(f"The largest number is {c}")
二、使用max()和min()函数
Python内置的max()和min()函数可以直接用于比较三个数,找到最大值和最小值。这种方法简洁明了,适合快速实现需求。
1. 使用max()函数
a = 10
b = 15
c = 20
largest = max(a, b, c)
print(f"The largest number is {largest}")
2. 使用min()函数
a = 10
b = 15
c = 20
smallest = min(a, b, c)
print(f"The smallest number is {smallest}")
3. 综合使用max()和min()函数
我们可以同时使用max()和min()函数来找到最大值和最小值:
a = 10
b = 15
c = 20
largest = max(a, b, c)
smallest = min(a, b, c)
print(f"The largest number is {largest}")
print(f"The smallest number is {smallest}")
三、使用sorted()函数
Python的sorted()函数可以将一个可迭代对象排序,然后我们可以直接获取排序后的第一个和最后一个元素,分别表示最小值和最大值。
1. 使用sorted()函数
a = 10
b = 15
c = 20
sorted_numbers = sorted([a, b, c])
smallest = sorted_numbers[0]
largest = sorted_numbers[-1]
print(f"The largest number is {largest}")
print(f"The smallest number is {smallest}")
2. 处理相等情况
在实际使用中,我们还需要处理数值相等的情况:
a = 10
b = 10
c = 5
sorted_numbers = sorted([a, b, c])
smallest = sorted_numbers[0]
largest = sorted_numbers[-1]
if smallest == largest:
print("All three numbers are equal")
else:
print(f"The largest number is {largest}")
print(f"The smallest number is {smallest}")
四、使用自定义函数
我们可以定义一个函数来比较三个数,这样可以提高代码的复用性和可维护性。
1. 定义比较函数
def find_largest_and_smallest(a, b, c):
largest = max(a, b, c)
smallest = min(a, b, c)
return largest, smallest
a = 10
b = 15
c = 20
largest, smallest = find_largest_and_smallest(a, b, c)
print(f"The largest number is {largest}")
print(f"The smallest number is {smallest}")
2. 处理相等情况
在函数中处理相等情况:
def find_largest_and_smallest(a, b, c):
if a == b == c:
return "All three numbers are equal"
largest = max(a, b, c)
smallest = min(a, b, c)
return largest, smallest
a = 10
b = 10
c = 5
result = find_largest_and_smallest(a, b, c)
if isinstance(result, str):
print(result)
else:
largest, smallest = result
print(f"The largest number is {largest}")
print(f"The smallest number is {smallest}")
五、使用自定义类
我们还可以定义一个类来封装比较三个数的逻辑,这样可以提高代码的组织性和可扩展性。
1. 定义比较类
class NumberComparator:
def __init__(self, a, b, c):
self.a = a
self.b = b
self.c = c
def find_largest(self):
return max(self.a, self.b, self.c)
def find_smallest(self):
return min(self.a, self.b, self.c)
def compare(self):
if self.a == self.b == self.c:
return "All three numbers are equal"
largest = self.find_largest()
smallest = self.find_smallest()
return largest, smallest
comparator = NumberComparator(10, 15, 20)
result = comparator.compare()
if isinstance(result, str):
print(result)
else:
largest, smallest = result
print(f"The largest number is {largest}")
print(f"The smallest number is {smallest}")
六、使用lambda函数和列表解析
Python的lambda函数和列表解析提供了一种更为简洁的方法来比较三个数。
1. 使用lambda函数
a = 10
b = 15
c = 20
largest = (lambda x, y, z: max(x, y, z))(a, b, c)
smallest = (lambda x, y, z: min(x, y, z))(a, b, c)
print(f"The largest number is {largest}")
print(f"The smallest number is {smallest}")
2. 使用列表解析
a = 10
b = 15
c = 20
numbers = [a, b, c]
largest = max(numbers)
smallest = min(numbers)
print(f"The largest number is {largest}")
print(f"The smallest number is {smallest}")
七、总结
在Python中,比较三个数的大小有多种方法可供选择,包括if-else语句、max()和min()函数、sorted()函数、自定义函数、自定义类、lambda函数和列表解析。每种方法都有其优缺点,适用于不同的场景。通过掌握这些方法,我们可以更加灵活地处理数字比较问题,从而编写出更加高效、可维护的代码。
相关问答FAQs:
如何使用Python比较三个数的大小?
在Python中,可以使用内置的比较运算符来轻松比较三个数的大小。首先,您可以使用if
语句来判断哪个数最大。以下是一个示例代码:
a = 5
b = 10
c = 3
if a >= b and a >= c:
print(f"{a} 是最大的数")
elif b >= a and b >= c:
print(f"{b} 是最大的数")
else:
print(f"{c} 是最大的数")
通过这种方式,您可以比较任意三个数并找出最大值。
Python中可以使用哪些内置函数来比较数值?
Python提供了一些内置函数来帮助您比较数值,例如max()
函数。使用max()
函数可以简化比较过程。示例代码如下:
a = 5
b = 10
c = 3
最大值 = max(a, b, c)
print(f"最大值是 {最大值}")
这个方法不仅简洁,而且易于理解,适合初学者和有经验的开发者使用。
如何处理用户输入以比较三个数的大小?
在实际应用中,您可能需要从用户那里获取输入。使用input()
函数可以轻松实现这一点。以下是一个示例,展示如何获取用户输入并比较三个数的大小:
a = float(input("请输入第一个数: "))
b = float(input("请输入第二个数: "))
c = float(input("请输入第三个数: "))
最大值 = max(a, b, c)
print(f"最大值是 {最大值}")
这种方法使得程序更加互动,用户可以根据自己的需求输入不同的数值进行比较。
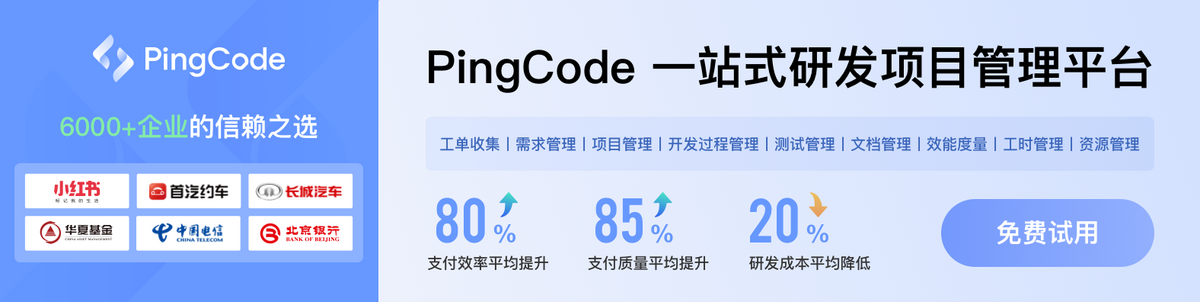