使用Python绘制带有阴影的四个矩形
在Python中绘制带有阴影的矩形,可以利用诸如Matplotlib、Pillow等库。使用Matplotlib的patches模块、利用阴影参数、使用Pillow的ImageDraw模块,这三种方法都可以实现矩形阴影效果。接下来,我们将详细介绍如何使用这些方法来绘制带有阴影的矩形。
一、使用Matplotlib的patches模块
Matplotlib是Python中广泛使用的绘图库,可以轻松绘制各种图形。通过patches
模块中的Rectangle
类可以创建矩形,再利用阴影参数实现阴影效果。
1. 安装和导入必要的库
import matplotlib.pyplot as plt
import matplotlib.patches as patches
import matplotlib.colors as mcolors
2. 创建绘图对象和添加矩形
fig, ax = plt.subplots()
定义矩形参数
rect_params = [
(0.1, 0.1, 0.2, 0.3),
(0.4, 0.1, 0.2, 0.3),
(0.1, 0.5, 0.2, 0.3),
(0.4, 0.5, 0.2, 0.3)
]
阴影偏移量
shadow_offset = 0.02
添加矩形和阴影
for (x, y, width, height) in rect_params:
shadow = patches.Rectangle(
(x + shadow_offset, y - shadow_offset), width, height,
linewidth=1, edgecolor='gray', facecolor='gray', alpha=0.5)
rect = patches.Rectangle(
(x, y), width, height,
linewidth=1, edgecolor='black', facecolor='blue')
ax.add_patch(shadow)
ax.add_patch(rect)
设置显示范围
ax.set_xlim(0, 1)
ax.set_ylim(0, 1)
ax.set_aspect('equal')
plt.show()
在上述代码中,shadow_offset
参数用于控制阴影的偏移量,使阴影效果更为明显和自然。通过调整alpha
参数可以控制阴影的透明度。
二、利用阴影参数
Matplotlib中的FancyBboxPatch
类提供了更加灵活的矩形绘制方法,可以使用boxstyle
参数来控制矩形的边框样式,并通过shadow
参数直接添加阴影。
1. 使用FancyBboxPatch绘制矩形
from matplotlib.patches import FancyBboxPatch
fig, ax = plt.subplots()
定义FancyBboxPatch参数
box_params = [
(0.1, 0.1, 0.2, 0.3),
(0.4, 0.1, 0.2, 0.3),
(0.1, 0.5, 0.2, 0.3),
(0.4, 0.5, 0.2, 0.3)
]
添加FancyBboxPatch矩形
for (x, y, width, height) in box_params:
fancy_box = FancyBboxPatch(
(x, y), width, height,
boxstyle="round,pad=0.1", edgecolor='black', facecolor='blue',
shadow=True)
ax.add_patch(fancy_box)
ax.set_xlim(0, 1)
ax.set_ylim(0, 1)
ax.set_aspect('equal')
plt.show()
FancyBboxPatch
类中的shadow
参数可以直接控制矩形的阴影效果,boxstyle
参数用于控制矩形的边框样式。
三、使用Pillow的ImageDraw模块
Pillow是一个强大的图像处理库,可以灵活地处理图像和绘制图形。通过ImageDraw
模块,可以在图像上绘制矩形并添加阴影效果。
1. 安装和导入Pillow库
from PIL import Image, ImageDraw
2. 创建图像和绘制矩形
# 创建空白图像
width, height = 400, 400
image = Image.new('RGB', (width, height), 'white')
draw = ImageDraw.Draw(image)
定义矩形参数
rect_params = [
(50, 50, 150, 200),
(200, 50, 300, 200),
(50, 250, 150, 400),
(200, 250, 300, 400)
]
阴影偏移量
shadow_offset = 10
绘制矩形和阴影
for (x1, y1, x2, y2) in rect_params:
draw.rectangle((x1 + shadow_offset, y1 + shadow_offset, x2 + shadow_offset, y2 + shadow_offset), fill='gray')
draw.rectangle((x1, y1, x2, y2), outline='black', fill='blue')
显示图像
image.show()
在上述代码中,通过ImageDraw
模块的rectangle
方法可以绘制矩形,先绘制阴影矩形,再绘制实际矩形以实现阴影效果。
结论
通过上述方法,可以在Python中使用不同的库和模块绘制带有阴影效果的矩形。Matplotlib的patches模块、利用阴影参数、Pillow的ImageDraw模块,这些方法都具有各自的优势和应用场景,可以根据具体需求选择合适的方法来实现图形绘制和阴影效果。
希望本文提供的详细步骤和代码示例能够帮助你在Python中轻松绘制带有阴影的矩形,并进一步应用到其他图形绘制场景中。
相关问答FAQs:
如何在Python中给矩形添加阴影效果?
在Python中,可以使用Matplotlib库绘制矩形并添加阴影效果。可以通过调整矩形的透明度和颜色来实现阴影效果,或者使用PathPatch
类为矩形添加阴影。具体步骤包括设置颜色、透明度,以及利用偏移量来模拟阴影位置。
使用哪个库最适合绘制带阴影的矩形?
Matplotlib是一个非常流行且功能强大的库,适合用于绘制各种图形,包括带阴影的矩形。除了Matplotlib,Pygame和Tkinter等库也可以实现类似效果,但Matplotlib在数据可视化方面更为出色。
如何控制矩形的阴影深度和方向?
可以通过调整阴影的颜色和透明度来控制阴影的深度,同时通过设置阴影的偏移量来改变阴影的方向。例如,增加偏移量可以让阴影看起来更远,降低透明度则可以使阴影显得更淡。通过对这些参数的调整,可以实现不同风格的阴影效果。
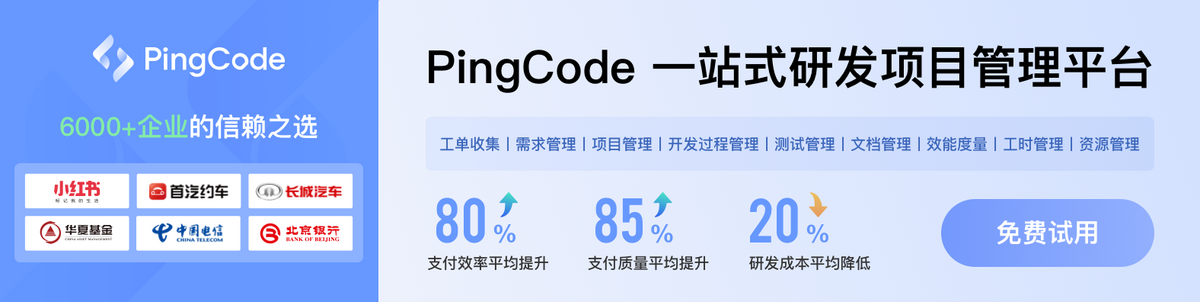