Python 去除数字前后的中括号的方法有很多种,包括使用正则表达式、字符串替换等。其中,正则表达式 是处理这种任务的最佳工具,因为它能够高效且灵活地匹配和替换字符串中的特定模式。下面是一个详细的解释,展示如何通过正则表达式去除数字前后的中括号。
一、正则表达式的使用
正则表达式(Regular Expression)是一种用来匹配文本模式的强大工具。Python 提供了 re
模块来处理正则表达式。
1. 安装和导入正则表达式模块
Python 标准库中已经包含了 re
模块,因此不需要额外安装。只需在代码开头导入即可:
import re
2. 正则表达式匹配模式
为了去除数字前后的中括号,可以使用以下正则表达式模式:
\[
: 匹配左中括号[
\]
: 匹配右中括号]
\d+
: 匹配一个或多个数字
综合起来,整个正则表达式模式为 \[\d+\]
,这将匹配包含在中括号内的数字。
3. 使用 re.sub
方法替换
re.sub
是用于替换字符串中符合正则表达式模式的部分。以下是示例代码:
import re
def remove_brackets(input_string):
# 使用正则表达式去除数字前后的中括号
result = re.sub(r'\[\d+\]', lambda x: x.group(0)[1:-1], input_string)
return result
示例字符串
example_string = "This is a test string with numbers [123] and more text [456]."
调用函数
cleaned_string = remove_brackets(example_string)
print(cleaned_string)
在这个函数中,re.sub
方法使用了一个 lambda 函数来处理匹配的部分,通过 x.group(0)[1:-1]
去除中括号。
二、字符串替换方法
除了正则表达式,Python 的字符串方法也可以用来处理这种任务。
1. 使用 str.replace
方法
虽然 str.replace
方法比较简单,但需要多次调用来处理多种情况:
def remove_brackets(input_string):
# 使用字符串替换方法
result = input_string.replace('[', '').replace(']', '')
return result
示例字符串
example_string = "This is a test string with numbers [123] and more text [456]."
调用函数
cleaned_string = remove_brackets(example_string)
print(cleaned_string)
这种方法虽然简单,但不如正则表达式灵活。
2. 使用 str.translate
方法
str.translate
方法结合 str.maketrans
可以高效地替换多个字符:
def remove_brackets(input_string):
# 使用字符串翻译方法
translation_table = str.maketrans('', '', '[]')
result = input_string.translate(translation_table)
return result
示例字符串
example_string = "This is a test string with numbers [123] and more text [456]."
调用函数
cleaned_string = remove_brackets(example_string)
print(cleaned_string)
这种方法相比 str.replace
更加简洁高效。
三、示例和应用场景
1. 日志处理
在日志处理中,通常需要清理包含时间戳或标识符的中括号:
log_entry = "2023-01-01 12:00:00 [INFO] [123] User logged in."
cleaned_log_entry = remove_brackets(log_entry)
print(cleaned_log_entry)
2. 数据清洗
在数据处理和清洗过程中,可能需要清理数据字段中的中括号:
data = ["[123] John", "[456] Jane", "[789] Doe"]
cleaned_data = [remove_brackets(item) for item in data]
print(cleaned_data)
四、总结
去除数字前后的中括号在Python中可以通过正则表达式、字符串替换、字符串翻译等多种方法实现。正则表达式提供了最灵活和强大的解决方案,而字符串替换和翻译方法则提供了简洁高效的替代方案。根据具体需求选择合适的方法,可以高效完成数据清洗和文本处理任务。
相关问答FAQs:
如何在Python中处理字符串,去除数字前后的中括号?
在Python中,可以使用字符串的strip()
方法来去除字符串前后的中括号。只需调用strip()
并传入要去除的字符,如下示例所示:
string_with_brackets = "[12345]"
cleaned_string = string_with_brackets.strip("[]")
print(cleaned_string) # 输出:12345
是否可以使用正则表达式来去除字符串中的中括号?
确实可以使用正则表达式来处理更复杂的情况。例如,使用re
模块的sub()
函数可以有效去除字符串中所有的中括号:
import re
string_with_brackets = "[12345]"
cleaned_string = re.sub(r'[\[\]]', '', string_with_brackets)
print(cleaned_string) # 输出:12345
这种方法非常适合处理包含多个中括号的字符串。
如何处理列表中的多个字符串,去除每个字符串的中括号?
如果你有一个包含多个带中括号的字符串的列表,可以使用列表推导式结合strip()
或正则表达式来处理。示例代码如下:
strings_with_brackets = ["[123]", "[456]", "[789]"]
cleaned_strings = [s.strip("[]") for s in strings_with_brackets]
print(cleaned_strings) # 输出:['123', '456', '789']
这种方式使得处理多个字符串变得简单高效。
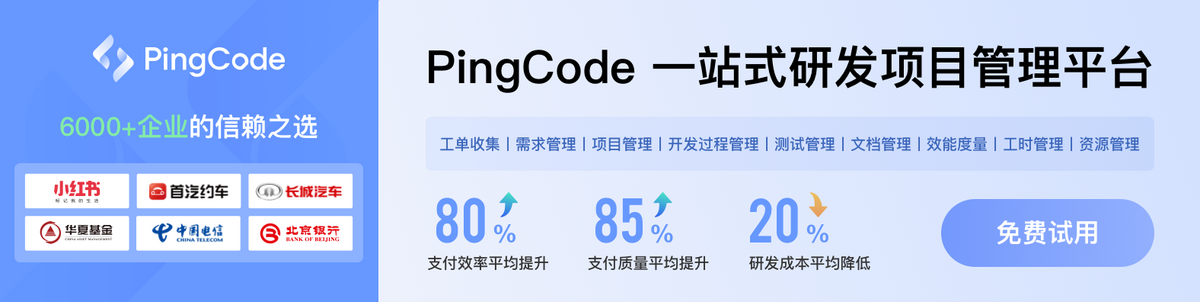