如何用Python做一个杂货店
在利用Python开发一个杂货店系统时,关键在于库存管理、销售系统、用户界面设计、数据存储。这些功能的实现可以使杂货店高效运营。本文将深入探讨如何用Python实现这些功能,帮助你创建一个功能完善的杂货店系统。
一、库存管理
库存管理是杂货店运营的核心,它直接影响到商品的存货状况和销售情况。通过有效的库存管理,可以确保商品的充足供应,避免缺货和积压。
1、库存数据的存储
为了实现库存管理,首先需要一个地方来存储库存数据。常见的选择有数据库(如SQLite、MySQL)或者简单的文件存储(如CSV、JSON文件)。数据库通常更适合大型数据存储和复杂查询。
使用SQLite数据库
SQLite是一个轻量级的嵌入式数据库,适用于中小型应用。我们可以通过Python的sqlite3模块来操作SQLite数据库。
import sqlite3
连接到数据库(如果数据库不存在,则会自动创建)
conn = sqlite3.connect('grocery_store.db')
cursor = conn.cursor()
创建库存表
cursor.execute('''
CREATE TABLE IF NOT EXISTS inventory (
id INTEGER PRIMARY KEY,
product_name TEXT NOT NULL,
quantity INTEGER NOT NULL,
price REAL NOT NULL
)
''')
conn.commit()
conn.close()
2、添加和更新库存
为了管理库存,我们需要能够添加新商品和更新现有商品的库存信息。
def add_product(product_name, quantity, price):
conn = sqlite3.connect('grocery_store.db')
cursor = conn.cursor()
cursor.execute('''
INSERT INTO inventory (product_name, quantity, price)
VALUES (?, ?, ?)
''', (product_name, quantity, price))
conn.commit()
conn.close()
def update_product(product_id, quantity):
conn = sqlite3.connect('grocery_store.db')
cursor = conn.cursor()
cursor.execute('''
UPDATE inventory
SET quantity = ?
WHERE id = ?
''', (quantity, product_id))
conn.commit()
conn.close()
3、查询库存
为了确保库存信息的准确性,我们需要定期查询库存信息,并根据需要进行调整。
def get_inventory():
conn = sqlite3.connect('grocery_store.db')
cursor = conn.cursor()
cursor.execute('SELECT * FROM inventory')
rows = cursor.fetchall()
conn.close()
return rows
示例:打印库存信息
inventory = get_inventory()
for item in inventory:
print(item)
二、销售系统
销售系统是杂货店运营的核心环节,它直接关系到商品的销售和顾客的购买体验。
1、创建销售表
我们需要一个表来存储销售记录,以便跟踪销售情况和库存变化。
conn = sqlite3.connect('grocery_store.db')
cursor = conn.cursor()
创建销售表
cursor.execute('''
CREATE TABLE IF NOT EXISTS sales (
id INTEGER PRIMARY KEY,
product_id INTEGER NOT NULL,
quantity INTEGER NOT NULL,
total_price REAL NOT NULL,
sale_date TEXT NOT NULL,
FOREIGN KEY (product_id) REFERENCES inventory (id)
)
''')
conn.commit()
conn.close()
2、记录销售
每当有商品售出时,我们需要记录销售信息,并相应地更新库存。
import datetime
def record_sale(product_id, quantity):
conn = sqlite3.connect('grocery_store.db')
cursor = conn.cursor()
cursor.execute('SELECT price FROM inventory WHERE id = ?', (product_id,))
price = cursor.fetchone()[0]
total_price = price * quantity
sale_date = datetime.datetime.now().strftime('%Y-%m-%d %H:%M:%S')
cursor.execute('''
INSERT INTO sales (product_id, quantity, total_price, sale_date)
VALUES (?, ?, ?, ?)
''', (product_id, quantity, total_price, sale_date))
cursor.execute('''
UPDATE inventory
SET quantity = quantity - ?
WHERE id = ?
''', (quantity, product_id))
conn.commit()
conn.close()
3、查询销售记录
为了分析销售情况,我们需要能够查询销售记录。
def get_sales():
conn = sqlite3.connect('grocery_store.db')
cursor = conn.cursor()
cursor.execute('SELECT * FROM sales')
rows = cursor.fetchall()
conn.close()
return rows
示例:打印销售记录
sales = get_sales()
for sale in sales:
print(sale)
三、用户界面设计
用户界面设计是提高用户体验的关键,一个好的界面可以使用户更容易操作系统。
1、命令行界面
对于简单的杂货店系统,命令行界面是一个不错的选择。我们可以使用Python的input()函数来接收用户输入,并通过一系列菜单选项引导用户完成操作。
def main():
while True:
print('''
1. 添加商品
2. 更新库存
3. 查看库存
4. 记录销售
5. 查看销售记录
6. 退出
''')
choice = input('请选择操作: ')
if choice == '1':
product_name = input('商品名称: ')
quantity = int(input('数量: '))
price = float(input('价格: '))
add_product(product_name, quantity, price)
elif choice == '2':
product_id = int(input('商品ID: '))
quantity = int(input('新数量: '))
update_product(product_id, quantity)
elif choice == '3':
inventory = get_inventory()
for item in inventory:
print(item)
elif choice == '4':
product_id = int(input('商品ID: '))
quantity = int(input('数量: '))
record_sale(product_id, quantity)
elif choice == '5':
sales = get_sales()
for sale in sales:
print(sale)
elif choice == '6':
break
else:
print('无效的选择,请重新输入')
if __name__ == '__main__':
main()
2、图形用户界面
对于更复杂的系统,可以使用图形用户界面(GUI)。Python的Tkinter库提供了构建GUI应用的基本工具。
import tkinter as tk
from tkinter import messagebox
def add_product_gui():
# 创建子窗口
add_window = tk.Toplevel()
add_window.title('添加商品')
tk.Label(add_window, text='商品名称').grid(row=0, column=0)
tk.Label(add_window, text='数量').grid(row=1, column=0)
tk.Label(add_window, text='价格').grid(row=2, column=0)
product_name_entry = tk.Entry(add_window)
quantity_entry = tk.Entry(add_window)
price_entry = tk.Entry(add_window)
product_name_entry.grid(row=0, column=1)
quantity_entry.grid(row=1, column=1)
price_entry.grid(row=2, column=1)
def add_product_action():
product_name = product_name_entry.get()
quantity = int(quantity_entry.get())
price = float(price_entry.get())
add_product(product_name, quantity, price)
messagebox.showinfo('成功', '商品添加成功')
add_window.destroy()
tk.Button(add_window, text='添加', command=add_product_action).grid(row=3, column=0, columnspan=2)
def main_gui():
root = tk.Tk()
root.title('杂货店系统')
tk.Button(root, text='添加商品', command=add_product_gui).pack()
tk.Button(root, text='退出', command=root.quit).pack()
root.mainloop()
if __name__ == '__main__':
main_gui()
四、数据存储
数据存储是系统运行的基础,选择合适的数据存储方式可以提高系统的稳定性和效率。
1、数据库
如前文所述,SQLite是一个轻量级的数据库,适用于中小型应用。对于更大型的应用,可以选择MySQL、PostgreSQL等。
2、文件存储
对于数据量较小的应用,可以选择使用文件存储,如CSV、JSON文件。文件存储方式简单易用,但不适合处理复杂查询。
使用JSON文件存储
import json
def save_inventory_to_file(file_path='inventory.json'):
inventory = get_inventory()
with open(file_path, 'w') as file:
json.dump(inventory, file)
def load_inventory_from_file(file_path='inventory.json'):
with open(file_path, 'r') as file:
inventory = json.load(file)
return inventory
总结
通过本文的详细介绍,我们可以了解到如何利用Python开发一个功能完善的杂货店系统,涵盖了库存管理、销售系统、用户界面设计、数据存储等方面。希望这篇文章能够帮助你在实际开发中快速上手,并创建出一个高效、实用的杂货店系统。
相关问答FAQs:
如何用Python创建一个简单的杂货店管理系统?
可以使用Python中的各种库来创建一个杂货店管理系统。推荐使用Flask或Django框架来构建网页应用,使用SQLite或PostgreSQL作为数据库。你可以创建商品管理、库存跟踪和销售记录等功能模块,从而实现一个完整的杂货店系统。
在Python中如何处理杂货店的库存管理?
库存管理是杂货店运营的核心部分。可以通过建立一个数据库来存储商品信息,包括名称、数量和价格等。在Python中,可以使用SQLAlchemy库来与数据库交互,实现库存的增减、查询和警报功能,以便及时补货。
如何使用Python生成销售报表?
生成销售报表可以帮助你分析杂货店的业绩。你可以使用Pandas库来处理销售数据,创建数据框并进行数据分析。通过绘制图表(例如使用Matplotlib或Seaborn库),可以直观地展示销售趋势和热门商品,帮助做出更好的经营决策。
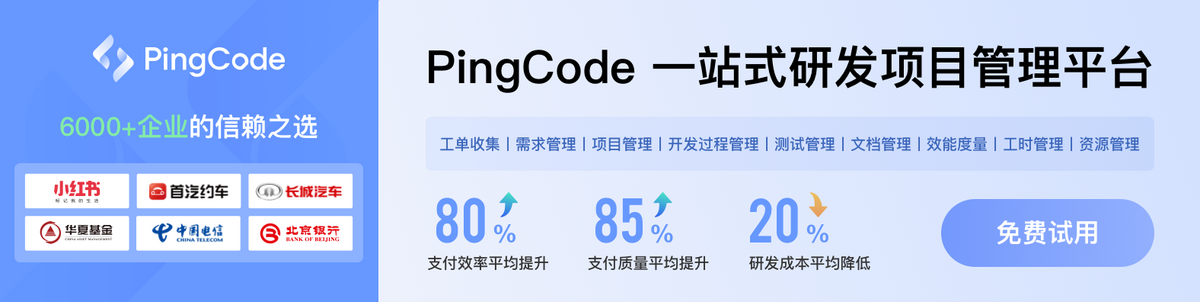