在Python中,可以使用内置的 isinstance()
函数来判断一个变量是列表还是字符串。 具体来说,isinstance()
函数可以接受两个参数,第一个是变量,第二个是类型名。通过调用 isinstance(variable, list)
和 isinstance(variable, str)
,可以分别判断变量是否是列表或字符串。如果一个变量是列表,则 isinstance(variable, list)
会返回 True
,否则返回 False
;类似地,对于字符串,isinstance(variable, str)
可以做出相同的判断。
详细描述:
使用 isinstance()
函数不仅可以检查变量的类型,还能处理继承关系。例如,假如一个类继承了 list
或 str
,isinstance()
依旧能够正确识别它的类型。这种灵活性使得 isinstance()
在类型检查中非常实用。此外,isinstance()
函数的使用非常简单、直接,适合在代码中频繁使用。
以下是关于如何用Python判断变量是列表还是字符串的详细内容:
一、Python的基本类型判断方法
在Python中,类型是动态的,也就是说,变量的类型是在运行时确定的。为了进行类型判断,可以使用以下几种方法:
1、使用 isinstance()
isinstance()
是Python提供的一个内置函数,可以用来判断一个变量是否是某个特定的类型。它接受两个参数,第一个是变量,第二个是类型。
variable = "Hello, World!"
if isinstance(variable, str):
print("The variable is a string.")
elif isinstance(variable, list):
print("The variable is a list.")
else:
print("The variable is neither a string nor a list.")
在上述代码中,isinstance(variable, str)
返回 True
,因此输出是 "The variable is a string."。类似地,如果 variable
是一个列表,则 isinstance(variable, list)
会返回 True
。
2、使用 type()
除了 isinstance()
,还可以使用 type()
函数来获取变量的类型。虽然 type()
也能完成类型判断,但通常不如 isinstance()
灵活。
variable = ["apple", "banana", "cherry"]
if type(variable) is str:
print("The variable is a string.")
elif type(variable) is list:
print("The variable is a list.")
else:
print("The variable is neither a string nor a list.")
尽管 type()
可以用来做类型判断,但它不考虑继承关系,因此在大多数情况下推荐使用 isinstance()
。
二、深入理解 isinstance()
和 type()
1、isinstance()
的优势
isinstance()
有几个显著的优势,使得它在大多数情况下比 type()
更适合:
-
处理继承关系:
isinstance()
可以正确识别继承关系。也就是说,如果一个类继承自另一个类,isinstance()
依旧能够正确判断其类型。 -
多类型判断:
isinstance()
可以同时判断多个类型。通过将多个类型放在一个元组中,可以一次性判断变量是否属于这些类型中的任意一个。
variable = "Hello, World!"
if isinstance(variable, (str, list)):
print("The variable is either a string or a list.")
else:
print("The variable is neither a string nor a list.")
在这个例子中,isinstance(variable, (str, list))
如果 variable
是字符串或列表中的任意一种,则返回 True
。
2、type()
的局限性
尽管 type()
也能用来判断变量的类型,但它的局限性使得它在某些情况下不如 isinstance()
实用:
-
不处理继承关系:
type()
只检查变量的直接类型,不考虑继承关系。因此,如果一个类继承自另一个类,type()
可能会返回意外的结果。 -
语法不如
isinstance()
简洁:为了检查多个类型,type()
需要重复代码,而isinstance()
可以通过元组一次性完成。
variable = ["apple", "banana", "cherry"]
if type(variable) is str or type(variable) is list:
print("The variable is either a string or a list.")
else:
print("The variable is neither a string nor a list.")
虽然可以实现相同的功能,但代码显得冗长、不够简洁。
三、实践中的类型判断
1、在函数中使用类型判断
在编写函数时,可能需要确保传入的参数是特定的类型。通过 isinstance()
可以轻松实现这一点。
def process_data(data):
if isinstance(data, str):
print("Processing string data...")
# 对字符串数据进行处理
elif isinstance(data, list):
print("Processing list data...")
# 对列表数据进行处理
else:
raise ValueError("Unsupported data type")
测试函数
process_data("Hello, World!")
process_data(["apple", "banana", "cherry"])
在这个例子中,函数 process_data
根据 data
的类型执行不同的操作。如果 data
是字符串,则处理字符串数据;如果是列表,则处理列表数据;如果是其他类型,则抛出异常。
2、在类中使用类型判断
在面向对象编程中,可以在类的方法中使用类型判断,确保方法参数是期望的类型。
class DataProcessor:
def __init__(self, data):
if not isinstance(data, (str, list)):
raise ValueError("Data must be a string or a list")
self.data = data
def process(self):
if isinstance(self.data, str):
print("Processing string data...")
# 对字符串数据进行处理
elif isinstance(self.data, list):
print("Processing list data...")
# 对列表数据进行处理
测试类
processor1 = DataProcessor("Hello, World!")
processor1.process()
processor2 = DataProcessor(["apple", "banana", "cherry"])
processor2.process()
在这个例子中,类 DataProcessor
的构造函数确保 data
是字符串或列表。如果 data
是其他类型,则抛出异常。方法 process
根据 data
的类型执行不同的处理逻辑。
四、类型判断的性能考量
在某些情况下,类型判断可能会引入性能开销。尽管 isinstance()
和 type()
都是高效的操作,但在性能关键的代码中,频繁的类型判断可能会影响性能。
1、避免频繁的类型判断
在性能关键的代码中,尽量减少类型判断的次数。可以通过提前缓存类型信息,避免在循环中重复进行类型判断。
data_list = ["apple", "banana", "cherry"]
is_list = isinstance(data_list, list)
for item in data_list:
if is_list:
# 执行与列表相关的操作
pass
在这个例子中,提前缓存了 data_list
的类型信息,避免在循环中重复调用 isinstance()
。
2、使用类型注解
Python 3.5 引入了类型注解,可以在函数和变量声明中指定类型。虽然类型注解不会在运行时强制执行,但可以通过静态类型检查工具(如 mypy
)进行类型检查,从而提高代码的可靠性。
from typing import Union
def process_data(data: Union[str, list]) -> None:
if isinstance(data, str):
print("Processing string data...")
# 对字符串数据进行处理
elif isinstance(data, list):
print("Processing list data...")
# 对列表数据进行处理
测试函数
process_data("Hello, World!")
process_data(["apple", "banana", "cherry"])
在这个例子中,函数 process_data
的参数 data
被注解为 Union[str, list]
,表示它可以是字符串或列表。虽然注解不会在运行时强制执行,但可以通过静态类型检查工具进行检查。
五、类型判断的最佳实践
1、优先使用 isinstance()
在大多数情况下,优先使用 isinstance()
进行类型判断,因为它考虑了继承关系,并且语法简洁、易读。
2、避免过度使用类型判断
尽量避免过度使用类型判断,特别是在性能关键的代码中。可以通过提前缓存类型信息或使用类型注解减少类型判断的次数。
3、利用多态性
在面向对象编程中,利用多态性可以减少类型判断的需求。通过定义通用的接口或基类,可以让不同类型的对象实现相同的方法,从而避免显式的类型判断。
class Data:
def process(self):
raise NotImplementedError("Subclasses should implement this method")
class StringData(Data):
def process(self):
print("Processing string data...")
class ListData(Data):
def process(self):
print("Processing list data...")
测试多态性
data1 = StringData()
data1.process()
data2 = ListData()
data2.process()
在这个例子中,通过定义通用的接口 Data
,不同类型的对象实现了相同的 process
方法,从而避免显式的类型判断。
六、常见的类型判断陷阱
1、误用 ==
和 is
在进行类型判断时,避免使用 ==
和 is
。它们用于比较对象的值和身份,不适用于类型判断。
variable = "Hello, World!"
if type(variable) == str:
print("The variable is a string.")
虽然上述代码可以正常工作,但不推荐使用 ==
进行类型判断。应该使用 isinstance()
或 type()
。
2、忽略继承关系
在使用 type()
进行类型判断时,可能会忽略继承关系。为了确保类型判断的正确性,优先使用 isinstance()
。
class MyList(list):
pass
variable = MyList()
if type(variable) is list:
print("The variable is a list.")
else:
print("The variable is not a list.")
在这个例子中,尽管 MyList
继承自 list
,但 type(variable) is list
返回 False
。应该使用 isinstance(variable, list)
进行类型判断。
七、总结
在Python中,类型判断是编写健壮代码的重要组成部分。通过使用 isinstance()
和 type()
,可以准确判断变量的类型。尽管 isinstance()
更加灵活、推荐使用,但在某些情况下,type()
也有其用武之地。
在编写代码时,尽量避免过度使用类型判断,特别是在性能关键的代码中。通过提前缓存类型信息、使用类型注解以及利用多态性,可以减少类型判断的次数,提高代码的可读性和性能。
无论是在函数、类还是其他代码结构中,合理地使用类型判断,可以确保代码的健壮性和可靠性。通过遵循最佳实践,避免常见的类型判断陷阱,可以编写出更加健壮、易维护的Python代码。
相关问答FAQs:
如何在Python中检查变量的类型?
要判断一个变量是列表还是字符串,可以使用内置的type()
函数。例如,type(variable) == list
会返回True如果变量是列表,type(variable) == str
则会返回True如果变量是字符串。这种方式简单直接,但使用isinstance()
函数会更灵活和推荐,因为它支持继承的情况。
使用isinstance()
函数时有哪些优势?
通过isinstance(variable, list)
和isinstance(variable, str)
,可以更准确地判断一个变量是否为指定类型,包括对子类的支持。这意味着即使变量是某个列表或字符串的子类,判断也会返回正确的结果。
如何处理变量类型不确定的情况?
在处理不确定类型的变量时,使用try-except
块是一个好的选择。可以尝试对变量进行特定操作,比如调用列表的方法或字符串的方法,如果出现AttributeError
,可以捕获异常并采取相应的措施。这样可以确保程序的稳定性并处理不同类型的变量。
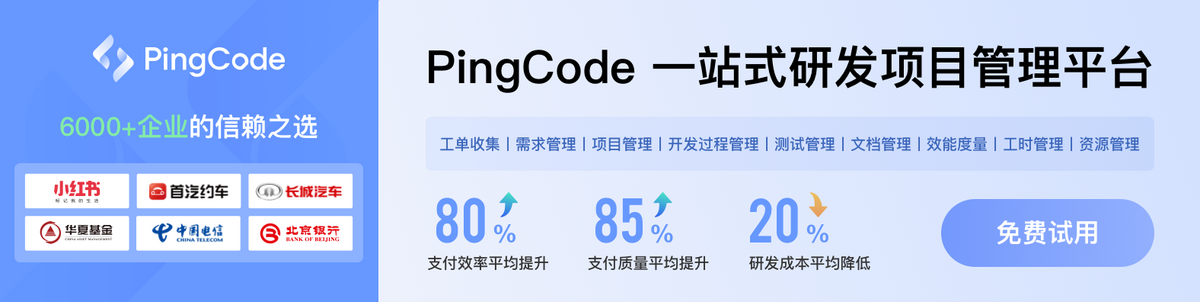