如何用Python统计四级高频词
使用Python统计四级高频词可以通过文本预处理、词频统计、数据可视化等步骤来实现。关键步骤包括:文本清洗、分词处理、词频统计、结果可视化。其中,文本清洗是整个过程中至关重要的一步,因为它直接影响后续步骤的准确性。
在详细描述文本清洗之前,我们需要了解整个过程的框架和各个步骤的具体操作。接下来,我们将详细讲解每个步骤,帮助你全面掌握用Python统计四级高频词的方法。
一、文本预处理
文本预处理是数据分析中的基础步骤,主要目的是将原始文本数据转换成适合分析的格式。这个过程通常包括去除标点符号、停用词过滤、大小写转换等。
1. 清理文本
清理文本是去除文本中无关内容的过程,这些内容可能包括标点符号、数字、HTML标签等。通过清理文本,可以减少噪音,提高词频统计的准确性。
import re
def clean_text(text):
# 去除HTML标签
text = re.sub(r'<.*?>', '', text)
# 去除标点符号和数字
text = re.sub(r'[^\w\s]', '', text)
text = re.sub(r'\d+', '', text)
# 转换为小写
text = text.lower()
return text
2. 分词处理
分词是将文本拆分成一个个单词的过程。在英文中,分词比较简单,可以直接使用空格进行拆分;在中文中,分词则需要使用专门的工具,如jieba库。
def tokenize(text):
words = text.split()
return words
3. 停用词过滤
停用词是指对文本分析没有帮助的常用词,如“the”、“is”、“and”等。这些词频率很高,但对文本的主题没有实际贡献。可以使用一个停用词列表来过滤掉这些词。
from nltk.corpus import stopwords
def remove_stopwords(words):
stop_words = set(stopwords.words('english'))
filtered_words = [word for word in words if word not in stop_words]
return filtered_words
二、词频统计
在完成文本预处理后,接下来就是统计每个单词出现的频率。可以使用Python的collections模块中的Counter类来实现这一功能。
1. 统计词频
from collections import Counter
def count_words(words):
word_counts = Counter(words)
return word_counts
2. 获取高频词
通常,我们只关心出现频率最高的若干个单词。可以使用Counter类的most_common方法来获取高频词。
def get_high_frequency_words(word_counts, n=10):
high_freq_words = word_counts.most_common(n)
return high_freq_words
三、结果可视化
为了更直观地展示高频词,可以使用一些数据可视化工具,如matplotlib、wordcloud等。这里我们介绍如何使用matplotlib绘制柱状图和wordcloud生成词云。
1. 绘制柱状图
import matplotlib.pyplot as plt
def plot_word_frequency(word_counts):
words, counts = zip(*word_counts)
plt.bar(words, counts)
plt.xlabel('Words')
plt.ylabel('Frequency')
plt.title('Top N High Frequency Words')
plt.show()
2. 生成词云
from wordcloud import WordCloud
def generate_wordcloud(word_counts):
wordcloud = WordCloud(width=800, height=400).generate_from_frequencies(dict(word_counts))
plt.figure(figsize=(10, 5))
plt.imshow(wordcloud, interpolation='bilinear')
plt.axis('off')
plt.show()
四、整合代码
将上述步骤整合成一个完整的代码示例,方便你直接运行和测试。
import re
from nltk.corpus import stopwords
from collections import Counter
import matplotlib.pyplot as plt
from wordcloud import WordCloud
def clean_text(text):
text = re.sub(r'<.*?>', '', text)
text = re.sub(r'[^\w\s]', '', text)
text = re.sub(r'\d+', '', text)
text = text.lower()
return text
def tokenize(text):
words = text.split()
return words
def remove_stopwords(words):
stop_words = set(stopwords.words('english'))
filtered_words = [word for word in words if word not in stop_words]
return filtered_words
def count_words(words):
word_counts = Counter(words)
return word_counts
def get_high_frequency_words(word_counts, n=10):
high_freq_words = word_counts.most_common(n)
return high_freq_words
def plot_word_frequency(word_counts):
words, counts = zip(*word_counts)
plt.bar(words, counts)
plt.xlabel('Words')
plt.ylabel('Frequency')
plt.title('Top N High Frequency Words')
plt.show()
def generate_wordcloud(word_counts):
wordcloud = WordCloud(width=800, height=400).generate_from_frequencies(dict(word_counts))
plt.figure(figsize=(10, 5))
plt.imshow(wordcloud, interpolation='bilinear')
plt.axis('off')
plt.show()
def main():
text = "Your text data here"
cleaned_text = clean_text(text)
words = tokenize(cleaned_text)
filtered_words = remove_stopwords(words)
word_counts = count_words(filtered_words)
high_freq_words = get_high_frequency_words(word_counts)
print("High frequency words:", high_freq_words)
plot_word_frequency(high_freq_words)
generate_wordcloud(word_counts)
if __name__ == "__main__":
main()
通过上述步骤和代码示例,你可以使用Python轻松统计四级高频词,并通过可视化工具更直观地展示统计结果。希望这篇文章对你有所帮助。
相关问答FAQs:
如何使用Python提取四级高频词的文本数据?
要提取四级高频词的文本数据,首先需要收集相关的四级考试材料,如历年真题、模拟题及词汇书等。可以通过网络爬虫技术抓取这些资料,或从教育资源网站下载。同时,确保文本数据的格式为纯文本,以便后续处理。
有哪些Python库可以帮助我进行文本分析和高频词统计?
Python中有几个非常实用的库可以帮助进行文本分析和高频词统计。常用的有nltk
(自然语言处理工具包),collections
模块中的Counter
类,以及pandas
库用于数据处理。jieba
库也可以用来进行中文分词,适合处理中文文本数据。
如何处理和清洗文本数据以提高高频词统计的准确性?
在进行高频词统计之前,文本数据的清洗至关重要。可以去除标点符号、数字和特殊字符,统一文本的大小写,删除停用词(如“的”、“是”等)以减少干扰。通过re
模块或nltk
的停用词表,可以有效地实现这些清洗步骤,从而提高高频词统计的准确性。
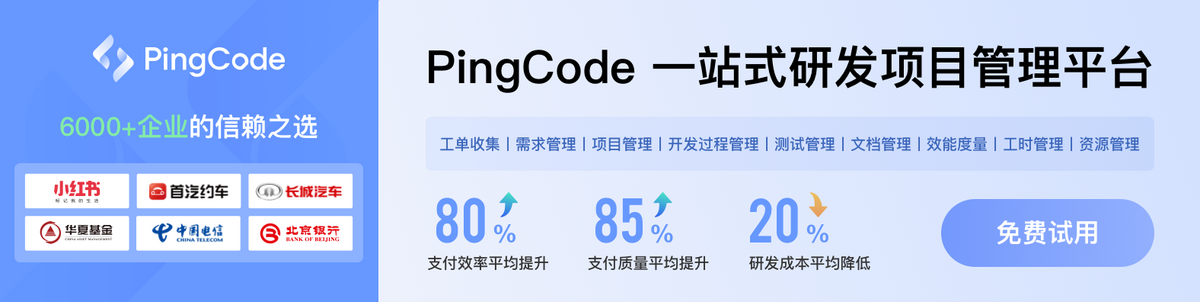