Python识别字符串大小写的方法有多种,包括使用内置方法、正则表达式等。常用的方法有:str.islower()、str.isupper()、str.lower()、str.upper()、str.capitalize()、str.title()。这些方法可以帮助开发者方便地处理和转换字符串的大小写格式。 其中,最常用的方法是str.lower()和str.upper(),因为它们可以将字符串全部转换为小写或大写,便于进行统一的字符串比较和处理。
下面将详细介绍Python中识别和处理字符串大小写的各种方法和技巧,并结合实际应用场景进行深入分析。
一、Python字符串大小写识别方法
1. str.islower() 和 str.isupper()
str.islower() 方法用于判断字符串中所有字符是否都是小写字母。如果字符串中包含非字母字符,如数字或特殊符号,则仍然返回True,只要这些非字母字符不妨碍小写字母的存在。
example_str = "hello world"
print(example_str.islower()) # 输出: True
example_str2 = "Hello World"
print(example_str2.islower()) # 输出: False
str.isupper() 方法则用于判断字符串中所有字符是否都是大写字母。与islower()类似,只要字符串中所有字母都是大写,其他非字母字符不会影响结果。
example_str = "HELLO WORLD"
print(example_str.isupper()) # 输出: True
example_str2 = "Hello World"
print(example_str2.isupper()) # 输出: False
2. str.lower() 和 str.upper()
str.lower() 方法将字符串中的所有字母转换为小写,非常适合在需要统一格式进行字符串比较时使用。
example_str = "Hello World"
lower_str = example_str.lower()
print(lower_str) # 输出: "hello world"
str.upper() 方法与str.lower()类似,将字符串中的所有字母转换为大写。
example_str = "Hello World"
upper_str = example_str.upper()
print(upper_str) # 输出: "HELLO WORLD"
二、实际应用场景中的字符串大小写处理
1. 用户输入统一格式化
在开发用户输入处理程序时,常常需要对用户输入的字符串进行格式化,以便进行一致性比较。例如,验证用户输入的邮箱地址时,可以将输入的邮箱地址全部转换为小写,以避免由于大小写不同而导致的验证失败。
email_input = "User@Example.Com"
formatted_email = email_input.lower()
print(formatted_email) # 输出: "user@example.com"
2. 标题和句子格式化
在处理标题和句子时,通常需要将每个单词的首字母大写。可以使用str.capitalize()和str.title()方法来完成这项任务。
str.capitalize() 方法将字符串的首字母转换为大写,其余字母转换为小写。
sentence = "hello world"
capitalized_sentence = sentence.capitalize()
print(capitalized_sentence) # 输出: "Hello world"
str.title() 方法将字符串中的每个单词的首字母都转换为大写,其余字母转换为小写。
title = "hello world"
titled_title = title.title()
print(titled_title) # 输出: "Hello World"
三、结合正则表达式进行高级处理
Python中的正则表达式模块re提供了更多高级的字符串处理功能,可以结合正则表达式对字符串的大小写进行更精细的控制。
1. 使用正则表达式匹配大小写
通过使用正则表达式,可以匹配并替换字符串中的特定大小写模式。例如,匹配所有大写字母并将其转换为小写。
import re
example_str = "HELLO World"
lower_str = re.sub(r'[A-Z]', lambda x: x.group().lower(), example_str)
print(lower_str) # 输出: "hello World"
2. 条件替换
结合正则表达式的条件替换功能,可以在特定条件下对字符串进行大小写转换。例如,仅将以特定字符开头的单词转换为大写。
import re
example_str = "hello world, this is a test"
modified_str = re.sub(r'\bthis\b', lambda x: x.group().upper(), example_str)
print(modified_str) # 输出: "hello world, THIS is a test"
四、性能优化和注意事项
在处理大量字符串时,性能是一个需要考虑的重要因素。下面是一些性能优化的建议和注意事项:
1. 批量处理字符串
如果需要对大量字符串进行相同的大小写转换,可以考虑批量处理,而不是逐个处理。
strings = ["Hello World", "Python Programming", "Data Science"]
lower_strings = [s.lower() for s in strings]
print(lower_strings) # 输出: ['hello world', 'python programming', 'data science']
2. 避免不必要的转换
在进行字符串比较时,尽量避免不必要的大小写转换。可以先判断字符串是否已经符合要求,再进行转换。
example_str = "Hello World"
if not example_str.islower():
example_str = example_str.lower()
print(example_str) # 输出: "hello world"
五、总结
Python提供了丰富的字符串大小写处理方法,包括str.islower()、str.isupper()、str.lower()、str.upper()、str.capitalize()、str.title()等。通过合理使用这些方法,可以方便地识别和转换字符串的大小写,满足不同的应用需求。在实际开发中,结合正则表达式和性能优化技巧,可以进一步提高字符串处理的效率和灵活性。无论是处理用户输入、格式化标题和句子,还是进行高级的字符串匹配和替换,Python的字符串处理功能都能提供强大的支持。
相关问答FAQs:
如何在Python中检查一个字符串是否是全大写?
在Python中,可以使用字符串的isupper()
方法来检查字符串是否全为大写字母。此方法会返回一个布尔值,若字符串中的所有字母都是大写,返回True
,否则返回False
。例如:
text = "HELLO"
is_upper = text.isupper() # 返回 True
Python中如何将字符串转换为小写?
要将字符串转换为小写,可以使用lower()
方法。这个方法会返回一个新的字符串,其中所有的大写字母都被转换为小写。示例代码如下:
text = "Hello World"
lowercase_text = text.lower() # 结果为 "hello world"
怎样判断一个字符串中包含的字母是混合大小写?
可以结合使用isupper()
和islower()
方法来判断字符串是否包含混合大小写。具体来说,如果字符串既有大写字母也有小写字母,且不是全大写或全小写,就可以认为是混合大小写。示例代码如下:
text = "Hello World"
is_mixed = not text.isupper() and not text.islower() # 返回 True
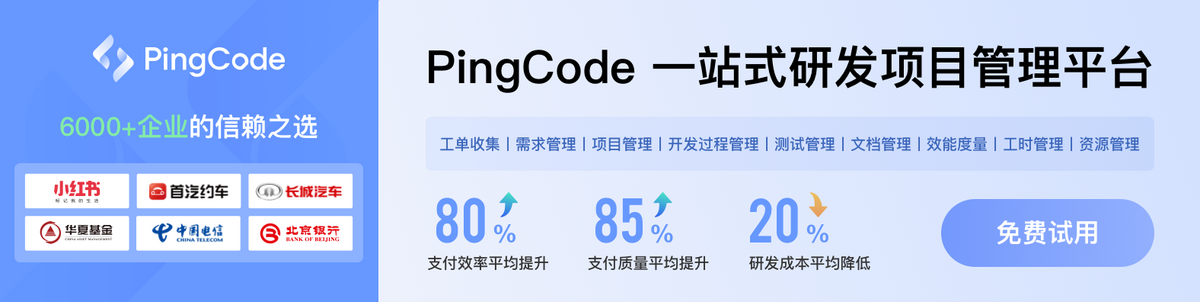