如何用Python语言编写两点之间的距离
使用Python语言编写两点之间的距离可以通过欧几里得距离公式、曼哈顿距离公式、Scipy库、Numpy库等方法来实现。本文将详细介绍这几种方法,并提供示例代码。欧几里得距离公式是最常用的一种方法,它计算两个点在二维平面上的直线距离。以下是详细描述:
欧几里得距离公式
欧几里得距离是最直观的距离度量方法,通过两点的坐标差的平方和再开平方根得到。其公式为:
[ d = \sqrt{(x_2 – x_1)^2 + (y_2 – y_1)^2} ]
在Python中,可以通过基本的数学运算来实现这一公式。下面是一个简单的示例代码:
import math
def euclidean_distance(point1, point2):
return math.sqrt((point2[0] - point1[0])<strong>2 + (point2[1] - point1[1])</strong>2)
point1 = (1, 2)
point2 = (4, 6)
distance = euclidean_distance(point1, point2)
print(f"Euclidean Distance: {distance}")
一、欧几里得距离公式
1、基本数学计算
通过基本的数学运算来计算欧几里得距离,如前文所示,利用Python的math库实现平方和开平方操作。这个方法非常直接且易于理解,但当处理高维空间时,代码会变得复杂。因此,使用Numpy库会更方便。
import math
def euclidean_distance(point1, point2):
return math.sqrt((point2[0] - point1[0])<strong>2 + (point2[1] - point1[1])</strong>2)
point1 = (1, 2)
point2 = (4, 6)
distance = euclidean_distance(point1, point2)
print(f"Euclidean Distance: {distance}")
2、使用Numpy库
Numpy库是Python中处理数组和矩阵的标准库,其提供了丰富的数学函数,可以大大简化计算。使用Numpy库计算欧几里得距离可以处理更高维度的数据,并且代码更加简洁。
import numpy as np
def euclidean_distance(point1, point2):
point1 = np.array(point1)
point2 = np.array(point2)
return np.linalg.norm(point1 - point2)
point1 = (1, 2)
point2 = (4, 6)
distance = euclidean_distance(point1, point2)
print(f"Euclidean Distance using Numpy: {distance}")
二、曼哈顿距离公式
曼哈顿距离是另一种常用的距离度量方法,它计算两个点在坐标系上的绝对距离。其公式为:
[ d = |x_2 – x_1| + |y_2 – y_1| ]
这种距离度量在很多实际场景中,比如城市街区的距离计算中,比欧几里得距离更适用。以下是其实现代码:
1、基本数学计算
def manhattan_distance(point1, point2):
return abs(point2[0] - point1[0]) + abs(point2[1] - point1[1])
point1 = (1, 2)
point2 = (4, 6)
distance = manhattan_distance(point1, point2)
print(f"Manhattan Distance: {distance}")
2、使用Numpy库
同样地,我们可以使用Numpy库来简化曼哈顿距离的计算:
import numpy as np
def manhattan_distance(point1, point2):
point1 = np.array(point1)
point2 = np.array(point2)
return np.sum(np.abs(point1 - point2))
point1 = (1, 2)
point2 = (4, 6)
distance = manhattan_distance(point1, point2)
print(f"Manhattan Distance using Numpy: {distance}")
三、使用Scipy库计算距离
Scipy库提供了更丰富的距离计算方法,包括欧几里得距离和曼哈顿距离,以及其他高维空间的距离度量。使用Scipy库可以大大简化代码,并且提供了更高的灵活性。
1、计算欧几里得距离
Scipy库的spatial.distance
模块提供了直接计算欧几里得距离的函数:
from scipy.spatial import distance
point1 = (1, 2)
point2 = (4, 6)
distance = distance.euclidean(point1, point2)
print(f"Euclidean Distance using Scipy: {distance}")
2、计算曼哈顿距离
同样地,Scipy库也提供了计算曼哈顿距离的函数:
from scipy.spatial import distance
point1 = (1, 2)
point2 = (4, 6)
distance = distance.cityblock(point1, point2)
print(f"Manhattan Distance using Scipy: {distance}")
四、其他距离计算方法
除了欧几里得距离和曼哈顿距离,Scipy库还提供了其他距离计算方法,如切比雪夫距离、闵可夫斯基距离等。这些方法在不同的应用场景中有不同的适用性。
1、切比雪夫距离
切比雪夫距离用于计算两个点之间的最大坐标差。其公式为:
[ d = \max(|x_2 – x_1|, |y_2 – y_1|) ]
Scipy库中的实现如下:
from scipy.spatial import distance
point1 = (1, 2)
point2 = (4, 6)
distance = distance.chebyshev(point1, point2)
print(f"Chebyshev Distance using Scipy: {distance}")
2、闵可夫斯基距离
闵可夫斯基距离是欧几里得距离和曼哈顿距离的广义形式,可以通过调节参数p来改变距离度量的方式。其公式为:
[ d = \left( \sum_{i=1}^{n} |x_i – y_i|^p \right)^{1/p} ]
Scipy库中的实现如下:
from scipy.spatial import distance
point1 = (1, 2)
point2 = (4, 6)
distance = distance.minkowski(point1, point2, p=3)
print(f"Minkowski Distance using Scipy with p=3: {distance}")
总结
通过上述方法,我们可以在Python中轻松实现两点之间的距离计算。欧几里得距离适用于大多数情况,曼哈顿距离适用于城市街区等特定场景,Scipy库提供了丰富的距离计算方法,适用于更复杂的高维空间计算。选择合适的方法可以提高计算效率和代码简洁性。希望本文对您理解和实现两点之间的距离计算有所帮助。
相关问答FAQs:
如何计算两点之间的距离?
计算两点之间的距离可以使用欧几里得距离公式。具体来说,如果有两点A(x1, y1)和B(x2, y2),可以通过以下公式计算距离:
[ \text{距离} = \sqrt{(x2 – x1)^2 + (y2 – y1)^2} ]
在Python中,可以使用math
库中的sqrt
函数来实现这个计算。
在Python中如何实现这个计算?
可以通过定义一个函数来实现两点距离的计算。以下是一个简单的示例代码:
import math
def calculate_distance(point1, point2):
return math.sqrt((point2[0] - point1[0])<strong>2 + (point2[1] - point1[1])</strong>2)
# 示例
distance = calculate_distance((1, 2), (4, 6))
print(distance)
这种方法很方便,能够快速计算任意两个点之间的距离。
是否可以使用其他库来计算距离?
是的,Python中有多个库可以简化距离计算,比如NumPy和SciPy。使用NumPy的numpy.linalg.norm
方法可以更高效地计算距离。以下是一个示例:
import numpy as np
point1 = np.array([1, 2])
point2 = np.array([4, 6])
distance = np.linalg.norm(point2 - point1)
print(distance)
这种方式特别适合需要处理大量数据的情况,性能更优。
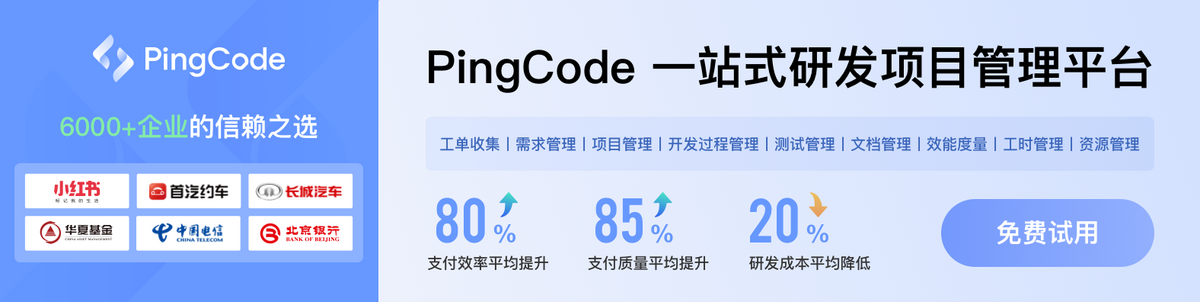