在构建一个搜索引擎时,Python是一种非常强大的编程语言。它不仅拥有丰富的库和框架,还能通过其简洁的语法和强大的功能,帮助开发者快速实现复杂的功能。构建一个搜索引擎的核心步骤包括:数据收集、数据处理、索引创建、查询处理、用户界面设计等。 其中,数据收集是最基础且最关键的一环,它直接决定了搜索引擎的覆盖范围和数据质量。
一、数据收集
数据收集,也称为Web抓取或爬取,是搜索引擎的第一步。它涉及从互联网上收集大量信息,并将其存储在数据库中以供后续处理。
1、使用Scrapy进行Web抓取
Scrapy是一个强大的Python库,专门用于Web抓取。它提供了丰富的功能,可以轻松地从网页中提取数据。
import scrapy
class MySpider(scrapy.Spider):
name = 'my_spider'
start_urls = ['http://example.com']
def parse(self, response):
for title in response.css('title::text').extract():
yield {'title': title}
for next_page in response.css('a::attr(href)').extract():
if next_page is not None:
yield response.follow(next_page, self.parse)
2、数据存储
抓取的数据需要存储在一个数据库中,以便后续处理。常用的数据库包括MySQL、PostgreSQL、MongoDB等。
import pymongo
client = pymongo.MongoClient("mongodb://localhost:27017/")
db = client["search_engine"]
collection = db["web_data"]
插入数据
collection.insert_one({"title": "Example Title", "url": "http://example.com"})
二、数据处理
数据处理是指对收集到的原始数据进行清洗、格式化和预处理,以便于后续的索引和搜索。
1、数据清洗
数据清洗是指去除无用信息,如HTML标签、脚本、样式等,只保留有用的文本信息。
from bs4 import BeautifulSoup
html_content = "<html><head><title>Example</title></head><body><p>Some text.</p></body></html>"
soup = BeautifulSoup(html_content, 'html.parser')
提取纯文本
text = soup.get_text()
2、词干提取和去停用词
词干提取和去停用词是自然语言处理中的常见步骤,用于减少词汇的多样性,提高搜索的准确性。
from nltk.corpus import stopwords
from nltk.stem import PorterStemmer
from nltk.tokenize import word_tokenize
停用词
stop_words = set(stopwords.words('english'))
词干提取
ps = PorterStemmer()
words = word_tokenize(text)
processed_words = [ps.stem(word) for word in words if word not in stop_words]
三、索引创建
索引创建是指将处理后的数据存储在一个高效的数据结构中,以便于快速搜索。
1、倒排索引
倒排索引是一种常用的数据结构,用于快速全文搜索。它将每个词映射到包含该词的文档集合。
from collections import defaultdict
inverted_index = defaultdict(list)
for doc_id, text in enumerate(processed_texts):
for word in text.split():
inverted_index[word].append(doc_id)
2、使用Whoosh创建索引
Whoosh是一个纯Python编写的全文搜索库,可以方便地创建和查询索引。
from whoosh.index import create_in
from whoosh.fields import Schema, TEXT, ID
from whoosh.qparser import QueryParser
定义索引架构
schema = Schema(title=TEXT(stored=True), content=TEXT, url=ID(stored=True))
创建索引
index_dir = "indexdir"
if not os.path.exists(index_dir):
os.mkdir(index_dir)
ix = create_in(index_dir, schema)
添加文档
writer = ix.writer()
writer.add_document(title="Example Title", content="Some example text.", url="http://example.com")
writer.commit()
查询索引
with ix.searcher() as searcher:
query = QueryParser("content", ix.schema).parse("example")
results = searcher.search(query)
for result in results:
print(result['title'], result['url'])
四、查询处理
查询处理是指解析用户输入的查询,并根据索引找到匹配的文档。
1、查询解析
查询解析是指将用户输入的查询转换为可以在索引中搜索的格式。
query = "example query"
processed_query = [ps.stem(word) for word in word_tokenize(query) if word not in stop_words]
2、查询匹配
查询匹配是指在索引中找到与查询匹配的文档。
matched_docs = set()
for word in processed_query:
if word in inverted_index:
matched_docs.update(inverted_index[word])
五、用户界面设计
用户界面设计是指为搜索引擎创建一个友好的用户界面,使用户可以方便地输入查询并查看搜索结果。
1、使用Flask创建Web界面
Flask是一个轻量级的Python Web框架,可以方便地创建Web应用。
from flask import Flask, request, render_template
app = Flask(__name__)
@app.route('/', methods=['GET', 'POST'])
def index():
if request.method == 'POST':
query = request.form['query']
results = search(query)
return render_template('results.html', query=query, results=results)
return render_template('index.html')
def search(query):
processed_query = [ps.stem(word) for word in word_tokenize(query) if word not in stop_words]
matched_docs = set()
for word in processed_query:
if word in inverted_index:
matched_docs.update(inverted_index[word])
# 返回匹配的文档
return [{'title': "Example Title", 'url': "http://example.com"}]
if __name__ == '__main__':
app.run(debug=True)
2、前端设计
使用HTML和CSS设计用户界面,使其美观且易于使用。
<!-- index.html -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Search Engine</title>
<style>
body { font-family: Arial, sans-serif; }
.search-box { margin: 50px auto; max-width: 600px; }
.search-box input[type="text"] { width: 80%; padding: 10px; }
.search-box input[type="submit"] { padding: 10px 20px; }
</style>
</head>
<body>
<div class="search-box">
<form method="post">
<input type="text" name="query" placeholder="Enter your search query">
<input type="submit" value="Search">
</form>
</div>
</body>
</html>
<!-- results.html -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Search Results</title>
<style>
body { font-family: Arial, sans-serif; }
.results { margin: 50px auto; max-width: 600px; }
.result-item { margin-bottom: 20px; }
</style>
</head>
<body>
<div class="results">
<h1>Search Results for "{{ query }}"</h1>
{% for result in results %}
<div class="result-item">
<h2><a href="{{ result['url'] }}">{{ result['title'] }}</a></h2>
</div>
{% endfor %}
</div>
</body>
</html>
六、性能优化
为了使搜索引擎在处理大规模数据时保持高效,性能优化是必不可少的。
1、缓存
使用缓存可以显著提高查询速度,特别是对于频繁查询的内容。
from cachetools import LRUCache
cache = LRUCache(maxsize=100)
def search(query):
if query in cache:
return cache[query]
# 执行实际的查询
results = perform_search(query)
cache[query] = results
return results
2、多线程和分布式处理
对于大规模数据,可以使用多线程和分布式处理来提高数据抓取和处理的效率。
import threading
def fetch_data(url):
# 执行数据抓取操作
pass
urls = ['http://example.com/page1', 'http://example.com/page2']
threads = [threading.Thread(target=fetch_data, args=(url,)) for url in urls]
for thread in threads:
thread.start()
for thread in threads:
thread.join()
构建一个搜索引擎涉及多个复杂的步骤和技术,但Python提供了丰富的工具和库,可以帮助我们高效地实现这一目标。通过数据收集、数据处理、索引创建、查询处理、用户界面设计和性能优化,我们可以构建一个功能强大且高效的搜索引擎。
相关问答FAQs:
如何开始使用Python构建一个简单的搜索引擎?
构建一个简单的搜索引擎可以从几个基本步骤入手:首先,您需要选择一个数据源,这可以是网页、文档或数据库。接下来,使用Python的爬虫库(如Scrapy或Beautiful Soup)抓取数据。然后,利用文本处理库(如NLTK或spaCy)进行数据清洗和索引构建。最后,可以使用Flask或Django等框架开发一个前端界面,供用户进行搜索。
在Python中实现搜索引擎时需要注意哪些性能问题?
性能是搜索引擎设计中的关键因素。应考虑使用合适的数据结构来存储索引,比如倒排索引,以提高搜索速度。此外,使用缓存机制可以加快重复查询的响应时间。优化查询算法和减少不必要的计算也是提升性能的有效方法。
是否有现成的Python库可以帮助我创建搜索引擎?
是的,Python有一些强大的库可以帮助您创建搜索引擎。例如,Whoosh是一个纯Python实现的搜索引擎库,适合小型项目;Elasticsearch是一个分布式搜索引擎,适合处理大规模数据。使用这些库可以节省开发时间,同时提供强大的搜索功能。
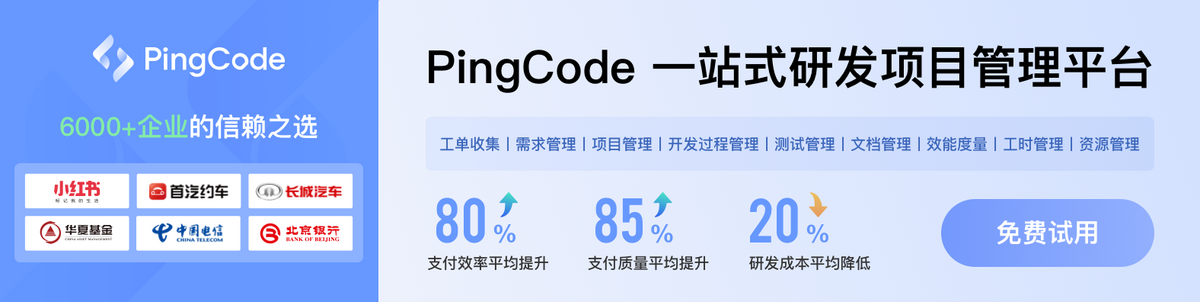