如何在Python中同时输入多个数据库
要在Python中同时输入多个数据库,你可以使用多线程、多进程、连接池、ORM(如SQLAlchemy)等方法。其中,使用SQLAlchemy的连接池功能是一种高效且灵活的方法,因为它支持多种数据库类型,并能自动管理连接。以下将详细介绍使用SQLAlchemy连接池的方法。
一、安装和配置SQLAlchemy
安装SQLAlchemy
首先,你需要安装SQLAlchemy。如果还没有安装,可以通过以下命令进行安装:
pip install SQLAlchemy
此外,如果你需要连接特定类型的数据库,还需要安装对应的数据库驱动。例如:
pip install psycopg2-binary # PostgreSQL
pip install mysql-connector-python # MySQL
pip install cx_Oracle # Oracle
配置连接
在开始编写代码之前,需配置数据库连接信息。假设你有两个数据库,一个是PostgreSQL,另一个是MySQL。以下是连接字符串的示例:
from sqlalchemy import create_engine
PostgreSQL
postgresql_engine = create_engine('postgresql+psycopg2://user:password@host:port/dbname')
MySQL
mysql_engine = create_engine('mysql+mysqlconnector://user:password@host:port/dbname')
二、使用SQLAlchemy连接池
SQLAlchemy的连接池功能可以帮助你高效地管理多个数据库连接。
创建连接池
你可以通过create_engine
函数创建连接池。以下是创建连接池的示例:
from sqlalchemy.orm import sessionmaker
PostgreSQL
postgresql_engine = create_engine('postgresql+psycopg2://user:password@host:port/dbname', pool_size=10, max_overflow=20)
PostgresqlSession = sessionmaker(bind=postgresql_engine)
MySQL
mysql_engine = create_engine('mysql+mysqlconnector://user:password@host:port/dbname', pool_size=10, max_overflow=20)
MysqlSession = sessionmaker(bind=mysql_engine)
使用连接池
使用连接池时,可以通过sessionmaker
创建会话(session),并在会话中进行数据库操作。以下是使用示例:
# PostgreSQL
postgresql_session = PostgresqlSession()
postgresql_result = postgresql_session.execute("SELECT * FROM my_table")
for row in postgresql_result:
print(row)
MySQL
mysql_session = MysqlSession()
mysql_result = mysql_session.execute("SELECT * FROM my_table")
for row in mysql_result:
print(row)
关闭会话
postgresql_session.close()
mysql_session.close()
三、多线程和多进程
在处理多个数据库时,多线程和多进程也是一种有效的方法。Python的threading
和multiprocessing
模块可以帮助你实现这一点。
多线程
以下是使用多线程的示例:
import threading
def query_postgresql():
postgresql_session = PostgresqlSession()
result = postgresql_session.execute("SELECT * FROM my_table")
for row in result:
print(row)
postgresql_session.close()
def query_mysql():
mysql_session = MysqlSession()
result = mysql_session.execute("SELECT * FROM my_table")
for row in result:
print(row)
mysql_session.close()
创建线程
thread1 = threading.Thread(target=query_postgresql)
thread2 = threading.Thread(target=query_mysql)
启动线程
thread1.start()
thread2.start()
等待所有线程完成
thread1.join()
thread2.join()
多进程
以下是使用多进程的示例:
import multiprocessing
def query_postgresql():
postgresql_session = PostgresqlSession()
result = postgresql_session.execute("SELECT * FROM my_table")
for row in result:
print(row)
postgresql_session.close()
def query_mysql():
mysql_session = MysqlSession()
result = mysql_session.execute("SELECT * FROM my_table")
for row in result:
print(row)
mysql_session.close()
创建进程
process1 = multiprocessing.Process(target=query_postgresql)
process2 = multiprocessing.Process(target=query_mysql)
启动进程
process1.start()
process2.start()
等待所有进程完成
process1.join()
process2.join()
四、ORM(对象关系映射)
使用ORM可以简化数据库操作,通过定义模型类来映射数据库表。SQLAlchemy提供了强大的ORM功能。
定义模型
以下是定义模型的示例:
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy import Column, Integer, String
Base = declarative_base()
class MyTable(Base):
__tablename__ = 'my_table'
id = Column(Integer, primary_key=True)
name = Column(String)
使用模型进行查询
你可以通过会话使用模型类进行查询:
# PostgreSQL
postgresql_session = PostgresqlSession()
postgresql_result = postgresql_session.query(MyTable).all()
for row in postgresql_result:
print(row.name)
MySQL
mysql_session = MysqlSession()
mysql_result = mysql_session.query(MyTable).all()
for row in mysql_result:
print(row.name)
关闭会话
postgresql_session.close()
mysql_session.close()
五、总结
在Python中同时输入多个数据库并不是一件复杂的事情。通过使用SQLAlchemy的连接池、多线程、多进程和ORM功能,可以高效地管理和操作多个数据库。选择合适的方法和工具将帮助你更好地应对多数据库环境中的各种挑战。希望本文能为你提供有价值的参考和帮助。
相关问答FAQs:
如何在Python中同时连接多个数据库?
在Python中,可以使用不同的数据库连接库来连接多个数据库。首先,确保安装了所需的库,例如pymysql
用于MySQL,psycopg2
用于PostgreSQL等。接着,可以使用不同的连接对象分别连接到不同的数据库。通过创建多个连接实例,你能够在一个脚本中同时与多个数据库进行交互。
在连接多个数据库时,有哪些最佳实践?
在同时连接多个数据库时,确保使用适当的连接池管理,避免频繁的连接和断开操作。可以使用SQLAlchemy
等ORM工具来简化数据库操作和连接管理。此外,使用上下文管理器来自动处理连接的打开和关闭,能够提高代码的清晰度和可维护性。
如何在Python中处理多个数据库的查询结果?
处理多个数据库的查询结果时,可以将每个数据库的查询结果存储在不同的变量中。建议使用字典或列表来管理这些结果,便于后续的数据处理和分析。使用pandas
库可以进一步优化数据的处理,能够轻松地将查询结果转化为DataFrame进行分析和可视化。
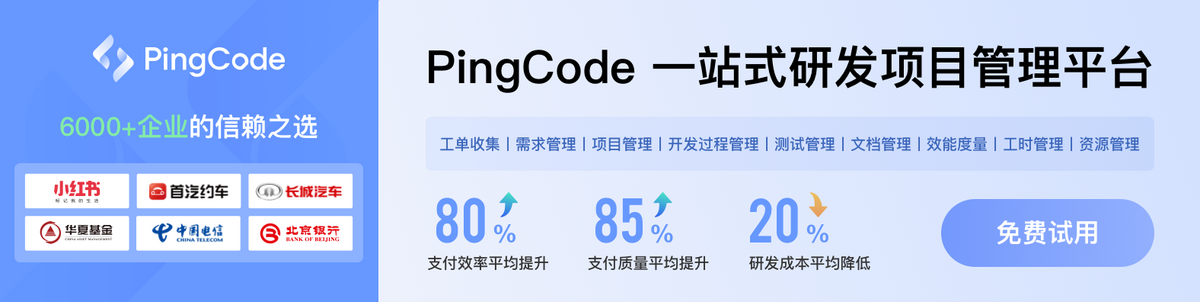