通过Python查看虚拟机eth0的网关,您可以使用 subprocess 模块、读取系统文件、利用 netifaces 库等方法。其中,subprocess 模块最为常用,因为它可以执行系统命令并获取输出结果。下面将详细介绍如何使用 subprocess 模块查看虚拟机eth0的网关。
一、使用subprocess模块
概述
subprocess模块是Python标准库中的一个模块,用于生成新的进程、连接其输入/输出/错误管道并获取其返回码。通过调用系统命令,我们可以获取到eth0接口的网关信息。
示例代码
import subprocess
def get_gateway(interface='eth0'):
try:
result = subprocess.run(['ip', 'route', 'show', 'dev', interface],
stdout=subprocess.PIPE, text=True, check=True)
output = result.stdout
for line in output.split('\n'):
if 'default' in line:
return line.split()[2]
except subprocess.CalledProcessError as e:
print(f"Command failed with {e.returncode}")
except Exception as e:
print(f"An error occurred: {e}")
gateway = get_gateway()
print(f"The gateway for eth0 is: {gateway}")
二、使用读取系统文件的方法
概述
在Linux系统中,网络接口的配置信息通常存储在 /proc
文件系统中。可以通过读取 /proc/net/route
文件来获取网关信息。
示例代码
def get_gateway_from_proc(interface='eth0'):
try:
with open('/proc/net/route') as file:
for line in file:
fields = line.strip().split()
if fields[0] == interface and fields[1] == '00000000':
return '.'.join([str(int(fields[2][i:i+2], 16)) for i in range(0, 8, 2)])
except Exception as e:
print(f"An error occurred: {e}")
gateway = get_gateway_from_proc()
print(f"The gateway for eth0 is: {gateway}")
三、使用netifaces库
概述
netifaces是一个Python库,用于跨平台获取网络接口的地址、网关等信息。它可以简化获取网关的过程。
安装netifaces库
pip install netifaces
示例代码
import netifaces as ni
def get_gateway_with_netifaces(interface='eth0'):
try:
gateways = ni.gateways()
return gateways['default'][ni.AF_INET][0]
except KeyError:
print(f"No gateway found for interface {interface}")
except Exception as e:
print(f"An error occurred: {e}")
gateway = get_gateway_with_netifaces()
print(f"The gateway for eth0 is: {gateway}")
四、总结
通过上述三种方法,您可以方便地在Python中查看虚拟机eth0的网关。其中,使用subprocess模块执行系统命令是一种通用且灵活的方法;读取系统文件的方法适合在Linux环境下直接访问系统信息;使用netifaces库则提供了一种跨平台的解决方案。根据具体需求和环境,您可以选择合适的方法来实现。
在实际应用中,选择适合的方法尤为重要。例如,subprocess模块在需要执行复杂系统命令时非常有用,而netifaces库则在需要跨平台兼容性时更为便利。通过合理利用这些工具,您可以高效地获取网络接口的配置信息,为进一步的网络编程打下坚实的基础。
相关问答FAQs:
如何在Python中获取虚拟机eth0的网关信息?
要在Python中获取虚拟机eth0的网关信息,可以使用os
模块或subprocess
模块。通过执行命令如ip route
或route -n
,可以提取网关地址。以下是一个示例代码:
import subprocess
def get_gateway():
result = subprocess.run(['ip', 'route'], stdout=subprocess.PIPE, text=True)
for line in result.stdout.splitlines():
if 'default' in line:
return line.split()[2]
return None
gateway = get_gateway()
print(f'eth0的网关是: {gateway}')
在Linux系统中,如何手动检查eth0的网关?
在Linux系统中,打开终端并输入命令ip route
或route -n
。这将显示网络路由表,其中会列出默认路由(通常是网关地址)。查找以“default”开头的行,网关地址通常在第二列。
为什么我在虚拟机中获取不到eth0的网关信息?
如果无法获取eth0的网关信息,可能是由于虚拟机的网络配置不正确或虚拟机未连接到网络。请检查虚拟机的网络适配器设置,确保其处于正确的网络模式(如桥接模式或NAT模式),并确认网络服务已启用。
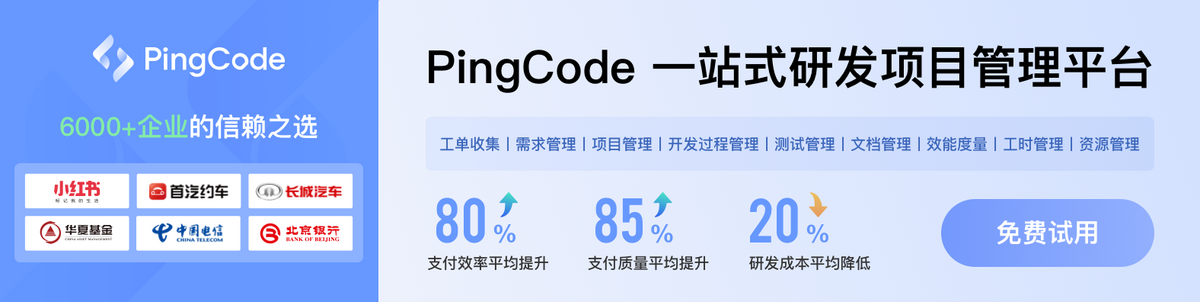