Python绘图中显示点的坐标系有几种方法:使用Matplotlib的annotate函数、使用text函数、以及使用交互式工具如mplcursors。 在这些方法中,最常用和灵活的是annotate
函数,因为它提供了丰富的选项来定制注释的外观和位置。
使用Matplotlib的annotate
函数,我们可以在图中添加注释来标示点的坐标。annotate
函数允许我们指定注释文本的内容、位置和样式,使得我们能够在绘图时精确地传达所需的信息。例如,我们可以通过annotate
函数在散点图中标注每个点的坐标,以便在数据可视化时更清晰地了解每个数据点的位置。
一、安装和导入必要的库
在开始绘图之前,我们需要确保已安装Matplotlib库。可以使用以下命令安装:
pip install matplotlib
安装完成后,导入库:
import matplotlib.pyplot as plt
二、使用Matplotlib的annotate函数
1. 创建基本的散点图
首先,我们创建一个简单的散点图。
import matplotlib.pyplot as plt
示例数据
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
创建散点图
plt.scatter(x, y)
显示图形
plt.show()
2. 使用annotate函数标注点的坐标
接下来,我们使用annotate
函数在图中显示每个点的坐标。
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
plt.scatter(x, y)
添加注释
for i in range(len(x)):
plt.annotate(f'({x[i]}, {y[i]})', (x[i], y[i]), textcoords="offset points", xytext=(0,10), ha='center')
plt.show()
在上述代码中,我们遍历每个点,并使用plt.annotate
函数在每个点的上方偏移10个像素的位置添加注释。
三、使用Matplotlib的text函数
text
函数是另一种在图中添加文本注释的方法。
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
plt.scatter(x, y)
使用text函数添加注释
for i in range(len(x)):
plt.text(x[i], y[i], f'({x[i]}, {y[i]})', fontsize=9, ha='right')
plt.show()
四、使用交互式工具mplcursors
mplcursors是一个用于Matplotlib的交互式注释库,能够动态显示点的坐标。
1. 安装mplcursors
pip install mplcursors
2. 使用mplcursors显示点的坐标
import matplotlib.pyplot as plt
import mplcursors
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
plt.scatter(x, y)
使用mplcursors动态显示坐标
mplcursors.cursor(hover=True)
plt.show()
五、定制注释的样式和位置
1. 自定义注释的外观
使用annotate
函数时,我们可以通过设置多个参数来自定义注释的外观。
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
plt.scatter(x, y)
自定义注释样式
for i in range(len(x)):
plt.annotate(f'({x[i]}, {y[i]})', (x[i], y[i]), textcoords="offset points", xytext=(0,10), ha='center',
bbox=dict(boxstyle="round,pad=0.3", edgecolor="red", facecolor="yellow", alpha=0.5),
arrowprops=dict(arrowstyle="->", color="blue"))
plt.show()
在上述代码中,我们使用bbox
参数来设置注释框的样式,并使用arrowprops
参数来设置箭头的样式。
2. 动态更新注释
在某些情况下,我们可能需要在图形更新时动态更新注释。可以通过事件处理来实现这一点。
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
fig, ax = plt.subplots()
sc = plt.scatter(x, y)
初始注释
annot = ax.annotate("", xy=(0,0), xytext=(20,20), textcoords="offset points",
bbox=dict(boxstyle="round", fc="w"),
arrowprops=dict(arrowstyle="->"))
annot.set_visible(False)
def update_annot(ind):
pos = sc.get_offsets()[ind["ind"][0]]
annot.xy = pos
text = f"{pos[0]}, {pos[1]}"
annot.set_text(text)
annot.get_bbox_patch().set_alpha(0.4)
def hover(event):
vis = annot.get_visible()
if event.inaxes == ax:
cont, ind = sc.contains(event)
if cont:
update_annot(ind)
annot.set_visible(True)
fig.canvas.draw_idle()
else:
if vis:
annot.set_visible(False)
fig.canvas.draw_idle()
fig.canvas.mpl_connect("motion_notify_event", hover)
plt.show()
在上述代码中,我们定义了一个update_annot
函数来更新注释,并通过hover
事件处理器在鼠标悬停时动态更新注释内容。
六、总结
在Python中显示图中点的坐标系主要有几种方法,包括使用Matplotlib的annotate
函数、text
函数以及交互式工具如mplcursors。annotate
函数提供了丰富的选项来定制注释的外观和位置,使其成为最常用的方法。通过这些方法,我们可以在数据可视化中更加清晰地传达每个数据点的信息,提高图形的可读性和专业性。
相关问答FAQs:
如何在Python绘图中显示坐标点的具体数值?
在Python绘图中,可以使用matplotlib
库来显示坐标点的具体数值。通过annotate
函数,可以在图中添加文本标注,显示每个点的坐标。例如,使用plt.annotate
函数结合点的坐标,可以将这些数值直接标注在图上,便于观察和分析。
使用哪些库可以在Python中绘制带有坐标显示的图形?
在Python中,matplotlib
是最常用的绘图库,适合用于创建各种类型的图形,包括折线图、散点图和柱状图等。此外,seaborn
和plotly
等库也提供了强大的绘图功能,能够实现坐标点显示和更多的可视化效果。选择合适的库可以根据具体需求而定。
如何自定义坐标系的格式和外观?
在使用matplotlib
绘图时,可以通过设置plt.xticks()
和plt.yticks()
来自定义坐标轴的刻度和标签。同时,可以使用plt.grid()
来添加网格线,增强图形的可读性。此外,利用plt.title()
、plt.xlabel()
和plt.ylabel()
可以为图形添加标题和坐标轴标签,提升整体的视觉效果。
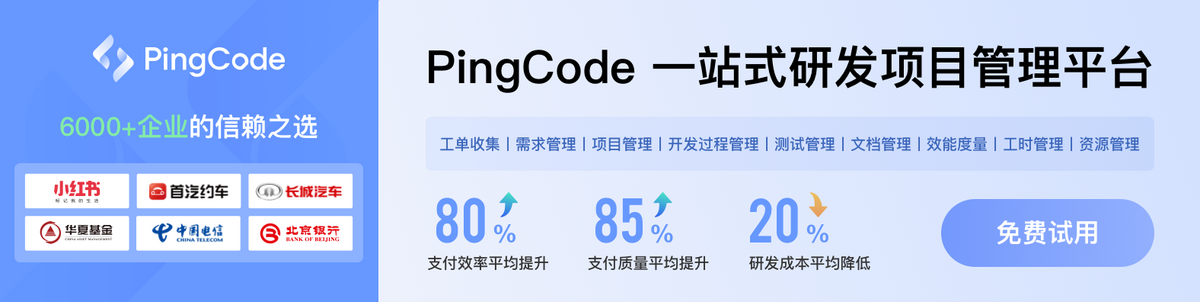