Python中比较两个字符串是否相等的方法有多种,包括使用==运算符、is运算符、locale模块、以及字符串的标准化处理。 其中,最常用且直观的方法是使用==运算符。==运算符 比较的是两个字符串的内容是否相同,而 is运算符 比较的是两个字符串的内存地址是否相同。为了处理特殊字符和不同编码的字符串,locale模块 和字符串的标准化处理也非常重要。 下面将详细介绍这些方法的使用场景和注意事项。
一、使用==运算符
基本用法
在Python中,==运算符用于比较两个字符串的内容是否相同。它是最常用的字符串比较方法,因为它简单且直观。
str1 = "hello"
str2 = "hello"
if str1 == str2:
print("The strings are equal.")
else:
print("The strings are not equal.")
注意事项
- 大小写敏感:==运算符是大小写敏感的,这意味着 "Hello" 和 "hello" 被视为不同的字符串。
- 空格:空格也是字符串的一部分,因此 "hello " 和 "hello" 是不同的字符串。
str1 = "Hello"
str2 = "hello"
if str1 == str2:
print("The strings are equal.")
else:
print("The strings are not equal.")
二、使用is运算符
基本用法
is运算符用于比较两个字符串是否是同一个对象(即它们的内存地址是否相同)。在大多数情况下,不推荐使用is运算符来比较字符串内容。
str1 = "hello"
str2 = "hello"
if str1 is str2:
print("The strings are the same object.")
else:
print("The strings are not the same object.")
注意事项
- 不可变性:Python中的字符串是不可变的,因此字符串常量通常会被重用,这可能导致is运算符判断两个不同变量为同一个对象。
- 不同对象:尽管字符串内容相同,但如果它们是通过不同的方式创建的,is运算符可能会返回False。
str1 = "hello"
str2 = "hello"
str3 = ''.join(['h', 'e', 'l', 'l', 'o'])
if str1 is str2:
print("str1 and str2 are the same object.")
else:
print("str1 and str2 are not the same object.")
if str1 is str3:
print("str1 and str3 are the same object.")
else:
print("str1 and str3 are not the same object.")
三、使用locale模块
基本用法
locale模块提供了一种根据区域设置进行字符串比较的方法。它可以用于处理不同语言和文化背景下的字符串比较。
import locale
locale.setlocale(locale.LC_ALL, 'en_US.UTF-8')
str1 = "hello"
str2 = "hello"
if locale.strcoll(str1, str2) == 0:
print("The strings are equal according to the locale.")
else:
print("The strings are not equal according to the locale.")
注意事项
- 区域设置:在使用locale模块之前,必须设置适当的区域设置。不同的区域设置可能会影响字符串比较的结果。
- 性能:locale.strcoll相比==运算符可能更慢,因为它需要考虑区域设置和字符编码。
四、字符串标准化处理
基本用法
有时,两个字符串的内容可能相同,但由于字符编码或其他原因,它们可能被认为是不同的。在这种情况下,可以使用unicodedata模块进行字符串标准化处理。
import unicodedata
str1 = "café"
str2 = "cafe\u0301" # "é" can be represented as "e" + "´"
str1_normalized = unicodedata.normalize('NFC', str1)
str2_normalized = unicodedata.normalize('NFC', str2)
if str1_normalized == str2_normalized:
print("The strings are equal after normalization.")
else:
print("The strings are not equal after normalization.")
注意事项
- 标准化形式:unicodedata.normalize函数支持多种标准化形式(NFC、NFD、NFKC、NFKD),选择适当的标准化形式非常重要。
- 字符编码:标准化处理有助于解决由于字符编码导致的字符串比较问题。
五、忽略大小写和空格的字符串比较
基本用法
有时,我们需要忽略字符串的大小写和空格来进行比较。在这种情况下,可以使用lower()或upper()方法将字符串转换为相同的大小写,并使用strip()方法去除空格。
str1 = " Hello "
str2 = "hello"
if str1.strip().lower() == str2.strip().lower():
print("The strings are equal after ignoring case and spaces.")
else:
print("The strings are not equal after ignoring case and spaces.")
注意事项
- 预处理:在进行比较之前,必须对字符串进行预处理(如去除空格和转换大小写)。
- 性能:预处理操作可能会增加一些性能开销,特别是对于长字符串。
六、正则表达式
基本用法
正则表达式提供了一种强大的字符串匹配和比较工具。它允许我们使用模式匹配来比较字符串。
import re
str1 = "hello"
str2 = "hello"
pattern = re.compile(re.escape(str2), re.IGNORECASE)
if pattern.fullmatch(str1):
print("The strings match the pattern.")
else:
print("The strings do not match the pattern.")
注意事项
- 复杂性:正则表达式语法可能比较复杂,不适合简单的字符串比较。
- 性能:正则表达式匹配操作可能比简单的==运算符更慢。
七、总结
在Python中比较两个字符串是否相等的方法有很多,每种方法都有其适用的场景和注意事项。==运算符 是最常用的方法,适用于大多数情况。is运算符 用于比较对象的内存地址,但不推荐用于字符串内容比较。locale模块 和 字符串标准化处理 则适用于处理不同语言和字符编码的字符串比较。此外,忽略大小写和空格的比较 以及 正则表达式 也提供了灵活的字符串比较方法。在实际应用中,选择适当的方法取决于具体的需求和场景。
相关问答FAQs:
如何在Python中判断两个字符串是否完全相同?
在Python中,可以使用==
运算符来判断两个字符串是否相等。如果两个字符串的内容完全一致,结果将返回True
,否则返回False
。例如,"hello" == "hello"
的结果为True
,而"hello" == "Hello"
则为False
,因为大小写不同。
在字符串比较时,Python是否区分大小写?
是的,Python在比较字符串时会区分大小写。这意味着字符串"abc"
与"ABC"
被认为是不相等的。如果希望忽略大小写进行比较,可以将两个字符串都转换为小写或大写,例如使用str.lower()
或str.upper()
方法。
在比较字符串相等时,如何处理空字符串?
空字符串在Python中被表示为""
。两个空字符串是相等的,"" == ""
的结果为True
。当进行字符串比较时,务必考虑到空字符串的情况,以确保逻辑的正确性。例如,比较一个字符串与空字符串时,只有当该字符串确实为空时,比较结果才会为True
。
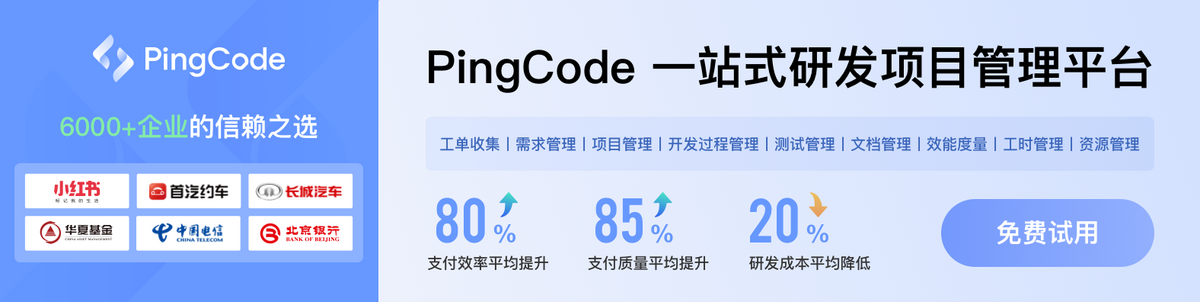