Python在函数中调用另一个函数的方法有以下几种:直接调用、使用函数作为参数、嵌套函数。直接调用是最简单的方法,下面我们来详细解释这一点。
在Python中,函数是第一类对象(First-Class Objects),这意味着函数可以作为参数传递、作为变量赋值、作为返回值、以及在其他函数中调用。直接调用另一个函数只需要在函数体内使用该函数的名字和参数即可。这种方法简单直观,适用于大多数场景。接下来,我们会深入探讨不同的方法和具体的代码示例。
一、直接调用
直接调用是最常见且简单的方法。例如:
def greet():
return "Hello, World!"
def display_message():
message = greet()
print(message)
display_message()
在这个例子中,display_message
函数内部直接调用了greet
函数,并将其返回值赋给变量message
,然后打印出来。
二、使用函数作为参数
Python允许将一个函数作为参数传递给另一个函数,这种方法在需要执行不同的操作时非常有用。例如:
def add(x, y):
return x + y
def subtract(x, y):
return x - y
def operate(func, x, y):
return func(x, y)
print(operate(add, 5, 3)) # 输出: 8
print(operate(subtract, 5, 3)) # 输出: 2
在这个例子中,operate
函数接受另一个函数func
作为参数,并对x
和y
执行func
函数。
三、嵌套函数
嵌套函数是指在一个函数内部定义另一个函数,这在需要封装逻辑或闭包时很有用。例如:
def outer_function(text):
def inner_function():
print(text)
inner_function()
outer_function("Hello from the inner function!")
在这个例子中,inner_function
被定义在outer_function
内部,并在outer_function
内部被调用。
四、递归调用
递归调用是函数调用自身的一种特殊形式,适用于解决分而治之的问题,如二分查找、快速排序等。例如:
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n-1)
print(factorial(5)) # 输出: 120
在这个例子中,factorial
函数调用自身来计算阶乘。
五、使用装饰器
装饰器是一种高级用法,允许在一个函数的前后执行额外的代码。例如:
def my_decorator(func):
def wrapper():
print("Something is happening before the function is called.")
func()
print("Something is happening after the function is called.")
return wrapper
@my_decorator
def say_hello():
print("Hello!")
say_hello()
在这个例子中,my_decorator
函数接受另一个函数func
作为参数,并返回一个新的函数wrapper
,该函数在执行func
前后添加了一些额外的代码。
六、闭包
闭包是指一个函数可以记住定义它时的环境变量,即使这个环境在函数调用时已经不存在。例如:
def make_multiplier_of(n):
def multiplier(x):
return x * n
return multiplier
times3 = make_multiplier_of(3)
times5 = make_multiplier_of(5)
print(times3(9)) # 输出: 27
print(times5(3)) # 输出: 15
print(times3(times5(2))) # 输出: 30
在这个例子中,make_multiplier_of
函数返回一个新的multiplier
函数,该函数记住了n
的值。
七、使用lambda函数
lambda函数是一种简洁的匿名函数,用于定义短小的函数。例如:
def high_order_function(f, x):
return f(x)
result = high_order_function(lambda x: x * x, 5)
print(result) # 输出: 25
在这个例子中,lambda x: x * x
是一个匿名函数,传递给high_order_function
并执行。
八、闭包与装饰器结合
闭包和装饰器可以结合使用,创建更加灵活和强大的函数。例如:
def decorator_with_args(decorator_arg1, decorator_arg2):
def decorator(func):
def wrapper(*args, kwargs):
print(f"Decorator arguments: {decorator_arg1}, {decorator_arg2}")
return func(*args, kwargs)
return wrapper
return decorator
@decorator_with_args("arg1", "arg2")
def say_hello():
print("Hello!")
say_hello()
在这个例子中,decorator_with_args
函数生成一个装饰器,该装饰器可以接受参数,并应用于say_hello
函数。
通过以上各种方法,您可以在Python中灵活地在一个函数中调用另一个函数,根据具体需求选择最合适的方法,从而实现代码的复用和逻辑的封装。
相关问答FAQs:
如何在Python中定义和调用一个函数?
在Python中,定义一个函数使用def
关键字。可以通过函数名和括号来调用它。例如,定义一个简单的函数如下:
def greet(name):
return f"Hello, {name}!"
要调用这个函数,可以使用:greet("Alice")
,它将返回"Hello, Alice!"
。
在一个函数中调用另一个函数的最佳实践是什么?
在一个函数中调用另一个函数时,确保被调用的函数已经定义。此外,保持函数的功能单一是一个好习惯,这样可以提高代码的可读性和可维护性。例如:
def add(a, b):
return a + b
def calculate_sum(x, y):
return add(x, y)
通过这种方式,calculate_sum
函数利用了add
函数来完成任务。
如何处理函数调用中的参数传递?
当在一个函数中调用另一个函数时,可以直接传递参数。确保参数的数量和类型匹配。如果需要传递可变数量的参数,可以使用*args
和**kwargs
语法。例如:
def multiply(a, b):
return a * b
def calculate_product(*args):
product = 1
for num in args:
product = multiply(product, num)
return product
在这个例子中,calculate_product
函数能够接受任意数量的参数并通过multiply
函数计算它们的乘积。
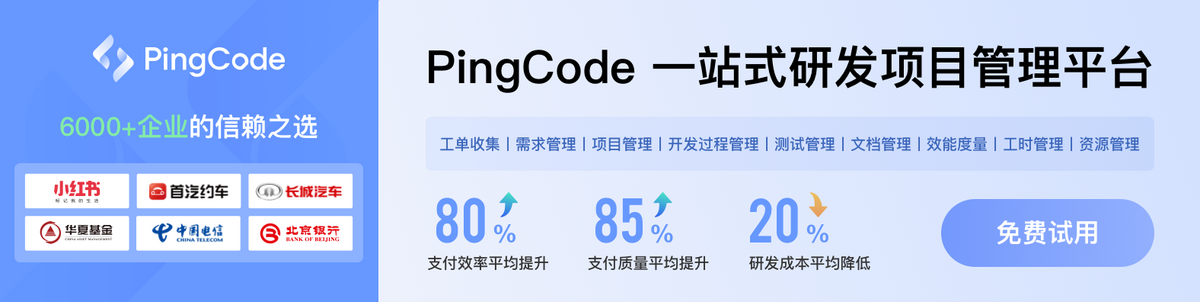